Selection
Although they often go together, selection and highlighting are two separate concepts in the HNP framework. We’ll talk about how geometry is selected first before discussing highlighting.
HNP offers a few different ways to select geometry. First of all, you should be aware that selection can take place at one of three different levels. A selection level corresponds to which part of the object hierarchy you’re selecting against. For example, selecting geometry with a segment-level selection will return a reference to the segment that owns that geometry. Selecting geometry at the entity level will select the geometry itself. Subentity selection selects the geometry’s associated faces, vertices, and edges at the point the selection occurred.
In the sandbox, we can use the drop-down menu to choose the selection level:
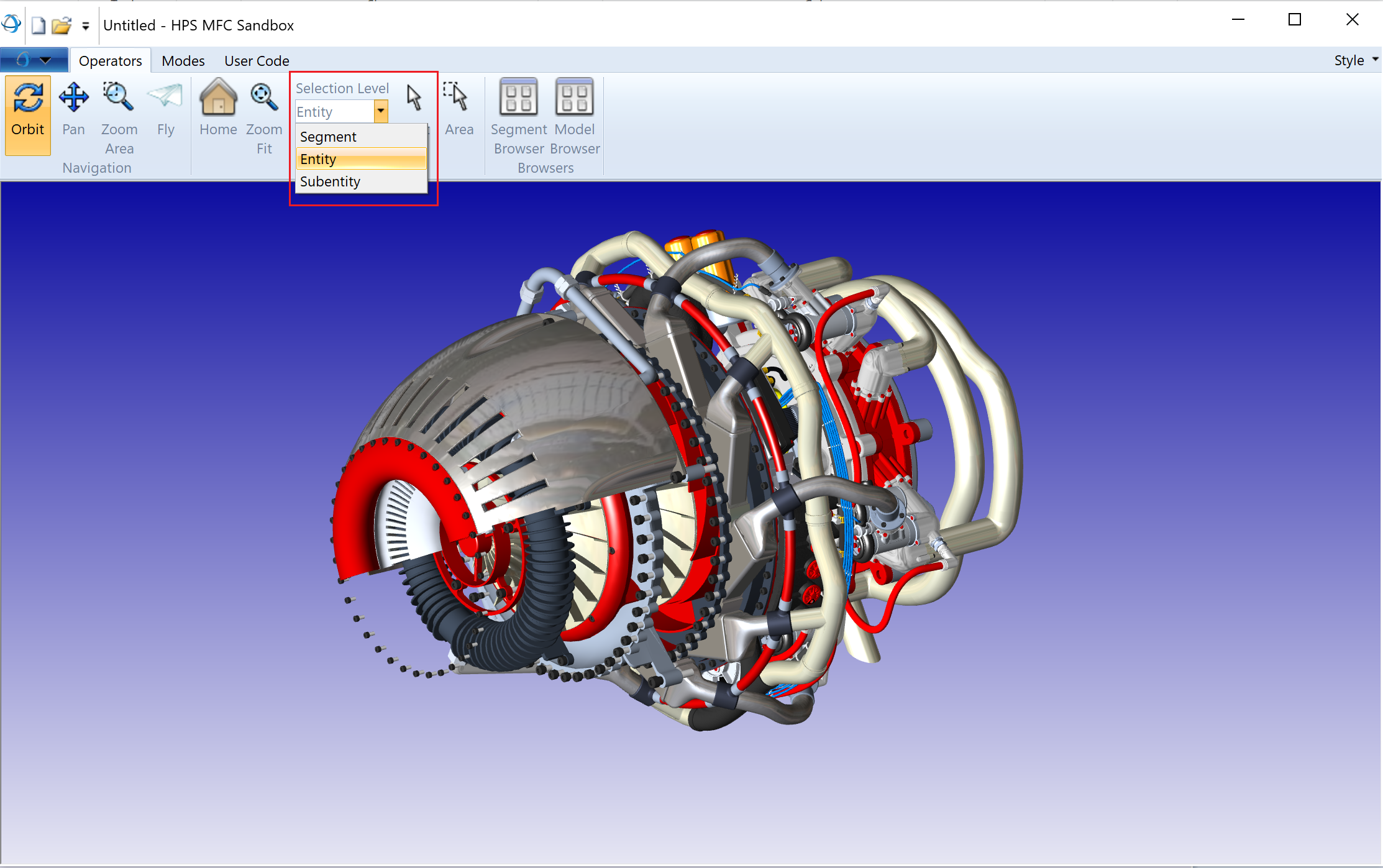
If we then choose the “Point” button in the ribbon menu (located beside the selection level drop-down), the HPS::SelectOperator
will be activated, which will allow us to select a piece of geometry in the model. Behind the scenes, HNP activates the operator like this:
void CHPSView::OnOperatorsSelectPoint()
{
GetCanvas().GetFrontView().GetOperatorControl().Pop();
GetCanvas().GetFrontView().GetOperatorControl().Push(new SandboxHighlightOperator(this));
UpdateOperatorStates(SandboxOperators::PointOperator);
}
In our case, the SelectOperator has been subclassed to SandboxHighlightOperator
. This subclass modifies the default logic slightly to support various highlighting behaviors, which can be seen in SandboxHighlightOp.cpp. Likewise, you are encouraged to subclass any operator needed to fulfill the requirements of your application.
To illustrate the difference in selection level, notice the cowling around the turbine (highlighted in orange) has been selected using entity-level selection in the following screenshot:
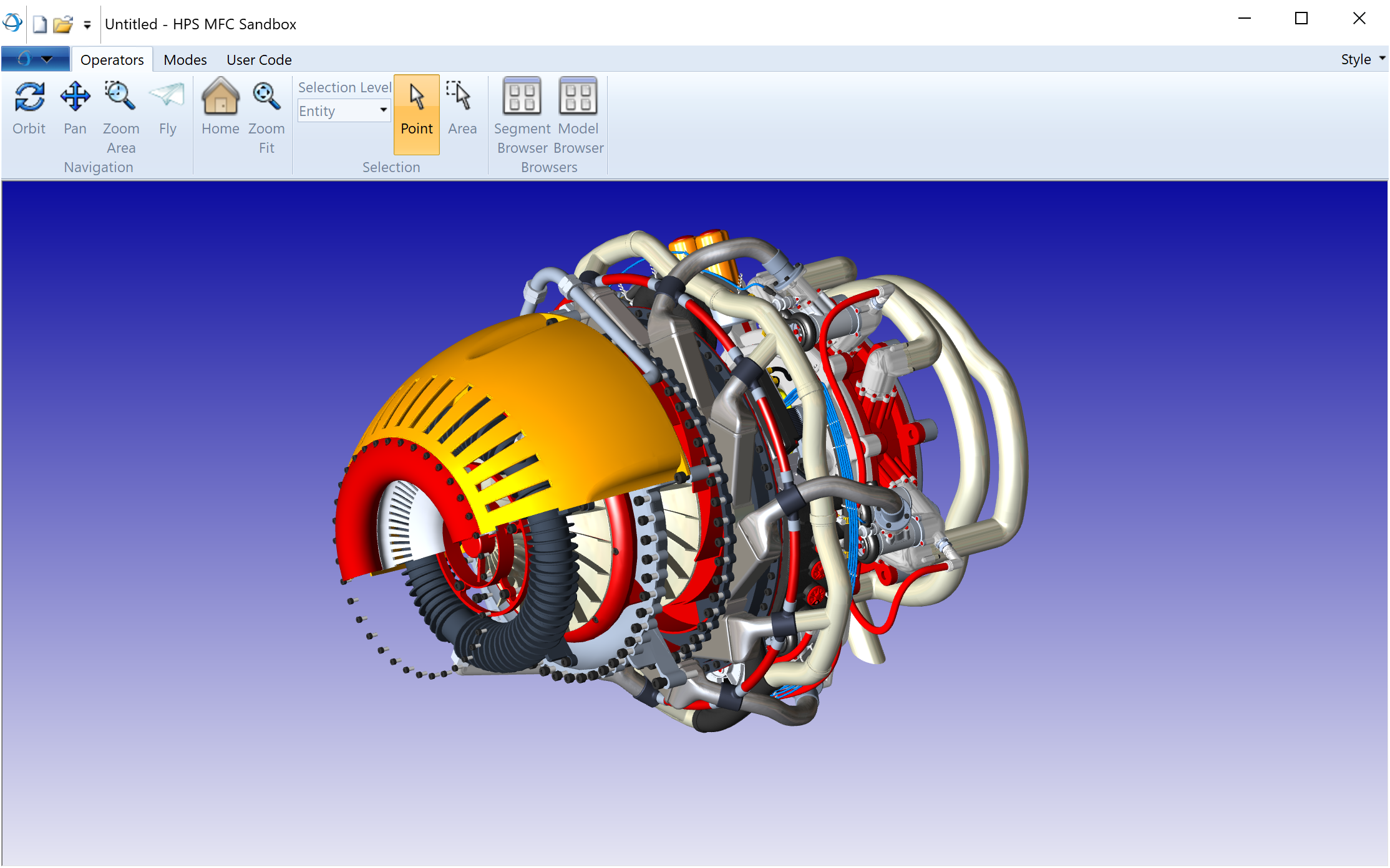
Using subentity selection, clicking on the cowling only selects a single face:
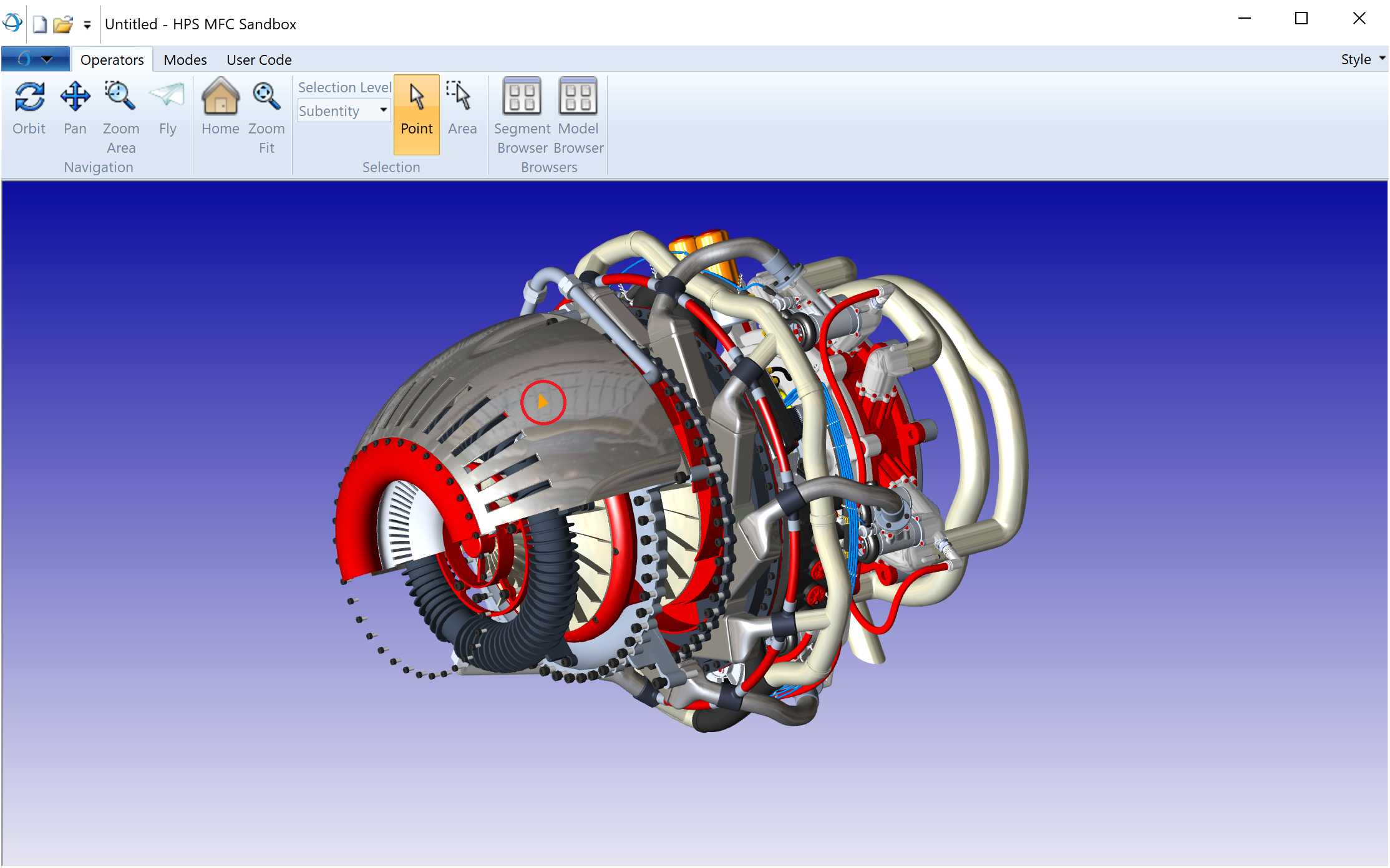
In the sandbox, the only job of the selection operator is to highlight the geometry (highlighting is discussed in the next section), but this is not required. You can use the selection results to perform any logic your application requires.
bool SandboxHighlightOperator::OnMouseDown(
HPS::MouseState const & in_state)
{
auto sel_opts = GetSelectionOptions();
sel_opts.SetLevel(SandboxHighlightOperator::SelectionLevel);
SetSelectionOptions(sel_opts);
if (IsMouseTriggered(in_state) && HPS::SelectOperator::OnMouseDown(in_state))
{
HighlightCommon();
return true;
}
return false;
}
In addition to using the operator, there are a few different ways of performing the selection itself. The most common type of selection would be point-and-click, but you can also select by area, volume, or by shell. Analytic and visual selections are also supported, as well as selection sorting, granularity, and other options - see our Programming Guide page on selection for detailed information related to this topic.