Simple Load and Export
HOOPS Exchange can be used within your application in two different ways - as a direct or indirect integration.
A direct integration means you are accessing the assembly tree structure, parsing entities, and using that information to inspect the model data or otherwise provide a feature-rich experience to the end user. For information on how you would do this, see Reading Model Geometry.
An indirect integration means you are simply reading a model file and using HOOPS Exchange to write it in a different format without reading the assembly tree.
Start HOOPS Exchange by initializing the libraries and setting the license key. That process is described in the initialization section.
Once HOOPS Exchange is initialized, you can use it to load a CAD model into memory. CAD files can be imported with a minimal amount of code:
A3DSDKHOOPSExchangeLoader sHoopsExchangeLoader(myFilename);
A3DStatus importResult = sHoopsExchangeLoader.Import(A3DImport(filename));
If you’re doing an indirect integration, you’re done. You can export the file as another format by using the code in the next subsection.
For direct integrations, you’ll need access to the model file pointer. This pointer gives you a reference to the root of the assembly tree:
A3DAsmModelFile* myModelPtr = sHoopsExchangeLoader.m_psModelFile;
Note
While reading CAD data, HOOPS Exchange identifies the format and version. If the version is not supported by our reader, the associated error code is sent back:
A3D_LOAD_FILE_TOO_OLD if the input format is too old for HOOPS Exchange
A3D_LOAD_FILE_TOO_RECENT if the input format is too recent for HOOPS Exchange
The File Formats page details the list of supported versions for each format.
Loading a CAD File With Custom Options
Many file formats contain extraneous data that is not necessary for all situations. Therefore, before loading the file, you should set the file import parameters to load only the specific data required by your application. Doing so decreases the memory footprint, CPU load, and load time. These parameters are set using the ref A3DRWParamsLoadData structure:
A3DRWParamsLoadData sReadParam;
A3D_INITIALIZE_DATA(A3DRWParamsLoadData, sReadParam);
sReadParam.m_sGeneral.m_bReadSolids = true;
sReadParam.m_sGeneral.m_bReadSurfaces = true;
sReadParam.m_sGeneral.m_bReadPmis = true;
// ... set other A3DRWParamsLoadData fields as necessary
A3DAsmModelFile* pModelFile = NULL;
A3DAsmModelFileLoadFromFile("output.3.prc", &sReadParam, &pModelFile);
// alternatively, if you've used the A3DSDKHOOPSExchangeLoader:
A3DAsmModelFileGet(sHoopsExchangeLoader.m_psModelFile, &modelFileData);
The end result of successful file load is a model file pointer. This structure represents the root entity for a model file. Through it, you can access the assembly tree. There is only one model file per model.
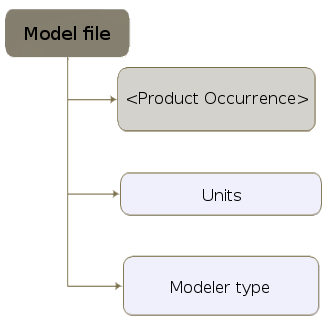
Once in memory, the model is represented by a PRC assembly tree that is equivalent to its native structure. Note that pModelFile points to a model file node. Nodes always have data associated with them, but this data is not populated by default. The following code sample fills the node’s data structure so that you can access the information within:
A3DAsmModelFileData modelFileData; // will contain node data
A3D_INITIALIZE_DATA(A3DAsmModelFileData, modelFileData);
A3DAsmModelFileGet(pModelFile, &modelFileData);
At this point, the model file is loaded into memory and you can use the modelFileData structure to access the assembly tree. As noted above, this is the route you would take to perform a direct integration. For information on how you would do this, see Reading Model Geometry.
A PRC assembly tree can contain any number of nodes, and in many cases, they will be nested several levels deep. In order to gather all the information from the PRC, your traversal algorithm must visit every node. Typically, this is done through recursion.
Incremental Loading
With incremental load, it’s possible to load specific parts into memory without loading extraneous parts. More precisely, Exchange will load the full assembly structure, the product occurrences, but only the geometry and tessellation of the specified parts, thereby reducing the overhead of the application and boosting relative performance. This is especially useful for large CAD models.
Incremental load is only available for certain file formats, including SolidWorks, NX (Unigraphics), Creo (Pro/E), JT and CATIA V5. Incremental load support is specified on the information pages for most formats. A full code example of incremental load is available in Incremental Load, and a working demo of incremental mode can be evaluated by opening a supported CAD model in the HOOPS Demo Viewer. Instructions for loading a model in the HOOPS Demo Viewer in incremental mode are available in the HOOS Demo Viewer docs.
The following code example demonstrates a simple example of an incremental load of a single part:
// Load the assembly structure only
A3DImport sImport(path_to_CAD_file);
sImport.m_sLoadData.m_sIncremental.m_bLoadStructureOnly = true;
A3DStatus iRet = sHoopsExchangeLoader.Import(sImport);
// Here, you would traverse the assembly tree until you locate the leaf node you want to load...
// ...and store it in pMyProductOccurrence and its containing product occurrence in pRoot.
// For a detailed walkthrough, see the Incremental Load example in the package.
// Initialize our root and leaf product occurrence data
A3DAsmProductOccurrenceData sRootProductOccurrenceData;
A3D_INITIALIZE_DATA(A3DAsmProductOccurrenceData, sRootProductOccurrenceData);
CHECK_RET(A3DAsmProductOccurrenceGet(pRoot, &sRootProductOccurrenceData));
A3DAsmProductOccurrenceData sProductOccurrenceData;
A3D_INITIALIZE_DATA(A3DAsmProductOccurrenceData, sProductOccurrenceData);
CHECK_RET(A3DAsmProductOccurrenceGet(pMyProductOccurrence, &sProductOccurrenceData));
// Set options
sImport.m_sLoadData.m_sGeneral.m_eReadGeomTessMode = kA3DReadGeomAndTess;
sImport.m_sLoadData.m_sIncremental.m_bLoadStructureOnly = false;
sImport.m_sLoadData.m_sIncremental.m_ppProductOccurrences = &pMyProductOccurrence;
sImport.m_sLoadData.m_sIncremental.m_pRootProductOccurrence = pRoot;
sImport.m_sLoadData.m_sIncremental.m_uiProductOccurrencesSize = 1;
sHoopsExchangeLoader.Import(sImport);
In this example, pMyProductOccurrence is a pointer to a leaf node containing geometry and/or tessellation. The variable pRoot is the Product Occurrence containing the leaf node pMyProductOccurrence.
After these variables are initialized, the options for the incremental load are set via the m_sLoadData member, which is an object of type A3DRWParamsIncrementalLoadData
.
Although we’re only loading one leaf node in our example above, it is also possible to load more than one leaf node at a single time.
Limitation: only leaf nodes can be loaded. It’s not possible to load assembly geometries, assembly PMIs or views with the Incremental load workflow. Trying to load assembly product occurrence will return A3D_LOAD_INVALID_PARAMETERS_FOR_INCREMENTAL_LOAD.
Once a part has been loaded, it can subsequently be unloaded with a call to the function A3DAsmModelFileUnloadParts()
.
Please note, CAD views are not supported in incremental mode. Metadata for JT format are not supported in incremental mode.
Loading a PDF Model
A PDF file can potentially contain multiple 3D data streams.
Therefore, simply using A3DAsmModelFileLoadFromFile()
or A3DAsmModelFileGet()
will not work because those functions can only handle a single data stream.
To load a PDF, you need to use A3DGet3DPDFStreams()
.
PDF data can be either in PRC or U3D format.
If the data is PRC, you can load it from memory.
If U3D, you must write the data to a temporary file before reading it using A3DPDF3DStreamCreateFromFile()
.
A3DStream3DPDFData* pStream3DPDFData;
A3DInt32 iNumStreams;
A3DGet3DPDFStreams((char*) pcFileName, &pStream3DPDFData, &iNumStreams);
// a real use case might parse every stream in the input file. Here, we just take the first one (pStream3DPDFData[0]).
if (pStream3DPDFData[0].m_bIsPrc) // test whether the data is PRC or U3D
{
// even an input PRC file must be read in memory and mapped into modelfile data structures
A3DRWParamsPrcReadHelper* pPrcReadHelper;
A3DAsmModelFileLoadFromPrcStream(pStream3DPDFData[0].m_pcStream, pStream3DPDFData[0].m_iLength, &pPrcReadHelper, ppModelFile);
}
else
{
// A U3D stream must first be stored as a physical file, then read by A3DAsmModelFileLoadFromFile
sprintf(tempfilename, "%s.u3d", OUT_FILE);
FILE * pFile = fopen(tempfilename , "wb");
fwrite(pStream3DPDFData[0].m_pcStream, 1, pStream3DPDFData[0].m_iLength , pFile);
fclose(pFile);
// physical file to stream
A3DAsmModelFileLoadFromFile(tempfilename, sReadParam, ppModelFile);
// the temp file can be disposed of afterwards
remove(tempfilename);
}
Exporting your model is similar to importing - simply reverse the procedure. You need only three things: the model file pointer, the export parameters structure, and the target filename. The supported export formats can be found here.
Indirect Integration With the HOOPSExchangeLoader
Exporting a file with the HOOPSExchangeLoader
is the preferred method when you are doing an indirect integration.
Simply specify the filename of the import file and the export file.
The export file type is determined by the file extension, so you should use a known file extension for the file format.
A3DImport sImport(importFilename);
A3DExport sExport(exportFilename);
A3DStatus iRet = sHoopsExchangeLoader.Convert(sImport, sExport);
Direct Integration
Export options vary widely between file types. However, all export data structures have the following name:
A3DRWParamsExport*Data
…where * is substituted with the file type.
Suppose you are exporting a STEP file.
Configure a A3DRWParamsExportStepData
data structure to suit your needs.
Then, to export your model, you would do something like this:
A3DRWParamsExportStepData sParamsExportData;
A3D_INITIALIZE_DATA(A3DRWParamsExportStepData, sParamsExportData);
// set export options
sParamsExportData.m_bSaveFacetedToWireframe = true;
sParamsExportData.m_bSaveAnalyticsToNurbs = true;
iRet = A3DAsmModelFileExportToStepFile(pModelFile, &sParamsExportData, pcOutputFileName);
if (iRet != A3D_SUCCESS)
// handle error