Running user code
Some of the sandbox applications come with “User Code” buttons:
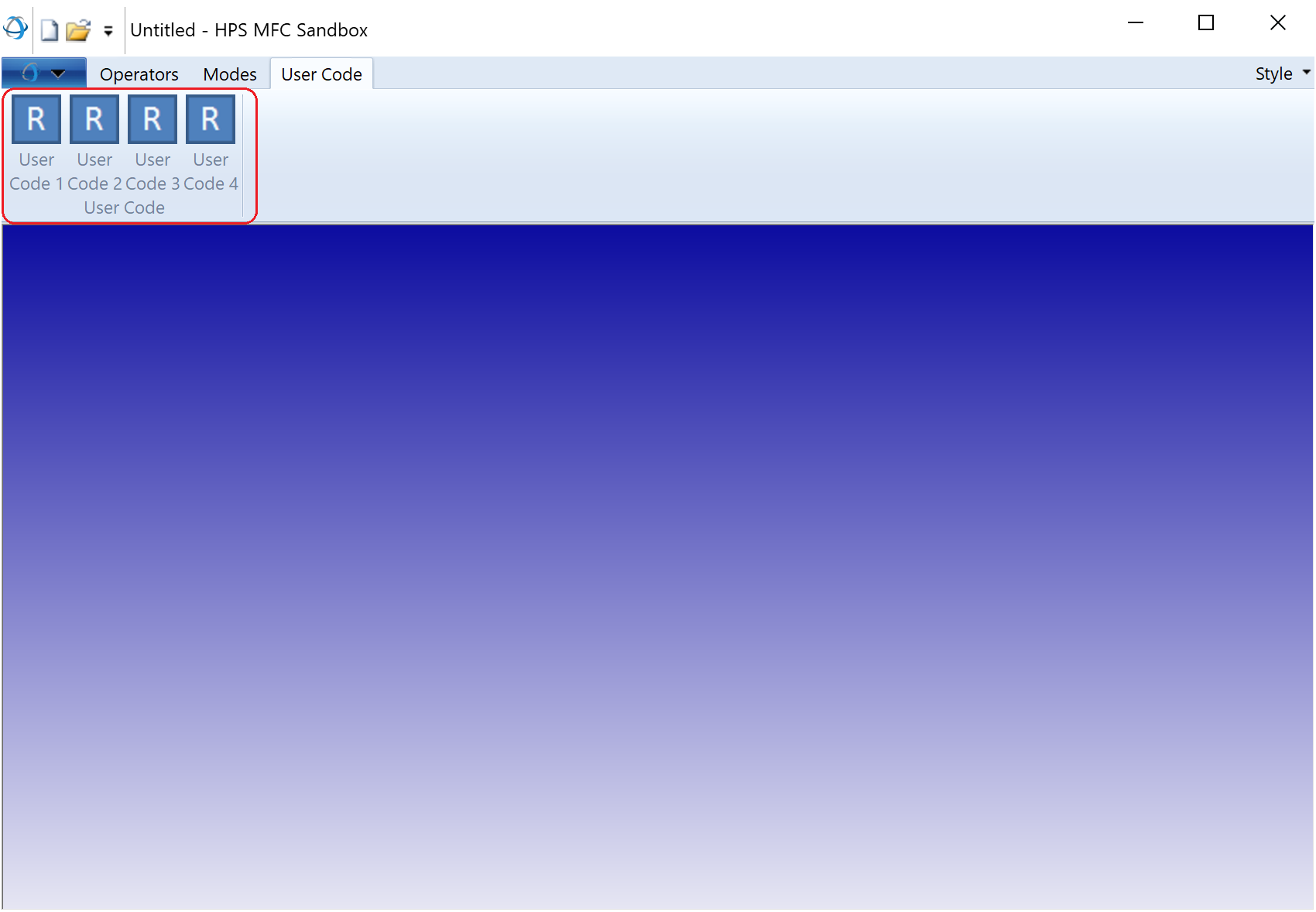
When pressed, each button will call one of four customizable functions in CHPSView.cpp. In the code snippet below, you can see that the first user code button will cause the resource monitor to appear:
void CHPSView::OnUserCode1()
{
// Toggle display of resource monitor using the DebuggingControl
_displayResourceMonitor = !_displayResourceMonitor;
_canvas.GetWindowKey().GetDebuggingControl().SetResourceMonitor(_displayResourceMonitor);
GetCanvas().Update();
}
void CHPSView::OnUserCode2()
{
// TODO: Add your command handler code here
}
void CHPSView::OnUserCode3()
{
// TODO: Add your command handler code here
}
void CHPSView::OnUserCode4()
{
// TODO: Add your command handler code here
}
You can put your own code into these functions to run small snippets within the context of the sandbox. This is valuable because it enables you to quickly prototype or test functionality without having to write an entire application. In order to do this, you will have to recompile the sandbox.
To start writing code, simply obtain a reference to the four component hierarchy objects:
HPS::Canvas canvas = GetCanvas();
HPS::Layout layout = canvas.GetAttachedLayout();
HPS::View view = layout.GetFrontView();
HPS::Model model = view.GetAttachedModel();
Each view hierarchy object has a HPS::SegmentKey
associated with it, which can be obtained by calling GetSegmentKey
(or GetWindowKey
in the case of the Canvas
). From here, you can manipulate the segment tree, import and export models, experiment with settings, and more.
For example, geometry would typically go in the model segment, so you can specify or import geometry here as needed. Importing whole models is slightly different in that the import process creates a HPS::CADModel
object which can be used in place of the HPS::Model
, as shown below:
void CHPSView::OnUserCode1()
{
HPS::Canvas canvas = GetCanvas();
HPS::Layout layout = canvas.GetAttachedLayout();
HPS::View view = layout.GetFrontView();
// ... other import setup code goes here - see section on "Importing" for details
Notifier notifier = HPS::Exchange::File::Import(filename, options);
CADModel cadModel = notifier.GetCADModel();
view.AttachModel(cadModel);
canvas.Update();
}
The above code snippet is just one general example of how models can be imported. There are a few different ways to accomplish this, but the most important concept to understand is the relationship between the view hierarchy segments.
You can also load a model into the MFC Sandbox by simply using File > Open. Whether you load via the menu or programmatically, once you have a model on the screen, you can use the other user code functions to run HOOPS code to operate on the model.
Next steps
When you are ready to investigate a more complex application, please move on to the BIM Viewer tutorial, which describes a common workflow for loading and displaying BIM models.