Rendering effects
The MFC Sandbox has a few different rendering modes that can be enabled: shadows, hidden line, smooth, and frame rate.
The shadow method available is called “simple shadow”. This is not a ray-traced or light-interpolated shadow - it is a visual effect created by texturing. Enabling simple shadows is done on the HPS::View object using the HPS::VisualEffectsControl:
void CHPSView::OnModesSimpleShadow()
{
// Toggle state and bail early if we're disabling
_enableSimpleShadows = !_enableSimpleShadows;
if (_enableSimpleShadows == false)
{
GetCanvas().GetFrontView().GetSegmentKey().GetVisualEffectsControl().SetSimpleShadow(false);
_canvas.Update();
return;
}
UpdatePlanes();
}
Shadow maps are also available…please see this section for more information about shadows.
“Smooth” is a render mode that enables Phong rendering:
void CHPSView::OnModesSmooth()
{
if (!_smoothRendering)
{
_canvas.GetFrontView().SetRenderingMode(Rendering::Mode::Phong);
if (GetCHPSDoc()->GetCADModel().Type() == HPS::Type::DWGCADModel)
_canvas.GetFrontView().GetSegmentKey().GetVisibilityControl().SetLines(true);
_canvas.Update();
_smoothRendering = true;
}
}
The “Framerate” icon refers to the “fixed-framerate” mode of HNP. In this mode, the number of frames drawn per second is capped at a specified value. In this case, we are capping the framerate at 20:
void CHPSView::OnModesFrameRate()
{
const float frameRate = 20.0f;
// Toggle frame rate and set. Note that 0 disables frame rate.
_enableFrameRate = !_enableFrameRate;
if (_enableFrameRate)
_canvas.SetFrameRate(frameRate);
else
_canvas.SetFrameRate(0);
// fixed framerate is not compatible with hidden line mode
if(!_smoothRendering)
{
_canvas.GetFrontView().SetRenderingMode(Rendering::Mode::Phong);
_smoothRendering = true;
}
_canvas.Update();
}
This screenshot shows shadows, frame rate, and Phong rendering all enabled:
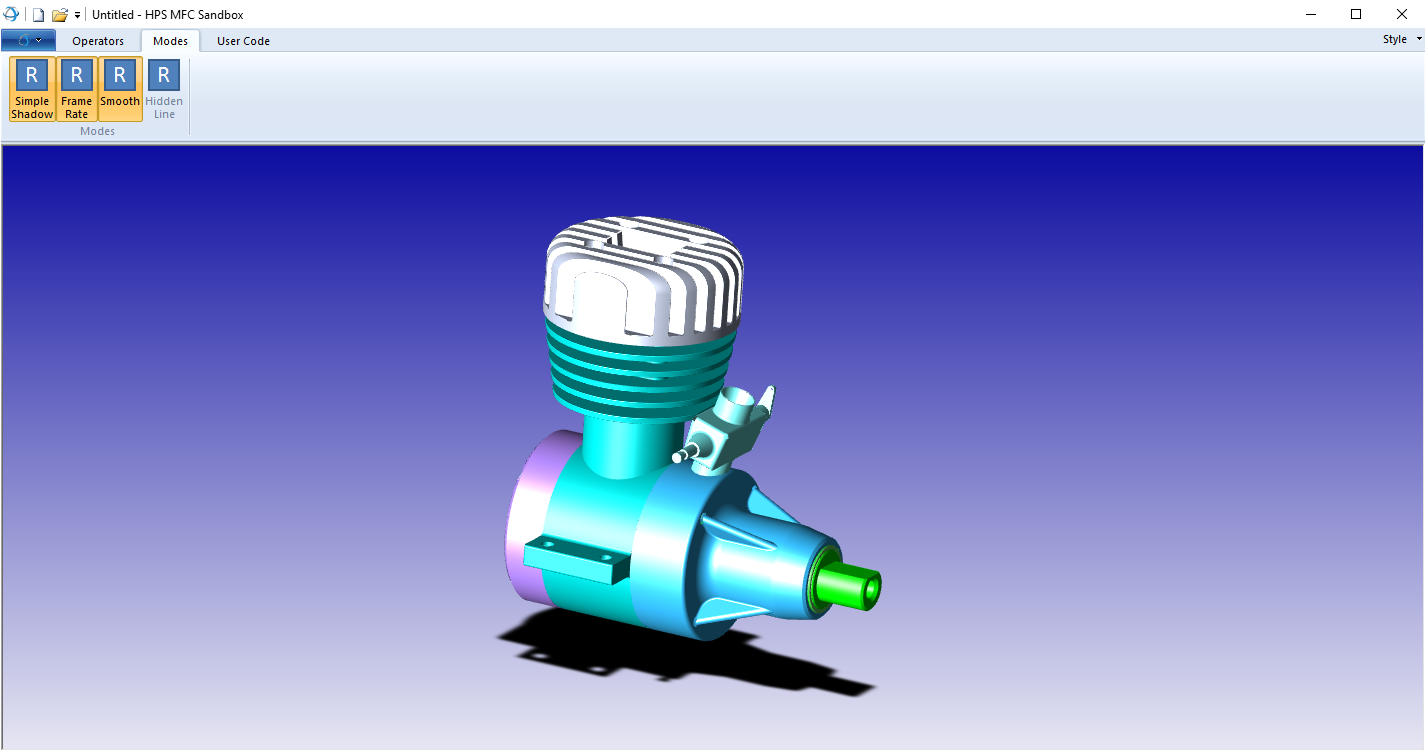
Hidden line rendering (HLR) is a rendering mode that draws edges visible from the camera location (as opposed to a wireframe rendering which draws all edges regardless of whether they are behind other geometry). Hidden line rendering is enabled in the following way:
void CHPSView::OnModesHiddenLine()
{
if (_smoothRendering)
{
_canvas.GetFrontView().SetRenderingMode(Rendering::Mode::FastHiddenLine);
_canvas.Update();
_smoothRendering = false;
// fixed framerate is not compatible with hidden line mode
if (_enableFrameRate)
{
_canvas.SetFrameRate(0);
_enableFrameRate = false;
}
}
}
This screenshot shows shadows and HLR enabled, but notice frame rate has been automatically disabled. Additionally, even though shadows are enabled, we don’t see them because the geometry’s faces are not being rendered, and lines cast no shadow:
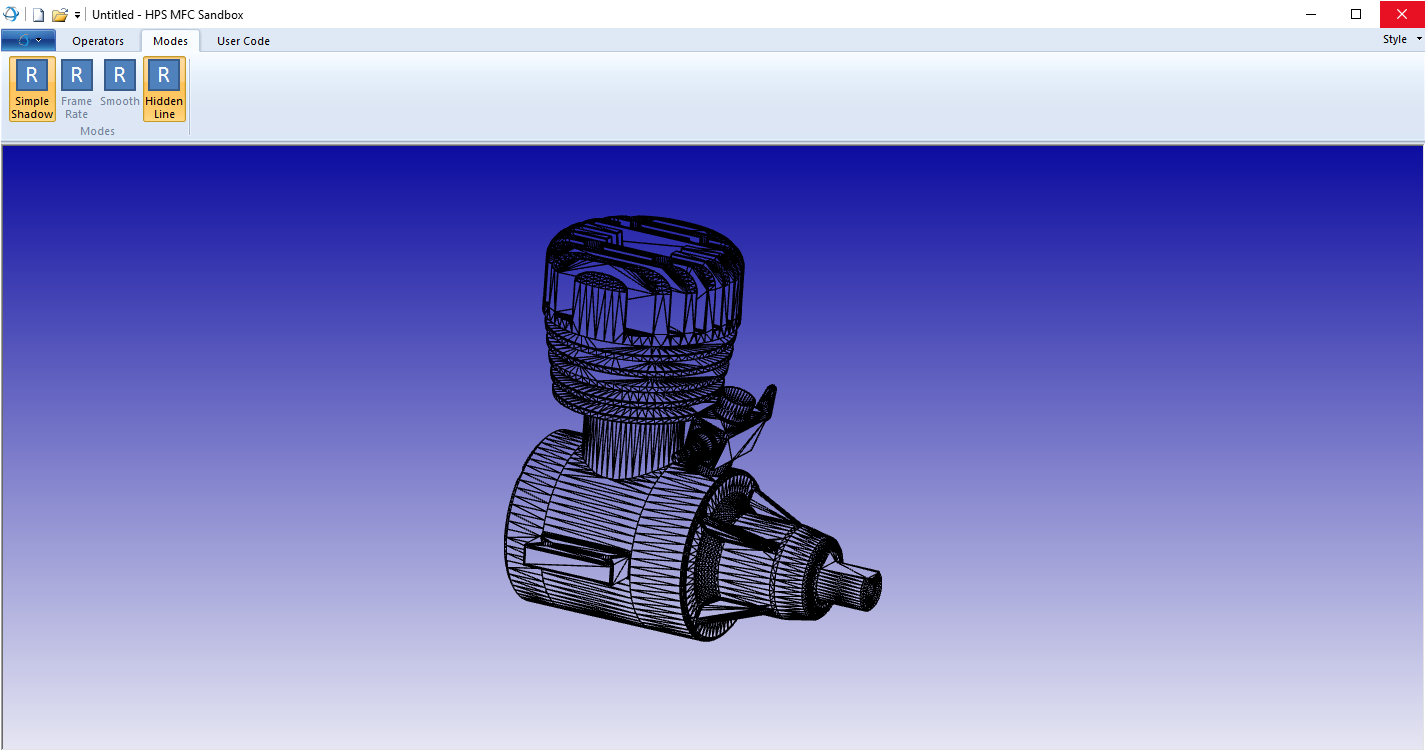
Note that if fixed framerate mode is enabled at this point, it will be automatically disabled because it is not compatible with HLR. More information on hidden line rendering is available here.