Lines
In Visualize, all lines are polylines, even if they consist of one segment. Like other geometry, the line object has a HPS::LineKey
and HPS::LineKit
. Lines can also be specified without a kit using a PointArray
object as shown below:
PointArray pointArray(3);
// the points specified are the endpoints of the line segments
pointArray[0] = Point(0, 0, 0);
pointArray[1] = Point(0, 1, 0);
pointArray[2] = Point(1, 0, 0);
// inserts a line according to the points contained in pointArray
mySegmentKey.InsertLine(pointArray);
HPS.Point[] pointArray = new HPS.Point[3];
// the points specified are the endpoints of the line segments
pointArray[0] = new HPS.Point(0, 0, 0);
pointArray[1] = new HPS.Point(0, 1, 0);
pointArray[2] = new HPS.Point(1, 0, 0);
// inserts a line according to the points contained in pointArray
mySegmentKey.InsertLine(pointArray);
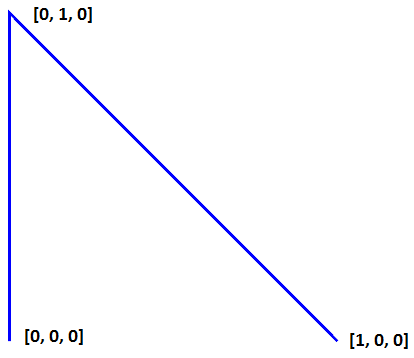
A two-segment polyline
Note that polylines are not assumed to be closed, so if a closed shape is desired, it must be explicitly specified.
Segment-level line attributes are set using the HPS::LineAttributeControl
. These attributes include line weight and pattern. The line above was rendered with a weight of 2. (See the chapter on pattern for more information about this topic.)
mySegmentKey.GetLineAttributeControl().SetWeight(2.0f);
mySegmentKey.GetLineAttributeControl().SetWeight(2.0f);
Infinite Lines
Infinite lines have no start or end point. They extend indefinitely into space. To draw an infinite line, call InsertInfiniteLine
using your HPS::SegmentKey
. Two points along the line are needed. Note that the type of infinite line (HPS::InfiniteLine
or HPS::Ray
) must be specified. The different colors are set at the segment level using the segment key’s HPS::MaterialMappingControl
. Continuing from the example above:
HPS::InfiniteLineKit ilk;
ilk.SetType(HPS::InfiniteLine::Type::Line);
ilk.SetFirst(Point(0, 0, 0));
ilk.SetSecond(Point(1, 1, 0));
mySegmentKey.InsertInfiniteLine(ilk);
HPS.InfiniteLineKit ilk = new HPS.InfiniteLineKit();
ilk.SetType(HPS.InfiniteLine.Type.Line);
ilk.SetFirst(new Point(0, 0, 0));
ilk.SetSecond(new Point(1, 1, 0));
mySegmentKey.InsertInfiniteLine(ilk);
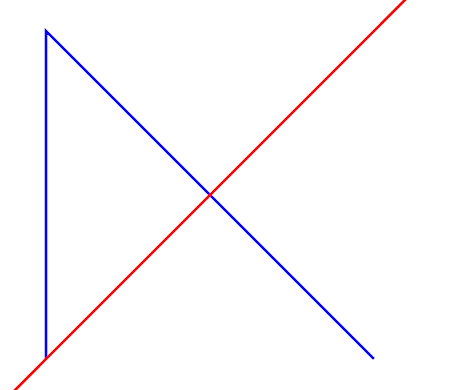
The infinite line drawn over the first polyline
Note that infinite lines are considered unbounded geometry. Thus, they will be ignored in bounding calculations.
Rays
Rays are a type of infinite line. Just like the infinite line, two points are needed in order to insert it. However, in the case of the ray, the first point specified acts as the starting point of the ray. The other end extends indefinitely into space. The only other difference between the two is that the object type is set to HPS::InfiniteLine::Ray
.
HPS::InfiniteLineKit rayKit;
rayKit.SetType(HPS::InfiniteLine::Type::Ray);
rayKit.SetFirst(Point(0, 0.5, 0));
rayKit.SetSecond(Point(1, 0.5, 0));
mySegmentKey.InsertInfiniteLine(rayKit);
HPS.InfiniteLineKit rayKit = new HPS.InfiniteLineKit();
rayKit.SetType(HPS.InfiniteLine.Type.Ray);
rayKit.SetFirst(new HPS.Point(0, 0.5f, 0));
rayKit.SetSecond(new HPS.Point(1, 0.5f, 0));
mySegmentKey.InsertInfiniteLine(rayKit);
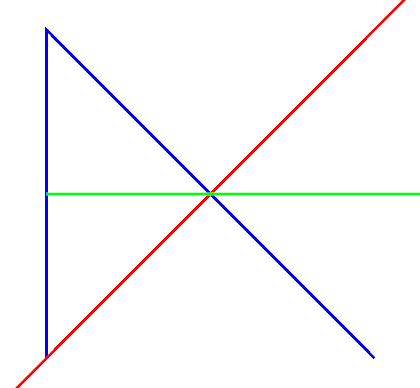
Rays are unbounded on one end
Note that rays are considered unbounded geometry. Thus, they will be ignored in bounding calculations.
Editing Lines
Sometimes it is more desirable to edit a line in place instead of deleting and recreating it. Visualize allows editing of line points through the use of a kit or through direct action on the key. Legal edits include inserting a point into the line, deleting points, and modifying points. In the example below, the position of the first point from the original blue polyline has been modified, then the polygon is closed by inserting a new point at the end of the point list.
// declare point array to hold edited points
Point editedPoints[1];
// declare the new point
editedPoints[0] = Point(0, 0.5f, 0);
// this is the actual modification
myLineKey.EditPointsByReplacement(0, 1, editedPoints);
// this is the insertion of the new point
myLineKey.EditPointsByInsertion(3, 1, editedPoints);
// declare point array to hold edited points
HPS.Point[] editedPoints = new HPS.Point[1];
// declare the new point
editedPoints[0] = new HPS.Point(0, 0.5f, 0);
// this is the actual modification
myLineKey.EditPointsByReplacement(0, editedPoints);
// this is the insertion of the new point
myLineKey.EditPointsByInsertion(3, editedPoints);
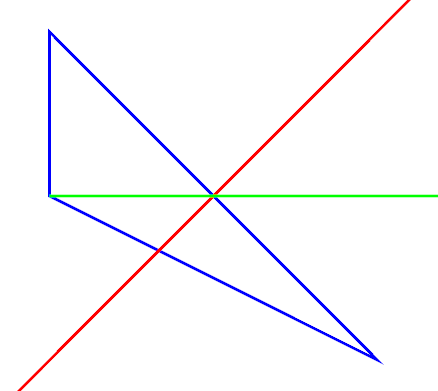
The blue edited polyline
Infinite lines and rays can also be modified by calling SetFirst
or SetSecond
again.
End Caps and Joins
Often, it is desirable to show a symbol as a line endpoint. Any glyph can be used for this purpose. For example, to set the endpoints, use a HPS::LinePatternOptionsKit
to set the start and end caps:
HPS::LinePatternOptionsKit lpok;
lpok.SetEndCap("solid_circle");
lpok.SetStartCap("circle");
mySegmentKey.GetLineAttributeControl().SetPattern("solid", lpok);
HPS.LinePatternOptionsKit lpok = new HPS.LinePatternOptionsKit();
lpok.SetEndCap("solid_circle");
lpok.SetStartCap("circle");
mySegmentKey.GetLineAttributeControl().SetPattern("solid", lpok);
Visualize also supports joining of lines by styling the intersection of line segments. Three styles are offered: HPS::LinePattern::Join::Bevel
, HPS::LinePattern::Join::Mitre
(the default), and HPS::LinePattern::Join::Round
. The image below shows each join, respectively.
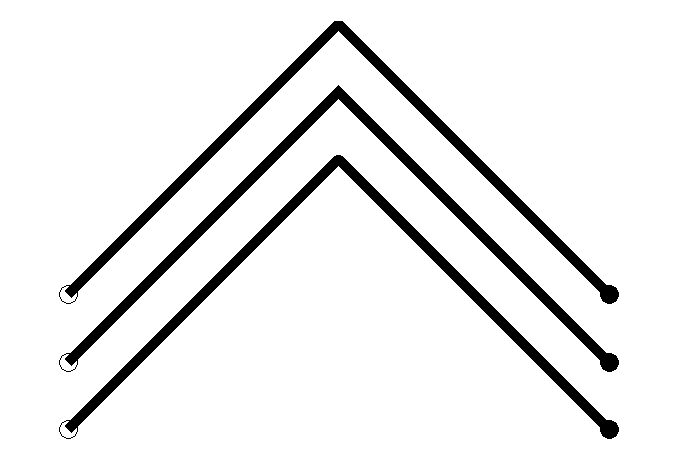
A two-segment polyline showing start and end caps as well as the different types of joins offered by Visualize
Arrowheads
In engineering drawings, arrowheads are a common endpoint to a line segment. In Visualize, arrowheads are a type of line end cap. The HPS::Glyph::Default::SolidTriangleLeft
and HPS::Glyph::Default::SolidTriangleRight
glyphs are commonly used as arrowheads. All of the predefined glyphs can be found in the appendix.