Subwindows
Subwindows are either used to divide the main window into sections, or they are used in an overlapping manner to create “picture-in-picture” displays. While windows are traditionally thought of as a discrete, top-level component, in HOOPS Visualize, a subwindow is actually an attribute of a segment. When set, the segment (and all subsegments) are rendered into the subwindow. When specifying the subwindow, use window coordinates to define the rectangle:
HPS::SubwindowKit subwindowKit;
subwindowKit.SetSubwindow(HPS::Rectangle(0.4f, 0.95f, -0.5f, 0), HPS::Subwindow::Type::Standard);
HPS::SegmentKey anotherSegmentKey = mySegmentKey.Down("subwindow", true);
anotherSegmentKey.SetSubwindow(subwindowKit);
// insert geometry and set attrubutes on 'anotherSegmentKey' as you normally would
HPS.SubwindowKit subwindowKit = new HPS.SubwindowKit();
subwindowKit.SetSubwindow(new HPS.Rectangle(0.4f, 0.95f, -0.5f, 0), HPS.Subwindow.Type.Standard);
HPS.SegmentKey anotherSegmentKey = mySegmentKey.Down("subwindow", true);
anotherSegmentKey.SetSubwindow(subwindowKit);
// insert geometry and set attrubutes on 'subwindowKey' segment as you normally would
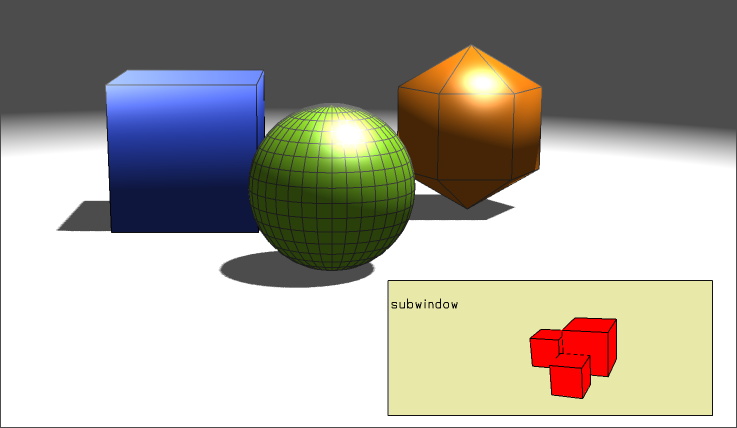
A standard subwindow within a top-level window
Unlike other attributes, subwindows inherit in an unorthodox fashion. When you set a subwindow attribute on a segment, its child segments appear in the subwindow. However, unlike the traditional inheritance paradigm, the child segments have no net subwindow attribute of their own. This exception is made to prevent unwanted recursive nested subwindows. For this reason, many subwindow settings don’t have an effect if called from arbitrary segments where there is no explicitly defined subwindow. If you require a nested subwindow, set it on the particular subsegment where it is needed.
IMPORTANT: It should be noted that all Visualize windows have an implicit standard subwindow that is the size of the main window, even if no subwindow is explicitly defined. There is an important distinction to be made here regarding window coordinate systems. The implicit subwindow uses the HPS::Coordinate::Space::Window
system which varies between -1 and 1 in both the x and y directions. The point 0, 0 marks the center of the screen. These coordinates never change. HPS::Coordinate::Space::InnerWindow
is the coordinate system of any other subwindow.
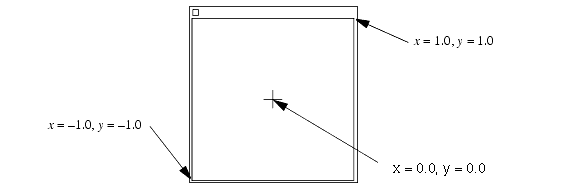
The Coordinate::Space::Window coordinate system
Visualize offers two types of subwindows: standard and lightweight. Both types specify a section of the main window to render into. Unlike a regular window, subwindows have no top-level controls such as minimize or maximize.
A standard subwindow has the same characteristics of a top-level Visualize rendering context while the lightweight version has a few features and limitations:
Lightweight Subwindow Features and Limitations
Uses less resources than standard subwindow
Cannot be nested
Lights positioned in lightweight subwindows will affect geometry in the parent window
Background is always transparent
Selection events pass through
Lightweight subwindows are created in almost the same way as standard subwindows. The only difference is in the call to SetSubwindow</span>, the ``Lightweight
enum is specified:
subwindowKit.SetSubwindow(HPS::Rectangle(0.4f, 0.95f, -0.5f, 0), HPS::Subwindow::Type::Lightweight);
subwindowKit.SetSubwindow(new HPS.Rectangle(0.4f, 0.95f, -0.5f, 0), HPS.Subwindow.Type.Lightweight);
Subwindow Usage
A typical use for a lightweight subwindow would be for an axis triad, where you want the triad to be rendered in the window, but not actually be part of the scene.
When a subwindow is created, a new coordinate system relative to that window is also created. For example, to divide the screen equally between two subwindows, simply specify their bounding rectangle accordingly. Remember that the rectangles are always specified in window coordinates:
// dividing the main window into two subwindows
subwindowKit.SetSubwindow(HPS::Rectangle(-1, 0, -1, 1), HPS::Subwindow::Type::Standard);
HPS::SegmentKey leftSubwindowSeg = mySegmentKey.Down("left", true);
leftSubwindowSeg.SetSubwindow(subwindowKit);
subwindowKit.SetSubwindow(HPS::Rectangle(0, 1, -1, 1), HPS::Subwindow::Type::Standard);
HPS::SegmentKey rightSubwindowSeg = mySegmentKey.Down("right", true);
rightSubwindowSeg.SetSubwindow(subwindowKit);
// dividing the main window into two subwindows
subwindowKit.SetSubwindow(new HPS.Rectangle(-1, 0, -1, 1), HPS.Subwindow.Type.Standard);
HPS.SegmentKey leftSubwindowSeg = mySegmentKey.Down("left", true);
leftSubwindowSeg.SetSubwindow(subwindowKit);
subwindowKit.SetSubwindow(new HPS.Rectangle(0, 1, -1, 1), HPS.Subwindow.Type.Standard);
HPS.SegmentKey rightSubwindowSeg = mySegmentKey.Down("right", true);
rightSubwindowSeg.SetSubwindow(subwindowKit);
A common use case for a subwindow is incorporating an axis triad or other navigation entity in a corner of the screen:
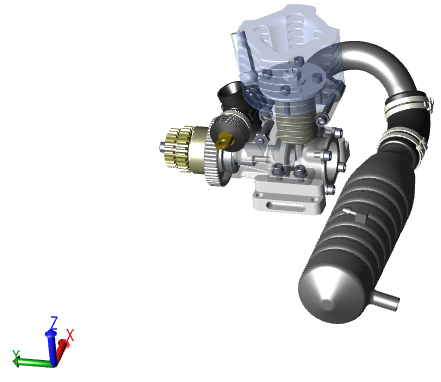
This engine model is in the main window, and the axis triad is in a subwindow.
Windows are normally opaque and cover up the scene underneath, but transparent windows are available via SetBackground
. Borders are also supported:
subwindowKit.SetBorder(HPS::Subwindow::Border::InsetBold);
subwindowKit.SetBackground(HPS::Subwindow::Background::Transparent);
subwindowKit.SetBorder(HPS.Subwindow.Border.InsetBold);
subwindowKit.SetBackground(HPS.Subwindow.Background.Transparent);
Subwindow Priority
As subwindows are inserted into the scene, they are automatically assigned a priority drawing value by HOOPS Visualize. If you have multiple overlapping subwindows in a scene, you can set an integer priority value that determines the draw order. Subwindows are drawn in order from least to greatest, therefore, the subwindows with higher priorities are drawn on top. To set a priority value for a subwindow, simply call HPS::SegmentKey::SetPriority
with an integer value indicating the desired priority.
mySegmentKey.SetPriority(5);
mySegmentKey.SetPriority(5);
Model Compare Mode
Subwindows can be used to compare two models in model comparison mode. The model comparison algorithm uses a depth buffer based difference to compare two models located in other segments.
subwindowKit.SetModelCompareMode(true, segment1, segment2);
subwindowKit.SetModelCompareMode(true, segment1, segment2);
The two HPS::SegmentKey
arguments are to be keys to model segments (segments containing only geometry without camera or lighting attributes). The subwindow object will contain a view with a camera and other attributes with which to view the compare mode for the two models. Once you call this function, the subwindow will automatically display the comparison view. You can manipulate the comparison mode display using Visualize operators (to respond to UI events) or by setting a camera programmatically.
After you have done the comparison, two segments will be created under the subwindow which contain the two models. You can customize the colors by modifying the style segments that get created under the destination segment.
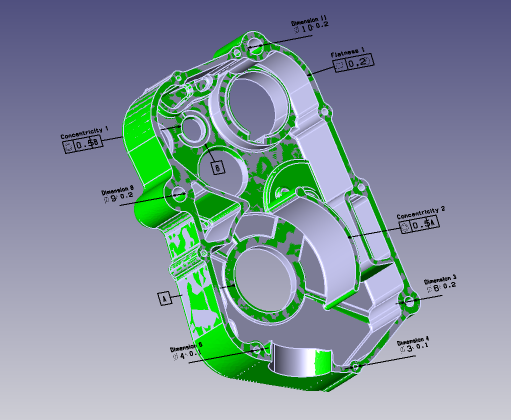
One possible result of the model compare algorithm, highlighting differences in two models.