Scene setup
At this point, the window is created, HOOPS Native Platform is running, and a model is loaded. Now let’s talk about the SetupScene
function (found in hps_basic.cpp), which is responsible for the scene layout and instantiating the operators. The main objects we deal with here include a Canvas
, View
, and CADModel
, which all get bound together hierarchically under the window key. This arrangement is called the view hierarchy. This hierarchy is a fundamental paradigm which allows you to more easily manage views within a window, scene updates, and user interactions. Four classes work together as nested containers to provide this functionality:
- HPS::Canvas - Analogous to the client area of an native application GUI window.
- HPS::Layout - Optional, determines how views are arranged in the window.
- HPS::View - Represents a camera's view of the model. Multiple views per window are possible if you use an HPS::Layout.
- HPS::Model - A portion of the scene graph which corresponds to a logical model. This is the geometry that's rendered on screen.
- HPS::View - Represents a camera's view of the model. Multiple views per window are possible if you use an HPS::Layout.
- HPS::Layout - Optional, determines how views are arranged in the window.
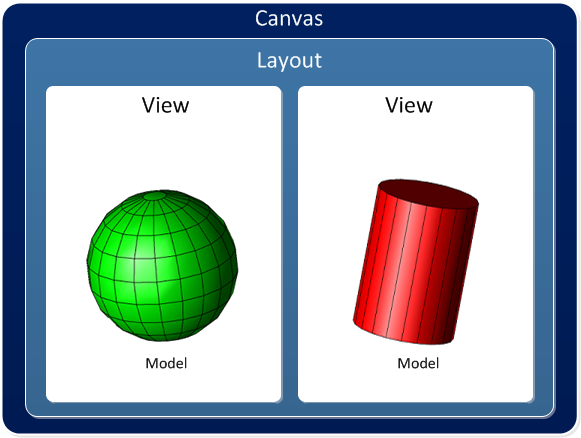
For this tutorial, we’ll only be using a single canvas, view, and model. The layout object won’t be needed in this particular case since the single view will occupy the entire window. Our tutorial code demonstrates how these objects are instantiated:
CADModel modelFile = exchangeNotifier.GetCADModel();
View view = modelFile.ActivateDefaultCapture();
Canvas canvas = Factory::CreateCanvas(windowKey);
canvas.AttachViewAsLayout(view);
There are a few different ways of creating your view hierarchy. The objects can be instantiated independently and bound together as needed (see the HPS::Factory static class), or they can be returned from various functions. In this example, the CADModel
is returned by the HOOPS Exchange import process. The View is obtained as a default capture from the model. Finally, the Canvas
is created statically from the Factory object, then the view is attached with AttachViewAsLayout
.
Note that the model we are loading contains an embedded default “capture”. Not every model contains a capture, but for those that do, HOOPS Exchange is able to create the view from that capture. If your model does not have a default capture, you’ll have to designate a segment as a view segment after the model is loaded and set the camera accordingly. Finally, the view is attached to the canvas. More information on the view hierarchy can be found in the HOOPS Visualize documentation.
Next, we’ll at a light source to the scene to give it some depth and make its features pop. To do this, we’ll need to insert a distant light into the model segment. Scene trees for large models can be complex, but HOOPS Visualize offers a convenient shortcut to get a reference to the model segment. We already have the View
object. We can simply call view.GetAttachedModel().GetSegmentKey()
to get the model segment. From there, we call InsertDistantLight
with a direction vector.
modelKey.InsertDistantLight(Vector(1, 1, 1));
// fit the camera to the scene extents
view.FitWorld();
view.FitWorld()
will render the scene, fit to the scene extents. Now, we can see our model rendered on screen:
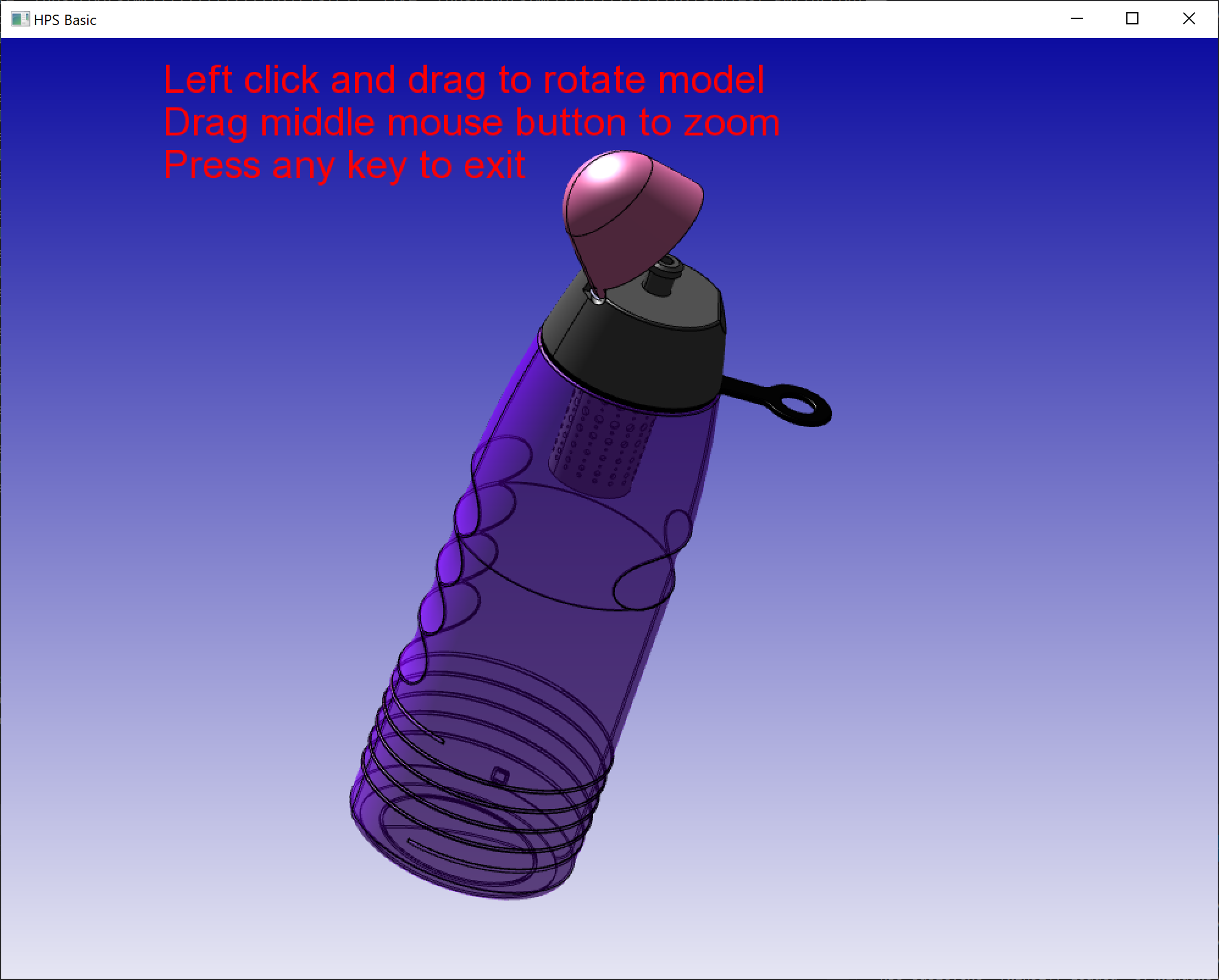
Now we have our model, but at this point it’s just a static scene. We’ll add some basic interactivity in the next section.