10. Manipulators - HandleBox, PolyBox, WorkPlane
The manipulator objects are designed to facilitate drawing glyphs with pickable handles which provide a user interface for manipulating, querying and constructing 3D geometry.
The HandleBox module is used to manipulate a rectilinear box oriented to the world coordinate system containing six clipping planes. The polybox may also be used for picking purposes.
The PolyBox module is used to construct polygons in the plane of the graphics screen which can be used for clipping and picking purposes.
The WorkPlane module is used to draw planar rectangular grids arbitrarily oriented in space to use as a reference for geometry construction. The work plane may be optionally used as a clipping plane.
10.1. Handle Box - HandleBox
Use HandleBox to manipulate a rectilinear box in 3D space. The handlebox is oriented to the world coordinate axes and contains selectable handles at each of its eight corners to translate any of the six individual faces of the box. The six faces are meant to be used primarily as clipping planes. The box may be used for node or element picking.
The following functions are associated with a HandleBox object.
- Begin and end an instance of an object, return object error flag
vis_HandleBoxBegin()
- create an instance of a HandleBox objectvis_HandleBoxEnd()
- destroy an instance of a HandleBox objectvis_HandleBoxError()
- return HandleBox object error flag
- Set attributes
vis_HandleBoxGetClipPlanes()
- get clipping plane equationsvis_HandleBoxSetExtent()
- set handlebox extentvis_HandleBoxGetExtent()
- get handlebox extentvis_HandleBoxGetBox()
- get eight corners of handleboxvis_HandleBoxSetObject()
- set pointers to attribute objects.vis_HandleBoxGetObject()
- get pointers to attribute objects.vis_HandleBoxSetParami()
- set handlebox display parametersvis_HandleBoxGetParami()
- get handlebox display parameters
- Operations
vis_HandleBoxTestHit()
- test for handle hitvis_HandleBoxGetHit()
- get internal hit statusvis_HandleBoxInitHit()
- initialize internal hit statusvis_HandleBoxCurrentDrag()
- get current drag statusvis_HandleBoxDrag()
- drag the handleboxvis_HandleBoxDraw()
- draw the handlebox
Instance a HandleBox object using
vis_HandleBoxBegin()
.
Once a HandleBox
object is instanced, define the extents of the handlebox using
vis_HandleBoxSetExtent()
.
The function vis_HandleBoxSetParami()
may be used to toggle various aspects of the handlebox display. The
handlebox manipulation handles consist of 3 vectors at each corner. Each
vector is normal to one of the three faces connected to a given corner.
Therefore each vector uniquely identifies a face and a direction. Use
vis_HandleBoxTestHit()
to check for manipulation handle hits at a given screen location. Use
vis_HandleBoxGetHit()
to get details of the picked handles. Use
vis_HandleBoxInitHit()
to clear the picked handles.
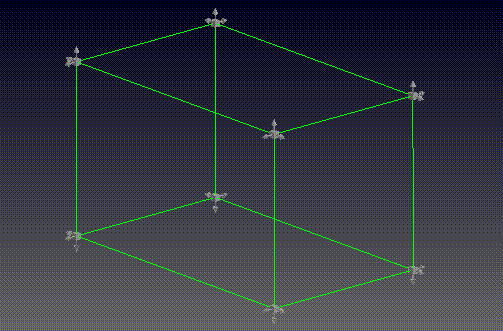
Figure 10-1, HandleBox
The vis_HandleBoxDraw()
function is used to draw the handlebox. Any picked handles detected with
vis_HandleBoxTestHit()
will be highlighted. The picked handles may be used with the
vis_HandleBoxDrag()
function to interactively translate the faces of the handlebox.
The handlebox is primarily meant to be used as 3 sets of parallel
clipping planes. The user may either activate an internal call to the
vgl_DrawFunClipPlane()
function for each plane or query for the current clipping plane
equations using vis_HandleBoxGetClipPlanes()
and manage the clipping plane interface to the graphics subsystem
externally.
The function vis_HandleBoxGetBox()
may be used to return the world coordinates of the eight corners of the
handlebox. This array of world coordinates may be passed directly to the
Space object function vis_SpaceBoxGroup()
to query for all element entities lying in the box or vis_SpaceBoxNodeGroup()
to query for all node entities lying in the box. Selected faces or edges
of the box may be passed to vis_SpacePlaneGroup()
or vis_SpaceLineGroup()
to select elements intersecting the respective face or edge.
10.2. Attribute Objects
A HandleBox object uses ColorMap,
DrawFun and VisContext
objects to define attributes required to generate a handlebox
visualization entity. A HandleBox
object uses the following VisContext
components.
Color | Handles |
ColorA | Handle highlight color |
LineWidth | Edge width |
MinorColor | Edge color |
ProjMatrix | Projection matrix, used for picking |
Viewport | Viewport, used for picking |
XfmMatrix | Modelview matrix, used for picking |
A HandleBox object references the following DrawFun functions.
vgl_DrawFunClipPlane
vgl_DrawFunColor
vgl_DrawFunLineWidth
vgl_DrawFunPolygon
vgl_DrawFunPolyLine
10.3. Function Descriptions
The currently available HandleBox functions are described in detail in this section.
-
vis_HandleBox *
vis_HandleBoxBegin
(void) create an instance of a HandleBox object
Create an instance of a HandleBox object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL. The handlebox extents are (0., 0., 0.) and (1., 1., 1.).
Destroy an instance of a HandleBox object using
void vis_HandleBoxEnd (vis_HandleBox *handlebox)
Return the current value of a HandleBox object error flag using
Vint vis_HandleBoxError (vis_HandleBox *handlebox)
Returns: The function returns a pointer to the newly created HandleBox object. If the object creation fails, NULL is returned.
-
void
vis_HandleBoxEnd
(vis_HandleBox *p) destroy an instance of a HandleBox object
-
Vint
vis_HandleBoxError
(vis_HandleBox *p) return the current value of a HandleBox object error flag
-
void
vis_HandleBoxSetObject
(vis_HandleBox *p, Vint objecttype, Vobject *object) set pointers to attribute objects
Set a pointer to an attribute object.
- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
Parameters: - p – Pointer to HandleBox object.
- objecttype – The name of the object type to be set.
x=VIS_COLORMAP ColorMap object =VGL_DRAWFUN DrawFun object =VIS_VISCONTEXT VisContext object
- object – Pointer to the object to be set.
-
void
vis_HandleBoxSetParami
(vis_HandleBox *p, Vint type, Vint param) set handlebox display parameters
Set display parameters. The parameter
HANDLEBOX_PIXTOL
specifies the pixel distance tolerance for picking handles. By defaultHANDLEBOX_PIXTOL
is set to 4.The parameter
HANDLEBOX_CLIPPLANE
toggles the calling of the ClipPlane drawing function internally for each of the 6 faces. By defaultHANDLEBOX_CLIPPLANE
is set toSYS_ON
.- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
Parameters: - p – Pointer to HandleBox object.
- type – Type of display parameter to set
x=HANDLEBOX_PIXTOL Pixel tolerance for handles =HANDLEBOX_CLIPPLANE Call ClipPlane drawing function
- param – Array of coordinate locations of handlebox corners
x=VIS_OFF Turn parameter off =VIS_ON Turn parameter on
-
void
vis_HandleBoxSetExtent
(vis_HandleBox *p, Vfloat extent[2][3]) set handlebox extent
Set handlebox extents, extent[0] contains the point xmin,ymin,zmin, extent[1] contains the point xmax,ymax,zmax. By default the extents are 0.,0.,0. and 1.,1.,1.
Get current extent as an output argument using
void vis_HandleBoxGetExtent (vis_HandleBox *handlebox, Vfloat extent[2][3])
Parameters: - p – Pointer to HandleBox object.
- extent – Extent
-
void
vis_HandleBoxGetExtent
(vis_HandleBox *p, Vfloat extent[2][3]) get handlebox extent
-
void
vis_HandleBoxGetBox
(vis_HandleBox *p, Vfloat box[8][3]) get eight corners of handlebox
Get coordinates of the 8 corners of the handlebox. This function is useful for obtaining a specification of the handlebox which may be passed directly to the Space object to query for elements and/or node lying in the box.
Parameters: - p – Pointer to HandleBox object.
- box – [out] Array of coordinate locations of handlebox corners
-
void
vis_HandleBoxGetClipPlanes
(vis_HandleBox *p, Vfloat eqn[6][4]) get clipping plane equations
Get plane equations. The planes are ordered xmin, xmax, ymin, ymax, zmin, zmax. The plane equation is this form is useful for defining clipping planes in a graphics subsystem.
Parameters: - p – Pointer to HandleBox object.
- eqn – [out] Plane equations.
-
void
vis_HandleBoxDrag
(vis_HandleBox *p, Vint action, Vint drag, Vint ix, Vint iy) drag the handlebox
Perform a stage, specified by drag, of a dragger action as a function of device coordinate location ix and iy. When the drag stage
VIS_DRAG_TERM
is used the drag action is ignored and the internal drag action is set toVIS_ACTION_NONE
. The drag actionVIS_ACTION_TRANSLATE
only operates when the manipulation handles of the handlebox are hit. The functionvis_HandleBoxDraw()
is called internally by this function.Parameters: - p – Pointer to HandleBox object
- action – [out] Drag action
x=VIS_ACTION_TRANSLATE Translate handlebox
- drag – [out] Drag stage
x=VIS_DRAG_INIT Dragger initialization =VIS_DRAG_MOVE Dragger movement =VIS_DRAG_TERM Dragger termination
- ix – [out] Horizontal device coordinate
- iy – [out] Vertical device coordinate
-
void
vis_HandleBoxCurrentDrag
(vis_HandleBox *p, Vint *action, Vint *drag) get current drag status
Return current drag action and stage used in the last call to
vis_HandleBoxDrag()
.Parameters: - p – Pointer to HandleBox object
- action – [out] Current drag action
- drag – [out] Current drag stage
-
void
vis_HandleBoxDraw
(vis_HandleBox *p) draw the handlebox
Draw the handlebox.
Parameters: p – Pointer to HandleBox object
-
void
vis_HandleBoxTestHit
(vis_HandleBox *p, Vint ix, Vint iy, Vint *hit) test for handle hit
Select a handle from the handlebox. The input point will be a location given in device coordinates. Device coordinates are expressed in terms of pixel coordinates on a graphics device. If the hit flag is non-zero then use
vis_HandleBoxGetHit()
to find the manipulation handle. The hit manipulation handle will be highlighted when drawn usingvis_HandleBoxDraw()
.Parameters: - p – Pointer to HandleBox object
- ix – Horizontal device coordinate
- iy – Vertical device coordinate
- hit – [out] Hit flag
-
void
vis_HandleBoxInitHit
(vis_HandleBox *p) initialize internal hit status
Initialize hit status. This sets the manipulation handle hit status to zero.
Parameters: p – Pointer to HandleBox object
-
void
vis_HandleBoxGetHit
(vis_HandleBox *p, Vint *corner, Vint *idir) get internal hit status
Get manipulation handle detected by a previous call to
vis_HandleBoxTestHit()
. The returned corner argument is zero if no manipulation handle is hit. The corners are ordered zmin first followed by zmax. At a given z plane they are ordered xmin,ymin, xmax,ymin, xmax,ymax and xmin,ymax. The argument idir is 1, 2 or 3 for the x, y or z directions.Parameters: - p – Pointer to HandleBox object
- corner – [out] Corner
- idir – [out] Direction
10.4. Polygon Box - PolyBox
Use PolyBox to construct and manipulate a polygon in the plane of the graphics screen. The polygon may be used for clipping and picking purposes.
The following functions are associated with a PolyBox object.
- Begin and end an instance of an object, return object error flag
vis_PolyBoxBegin()
- create an instance of a PolyBox objectvis_PolyBoxEnd()
- destroy an instance of a PolyBox objectvis_PolyBoxError()
- return PolyBox object error flag
- Set attributes
vis_PolyBoxGetClipPlanes()
- get clipping plane equationsvis_PolyBoxInitPoly()
- initialize polybox polygon to nonevis_PolyBoxSetPoly()
- set polybox polygonvis_PolyBoxGetPoly()
- get polybox polygonvis_PolyBoxGetTri()
- get polygon triangulationvis_PolyBoxGetPenta()
- get polygon extrusion pentahedravis_PolyBoxSetObject()
- set pointers to attribute objects.vis_PolyBoxGetObject()
- get pointers to attribute objects.vis_PolyBoxSetParami()
- set polybox display parametersvis_PolyBoxGetParami()
- get polybox display parameters
- Operations
vis_PolyBoxTestHit()
- test for handle hitvis_PolyBoxGetHit()
- get internal hit statusvis_PolyBoxInitHit()
- initialize internal hit statusvis_PolyBoxCurrentDrag()
- get current drag statusvis_PolyBoxDrag()
- drag the polyboxvis_PolyBoxDraw()
- draw the polybox
Instance a PolyBox object using vis_PolyBoxBegin()
.
Once a PolyBox object is instanced, a polygon may be set using
vis_PolyBoxSetPoly()
or it may be constructed using
vis_PolyBoxDrag()
.
Query for a set or constructed polygon using vis_PolyBoxGetPoly()
The function vis_PolyBoxSetParami()
may be used to toggle various aspects of the polybox display. Each of
the polygon corner points is a selectable handle. Use
vis_PolyBoxTestHit()
to check for handle hits at a given screen location. Use
vis_PolyBoxGetHit()
to get details of the picked handles. Use
vis_PolyBoxInitHit()
to clear the picked handles.
The vis_PolyBoxDraw()
function is used to draw the polybox. Any picked handles detected with
vis_PolyBoxTestHit()
will be highlighted. The picked handles may be used with the
vis_PolyBoxDrag()
function to interactively move the corners of the polybox.
The polybox is primarily meant to be used as a graphics screen oriented
picking device. The function vis_PolyBoxGetTri()
may be used to return the triangulation of the corners of the polybox.
The function vis_PolyBoxGetPenta()
may be used to get the extruded pentahedra which form the volume the the
polybox extruded from the near to the far graphics system z clipping
planes. This array of world coordinates and pentahedra connectivity may
be passed directly to the Space object functions
vis_SpaceBoxGroup()
and/or vis_SpaceBoxNodeGroup()
to query for all element and/or node entities lying in the extruded
volume.
10.5. Attribute Objects
A PolyBox object uses ColorMap, DrawFun and VisContext objects to define attributes required to generate a polybox visualization entity. A PolyBox object uses the following VisContext components.
Color | Handles |
ColorA | Handle highlight color |
LineWidth | Edge width |
MinorColor | Edge color |
ProjMatrix | Projection matrix, used for picking |
Viewport | Viewport, used for picking |
XfmMatrix | Modelview matrix, used for picking |
A PolyBox object references the following DrawFun functions.
vgl_DrawFunClipPlane
vgl_DrawFunColor
vgl_DrawFunLineWidth
vgl_DrawFunPointSize
vgl_DrawFunPolyLine
vgl_DrawFunPolyPoint
10.6. Function Descriptions
The currently available PolyBox functions are described in detail in this section.
-
vis_PolyBox *
vis_PolyBoxBegin
(void) create an instance of a PolyBox object
Create an instance of a PolyBox object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL.
Destroy an instance of a PolyBox object using
void vis_PolyBoxEnd (vis_PolyBox *polybox)
Return the current value of a PolyBox object error flag using
Vint vis_PolyBoxError (vis_PolyBox *polybox)
Returns: The function returns a pointer to the newly created PolyBox object. If the object creation fails, NULL is returned.
-
void
vis_PolyBoxEnd
(vis_PolyBox *p) destroy an instance of a PolyBox object
-
Vint
vis_PolyBoxError
(vis_PolyBox *p) return the current value of a PolyBox object error flag
-
void
vis_PolyBoxSetObject
(vis_PolyBox *p, Vint objecttype, Vobject *object) set pointers to attribute objects
Set a pointer to an attribute object.
- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
Parameters: - p – Pointer to PolyBox object.
- objecttype – The name of the object type to be set.
x=VIS_COLORMAP ColorMap object =VGL_DRAWFUN DrawFun object =VIS_VISCONTEXT VisContext object
- object – Pointer to the object to be set.
-
void
vis_PolyBoxSetParami
(vis_PolyBox *p, Vint type, Vint param) set polybox display parameters
Set display parameters. The parameter
POLYBOX_PIXTOL
specifies the pixel distance tolerance for picking handles. By defaultPOLYBOX_PIXTOL
is set to 4.- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
Parameters: - p – Pointer to PolyBox object.
- type – Type of display parameter to set
x=POLYBOX_PIXTOL Pixel tolerance for handles
- param – Specifies the integer value that type will be set to.
x=VIS_OFF Turn parameter off =VIS_ON Turn parameter on
-
void
vis_PolyBoxSetPoly
(vis_PolyBox *p, Vint npts, Vint pts[][2]) set polybox polygon
Set polybox polygon.
Get defined polygon as an output argument using
void vis_PolyBoxGetPoly (vis_PolyBox *polybox, Vint *npts, Vfloat pts[][2])
Parameters: - p – Pointer to PolyBox object.
- npts – Number of points in polygon
- pts – Pixel coordinates of points
-
void
vis_PolyBoxInitPoly
(vis_PolyBox *p) initialize polybox polygon to none
Initialize polybox polygon to none.
Parameters: p – Pointer to PolyBox object.
-
void
vis_PolyBoxGetClipPlanes
(vis_PolyBox *p, Vfloat eqn[6][4]) get clipping plane equations
Get plane equations.
Parameters: - p – Pointer to PolyBox object.
- eqn – [out] Plane equations.
-
void
vis_PolyBoxDrag
(vis_PolyBox *p, Vint action, Vint drag, Vint ix, Vint iy) drag the polybox
Perform a stage, specified by drag, of a dragger action as a function of device coordinate location ix and iy. When the drag stage
VIS_DRAG_TERM
is used the drag action is ignored and the internal drag action is set toVIS_ACTION_NONE
. The drag actionsPOLYBOX_ACTION_CORNER
only operates when a corner handle of the polybox is hit. The functionvis_PolyBoxDraw()
is called internally by this function.Parameters: - p – Pointer to PolyBox object
- action – [out] Drag action
x=POLYBOX_ACTION_CORNER Place polygon corner =POLYBOX_ACTION_MOVECORNER Move polygon corner =POLYBOX_ACTION_LINE Stretch line from placed corner. =POLYBOX_ACTION_TRANSLATE Translate polygon
- drag – [out] Drag stage
x=VIS_DRAG_INIT Dragger initialization =VIS_DRAG_MOVE Dragger movement =VIS_DRAG_TERM Dragger termination
- ix – [out] Horizontal device coordinate
- iy – [out] Vertical device coordinate
-
void
vis_PolyBoxCurrentDrag
(vis_PolyBox *p, Vint *action, Vint *drag) get current drag status
Return current drag action and stage used in the last call to
vis_PolyBoxDrag()
.Parameters: - p – Pointer to PolyBox object.
- action – [out] Current drag action
- drag – [out] Current drag stage
-
void
vis_PolyBoxDraw
(vis_PolyBox *p) draw the polybox
Draw the polybox.
Parameters: p – Pointer to PolyBox object
-
void
vis_PolyBoxTestHit
(vis_PolyBox *p, Vint ix, Vint iy, Vint *hit) test for handle hit
Select a handle from the polybox. The input point will be a location given in device coordinates. Device coordinates are expressed in terms of pixel coordinates on a graphics device. If the hit flag is non-zero then use
vis_PolyBoxGetHit()
to find the manipulation handle. The hit manipulation handle will be highlighted when drawn usingvis_PolyBoxDraw()
.Parameters: - p – Pointer to PolyBox object
- ix – Horizontal device coordinate
- iy – Vertical device coordinate
- hit – [out] Hit flag
-
void
vis_PolyBoxInitHit
(vis_PolyBox *p) initialize internal hit status
Initialize hit status. This sets the manipulation handle hit status to zero.
Parameters: p – Pointer to PolyBox object.
-
void
vis_PolyBoxGetHit
(vis_PolyBox *p, Vint *corner) get internal hit status
Get manipulation handle detected by a previous call to
vis_PolyBoxTestHit()
. The returned corner argument is zero if no manipulation handle is hit.Parameters: - p – Pointer to PolyBox object
- corner – [out] Corner
-
void
vis_PolyBoxGetTri
(vis_PolyBox *p, Vint *ntri, Vint tri[][3]) get polygon triangulation
Get triangulation of polygon corners. Use this triangulation for picking purposes.
Parameters: - p – Pointer to PolyBox object.
- ntri – [out] Number of triangles
- tri – [out] Connectivity of triangles
-
void
vis_PolyBoxGetPenta
(vis_PolyBox *p, Vint *npts, Vfloat pts[][3], Vint *npenta, Vint penta[][6]) get polygon extrusion pentahedra
Get pentahedra which cover the extruded polygon from the near to far graphics system z clipping planes. Use this set of pentahedra for picking purposes.
Parameters: - p – Pointer to PolyBox object.
- npts – [out] Number of points
- pts – [out] Point world coordinates
- npenta – [out] Number of pentahedra
- penta – [out] Pentahedra connectivity
10.7. Work Plane - WorkPlane
Use WorkPlane to select points constrained to a 2D plane, called a workplane, embedded in 3D space. The workplane can be used to define a cross section of a geometric object (e.g., the cross section of a beam).
The WorkPlane object employs a local 2D coordinate system embedded in 3D space called the workplane to constrain the selection of points within a 2D plane. The workplane can be positioned arbitrarily in 3D space. The orientation of the workplane is defined by a pair of 3D vectors which specify the x-axis and y-axis of the local coordinate system of the workplane.
Grid points and/or grid lines may be drawn within the workplane to serve as visual references when selecting points. A snap option is also available which restricts the selection of points to grid points only. A set of pickable handles for translating and rotating the plane may be displayed and queried. The workplane may also be used as a clipping and picking plane.
The following functions are associated with a WorkPlane object.
- Begin and end an instance of an object, return object error flag
vis_WorkPlaneBegin()
- create an instance of a WorkPlane objectvis_WorkPlaneEnd()
- destroy an instance of a WorkPlane objectvis_WorkPlaneError()
- return WorkPlane object error flag
- Set attributes
vis_WorkPlaneGetClipPlane()
- get clipping plane equationvis_WorkPlaneSetDimensions()
- set workplane height, width, spacingvis_WorkPlaneGetDimensions()
- get workplane height, width, spacingvis_WorkPlaneGetPlane()
- get four corners of workplanevis_WorkPlaneSetObject()
- set pointers to attribute objects.vis_WorkPlaneGetObject()
- get pointers to attribute objects.vis_WorkPlaneSetOriginPlane()
- set workplane origin and planevis_WorkPlaneGetOriginPlane()
- get workplane origin and planevis_WorkPlaneSetParami()
- set workplane display parametersvis_WorkPlaneGetParami()
- get workplane display parameters
- Operations
vis_WorkPlaneTestHit()
- test for handle hitvis_WorkPlaneGetHit()
- get internal hit statusvis_WorkPlaneInitHit()
- initialize internal hit statusvis_WorkPlaneCurrentDrag()
- get current drag statusvis_WorkPlaneDrag()
- drag the workplanevis_WorkPlaneDraw()
- draw the workplanevis_WorkPlaneGridPoint()
- get a grid pointvis_WorkPlaneNumGrid()
- get number of grid points
Instance a WorkPlane object using vis_WorkPlaneBegin()
.
Once a WorkPlane
object is instanced, define the origin and orientation of the plane
using vis_WorkPlaneSetOriginPlane()
.
Define the outer width and height dimensions of the workplane and the
grid (snap) point spacing using vis_WorkPlaneSetDimensions()
.
The number of grid points in the width and height directions is computed
from the spacing. The pickable entities on the workplane consist of the
grid points and any manipulation handles which are active. The
manipulation handles consist of three vector centered at the origin
which point in the local x (width), y (height) and z
(normal) directions. The function vis_WorkPlaneSetParami()
may be used to toggle various aspects of the workplane display. Use
vis_WorkPlaneTestHit()
to check for grid point and manipulation handle hits at a given screen
location. Use vis_WorkPlaneGetHit()
to get details of the picked grid points and handles. Use
vis_WorkPlaneInitHit()
to clear the picked grid point and handles.
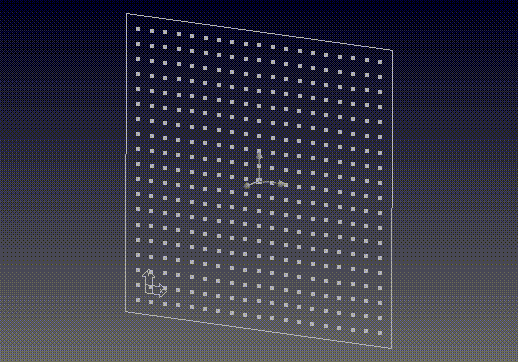
Figure 10-2, WorkPlane
The vis_WorkPlaneDraw()
function is used to draw the workplane. Any picked grid point and/or
handle detected with vis_WorkPlaneTestHit()
will be highlighted. The picked handles may be used with the
vis_WorkPlaneDrag()
function to interactively translate and rotate the workplane. Use
vis_WorkPlaneGridPoint()
to return the world coordinate location of a given grid point.
The workplane may be optionally used as a clipping plane. The user may
either activate an internal call to the vgl_DrawFunClipPlane()
function or query for the current clipping plane equation using
vis_WorkPlaneGetClipPlane()
and manage the clipping plane interface to the graphics subsystem
externally.
The function vis_WorkPlaneGetPlane()
may be used to return the world coordinates of the corners of the
workplane. This array of world coordinates may be passed directly to the
Space object function vis_SpacePlaneGroup()
to query for all element entities lying on the plane.
10.8. Attribute Objects
A WorkPlane object uses ColorMap, DrawFun, TransMap and VisContext objects to define attributes required to generate a workplane visualization entity. A WorkPlane object requires a VisContext object for line color, width and style. A WorkPlane object uses the following VisContext components.
Color | Outline, origin, handles, fill, grid points and axes color |
ColorA | Handle highlight color |
ColorB | Grid point highlight color |
Fill | Fill grid |
LineWidth | Line width outline and grid lines |
LineStyle | Line style for grid lines |
MinorColor | Line color |
PointSize | Point grid size |
ProjMatrix | Projection matrix, used for picking |
Trans | Fill grid transparency |
Viewport | Viewport, used for picking |
XfmMatrix | Modelview matrix, used for picking |
A WorkPlane object references the following DrawFun functions.
vgl_DrawFunClipPlane
vgl_DrawFunColor
vgl_DrawFunLineStyle
vgl_DrawFunLineWidth
vgl_DrawFunPointSize
vgl_DrawFunPolygon
vgl_DrawFunPolyLine
vgl_DrawFunPolyPoint
vgl_DrawFunTrans
10.9. Function Descriptions
The currently available WorkPlane functions are described in detail in this section.
-
vis_WorkPlane *
vis_WorkPlaneBegin
(void) create an instance of a WorkPlane object
Create an instance of a WorkPlane object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL. The workplane is positioned at (0.0, 0.0, 0.0) and oriented with the x and y global coordinate axes.
Destroy an instance of a WorkPlane object using
void vis_WorkPlaneEnd (vis_WorkPlane *workplane)
Return the current value of a WorkPlane object error flag using
Vint vis_WorkPlaneError (vis_WorkPlane *workplane)
Returns: The function returns a pointer to the newly created WorkPlane object. If the object creation fails, NULL is returned.
-
void
vis_WorkPlaneEnd
(vis_WorkPlane *p) destroy an instance of a WorkPlane object
-
Vint
vis_WorkPlaneError
(vis_WorkPlane *p) return the current value of a WorkPlane object error flag
-
void
vis_WorkPlaneSetObject
(vis_WorkPlane *p, Vint objecttype, Vobject *object) set pointers to attribute objects
Set a pointer to an attribute object.
- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
Parameters: - p – Pointer to WorkPlane object.
- objecttype – The name of the object type to be set.
x=VIS_COLORMAP ColorMap object =VGL_DRAWFUN DrawFun object =VIS_TRANSMAP TransMap object =VIS_VISCONTEXT VisContext object
- object – Pointer to the object to be set.
-
void
vis_WorkPlaneSetParami
(vis_WorkPlane *p, Vint type, Vint param) set workplane display parameters
Set display parameters. The parameter
WORKPLANE_AXES
specifies that the workplane axes showing the orientation of the local 2D coordinate system will be drawn. By defaultWORKPLANE_AXES
is set toVIS_ON
.The parameter
WORKPLANE_GRID
specifies that a grid will be drawn for the workplane. By defaultWORKPLANE_GRID
is set toVIS_OFF
.The parameter
WORKPLANE_GRID_LINE
specifies that grid lines will be drawn for the workplane. By defaultWORKPLANE_GRID_LINE
is set toVIS_OFF
.The parameter
WORKPLANE_GRID_POINT
specifies that grid points will be drawn for the workplane. By defaultWORKPLANE_GRID_POINT
is set toVIS_OFF
.The parameter
WORKPLANE_GRID_SNAP
specifies that a point selected from the workplane will snap to the closest grid point. By defaultWORKPLANE_GRID_SNAP
is set toVIS_OFF
.The parameter
WORKPLANE_GRID_X_SPACE
specifies in world coordinates the x spacing between grid points or lines. By defaultWORKPLANE_GRID_X_SPACE
is set to 1.0.The parameter
WORKPLANE_ORIGIN
specifies that the origin will be drawn for the workplane. By defaultWORKPLANE_ORIGIN
is set toVIS_ON
.The parameter
WORKPLANE_OUTLINE
specifies that the outline of the workplane will be drawn. By defaultWORKPLANE_OUTLINE
is set toVIS_ON
.- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
Parameters: - p – Pointer to WorkPlane object.
- type – Type of display parameter to set
x=WORKPLANE_AXES Draw workplane axes =WORKPLANE_GRID Draw workplane grid =WORKPLANE_GRID_LINE Draw grid as lines =WORKPLANE_GRID_POINT Draw grid as points =WORKPLANE_GRID_SNAP Snap to grid location =WORKPLANE_ORIGIN Draw workplane origin =WORKPLANE_OUTLINE Draw workplane outline
- param – Specifies the integer value that type will be set to.
x=VIS_OFF Turn parameter off =VIS_ON Turn parameter on
-
void
vis_WorkPlaneSetOriginPlane
(vis_WorkPlane *p, Vfloat origin[3], Vfloat right[3], Vfloat up[3]) set workplane origin and plane
Set workplane plane. The workplane plane is specified as the plane formed by the right and up vectors passing through the point origin. The right vector defines the x’ direction. The y’ direction lies in the workplane plane perpendicular to x’. The cross product of the x’ and y’ vectors is in the same direction as the cross product of the right and up vectors. The right and up vectors need not be normalized or orthogonal but they cannot be parallel. By default the origin is (0.,0.,0.), the right vector is (1.,0.,0.) and the up vector is (0.,1.,0.).
Get origin, right and up as output arguments using
void vis_WorkPlaneGetOriginPlane (vis_WorkPlane *workplane, Vfloat origin[3], Vfloat right[3], Vfloat up[3])
- Errors
VIS_ERROR_COMPUTE
is generated if right and up do not define a unique workplane plane.
Parameters: - p – Pointer to WorkPlane object
- origin – Point at which the workplane origin will be located
- right – Vector in direction of workplane local x’ axis.
- up – Vector in direction of workplane local y’ axis.
-
void
vis_WorkPlaneGetOriginPlane
(vis_WorkPlane *p, Vfloat origin[3], Vfloat right[3], Vfloat up[3]) get workplane origin and plane
-
void
vis_WorkPlaneSetDimensions
(vis_WorkPlane *p, Vfloat xspace, Vfloat yspace, Vfloat negwidth, Vfloat poswidth, Vfloat negheight, Vfloat posheight) set workplane height, width, spacing
Set workplane dimensions. The negative and positive widths and heights are measured from the work plane origin. The dimensions negwidth and poswidth are the width of the workplane in the negative and positive x direction. The dimensions negheight and posheight are the height of the workplane in the negative and positive y direction. By default xspace and yspace are set to 1. and the positive and negative widths and heights are set to 2.
Get defined dimensions as output arguments using
void vis_WorkPlaneGetDimensions (vis_WorkPlane *workplane, Vfloat *xspace, Vfloat *yspace, Vfloat *negwidth, Vfloat *poswidth, Vfloat *negheight, Vfloat *posheight)
- Errors
VIS_ERROR_VALUE
is generated if any input is less than or equal to zero.
Parameters: - p – Pointer to WorkPlane object.
- xspace – Grid x spacing
- yspace – Grid y spacing
- negwidth – Negative width
- poswidth – Positive width
- negheight – Negative height
- posheight – Positive height
-
void
vis_WorkPlaneGetClipPlane
(vis_WorkPlane *p, Vfloat eqn[4]) get clipping plane equation
Get plane equation. The plane equation is this form is useful for defining clipping planes in a graphics subsystem.
Parameters: - p – Pointer to WorkPlane object.
- eqn – [out] Plane equation.
-
void
vis_WorkPlaneGetPlane
(vis_WorkPlane *p, Vfloat pln[4][3]) get four corners of workplane
Get coordinates of the 4 corners of the workplane. This function is useful for obtaining a specification of the workplane which may be passed directly to the Space object function
vis_SpacePlaneGroup()
to query for all element entities lying on the plane.Parameters: - p – Pointer to WorkPlane object.
- pln – [out] Array of coordinate locations of workplane corners
-
void
vis_WorkPlaneDrag
(vis_WorkPlane *p, Vint action, Vint drag, Vint ix, Vint iy) drag the workplane
Perform a stage, specified by drag, of a dragger action as a function of device coordinate location ix and iy. When the drag stage
VIS_DRAG_TERM
is used the drag action is ignored and the internal drag action is set toVIS_ACTION_NONE
. The drag actionVIS_ACTION_ROTATE
only operates when the manipulation handles in the plane of the workplane are hit. The functionvis_WorkPlaneDraw()
is called internally by this function.Parameters: - p – Pointer to WorkPlane object
- action – [out] Drag action
x=VIS_ACTION_TRANSLATE Translate workplane =VIS_ACTION_ROTATE Rotate workplane
- drag – [out] Drag stage
x=VIS_DRAG_INIT Dragger initialization =VIS_DRAG_MOVE Dragger movement =VIS_DRAG_TERM Dragger termination
- ix – [out] Horizontal device coordinate
- iy – [out] Vertical device coordinate
-
void
vis_WorkPlaneCurrentDrag
(vis_WorkPlane *p, Vint *action, Vint *drag) get current drag status
Return current drag action and stage used in the last call to
vis_WorkPlaneDrag()
.Parameters: - p – Pointer to WorkPlane object
- action – [out] Current drag action
- drag – [out] Current drag stage
-
void
vis_WorkPlaneDraw
(vis_WorkPlane *p) draw the workplane
Draw the workplane.
Parameters: p – Pointer to WorkPlane object
-
void
vis_WorkPlaneTestHit
(vis_WorkPlane *p, Vint ix, Vint iy, Vint *hit, Vfloat pt[3]) test for handle hit
Select a point from the workplane. The input point will be a location given in device coordinates. Device coordinates are expressed in terms of pixel coordinates on a graphics device. The returned point will be constrained to the workplane and will lie on a grid point if snap is on. If the selected screen point is outside of the workplane boundary then the closest point on the workplane to the point will be returned. If the hit flag is non-zero then use
vis_WorkPlaneGetHit()
to find the hit grid point and/or manipulation handle. Usevis_WorkPlaneGridPoint()
to find the coordinate of any hit grid point. The hit grid point and/or manipulation handle will be highlighted when drawn usingvis_WorkPlaneDraw()
.Parameters: - p – Pointer to WorkPlane object
- ix – Horizontal device coordinate
- iy – Vertical device coordinate
- hit – [out] Hit flag
- pt – [out] Returned point on workplane in world coordinates
-
void
vis_WorkPlaneInitHit
(vis_WorkPlane *p) initialize internal hit status
Initialize hit status. This sets the grid point and manipulation handle hit status to zero.
Parameters: p – Pointer to WorkPlane object
-
void
vis_WorkPlaneGetHit
(vis_WorkPlane *p, Vint *igrid, Vint *jgrid, Vint *thand) get internal hit status
Get grid point and/or manipulation handle detected by a previous call to
vis_WorkPlaneTestHit()
. The returned thand argument is zero if no manipulation handle is hit. The value is 1 for the x-axis arrow, 2 for the y-axis arrow and 3 for the plane normal arrow.Parameters: - p – Pointer to WorkPlane object
- igrid – [out] Horizontal grid point
- jgrid – [out] Vertical grid point
- thand – [out] Manipulation handle
-
void
vis_WorkPlaneNumGrid
(vis_WorkPlane *p, Vint *numi, Vint *numj) get the number of grid points for a workplane
Get the number of grid points for a workplane.
Parameters: - p – Pointer to WorkPlane object
- numi – [out] Number of grid points in the local x’ direction.
- numj – [out] Number of grid points in the local y’ direction.
-
void
vis_WorkPlaneGridPoint
(vis_WorkPlane *p, Vint i, Vint j, Vfloat pt[3]) get a grid point from a workplane
Get a grid point in world coordinates from a workplane given an i and j index. The grid points for a workplane can be thought of as a 2D array of points which can be accessed as a zero-based array (e.g., grid[i][j]). (0, 0) is located at the lower left of the workplane. The function
vis_WorkPlaneNumGrid()
provides the valid limits of the indicies which are (0 <= i < num_i) and (0 <= j < num_j).- Errors
VIS_ERROR_VALUE
is generated if i or j is not within the valid limits.
Parameters: - p – Pointer to WorkPlane object
- i – I index for the grid point
- j – J index for the grid point
- pt – [out] Returned grid point in world coordinates at i,j