Populating Fields
HOOPS Publish has functions to populate fields on a page at creation time. These fields must have been created with Adobe Acrobat and the Forms tool. As an example, visit the pdf_samples directory in the HOOPS Publish package and open any PDF file using Adobe Acrobat. Then edit the file with the Forms tool and the Edit menu. There, you’ll have a view on all the fields contained in the PDF file. Using Acrobat Forms, you can design the PDF page to contain different type of fields. The properties for each of these fields can also be viewed and modified in Adobe Acrobat.
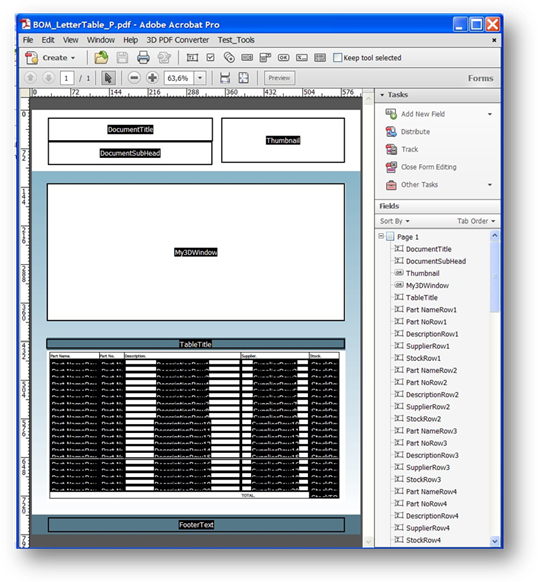
HOOPS Publish populate functions supports text fields or buttons fields. All functions have the same kind of prototype: It always has the field name as an argument to identify the field, as well as an argument to set a value to the field.
Field Names
The fields can be manipulated using different interfaces: Using Acrobat forms tool as described previously, or using JavaScript code, or finally using the HOOPS Publish API to browse fields on a page, or access to a named field.
Using these interfaces, a field can be easily accessed using its name, but there are specific situations:
Radio Buttons
Radio buttons are a specific type of fields where button choices belong to a common group. Then the names are as follows:
- In Acrobat form:
- group field name: “MyGroup”
- button choice name: “MyChoice”
- In Acrobat JavaScript
- JavaScript name for the group: “MyGroup”
- JavaScript name for the radio buttons choices (whatever choice name): “MyGroup.index” (index starting with 0)
- Using
A3DPDFPageGetFields
, thenA3DPDFFieldGetInformation
:
- sFieldData.m_acName = “MyGroup.index”
Copy-pasted field in Acrobat
- In Acrobat form:
- field names: “MyField#index” (index starting with 0)
- In Acrobat JavaScript:
- JavaScript name for the group: “MyField”
- JavaScript name for the fields: “MyField.index” (starting with 0)
- Using
A3DPDFPageGetFields
, thenA3DPDFFieldGetInformation
:
- sFieldData.m_acName = “MyField.index”
Populating a Text Field
Acrobat Forms enables customers to define text fields. With text fields you can predefine a large set of options for the text appearance or the behavior. These options will be respected by the population function. Options are for example the font, the text or background color, the alignment in the field, or a multi-line capability. To populate a text field, use the function A3DPDFPageFieldTextSetValue
. The text value is specified as a UTF-8 string and characters are only restricted to the characters supported by the font selected for the field. The text can have multiple lines, in this case the carriage return character should be ’r’.
iRet = A3DPDFPageFieldTextSetValue(pPage,
"AdditionalText",
"This is an example of a populated text field");
Populating a Field With an Image
Customers can design a PDF page to receive an image. For this, you need to design a button and set the layout option to contain an icon (’icon only’ for example). The image can be stored at the template creation and never change, or can be programmatically filled with the HOOPS Publish function A3DPDFPageFieldButtonSetIcon
. With this function, the value argument is an A3DPDFImage object which has to be created with the function A3DPDFImageCreate
.
A3DPDFImageData sImageData;
A3D_INITIALIZE_DATA(A3DPDFImageData, sImageData);
sImageData.m_pcFileName = "c:\\temp\\myimage.jpg";
sImageData.m_iHeight = 500;
sImageData.m_iWidth = 500;
sImageData.m_eFormat = kA3DPDFImageFormatJpg;
A3DPDFImage* pImage;
iRet = A3DPDFImageCreate(pDoc, &sImageData, &pImage);
iRet = A3DPDFPageFieldButtonSetIcon(pPage, "Logo", pImage);
Setting a Field Visibility
The visibility for a field can be programmatically specified. This enables customers to design a PDF template with a large list of fields and to adapt the list of visible fields at runtime depending on the context.
iRet = A3DPDFPageFieldSetVisibility(pPage, "SupplierRow40", false);
The UserDefinedViews sample shows how to use a PDF file as a template document to be opened and populated with new values.
The PDF file is created using the MultiViews_Letter_P.pdf template which is loaded from the ./samples/publish/publishquickstarts/templates/MultiViews directory.
#define IN_PDFFILE "..\\..\\samples\\publish\\publishquickstarts\\Templates\\MultiViews\\MultiViews_Letter_P.pdf"
// insert page from a template file
A3DPDFPage* pPage = NULL;
// the false boolean keeps the same fields names
iRet = A3DPDFDocumentAppendPageFromPDFFileEx(pDoc, in_pdftemplatefile, false, &pPage);
CHECK_RET;
Once the 3D annotation has been created, we can insert it into the “My3DWindow” button in the PDF template using the object’s name.
// populating the field with the 3D annotation
iRet = A3DPDFPageFieldSet3DAnnot(pPage, "My3DWindow", p3DAnnot);
CHECK_RET;
Similarly, we can populate some title text fields with text values.
First the text field is retrieved on the page with:
iRet = A3DPDFPageGetField(pPage, "DocumentTitle", &pTextDocumentTitle);
CHECK_RET;
Then the text field is populated with:
iRet = A3DPDFTextFieldSetValue(pTextDocumentTitle, "Slattery Business Park");
CHECK_RET;
The result is sample_UserDefinedViews.pdf:
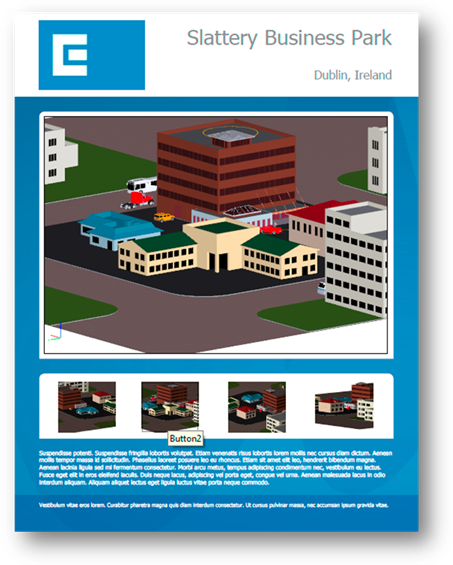