View hierarchy
Now that the application is set up with a window it can use for rendering, let’s take a closer look at the pipeline for how rendering is accomplished. The geometry and associated attributes of any HNP scene are stored in an internal database. This database is organized into a hierarchical scene graph which dictates how rendering takes place. While the scene graph itself is a low-level data structure, HNP provides higher level abstractions which facilitate viewing and scene manipulation. These higher level components make up the view hierarchy.
Four classes work together as nested containers to provide this functionality:
- HPS::Canvas - analogous to the client area of an native application GUI window
- HPS::Layout - optional, used to determine how views are arranged in the window when multiple views are in use
- HPS::View - represents a camera's view of the model, and many views can be active simultaneously
- HPS::Model - a portion of the scene graph which corresponds to a logical model - this contains the geometry
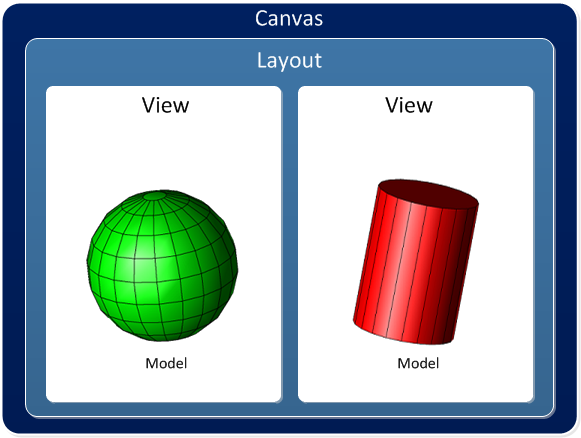
The objects which correspond to the classes listed above are all created using the static class HPS::Factory. For example, continuing from the last code snippet, we can see the View being created and immediately attached to the Canvas:
// Create a new Sprockets View and attach it to our Sprockets.Canvas
HPS::View view = HPS::Factory::CreateView("mfc_sandbox_view");
_canvas.AttachViewAsLayout(view);
HPS::Canvas, HPS:Layout, and HPS::Model are created in a similar way. All of these are static functions:
HPS::Canvas myCanvas = HPS::Factory::CreateCanvas()
HPS::Layout myLayout = HPS::Factory::CreateLayout()
HPS::Model myModel = HPS::Factory::CreateModel()
Additionally, each view hierarchy object has a function to attach children, which binds the hierarchy together. For example:
canvas.AttachLayout(myLayout);
myLayout.AttachViewFront(myView);
myView.AttachModel(myModel);
See the HPS Programming Guide and API reference for more information.
Note that in the example above, the HPS::Layout wasn’t used because there is only a single View which takes the whole window (this shortcut is made available since this is such a common situation). In this case, a View can be attached directly to a Canvas, but if you have multiple views, you’ll need to you a Layout to arrange them correctly inside the window.
The last part of the view hierarchy, the HPS::Model, is a branch of the scene graph which contains the geometry that is to be rendered in the window whenever an update is triggered. To trigger an update, simply call HPS::Canvas::Update. We’ll look at the HPS::Model object in the next section.