Segment and model browsers
The segment browser is a visual representation of the in-memory scene graph database. Activate it by clicking the “Segment Browser” icon in the ribbon menu.
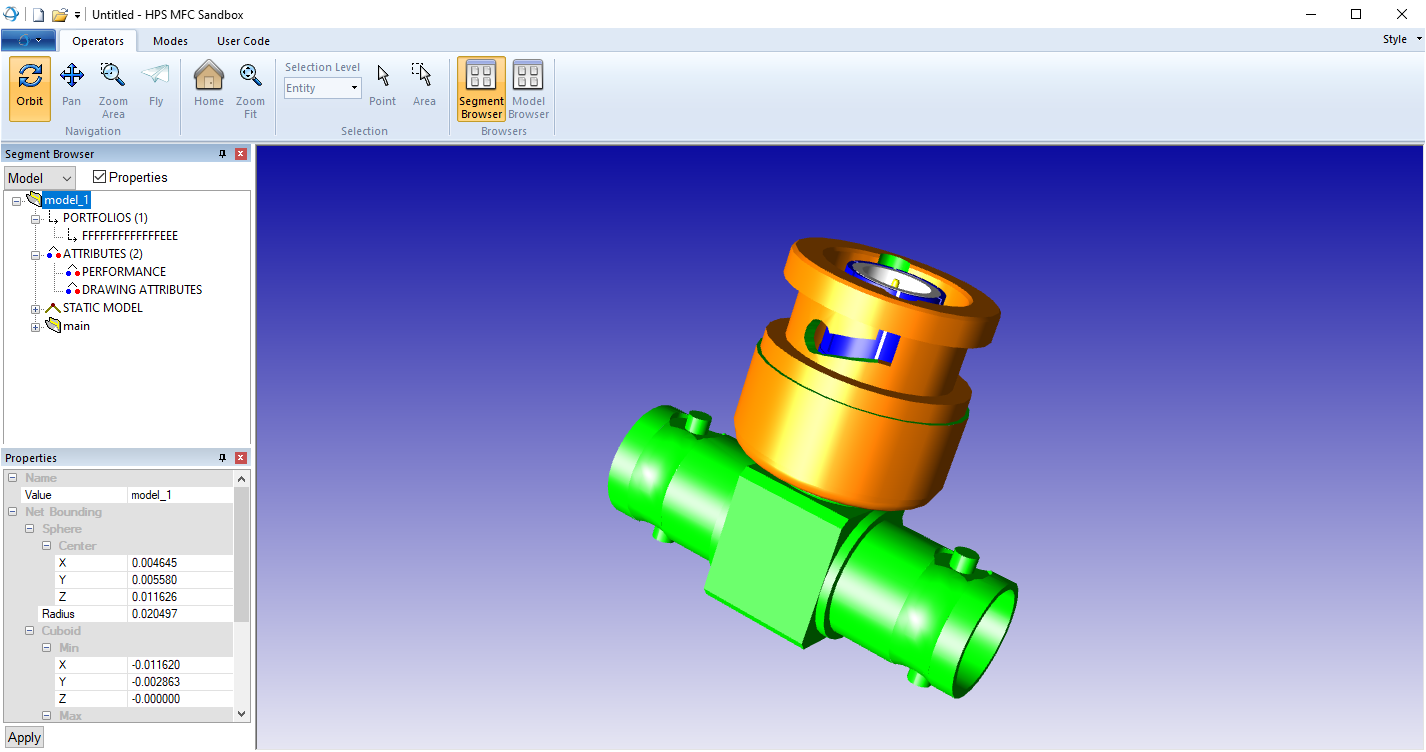
At the top left corner of the segment browser window, you’ll notice a drop down list which contains the four view hierarchy components discussed earlier. By selecting the “Canvas” menu item and expanding its tree, you’ll see how the view hierarchy is organized in memory. You’ll also see the various attributes, portfolios, includes, and geometry at various locations in the tree.
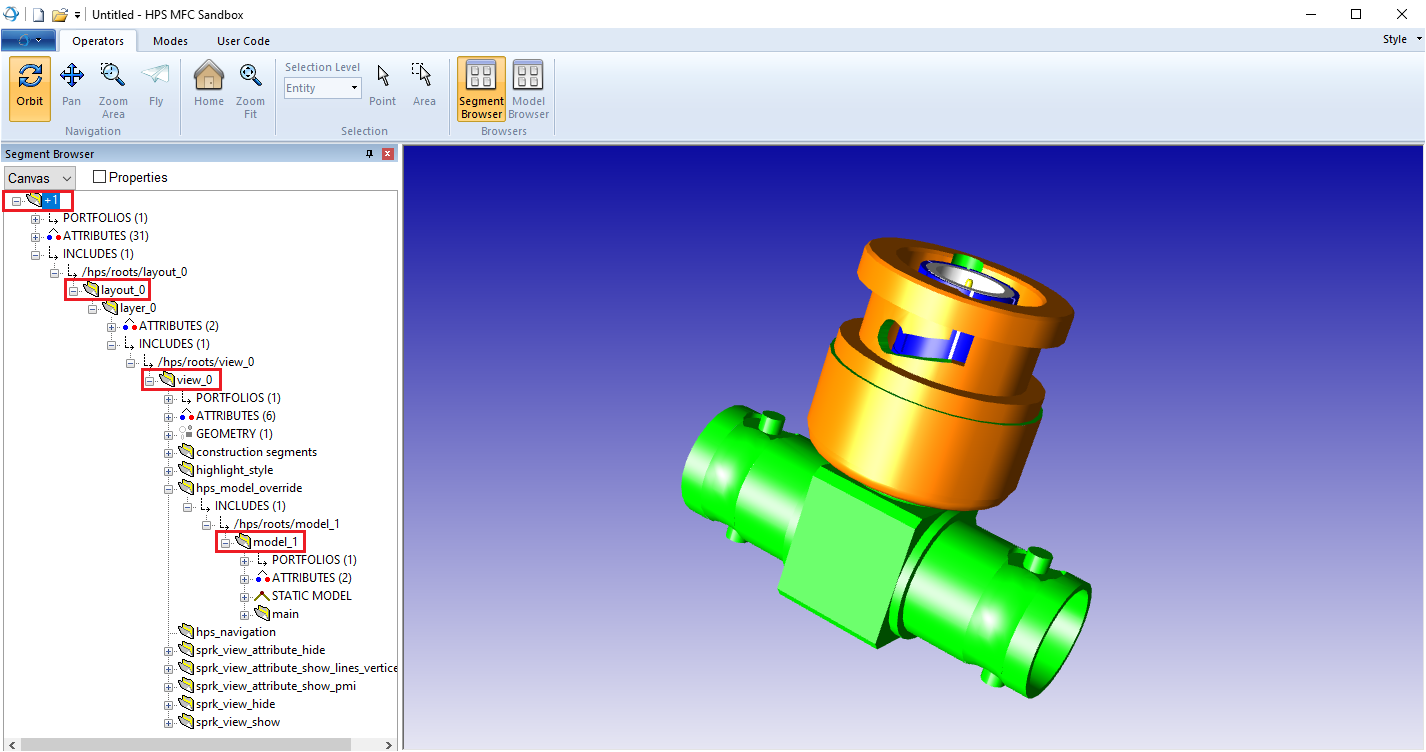
You can build a similar segment browser tree using the HPS::SceneTree and HPS::SceneTreeItem classes. These classes have members related to the HNP API and act as base classes when creating a segment tree in a GUI toolkit. The MFC Sandbox implementation can be used as an example. In the sandbox, CDockablePane
is the MFC base class behind the segment browser window. In CHPSSegmentBrowserPane.h, you’ll see CHPSSegmentBrowserPane
derives that base class and also has a reference to the view hierarchy drop-down menu, the MFCSceneTree
object itself, and others:
class CHPSSegmentBrowserPane : public CDockablePane
{
public:
CHPSSegmentBrowserPane();
virtual ~CHPSSegmentBrowserPane();
void Init();
void AdjustLayout();
protected:
CHPSTreeCtrl m_TreeCtrl;
CImageList m_ImageList;
CComboBox m_ComboBox;
CFont m_Font;
CButton m_CheckBox;
std::shared_ptr<MFCSceneTree> m_pSceneTree;
virtual BOOL OnShowControlBarMenu(CPoint pt);
afx_msg int OnCreate(LPCREATESTRUCT lpCreateStruct);
afx_msg void OnSize(UINT nType, int cx, int cy);
afx_msg void OnPaint();
afx_msg void OnSetFocus(CWnd * pOldWnd);
afx_msg void OnItemExpanding(NMHDR * pNMHDR, LRESULT * pResult);
afx_msg void OnSelectionChanged(NMHDR * pNMHDR, LRESULT * pResult);
afx_msg void OnProperitesCheckBox();
afx_msg void OnUpdatePropertiesCheckBox(CCmdUI *pCmdUI);
afx_msg void OnSelectedRoot();
DECLARE_MESSAGE_MAP()
};
In our implementation, HPS::SceneTree is the parent of MFCSceneTree
, which has its own GUI-dependent code. Similarly, MFCSceneTreeItem
derives from HPS::SceneTreeItem. In this way, the HNP-specfic code can be integrated into any system with a similar GUI control. If you happen to be building an MFC application, you can use this example as a starting point in your own code. If you’re using another GUI toolkit, you would need to subclass and implement child classes using HPS::SceneTree and HPS::SceneTreeItem as starting points.
Then you’d write code for you GUI to create the various capabilities you plan to implement. For example, to highlight an item in the tree, you’d do something like this:
void MFCComponentTreeItem::OnHighlight(HPS::HighlightOptionsKit const & in_options)
{
HPS::ComponentTreeItem::OnHighlight(in_options);
HPS::ComponentTreePtr tree = GetTree();
if (tree != nullptr)
{
CTreeCtrl * treeCtrl = GetTreeCtrl();
treeCtrl->SetItemState(treeItem, TVIS_BOLD, TVIS_BOLD);
GetModelBrowserPane()->UpdateWindow();
}
}
Model browser
The model browser is similar to the segment browser discussed previously, but it works on CAD models and their associated data. The class structure is similar - HPS::ComponentTree is used like HPS::SceneTree, and HPS::ComponentTreeItem is used like HPS::SceneTreeItem.
Notice that when you load a non-CAD model, the model browser is empty because there is no CAD data to display. But when you load a CAD model, both the segment browser and the model browser are populated. This is because the CAD model’s geometry becomes part of the the segment tree but the other CAD data is remains separate. The model browser is used to view that additional data easily.
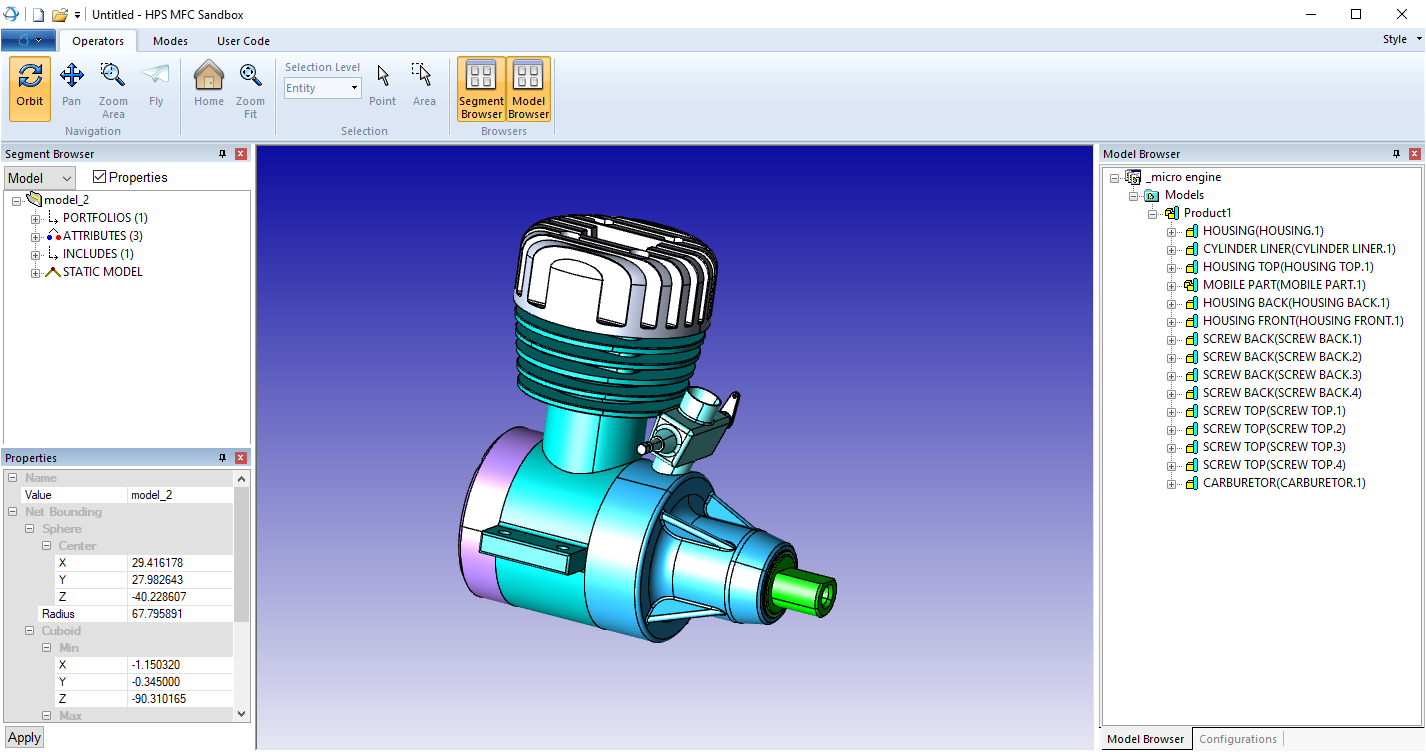
The segment and model browsers do correspond with one another. For example, in the image below, the body is highlighted orange and the two entries in the model and segment browsers are bolded:
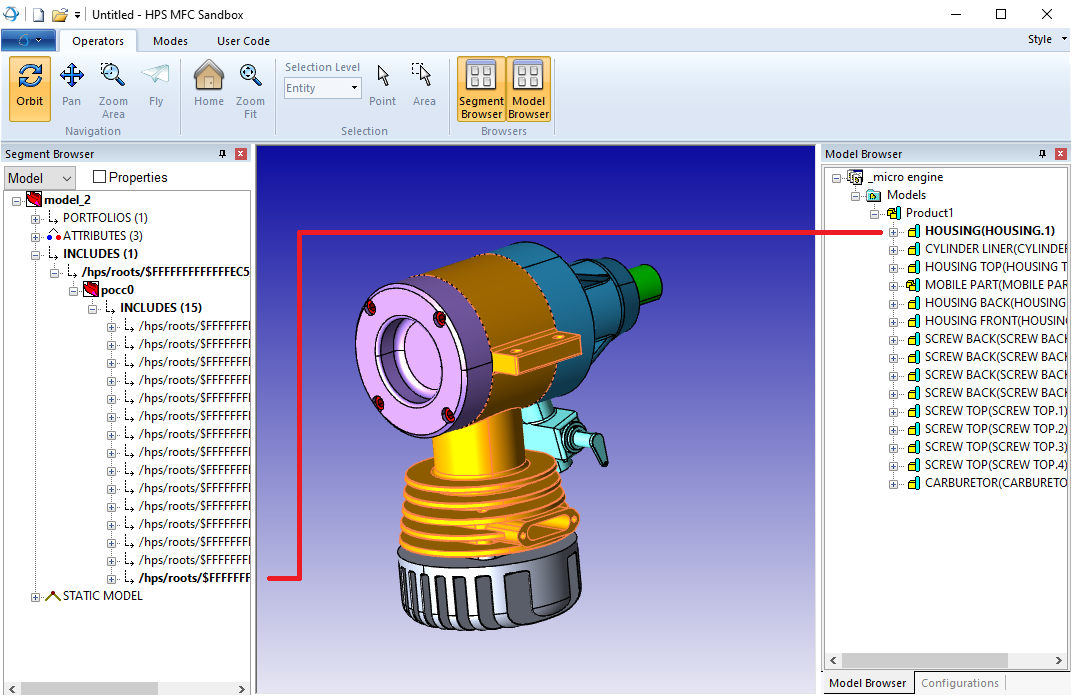