3. Color and Transparency Mapping - Levels, ColorMap, TransMap, Legend
Three modules are required to define the mapping of the magnitude of field values to color and transparency. The mapping is a two step process. The Levels object manages a table of field value magnitudes and associated map indices. The ColorMap and TransMap objects define the mapping of each map index to a specific color and transparency. In contouring for example, each contour line is drawn at an isovalue of the field value associated with each level in a Levels object. The color used to draw each contour line is determined by using the map index of the level to address a color in a ColorMap object. A basic design objective of the color and transparency features in VisTools has been to give them as many similar functions as possible.
The Legend module has been provided as a means to draw a color/transparency bar which diagrams the mapping of field values to color and transparency as represented by the Levels, ColorMap and TransMap objects.
3.1. Map Field Magnitude to Level - Levels
A Levels object maintains a table of field values, associated map indices and optional text label. Each (field value, map index, label) triple is termed a level. Each Levels object manages a table of 256 levels numbered from 0 to 255. The field values associated with a series of levels may be set explicitly or computed automatically given the field value extremes and one of the built-in rules for spacing the field values. If levels are automatically generated, field values may be spaced linearly or logarithmically. An option is provided to place field values at round numbers. If levels are explicitly set by the user then the field values may be arbitrarily spaced. In all cases the field values must increase monotonically as a function of level number. By default map indices are set equal to the level number. A Levels object does not require any attribute objects. The methods associated with a Levels object are the following.
Begin and end an instance of an object, generic object functions
vis_LevelsBegin()
- create an instance of a Levels objectvis_LevelsEnd()
- destroy an instance of a Levels objectvis_LevelsError()
- return Levels object error flagvis_LevelsCopy()
- make a copy of a Levels object
Operations
vis_LevelsDef()
- define suggested number of levels and scalingvis_LevelsInq()
- inquire suggested number of levels and scalingvis_LevelsFindOne()
- determine level given field valuevis_LevelsFindOneBound()
- determine bounding levels given field valuevis_LevelsFindScales()
- determine scales given field valuesvis_LevelsGenerate()
- compute field values at all levelsvis_LevelsGetNumLevels()
- get actual number of generated levelsvis_LevelsSetIndex()
- set map index at a specific levelvis_LevelsGetIndex()
- get map index at a specific levelvis_LevelsSetLabel()
- set text label at a specific levelvis_LevelsGetLabel()
- get text label at a specific levelvis_LevelsSetMinMax()
- set field value extremesvis_LevelsGetMinMax()
- get field value extremesvis_LevelsSetValue()
- set field value at a specific levelvis_LevelsGetValue()
- get field value at a specific level
A level is accessed using an integer identifier from 0 to 255 for a total of 256 levels. The defined constant LEVELS_MAX is currently set to 256 to reflect this fact. Discrete isovalue entities such as contour lines and threshold surfaces access levels numbers serially from 1 to the number of levels defined in the Levels object. Color filled isovalue entities such as color filled contour plots use the ith map index for all regions with a field value greater than or equal to the ith level (and less than the ith + 1 level if it exists). All regions with field value below level 1 use the map index of level 0 to address the appropriate ColorMap or TransMap. Note that the field value associated with level 0 is never used.
An example of a Levels object is represented schematically in Figure 3-1. The object was generated using the following code fragment.
levels = vis_LevelsBegin ();
vis_LevelsDef (levels,LEVELS_LINEAR,3);
vis_LevelsSetMinMax (levels,0.,1.);
vis_LevelsGenerate (levels,LEVELS_PADENDS);
vis_LevelsSetIndex (levels,0,5);
If this Levels object is set as an attribute to a Contour object and discrete contour lines are generated, then up to 3 contour lines are created at field values .25, .50 and .75 . The associated map indices, 1, 2 and 3, are used to specify colors or transparencies in a ColorMap or TransMap object. If color filled fringes are requested, then up to 5 color filled regions are generated. Note that any region falling below the field value of level 1 (.25) is colored with map index 5.
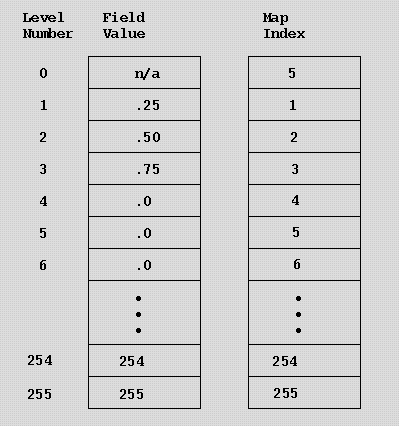
The functions vis_LevelsFindOne()
and
vis_LevelsFindOneBound()
may be used to determine the level information associated with a given
field value. The function vis_LevelsFindScales()
may be used to determine the relative scale factors associated with a
set of field values. The relative scale factor maps the interval [0.,1.]
linearly to the minimum and maximum field values respectively.
3.2. Function Descriptions
The currently available Levels functions are described in detail in this section.
-
vis_Levels *vis_LevelsBegin(void)
create an instance of a Levels object
Create an instance of a Levels object. Memory is allocated for the object private data and the pointer to the data is returned. By default all field values are 0. and map indices are set equal to the level number. The levels minimum and maximum are set to 0. and 1. respectively.
Destroy an instance of a Levels object using
void vis_LevelsEnd (vis_Levels *levels)
Return the current value of a Levels object error flag using
Vint vis_LevelsError (vis_Levels *levels)
Make a copy of a Levels object. The private data from the fromlevels object is copied to the levels object. Any previous private data in levels is lost.
void vgl_LevelsCopy (vgl_Levels *levels, vgl_Levels *fromlevels)
- Returns:
The function returns a pointer to the newly created Levels object. If the object creation fails, NULL is returned.
-
void vis_LevelsEnd(vis_Levels *p)
destroy an instance of a Levels object using
-
Vint vis_LevelsError(vis_Levels *p)
return the current value of a Levels object error flag
-
void vis_LevelsDef(vis_Levels *p, Vint type, Vint nlevels)
define suggested number of levels and spacing
Define the number of levels and the scaling of levels in a Levels object. All levels field values are set to 0. and map indices are set equal to the level number. All levels labels are undefined.
In general, the actual number of levels is set equal to the suggested number of levels. The actual number of levels changes only if using
vis_LevelsGenerate()
to generate levels automatically using a “round” spacing method or with the minimum and maximum values specified usingvis_LevelsSetMinMax()
set equal to one another. The defined constantLEVELS_MAX
is currently set to 256 to reflect the maximum number of levels, ie 1 <= nlevels <LEVELS_MAX
Inquire of defined type and nlevels as output arguments using
void vis_LevelsInq (vis_Levels *levels, Vint *type, Vint *nlevels)
- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.VIS_ERROR_VALUE
is generated if an improper nlevels is specified.
- Parameters:
p – Pointer to Levels object.
type – Method used to scale field values
x=LEVELS_LINEAR Scale field values linearly. =LEVELS_LOG Scale field values logarithmically.
nlevels – Suggested number of levels. 1 <= nlevels <
LEVELS_MAX
.
-
void vis_LevelsInq(vis_Levels *p, Vint *type, Vint *nlevels)
inquire of defined type and nlevels as output arguments
See
vis_LevelsDef()
-
void vis_LevelsSetMinMax(vis_Levels *p, Vfloat min, Vfloat max)
set field value extremes
Set field value extremes. If min is greater than max, then max and min become the minimum and maximum field values respectively. The minimum and maximum values should be set for use with any visualization object which maps size to quantity value. The field value extremes must be set before field values may be automatically calculated at levels using
vis_LevelsGenerate()
.Get min and max as output arguments using
void vis_LevelsGetMinMax (vis_Levels *levels, Vfloat *min, Vfloat *max)
- Parameters:
p – Pointer to Levels object.
min – Field value minimum
max – Field value maximum
-
void vis_LevelsGenerate(vis_Levels *p, Vint method)
generate field values at all levels
Generate field values at levels using the suggested number of levels and scaling rules specified with
vis_LevelsDef()
and the input spacing method. If aLEVELS_ROUND
orLEVELS_ROUNDSPAN
spacing method is used the actual number of levels assigned may differ from the suggested number of levels. Usevis_LevelsGetNumLevels()
to return the actual number of levels assigned byvis_LevelsGenerate()
. The field value extremes must be set usingvis_LevelsSetMinMax()
before field values may be automatically calculated at levels usingvis_LevelsGenerate()
. Field values should be regenerated any time the field value extremes, suggested number of levels or scaling rules are changed. If the field value minimum and maximum are equal then the number of levels is set to one.- Errors
VIS_ERROR_VALUE
is generated if field value extremes span zero for logarithmically spaced levels.VIS_ERROR_ENUM
is generated if an improper method is specified.
- Parameters:
p – Pointer to Levels object.
method – Method used to space field values
x=LEVELS_PADNONE Include the field value extremes. =LEVELS_PADENDS Exclude the field value extremes. =LEVELS_PADTOP Exclude the field value maximum. =LEVELS_ROUND Round numbers within field value extremes. =LEVELS_ROUNDSPAN Round numbers span field value extremes.
-
void vis_LevelsSetValue(vis_Levels *p, Vint ilevel, Vfloat value)
set field value at level
Set field value at a specified level. Note that field values must be monotonically increasing as a function of level number. No check is made to ensure that field values are monotonically increasing.
Get value as an output argument using
void vis_LevelsGetValue (vis_Levels *levels, Vint ilevel, Vfloat *value)
- Errors
VIS_ERROR_VALUE
is generated if an improper ilevel is specified.
- Parameters:
p – Pointer to Levels object.
ilevel – Level number
value – Field value at level ilevel
-
void vis_LevelsSetIndex(vis_Levels *p, Vint ilevel, Vint index)
set map index at level
Set map index at a specified level.
Get index as an output argument using
void vis_LevelsGetIndex (vis_Levels *levels, Vint ilevel, Vint *index)
- Errors
VIS_ERROR_VALUE
is generated if an improper ilevel or index is specified.
- Parameters:
p – Pointer to Levels object.
ilevel – Level number, 0 <= ilevel <=
LEVELS_MAX
index – Map index >= 0 at level ilevel
-
void vis_LevelsSetLabeltv(vis_Levels *p, Vint ilevel, Vtchar *lab)
set text label at a specific level
-
void vis_LevelsSetLabel(vis_Levels *p, Vint ilevel, Vchar *label)
set text label at a specific level
Set text label at a specified level. The label will be truncated to 32 characters. A label may be returned using
vis_LevelsGetLabel()
. The returned label will contain no more than 33 characters including the terminating null character. An undefined label is returned as a single null character. A label may be undefined by setting a string containing a single null character. This label is used by the Legend and Axis objects.Get label as an output argument using
void vis_LevelsGetLabel (vis_Levels *levels, Vint ilevel, Vchar label[]) void vis_LevelsGetLabeltv (vis_Levels *levels, Vint ilevel, Vtchar label[])
- Errors
VIS_ERROR_VALUE
is generated if an improper ilevel is specified.
- Parameters:
p – Pointer to Levels object.
ilevel – Level number
label – Text label at ilevel
-
void vis_LevelsFindScales(vis_Levels *p, Vint nvals, Vfloat values[], Vfloat scales[])
determine scales given field values
- Parameters:
p – Pointer to Levels object.
nvals – Number of field values
values – Field values
scales – [out] Scale factors in the interval [0.,1.]
-
void vis_LevelsFindOne(vis_Levels *p, Vfloat value, Vint *ilevel)
determine level given field value
Determine the level, ilevel, associated with a given field value. The level number returned is the level with the largest field value which is less than or equal to the given field value. If the given field value is less than the field value at level 1 then ilevel is returned as 0.
- Parameters:
p – Pointer to Levels object.
value – Field value
ilevel – [out] Level number
-
void vis_LevelsFindOneBound(vis_Levels *p, Vfloat value, Vint *ilevello, Vint *ilevelhi, Vfloat *w)
determine bounding levels given field value
Determine the bounding levels, ilevello and ilevelhi, and the relative interval position, w, associated with a given field value. The low level number returned is the level with the largest field value which is less than or equal to the given field value. If the given field value is less than the field value at level 1 then ilevel is returned as 0. The high level number returned is one plus the low level number unless the low level number is 0 or the highest level number in which case the high level number is set to the low level number and w is set to zero. The relative interval position, w, is a parameter which varies from 0. to
depending upon the relative position of the given field value in the interval bounded by ilevello and ilevelhi. It will equal 0. if the given field value is the same as the field value at ilevello and will equal 1. if the given field value is the same as the field value at ilevelhi. For example, if the given field value is exactly the average of the field values at ilevello and ilevelhi, then w will equal .5.
- Parameters:
p – Pointer to Levels object.
value – Field value
ilevello – [out] Low bound level number
ilevelhi – [out] High bound level number
w – [out] Relative interval position, 0. <= w <= 1.
-
void vis_LevelsGetLabeltv(vis_Levels *p, Vint ilevel, Vtchar lab[])
get text label at a specific level
-
void vis_LevelsGetLabel(vis_Levels *p, Vint ilevel, Vchar lab[])
get text label at a specific level
-
void vis_LevelsGetNumLevels(vis_Levels *p, Vint *numlevels)
get actual number of levels
Get actual number of levels. The actual number of levels may differ from the suggested number of levels specified using
vis_LevelsDef()
only if field values are assigned to levels withvis_LevelsGenerate()
using aLEVELS_ROUND
orLEVELS_ROUNDSPAN
spacing method.- Parameters:
p – Pointer to Levels object.
numlevels – [out] Actual number of levels
-
void vis_LevelsGetMinMax(vis_Levels *p, Vfloat *min, Vfloat *max)
get field value extremes
-
void vis_LevelsCopy(vis_Levels *p, vis_Levels *fromp)
make a copy of a Levels object
3.3. Color Mapping - ColorMap
Translate a map index to a pseudocolor index or a truecolor RGB triple and apply to the graphics subsystem. A ColorMap object may be declared to be of type pseudocolor or truecolor depending upon the color models supported by the graphics subsystem. A ColorMap maintains a table of entries each of which associate a map index to a color index or color RGB. If a pseudocolor model is used, it is the responsibility of the user to build the appropriate color table in the graphics subsystem. The methods associated with a ColorMap object are the following.
Begin and end an instance of an object, generic object functions
vis_ColorMapBegin()
- create an instance of a ColorMap objectvis_ColorMapEnd()
- destroy an instance of a ColorMap objectvis_ColorMapError()
- return ColorMap object error flagvis_ColorMapCopy()
- make a copy of a ColorMap object
Operations
vis_ColorMapInterpolate()
- generate color map by interpolationvis_ColorMapLevelsGetColors()
- compute spectrum of RGB triplesvis_ColorMapRamp()
- generate one of several built-in color mapsvis_ColorMapSetGamma()
- set gamma correction factorvis_ColorMapGetGamma()
- get gamma correction factorvis_ColorMapSetIndex()
- set pseudocolor index at map indexvis_ColorMapGetIndex()
- get pseudocolor index at map indexvis_ColorMapSetRGB()
- set truecolor RGB triple at map indexvis_ColorMapGetRGB()
- get truecolor RGB triple at map indexvis_ColorMapGetRGBBound()
- get truecolor RGB triple bounded by indicesvis_ColorMapSetHSL()
- set truecolor HSL triple at map indexvis_ColorMapGetHSL()
- get truecolor HSL triple at map indexvis_ColorMapSetType()
- set pseudocolor or true color color map typevis_ColorMapGetType()
- get pseudocolor or true color color map typevis_ColorMapValueDrawFun()
- apply color
A color is accessed using an integer identifier greater than or equal to 0. The components of a truecolor RGB triple range in the interval [0.,1.]. An example of a ColorMap object is represented schematically in Figure 3-2. The object was generated using the following code fragment.
colormap = vis_ColorMapBegin ();
vis_ColorMapSetType (colormap,COLORMAP_TRUECOLOR);
vis_ColorMapRamp (colormap,3,1,COLORMAP_MAGENTA,1.);
c[0] = .5;
c[1] = .5;
c[2] = .5;
vis_ColorMapSetRGB (colormap,1,5,(Vfloat(*)[3],c);
A simple magenta spectrum is set at map indices 1, 2 and 3. A 50 percent gray is placed at map index 5. The colormap is a truecolor colormap. If this ColorMap object were set as an attribute to a Contour object with the Levels object in Figure 3-1, and discrete contour lines are generated, then up to 3 contour lines are created with the RGB colors (1.,0.,1.), (1.,0.,.5), (1.,0.,0.) respectively. If color filled fringes are requested, then up to 4 color filled regions are generated. Note that any region falling below the lowest contour line is given the RGB color (.5,.5,.5).
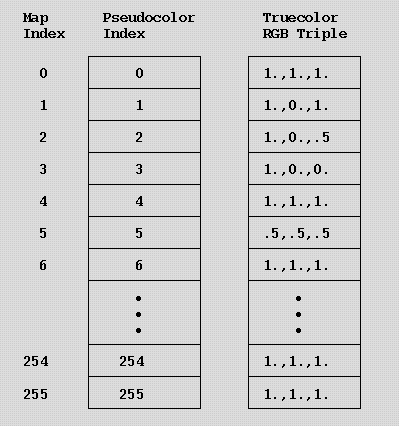
Figure 3-2, Diagram of a ColorMap object
3.4. Function Descriptions
The currently available ColorMap functions are described in detail in this section.
-
vis_ColorMap *vis_ColorMapBegin(void)
create an instance of a ColorMap object
Create an instance of a ColorMap object. Memory is allocated for the object private data and the pointer to the data is returned. By default the color map size is set to 256, the color map type is set to truecolor, all truecolor RGB triples are set to (1.,1.,1.) and all pseudocolor indices are set equal to the map indices.
Destroy an instance of a ColorMap object using
void vis_ColorMapEnd (vis_ColorMap *colormap)
Return the current value of a ColorMap object error flag using
Vint vis_ColorMapError (vis_ColorMap *colormap)
Make a copy of a ColorMap object. The private data from the fromcolormap object is copied to the colormap object. Any previous private data in colormap is lost.
void vis_ColorMapCopy (vis_ColorMap *colormap, vis_ColorMap *fromcolormap)
- Returns:
The function returns a pointer to the newly created ColorMap object. If the object creation fails, NULL is returned.
-
void vis_ColorMapEnd(vis_ColorMap *p)
destroy an instance of a ColorMap object
-
Vint vis_ColorMapError(vis_ColorMap *p)
return the current value of a ColorMap object error flag
-
void vis_ColorMapSetType(vis_ColorMap *p, Vint type)
set color map type as pseudocolor or truecolor
Specify a color map as being a pseudocolor or truecolor map. The type of color map determines whether a ColorIndex or Color drawing function is used to apply a color. This suggests that the color map type should match the color model of the underlying graphics subsystem.
Get type as an output argument using
void vis_ColorMapGetType (vis_ColorMap *colormap, Vint *type)
- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
- Parameters:
p – Pointer to ColorMap object.
type – Color map type
x=COLORMAP_PSEUDOCOLOR Set pseudocolor color map =COLORMAP_TRUECOLOR Set truecolor color map
-
void vis_ColorMapSetIndex(vis_ColorMap *p, Vint nmapindex, Vint imapindex, Vint index[])
set pseudocolor indices in color map
Set pseudocolor indices in a series of map indices. The first pseudocolor index is set at map index imapindex. Subsequent pseudocolor indices are set in map indices incremented by 1 from imapindex.
Get index as an output argument using
void vis_ColorMapGetIndex (vis_ColorMap *colormap, Vint nmapindex, Vint imapindex, Vint index[])
- Parameters:
p – Pointer to ColorMap object.
nmapindex – Number of pseudocolor indices to set
imapindex – Starting map index
index – Array of nmapindex pseudocolor indices
-
void vis_ColorMapSetRGB(vis_ColorMap *p, Vint nmapindex, Vint imapindex, Vfloat c[][3])
set RGB triples in color map
Set truecolor RGB triples in a series of map indices. The first truecolor RGB triple is set at map index imapindex. Subsequent triples are set in map indices incremented by 1 from imapindex.
Get truecolor RGB triples c as an output argument using
void vis_ColorMapGetRGB (vis_ColorMap *colormap, Vint nmapindex, Vint imapindex, Vfloat c[][3])
- Parameters:
p – Pointer to ColorMap object.
nmapindex – Number truecolor RGB triples to set
imapindex – Starting map index
c – Array of nmapindex truecolor RGB triples
-
void vis_ColorMapSetHSL(vis_ColorMap *p, Vint nlevels, Vint level, Vfloat c[][3])
set HSL triples in color map
Set truecolor HSL triples in a series of map indices. The first truecolor HSL triple is set at map index level. Subsequent triples are set in map indices incremented by 1 from level.
Get truecolor HSL triples c as an output argument using
void vis_ColorMapGetHSL (vis_ColorMap *colormap, Vint nmapindex, Vint imapindex, Vfloat c[][3])
- Parameters:
p – Pointer to ColorMap object.
nlevels – Number truecolor HSL triples to set
level – Starting map index
c – Array of nlevels truecolor HSL triples
-
void vis_ColorMapSetGamma(vis_ColorMap *p, Vfloat gamma)
set gamma correction factor
Set gamma correction factor to applied to any truecolor RGB triples output by the ColorMap module. In practice, the gamma correction, gamma is a floating point number between 1. and 3. which corrects for nonlinear color response of a monitor. If the gamma factor is set to zero, gamma correction is disabled. Any gamma input less than zero is set to zero internally. The default gamma correction factor is zero. The ColorMap object functions
vis_ColorMapGetRGB()
andvis_ColorMapValueDrawFun()
output RGB triples.Get gamma as an output argument using
void vis_ColorMapGetGamma (vis_ColorMap *colormap, Vfloat *gamma)
- Parameters:
p – Pointer to ColorMap object.
gamma – Gamma correction factor.
-
void vis_ColorMapRamp(vis_ColorMap *p, Vint nmapindex, Vint imapindex, Vint ramp)
generate one of several built-in color maps
Set truecolor RGB components using a built-in spectrum. If using a pseudocolor color map, the computed RGB components must be retrieved using
vis_ColorMapGetRGB()
and established in the graphics subsystem by the user.The
COLORMAP_SET
ramp defines 29 colors consisting of white and black, and light and dark shades of red, green, blue, magenta, cyan and yellow as well as several shades of gray. The color map index for each color is given by the following defined constants. It is guaranteed to be the case that the values for each defined constant are incremented in the order in which they are listed below. This means, for example, thatCOLORMAP_SET_BLUE
is the same asCOLORMAP_SET_RED
+2.COLORMAP_SET_RED - red COLORMAP_SET_GREEN - green< COLORMAP_SET_BLUE - blue COLORMAP_SET_YELLOW - yellow COLORMAP_SET_CYAN - cyan COLORMAP_SET_MAGENTA - magenta COLORMAP_SET_REDLITE - light red COLORMAP_SET_GREENLITE - light green COLORMAP_SET_BLUELITE - light blue COLORMAP_SET_YELLOWLITE - light yellow COLORMAP_SET_CYANLITE - light cyan COLORMAP_SET_MAGENTALITE - light magenta COLORMAP_SET_REDDARK - dark red COLORMAP_SET_GREENDARK - dark green COLORMAP_SET_BLUEDARK - dark blue COLORMAP_SET_YELLOWDARK - dark yellow COLORMAP_SET_CYANDARK - dark cyan COLORMAP_SET_MAGENTADARK - dark magenta COLORMAP_SET_BLACK - black COLORMAP_SET_WHITE - white COLORMAP_SET_GRAY10 - 10 percent gray (very dark gray) COLORMAP_SET_GRAY20 - 20 percent gray COLORMAP_SET_GRAY30 - 30 percent gray COLORMAP_SET_GRAY40 - 40 percent gray COLORMAP_SET_GRAY50 - 50 percent gray COLORMAP_SET_GRAY60 - 60 percent gray COLORMAP_SET_GRAY70 - 70 percent gray COLORMAP_SET_GRAY80 - 80 percent gray COLORMAP_SET_GRAY90 - 90 percent gray (very light gray)
- Errors
VIS_ERROR_VALUE
is generated if an improper nmapindex or imapindex is specified.VIS_ERROR_ENUM
is generated if an improper ramp is specified.
- Parameters:
p – Pointer to ColorMap object.
nmapindex – Number of truecolor RGB triples to generate
imapindex – Starting map index
ramp – Type of color map to generate
x=COLORMAP_TEMPERATURE Set a temperature like spectrum =COLORMAP_MAGENTA Set evenly spaced fully saturated hues from magenta to red counterclockwise in HSL color space. =COLORMAP_GRAYSCALE Set shades of gray ranging from nearly black to nearly white. =COLORMAP_HUE Set evenly spaced hues from magentared to red in HSL color space with full saturation and lightness. =COLORMAP_HUESCALE Set evenly spaced hues from magentared to red in HSL color space. Saturation and lightness also vary evenly from zero saturation and lightness to full saturation and lightness. =COLORMAP_ABAQUS Set an ABAQUS like spectrum with unevenly spaced hues from blue to red. This spectrum is similar to the ANSYS like spectrum with no restrictions on \a nmapindex. =COLORMAP_ANSYS Set an ANSYS like spectrum with evenly spaced hues from blue to red. This spectrum should be set with \a nmapindex = 12. =COLORMAP_PATRAN Set a PATRAN like spectrum. This spectrum should be set with \a nmapindex = 16. =COLORMAP_RAINBOW Set colors approximating the sequence of colors in a rainbow. =COLORMAP_SET Set a standard set of 29 colors This spectrum should be set with \a nmapindex = 29. =COLORMAP_4BAND Set a simple set of 4 colors, blue, green, yellow, red. This spectrum should be set with \a nmapindex = 4.
-
void vis_ColorMapInterpolate(vis_ColorMap *p, Vint nmapindex, Vint imapindex, Vint colorspace, Vint npts, Vfloat w[], Vfloat c[][3])
generate color map by interpolation
Set truecolor RGB components into a color map using linear interpolation between a set of colors, c specified at parametric coordinates, w in the interval [0.,1.]. Coordinate 0. maps to the imapindex index, and 1. maps to the imapindex + nmapindex - 1 index. The points 0. and 1. must be included in w and all points must be monotonically increasing.
If RGB colors are input, the individual red, green and blue components are normalized in the interval [0.,1.]. If HSL colors are input, the hue varies in the interval [0.,360.], saturation and lightness in [0.,1.]. Note that maximum saturation occurs at a lightness of .5.
- Parameters:
p – Pointer to ColorMap object.
nmapindex – Number of truecolor RGB or HSL triples to set
imapindex – Starting map index
colorspace – Color space of input colors
x=COLORMAP_HSL Hue, saturation and lightness =COLORMAP_RGB Red, green and blue
npts – Number of parametric coordinates with associated color triple
w – Vector of npts parametric coordinates in the interval [0.,1.] for the input colors.
c – Array of npts truecolor RGB or HSL triples at parametric coordinates w.
-
void vis_ColorMapLevelsGetColors(vis_ColorMap *p, vis_Levels *levels, Vint type, Vint ncolors, Vfloat c[][3])
compute spectrum of RGB triples
Compute a spectrum of truecolor RGB triples which represents the mapping of a range of field values in a given levels object to the colors in the colormap object.
Each color computed in the spectrum is the color computed assuming that the field values vary linearly from the minimum to the maximum field values set in the levels object (see
vis_LevelsSetMinMax()
). The first RGB triple, c[0], is computed at the minimum field value, the last RGB triple, c[ncolors-1] is computed at the maximum field value. Intermediate RGB triples are computed assuming the linear variation mentioned above.The computed spectrum may be used as a texture if performing contouring using texture mapping (see Contour module). In this case there are probable constraints on the value of ncolors. Normally ncolors must be a power of 2 or a multiple of 16, etc. A value of 256 is a safe choice. Other uses include displaying the spectrum as part of a “contour” legend.
- Errors
VIS_ERROR_OPERATION
is generated if the colormap is not of typeCOLORMAP_TRUECOLOR
(seevis_ColorMapSetType()
).VIS_ERROR_ENUM
is generated if an improper type is specified.VIS_ERROR_VALUE
is generated if an improper ncolors is specified.
- Parameters:
p – Pointer to ColorMap object
levels – Pointer to Levels object.
type – Type of color spectrum to compute
x=COLORMAP_FRINGE Constant color between levels. =COLORMAP_TONE Continuous color between levels.
ncolors – Number of colors to compute in spectrum 1 <= ncolors
c – Array of ncolors truecolor RGB triples.
-
void vis_ColorMapGetIndex(vis_ColorMap *p, Vint nlevels, Vint level, Vint index[])
get pseudocolor indices in color map
-
void vis_ColorMapGetRGB(vis_ColorMap *p, Vint nlevels, Vint level, Vfloat c[][3])
get RGB triples in color map
-
void vis_ColorMapGetRGBBound(vis_ColorMap *p, Vint imaplo, Vint imaphi, Vfloat w, Vfloat c[3])
get truecolor RGB triple bounded by indices
Get truecolor RGB triple given bounding map indices and relative position between indices. The function
vis_LevelsFindOneBound()
is useful for computing the map indices and relative position.- Parameters:
p – Pointer to ColorMap object.
imaplo – Low bound map index
imaphi – High bound map index
w – Relative interval position, 0. <= w <= 1.
c – [out] Truecolor RGB triple
-
void vis_ColorMapGetHSL(vis_ColorMap *p, Vint nlevels, Vint level, Vfloat c[][3])
get HSL triples in color map
-
void vis_ColorMapGetGamma(vis_ColorMap *p, Vfloat *gamma)
get gamma correction factor
-
void vis_ColorMapValueDrawFun(vis_ColorMap *p, vgl_DrawFun *drawfun, Vint mapindex)
apply color
Apply color associated with mapindex using the drawing functions supplied by the drawfun object.
If the color map is of type
COLORMAP_PSEUDOCOLOR
then a ColorIndex drawing function is invoked.If the color map is of type
COLORMAP_TRUECOLOR
then a Color drawing function is invoked.
-
void vis_ColorMapCopy(vis_ColorMap *p, vis_ColorMap *fromp)
make a copy of a ColorMap object
3.5. Transparency Mapping - TransMap
Translate a map index to a transparency index or transparency factor and apply to the graphics system. A TransMap object may be declared to be of type index or factor depending upon the transparency models supported by the graphics subsystem. A TransMap maintains a table of entries which associate a map index to a transparency index or factor. Generally an index model is appropriate if “screen door” transparency is used. If a transparency factor model is used then alpha planes are usually available to perform transparency compositing. If an index model is used, it is the responsibility of the user to build the appropriate transparency table in the graphics subsystem. The methods associated with a TransMap object are the following.
Begin and end an instance of an object, generic object functions
vis_TransMapBegin()
- create an instance of a TransMap objectvis_TransMapEnd()
- destroy an instance of a TransMap objectvis_TransMapError()
- return TransMap object error flagvis_TransMapCopy()
- make a copy of a TransMap object
Operations
vis_TransMapInterpolate()
- generate transparency map by interpolationvis_TransMapRamp()
- generate a built-in transparency mapvis_TransMapSetDecay()
- set decay exponentvis_TransMapGetDecay()
- get decay exponentvis_TransMapSetIndex()
- set transparency index at map indexvis_TransMapGetIndex()
- get transparency index at map indexvis_TransMapSetTrans()
- set transparency factor at map indexvis_TransMapGetTrans()
- get transparency factor at map indexvis_TransMapSetType()
- set index or factor transparency mapvis_TransMapGetType()
- get index or factor transparency mapvis_TransMapValueDrawFun()
- apply transparency
A transparency is accessed using an integer greater than or equal to 0. Transparency applies only to filled graphics entities. Transparency factors range in the interval [0.,1.]. A transparency factor of 1. is perfectly transparent, a factor of 0. is opaque.
An example of a TransMap object is represented schematically in Figure 3-3. The object was generated using the following code fragment.
transmap = vis_TransMapBegin ();
vis_TransMapSetType (transmap,TRANSMAP_FACTOR);
vis_TransMapRamp (transmap,5,0,TRANSMAP_UP);
vis_TransMapSetTrans (transmap,1,5,1.);
Evenly spaced transparency factors are set at map indices 0 through 4. A single transparency factor of 1. is placed at map index 5. The transmap is a factor transparency map. If this TransMap object were set as an attribute to a Contour object with the Levels object in Figure 3-1, and color filled fringes are requested, then up to 4 color filled regions are generated. Note that any region falling below the lowest contour line is given the transparency factor 1.
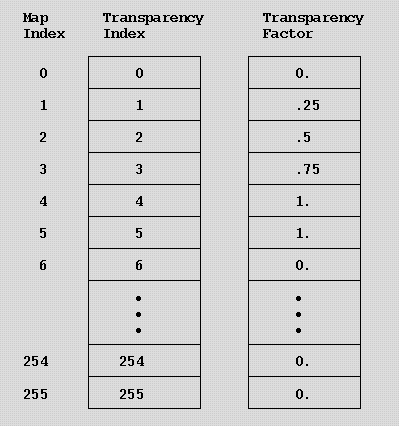
3.6. Function Descriptions
The currently available TransMap functions are described in detail in this section.
-
vis_TransMap *vis_TransMapBegin(void)
create an instance of a TransMap object
Create an instance of a TransMap object. Memory is allocated for the object private data and the pointer to the data is returned. By default the transparency map size is set to 256, the transparency type is set to index, all transparency factors are set to 1. and all transparency indices are set equal to the map indices.
Destroy an instance of a TransMap object using
void vis_TransMapEnd (vis_TransMap *transmap)
Return the current value of a TransMap object error flag using
Vint vis_TransMapError (vis_TransMap *transmap)
Make a copy of a TransMap object. The private data from the fromtransmap object is copied to the transmap object. Any previous private data in transmap is lost.
void vis_TransMapCopy (vis_TransMap *transmap, vis_TransMap *fromtransmap)
- Returns:
The function returns a pointer to the newly created TransMap object. If the object creation fails, NULL is returned.
-
void vis_TransMapEnd(vis_TransMap *p)
destroy an instance of a TransMap object
-
Vint vis_TransMapError(vis_TransMap *p)
return the current value of a TransMap object error flag
-
void vis_TransMapSetType(vis_TransMap *p, Vint type)
set transparency map type as index or factor
Specify a transparency map as being an index or factor map. The type of transparency map determines whether a TransIndex or Trans drawing function is used to apply a transparency. This suggests that the transparency map type should match the transparency model of the underlying graphics subsystem.
Get type as an output argument using
void vis_TransMapGetType (vis_TransMap *transmap, Vint *type)
- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
- Parameters:
p – Pointer to TransMap object.
type – Transparency map type
x=TRANSMAP_INDEX Set index type transparency map =TRANSMAP_FACTOR Set factor type transparency map
-
void vis_TransMapSetIndex(vis_TransMap *p, Vint nmapindex, Vint imapindex, Vint index[])
set transparency indices in transparency map
Set transparency indices in a series of map indices.The first transparency index is set at map index imapindex. Subsequent transparency indices are set in map indices incremented by 1 from imapindex.
Get index as an output argument using
void vis_TransMapGetIndex (vis_TransMap *transmap, Vint nmapindex, Vint imapindex, Vint index[])
- Parameters:
p – Pointer to TransMap object.
nmapindex – Number of transparency indices to set
imapindex – Starting map index
index – Array of nmapindex transparency indices
-
void vis_TransMapSetTrans(vis_TransMap *p, Vint nmapindex, Vint imapindex, Vfloat t[])
set transparency factors in transparency map
Set transparency factors in a series of map indices. The first transparency factor is set at map index imapindex. Subsequent factors are set in map indices incremented by 1 from imapindex.
Get t as an output argument using
void vis_TransMapGetTrans (vis_TransMap *transmap, Vint nmapindex, Vint imapindex, Vfloat t[])
- Parameters:
p – Pointer to TransMap object.
nmapindex – Number transparency factors to set
imapindex – Starting map index
t – Array of nmapindex transparency factors
-
void vis_TransMapSetDecay(vis_TransMap *p, Vfloat decay)
set decay exponent
Set decay exponent applied to any floating point transparency factor output by the TransMap module. The exponent decay is a floating point number greater than 0. to which the transparency factor is raised. If the decay factor is set to zero, the decay is disabled. Any decay input less than zero is set to zero internally. The default decay is zero. The TransMap module functions
vis_TransMapGetTrans()
andvis_TransMapValueDrawFun()
output transparency factors. The decay factor is useful for increasing regions of transparency (decay < 1.) or opacity (decay > 1.)Get decay as an output argument using
void vis_TransMapGetDecay (vis_TransMap *transmap, Vfloat *decay)
- Parameters:
p – Pointer to TransMap object.
decay – Decay exponent
-
void vis_TransMapRamp(vis_TransMap *p, Vint nmapindex, Vint imapindex, Vint ramp)
generate one of several built-in transparency maps
Set transparency factors using a built-in function. If using a transparency index model, the computed transparency factors must be retrieved using
vis_TransMapGetTrans()
and established in the graphics subsystem by the user.The
TRANSMAP_SET
ramp defines 11 transparency factors consisting of opaque and clear and several intermediate transparencies. The transparency map index for each factor is given by the following defined constants. It is guaranteed to be the case that the values for each defined constant are incremented in the order in which they are listed below. This means thatTRANSMAP_SET_FILL10
is the same asTRANSMAP_SET_OPAQUE
+2 .TRANSMAP_SET_OPAQUE - not transparent TRANSMAP_SET_CLEAR - completely transparent TRANSMAP_SET_FILL10 - 10 percent opaque, 90 percent transparent TRANSMAP_SET_FILL20 - 20 percent opaque TRANSMAP_SET_FILL30 - 30 percent opaque TRANSMAP_SET_FILL40 - 40 percent opaque TRANSMAP_SET_FILL50 - 50 percent opaque TRANSMAP_SET_FILL60 - 60 percent opaque TRANSMAP_SET_FILL70 - 70 percent opaque TRANSMAP_SET_FILL80 - 80 percent opaque TRANSMAP_SET_FILL90 - 90 percent opaque, 10 percent transparent
- Errors
VIS_ERROR_VALUE
is generated if an improper nmapindex or imapindex is specified.VIS_ERROR_ENUM
is generated if an improper ramp is specified.
- Parameters:
p – Pointer to TransMap object.
nmapindex – Number of transparency factors to generate
imapindex – Starting map index
ramp – Type of transparency map to generate
x=TRANSMAP_UP Set evenly spaced monotonically increasing transparency factors. =TRANSMAP_DOWN Set evenly spaced monotonically decreasing transparency factors. =TRANSMAP_SET Set a standard set of 11 factors This map should be set with \a nmapindex = 11.
-
void vis_TransMapInterpolate(vis_TransMap *p, Vint nmapindex, Vint imapindex, Vint npts, Vfloat w[], Vfloat t[])
generate transparency map by interpolation
Set transparency factors into a transparency map using linear interpolation between a set of transparencies, t specified at parametric coordinates, w in the interval [0.,1.]. Coordinate 0. maps to the imapindex index, and 1. maps to the imapindex + imapindex - 1 index. The points 0. and 1. must be included in w and all points must be monotonically increasing. The transparency factors are normalized in the interval [0.,1.].
- Parameters:
p – Pointer to TransMap object.
nmapindex – Number of transparency factors to generate
imapindex – Starting map index
npts – Number of parametric coordinates with associated transparency factor.
w – Vector of npts parametric coordinates in the interval [0.,1.] for the input transparencies.
t – Vector of npts transparency factors at parametric coordinates w.
-
void vis_TransMapGetDecay(vis_TransMap *p, Vfloat *decay)
get decay exponent
-
void vis_TransMapGetTrans(vis_TransMap *p, Vint nlevels, Vint level, Vfloat t[])
get transparency factors in transparency map
-
void vis_TransMapValueDrawFun(vis_TransMap *p, vgl_DrawFun *drawfun, Vint mapindex)
apply transparency
Apply transparency associated with mapindex using the drawing functions supplied by the drawfun object. If the transparency map is of type
TRANSMAP_INDEX
then a TransIndex drawing function is invoked. If the transparency map is of typeTRANSMAP_FACTOR
then a Trans drawing function is invoked.
-
void vis_TransMapCopy(vis_TransMap *p, vis_TransMap *fromp)
make a copy of a TransMap object
3.7. Color and Transparency Mapping Diagrams - Legend
The Legend module is designed to draw a simple color bar which displays the color and/or transparency mapping which is represented by a set of ColorMap, TransMap and Levels objects. The color bar displays the color associated with each level in a Levels object and labels the color with the associated field value. The color may be optionally drawn with the transparency mapping defined by a TransMap object. The methods associated with a Legend object are the following.
Begin and end an instance of an object, generic object functions
vis_LegendBegin()
- create an instance of a Legend objectvis_LegendEnd()
- destroy an instance of a Legend objectvis_LegendError()
- return Legend object error flag
Operations
vis_LegendDraw()
- draw a legend diagramvis_LegendSetObject()
- set pointers to attribute objects.vis_LegendGetObject()
- get pointers to attribute objects.vis_LegendSetParami()
- set display parameters
Instance a Legend object using vis_LegendBegin()
.
Once a Legend object is instanced, set the attribute objects using
vis_LegendSetObject()
and set display parameters using
vis_LegendSetParami()
.
The legend diagram is drawn using vis_LegendDraw()
.
The legend display is parameterized in part by the parameters set using
vis_LegendSetParami()
.
The user may choose between a vertical or horizontal layout by setting
the parameter type LEGEND_LAYOUT to LEGEND_VERTICAL or
LEGEND_HORIZONTAL respectively. The vertical layout draws the levels
in order, (minimum to maximum), from bottom to top. By default the
labels are drawn left justified to the right of the color bar. An option
is available to draw the labels right justified to the left of the color
bar. The horizontal layout draws the levels in order, (minimum to
maximum), from left to right. The labels are drawn below the color bar.
The color icon at each level may be drawn as a line or a filled rectangle. The representation may be set to correspond to the IsoValType used when performing contour or isosurface plotting. The parameter type LEGEND_SPECTRUM may be set to LEGEND_LINE for a line, LEGEND_FRINGE for a constant color filled rectangle, LEGEND_TONE for a continuous color filled rectangle or LEGEND_TEXTURE for a texture mapped filled rectangle.
The use of a stroked font as opposed to a raster font for drawing labels may be toggled on or off using the parameter LEGEND_STROKEFONT. A border may be toggled on or off using the parameter LEGEND_BORDER. The display of a filled rectangle for level 0 or the maximum level may be toggled on or off using the parameters LEGEND_PADBOTTOM and LEGEND_PADTOP respectively. Labels may be registered to the “fill” region above a level by enabling the LEGEND_LABELALIGNFILL parameter.
The labels may be drawn using the text labels contained in the Levels attribute object by enabling the LEGEND_LABELUSELEVELS parameter. In this case, if a text label has been defined in the Levels attribute object at a particular level it will replace the normal value label drawn for that level.
The result of drawing a legend with a vertical layout, a fringe spectrum type, a raster font, top padding and border is illustrated in Figure 3-4. Accents are enabled and drawn in black.
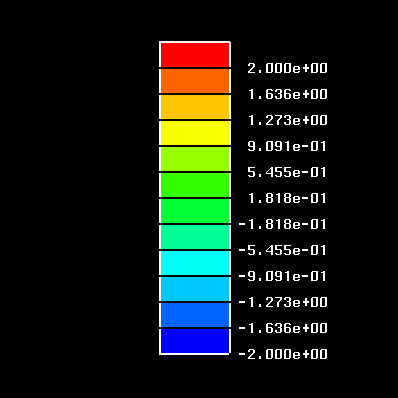
Figure 3-4, Vertical Legend Layout
The result of drawing a legend with a horizontal layout, a line spectrum type, a stroked font, bottom and top padding and a border is illustrated in Figure 3-5. Note that with a horizontal layout that the levels labels are staggered vertically.

Figure 3-5, Horizontal Legend Layout
3.8. Attribute Objects
A Legend object uses a DrawFun,
Levels, ColorMap, TransMap
and VisContext
objects to define attributes required to generate a legend diagram. All
attribute objects are set using vis_LegendSetObject()
.
The Levels and ColorMap
objects are required to define the basic color mapping to be diagrammed.
The TransMap
object is optional and is used for transparency mapping only.
The VisContext attribute object is required for all drawing. The following VisContext components are used.
Color |
Line levels color if not mapped to field value. Accent color. |
DeviceOffset |
Offset in pixels of raster text labels |
Flags |
OR the following flags: VIS_ISOVALACCENT marks the level with a |
Format |
Format of labels. |
LineStyle |
Line style of line levels and accents. |
LineWidth |
Line width of line levels and accents. |
MapColor |
Map levels lines to color if LEGEND_SPECTRUM parameter is |
MapTrans |
Map levels filled rectangles to transparency if LEGEND_SPECTRUM |
MinorColor |
Color of labels and border. |
MinorLineStyle |
Line style of border |
MinorLineWidth |
Line width of border |
MinorSize |
Total vertical size of levels color icons |
Size |
Total horizontal size of levels color icons |
TextBox |
Width and height of a single raster text character in pixels. |
Trans |
Transparency of filled rectangle color icons |
The Legend object calls the following drawing functions set in the DrawFun attribute object.
Color |
Only if ColorMap |
LineStyle |
Only if LEGEND_SPECTRUM is set to LEGEND_TONE. |
PolyLine |
Only if raster font labels are drawn |
Trans |
Only if TransMap |
3.9. Function Descriptions
The currently available Legend functions are described in detail in this section.
-
vis_Legend *vis_LegendBegin(void)
create an instance of a Legend object
Create an instance of a Legend object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL, The spectrum type is fringe, stroke fonts are off, the layout is vertical, bottom and top padding are off.
Destroy an instance of a Legend object using
void vis_LegendEnd (vis_Legend *legend)
Return the current value of a Legend object error flag using
Vint vis_LegendError (vis_Legend *legend)
- Returns:
The function returns a pointer to the newly created Legend object. If the object creation fails, NULL is returned.
-
void vis_LegendEnd(vis_Legend *p)
destroy an instance of a Legend object
-
Vint vis_LegendError(vis_Legend *p)
return the current value of a Legend object error flag
-
void vis_LegendSetObject(vis_Legend *p, Vint objecttype, Vobject *object)
set pointers to attribute objects
Set a pointer to an attribute object.
Get object as an output argument using
void vis_LegendGetObject (vis_Legend *legend, Vint objecttype, Vobject **object)
- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
- Parameters:
p – Pointer to Legend object.
objecttype – The name of the object type to be set.
x=VGL_DRAWFUN DrawFun object =VIS_COLORMAP ColorMap object =VIS_LEVELS Levels object =VIS_TRANSMAP TransMap object =VIS_VISCONTEXT VisContext object
object – Pointer to the object to be set.
-
void vis_LegendGetObject(vis_Legend *p, Vint objecttype, Vobject **object)
get pointers to attribute objects
-
void vis_LegendSetParami(vis_Legend *p, Vint type, Vint iparam)
set display parameters
Toggle the display of a border around the levels color icons. This border does not enclose the labels. By default
LEGEND_BORDER
is set toVIS_OFF
.The labels may be drawn using the text labels contained in the Levels attribute object by enabling the
LEGEND_LABELUSELEVELS
parameter. Labels may be registered to the “fill” region above a level by enabling theLEGEND_LABELALIGNFILL
parameter. By defaultLEGEND_LABELUSELEVELS
andLEGEND_LABELALIGNFILL
are set toVIS_OFF
.Toggle the drawing of the labels to the right or left of a vertical legend using
LEGEND_LABELLEFT
. If the labels are drawn to the right of the color bar, they are drawn left justified. If the labels are drawn to the left of the color bar, they are drawn right justified. The proper right justification of labels placed to the left of the color bar is dependent upon the TextBox visualization context component set usingvis_VisContextSetTextBox()
. Label positioning can be finely tuned using the DeviceOffset visualization context component set usingvis_VisContextSetDeviceOffset()
. This option is ignored for a horizontal legend layout. By defaultLEGEND_LABELLEFT
is set toVIS_OFF
.Specify the legend layout. By default
LEGEND_LAYOUT
is set toLEGEND_VERTICAL
.Toggle the padding of the bottom of the legend. if the spectrum type is fringe or tone, then level 0 fill is drawn in a constant color. By default
LEGEND_PADBOTTOM
is set toVIS_OFF
.Toggle the padding of the top of the legend. If the spectrum type is fringe or tone, then the maximum level fill is drawn in a constant color. By default
LEGEND_PADTOP
is set toVIS_OFF
.Specify the legend spectrum type. If the spectrum type,
LEGEND_SPECTRUM
is set toLEGEND_LINE
, then a line is drawn for each level, if set toLEGEND_FRINGE
, then a constant color filled rectangle is drawn for each level, if set toLEGEND_TONE
, then a continuous color filled rectangle is drawn for each level representing the color variation between levels. if set toLEGEND_TEXTURE
, then a texture mapped color filled rectangle is drawn for each level representing the texture mapped color variation between levels.Toggle the use of a stroked font as opposed to a raster font for drawing labels. By default
LEGEND_STROKEFONT
is set toVIS_OFF
.- Errors
VIS_ERROR_ENUM
is generated if an improper type or iparam is specified.
- Parameters:
p – Pointer to Legend object.
type – Type of display parameter to set
x=LEGEND_BORDER Toggle border display. =LEGEND_LABELALIGNFILL Toggle labels fill alignment. =LEGEND_LABELUSELEVELS Toggle Levels labels. =LEGEND_LABELLEFT Toggle labels drawn on left =LEGEND_LAYOUT Specify legend layout. =LEGEND_PADBOTTOM Toggle padding bottom of legend =LEGEND_PADTOP Toggle padding top of legend =LEGEND_SPECTRUM Specify level color display; line, constant color fill or continuous color fill.
iparam – Specifies the integer value that type will be set to.
x=VIS_ON Enable =VIS_OFF Disable =LEGEND_VERTICAL Vertical legend layout =LEGEND_HORIZONTAL Horizontal legend layout =LEGEND_LINE Levels line. =LEGEND_FRINGE Levels constant color fill. =LEGEND_TEXTURE Levels texture mapped color fill. =LEGEND_TONE Levels continuous color fill.
-
void vis_LegendDraw(vis_Legend *p, Vfloat x[3])
draw a legend diagram
Draw a legend diagram with origin at x. If the legend layout is vertical, then the origin is at the lower left corner of the legend. If the layout is horizontal, the origin is at the upper left corner of the legend.
- Errors
VIS_ERROR_NULLOBJECT
is generated if DrawFun, ColorMap, Levels and VisContext attribute objects are not set.
- Parameters:
p – Pointer to Legend object.
x – Origin of legend diagram.