10. User Input and Interaction - IActor,Popup
The IActor and Popup modules are designed to facilitate the implementation of certain elements of the graphical user interface. They support graphical user interactions resulting from graphics input which occurs in the graphics window. In particular, the IActor module provides functions for user object manipulation. This includes object rotation, translation, scaling, centering, zooming, windowing, fitting, etc. The Popup module implements a simple, lightweight and portable popup menu facility.
The actual event or message handling is not performed by the interaction modules but is left as the responsibility of the host application. The user therefore must manage all graphics input and call the associated IActor or Popup functions depending upon the "bindings" of input events and actions which the user has determined are appropriate for the host application.
10.1 Object Interaction - IActor
The interaction module, IActor, is designed to allow the user to bind specified sequences of graphics input events such as button, mouse movement and expose events to one of several object manipulation actions provided by the IActor module. Examples of object manipulation actions available are a trackball interface, window resizing, object centering, fitting an object to a window, panning, zooming, etc. In addition the IActor module may be used to manage clipping planes. The functions associated with a IActor module are the following.
-
Begin and end an instance of an object, return object error flag
*vgl_IActorBegin - create an instance of a IActor object vgl_IActorEnd - destroy an instance of a IActor object vgl_IActorError - return IActor object error flag
-
Drawing functions, attributes, query
vgl_IActorClick - control clicker actions vgl_IActorDisplay - call display callback vgl_IActorDrag - control dragger actions vgl_IActorDrawBackground - draw drop background vgl_IActorDrawClipCenter - draw clip plane center icon vgl_IActorDrawRectangle - draw last drag rectangle vgl_IActorDrawRadialBackground - draw a radial background vgl_IActorDrawRotCenter - draw triad at the center of rotation vgl_IActorDrawTrackBall - draw trackball vgl_IActorResize - resize function vgl_IActorFit - fit function vgl_IActorFitNearFar - fit near and far clipping planes function vgl_IActorFitTrackBall - fit trackball function vgl_IActorGetFloat - query float information vgl_IActorGetInteger - query integer information vgl_IActorProject - project a point to device coordinates vgl_IActorUnproject - unproject a point from device coordinates vgl_IActorSetFunction - set a call back function vgl_IActorSetObject - set attribute object GetObject - get attribute object vgl_IActorSetParamf - set display parameters vgl_IActorSetParamfv - set display parameters vgl_IActorSetParami - set display parameters vgl_IActorSpin - spin function vgl_IActorTransformInit - initialize modelview matrix vgl_IActorTransformView - specify modelview matrix
In addition to the utility functions, the IActor module supports the implementation of several user object manipulation actions. These actions may be categorized as either "clicker" or "dragger" actions. Clicker actions are meant to be performed when a button is pressed. Use the vgl_IActorClick function to implement clicker actions. Dragger actions are meant to be initiated when a button is depressed, then the cursor location is repeatedly queried and some action based upon the mouse movement and the mouse position is performed. The dragging action normally terminates when the button is released. Use the vgl_IActorDrag function to implement dragger actions. The IActor module allows all graphics input functions such as button, mouse movement and expose events to be managed by the user.
The IActor module provides for a display callback functions to be invoked under upon the completion of all clicker actions and the movement and termination stages of most dragger actions. The display callback function is set using vgl_IActorSetFunction
The actions currently available are are described as follows:
- IACTOR_CENTER, this clicker action translates the display so that the object location under the specified device coordinate is moved to the center of the viewport.
- IACTOR_CLIPPLANETRANSLATE, this dragger action translates the clip plane in the direction of the clip plane normal.
- IACTOR_CLIPPLANETRANSLATE_DEGEN, this dragger action translates the clip plane in the direction of the clip plane normal when the normal is nearly parallel to the viewing direction.
- IACTOR_CLIPPLANETRANSLATE_INPLANE, this dragger action translates the clip plane in the plane of the clip plane.
- IACTOR_CLIPPLANEROTATE, this dragger action rotates the clip plane about a vector in the plane of the clip plane.
- IACTOR_CLIPPLANEROTATE_DEGEN, this dragger action rotates the clip plane about a vector in the plane of the clip plane when the vector is nearly perpendicular to the viewing direction.
- IACTOR_DOLLY, this dragger action translates the object in the depth direction.
- IACTOR_DOLLYCENTER, this dragger action is like IACTOR_DOLLY except that the object dolly about the screen center rather than the rotation center.
- IACTOR_DOLLYRATE, this dragger action is like IACTOR_DOLLY except that the depth translation occurs continuously at a rate proportional to the distance of the device coordinate location from the center of the window.
- IACTOR_FARPLANE, this dragger action translates the far clipping plane.
- IACTOR_FIT, this clicker action sets the render mode to VGL_EXTENT, renders the display and computes the object extent. The render mode is set to VGL_RENDER and the object is translated and scaled to comfortably fit the viewport dimensions.
- IACTOR_FRAME, this dragger action allows a user to drag a frame (window) anchored at the device coordinate specified at dragger initialization and at the other by the device coordinate specified during mouse movements. Then at dragger termination the object is translated and scaled so that the current viewport fills the frame. This is the inverse of the IACTOR_WINDOW operation.
- IACTOR_NEARPLANE, this dragger action translates the near clipping plane.
- IACTOR_PAN, this dragger action pans the projection so that it tracks dragger movements exactly.
- IACTOR_ROTATE, this dragger action rotates the object about the screen x and y axes in response to mouse movements in the horizontal and vertical directions. Rotation about the z axis occurs when the device coordinate location is near the window margins when dragger initialization occurs. The object z rotation then tracks the specified device coordinate location.
- IACTOR_ROTATERATE, this dragger action is like IACTOR_ROTATE except that the rotation occurs continuously at a rate proportional to the distance of the device coordinate location from the center of the window.
- IACTOR_SCALE, this dragger action scales the object uniformly in response to dragger movement. Dragging the device coordinate location primarily upward or to the left scales the object up, dragging the device coordinate location downward or to the right scales the object down.
- IACTOR_SCALECENTER, this dragger action is like IACTOR_SCALE except that the object scale about the screen center rather than the rotation center.
- IACTOR_SCALERATE, this dragger action is like IACTOR_SCALE except that the scaling occurs continuously at a rate proportional to the distance of the device coordinate location from the center of the window.
- IACTOR_SQUIGGLE, this dragger action allows a user to free hand draw a line, the horizontal and vertical extents of which are used to compute a window. Then at dragger termination, like the IACTOR_WINDOW action, the object is translated and scaled so that the window formed by the line extents fills the current viewport.
- IACTOR_TRACKBALL, this dragger action emulates a virtual trackball interface for rotating the object.
- IACTOR_TRANSFORMINIT, this clicker action loads the matrix stack with a matrix in which specified components, (eg rotation, translation, scaling) have been initialized.
- IACTOR_TRANSLATE, this dragger action translates the object so that it tracks dragger movements exactly.
- IACTOR_TRANSLATERATE, this dragger action is like IACTOR_TRANSLATE except that the translation occurs continuously at a rate proportional to the distance of the device coordinate location from the center of the window.
- IACTOR_WINDOW, this dragger action allows a user to drag a window anchored at the device coordinate specified at dragger initialization and at the other by the device coordinate specified during mouse movements. Then at dragger termination the object is translated and scaled so that the window fills the current viewport. This is the inverse of the IACTOR_FRAME operation.
- IACTOR_ZOOM, this dragger action zooms the projection uniformly in response to dragger movement. Dragging the device coordinate location primarily upward or to the left zooms into the object, dragging the device coordinate location downward or to the right zooms away from the object.
- IACTOR_ZOOMCENTER, this dragger action is like IACTOR_ZOOM except that the zoom is performed toward the screen center rather than the rotation center. The zoom is performed by altering the projection matrix.
10.2 Managing Arbitrary Clip Planes
The IActor module supports the management of arbitrary clipping planes. A clipping plane is defined by a point in world coordinates and a normal vector at that point. Use vgl_IActorSetParamfv to specify the point and normal. The basic clipping plane interactions are to translate the clipping plane in the direction of the normal or in the plane of the clipping plane (perpendicular to the normal) and to rotate the clipping plane about a specified vector which lies in the plane of the clipping plane. All clip plane motions are performed using vgl_IActorDrag with one of five possible dragger actions.
IACTOR_CLIPPLANETRANSLATE IACTOR_CLIPPLANETRANSLATE_DEGEN IACTOR_CLIPPLANETRANSLATE_INPLANE IACTOR_CLIPPLANEROTATE IACTOR_CLIPPLANEROTATE_DEGENThe IActor module provides a function, vgl_IActorDrawClipCenter, to draw an icon representing the clip plane center and to detect the type of clip plane interaction to be performed. The type of clip plane interaction detected depends upon the mouse location on the clip plane icon. The appearance of the icon will change dependent upon the interaction which is detected by the function call. If a rotation action is detected, the components of the rotation vector are returned. Note that the use of the vgl_IActorDrawClipCenter is optional and is only provided as a convenience for drawing a clip plane icon and providing a reasonable means of detecting a dragger option for subsequent use with vgl_IActorDrag.
If the mouse is within the boundary of the center sphere, then the center of the clip plane is translated. If the mouse is within half the radius of the center sphere, then translation along the normal to the clip plane is detected. If normal translation is detected and the normal is nearly parallel to the viewing direction, then the icon on the right in Figure 10-1a is drawn. If the mouse is between half the radius and the full radius of the center sphere, then translation in the plane of the clip plane is detected and the icon in Figure 10-1b is drawn.
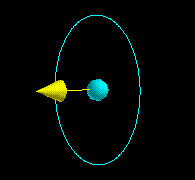
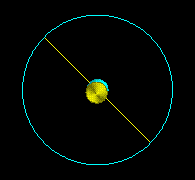
Figure 10-1a, Clip Plane Icon Normal Translation and in Viewing Direction
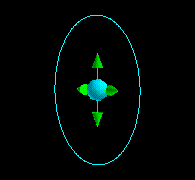
Figure 10-1b, Clip Plane Icon in Plane Translation
If the mouse is outside the boundary of the center sphere and within the neighborhood of the clip plane circle, then rotation about a vector lying in the plane of the clip plane is detected. The rotation vector is perpendicular to a line drawn from the clip plane center to a point on the clip plane icon circle which is closest to the mouse location. If rotation is detected and the rotation vector is nearly perpendicular to the viewing direction, then the icon on the right in Figure 10-1c is drawn.
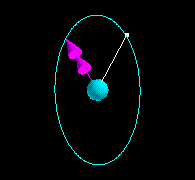
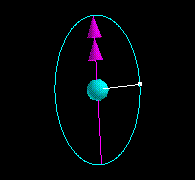
Figure 10-1c, Clip Plane Icon Rotation and about Vector in Viewing Plane
Given a clip plane action, use vgl_IActorDrag to help manipulate the clipping plane. The IActor object will not call any drawing functions related to clipping planes internally such as vgl_DrawFunClipPlane. Instead, IActor will change the values of the clipping plane center and normal which have been initially set using vgl_IActorSetParamfv. Use the function vgl_IActorGetFloat to query for the new locations of the clipping plane center and normal. If a rotation action is to be performed then a rotation vector must be specified using vgl_IActorSetParamfv. If vgl_IActorDrawClipCenter has been used, this rotation vector will be returned by the function. The colors of the various components of the clipping plane icon may be customized using the function vgl_IActorSetParamfv.10.3 Function Descriptions
The currently available IActor functions are described in detail in this section.IActor
NAME
*vgl_IActorBegin - create an instance of a IActor objectC SPECIFICATION
vgl_IActor *vgl_IActorBegin ()
ARGUMENTS
None
FUNCTION RETURN VALUE
The function returns a pointer to the newly created IActor object. If the object creation fails, NULL is returned.DESCRIPTION
Create an instance of a IActor object. Memory is allocated for the object private data and the pointer to the data is returned. The display callback function is set to NULL. The rotation center is set to the origin and the trackball radius is unity.Destroy an instance of a IActor object using
void vgl_IActorEnd (vgl_IActor *iactor)
Return the current value of a IActor object error flag using
Vint vgl_IActorError (vgl_IActor *iactor)
IActor
NAME
vgl_IActorClick - control clicker actionsC SPECIFICATION
void vgl_IActorClick (vgl_IActor *iactor, Vint action, Vint px, Vint py)
INPUT ARGUMENTS
iactor Pointer to IActor object. action Action type =IACTOR_CENTER Translate point to center of screen =IACTOR_ROTATERATE Rotate about screen x and y at a rate relative to mouse position. =IACTOR_SCALERATE Scale relative to mouse position. =IACTOR_SCALEBYFACTOR Scale about mouse position by a given factor. =IACTOR_TRANSLATERATE Translate in screen xy plane relative to mouse position. =IACTOR_NONE Take no action px Horizontal device coordinate. py Vertical device coordinate.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper action is specified.DESCRIPTION
Perform a clicker action as a function of device coordinate location px and py.The action, IACTOR_SCALEBYFACTOR, scales about the mouse position by a factor which is set using the IACTOR_SCALEFACTOR parameter with function vgl_IActorSetParamf.
No check is made on the input values of px and py. The display callback is invoked after the specified action is complete. No cursor changes are performed.
IActor
NAME
vgl_IActorDisplay - call display callbackC SPECIFICATION
void vgl_IActorDisplay (vgl_IActor *iactor, Vobject *dummy)
INPUT ARGUMENTS
iactor Pointer to IActor object. dummy Pointer to object which is ignored by this function
OUTPUT ARGUMENTS
None
DESCRIPTION
Invoke the display, IACTOR_FUN_DISPLAY, callback function.
IActor
NAME
vgl_IActorDrag - control dragger actionsC SPECIFICATION
void vgl_IActorDrag (vgl_IActor *iactor, Vint action, Vint drag, Vint px, Vint py)
INPUT ARGUMENTS
iactor Pointer to IActor object. action Action type =IACTOR_CLIPPLANETRANSLATE Translate normal to clipping plane =IACTOR_CLIPPLANETRANSLATE_DEGEN Translate normal to clipping plane =IACTOR_CLIPPLANETRANSLATE_INPLANE Translate in plane of clipping plane =IACTOR_CLIPPLANEROTATE Rotate clipping plane =IACTOR_CLIPPLANEROTATE_DEGEN Rotate clipping plane =IACTOR_DOLLY Translate in screen depth relative to mouse movement. =IACTOR_DOLLYCENTER Translate in screen depth about the center of the screen relative to mouse movement. =IACTOR_DOLLYRATE Translate in screen depth relative to mouse position. =IACTOR_FARPLANE Translate far clipping plane relative to mouse movement. =IACTOR_FRAME Translate and scale the viewport to a rectangular frame. =IACTOR_NEARPLANE Translate near clipping plane relative to mouse movement. =IACTOR_PAN Pan in screen xy plane relative to mouse movement. =IACTOR_ROTATE Rotate about screen x,y and z axes relative to mouse movement. =IACTOR_ROTATERATE Rotate about screen x and y at a rate relative to mouse position and about screen z relative to mouse movement. =IACTOR_SCALE Scale relative to mouse movement. =IACTOR_SCALECENTER Scale about screen center relative to mouse movement. =IACTOR_SCALERATE Scale relative to mouse position. =IACTOR_SQUIGGLE Translate and scale a rectangular extent. =IACTOR_TRACKBALL Simulate trackball manipulation =IACTOR_TRANSLATE Translate in screen xy plane relative to mouse movement. =IACTOR_TRANSLATERATE Translate in screen xy plane relative to mouse position. =IACTOR_WINDOW Translate and scale a rectangular window to the viewport. =IACTOR_ZOOM Zoom relative to mouse movement. =IACTOR_ZOOMCENTER Zoom about screen center relative to mouse movement. =IACTOR_NONE Take no action drag Drag stage =VGL_DRAG_INIT Dragger initialization =VGL_DRAG_MOVE Dragger movement =VGL_DRAG_TERM Dragger termination px Horizontal device coordinate. py Vertical device coordinate.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper action or drag is specified. VGL_ERROR_OPERATION is generated if a drag operation is specified out of order.DESCRIPTION
Perform a stage, specified by drag, of a dragger action as a function of device coordinate location px and py. The display callback is invoked after the VGL_DRAG_MOVE stage for all actions except IACTOR_FRAME, IACTOR_SQUIGGLE and IACTOR_WINDOW. The display callback is also invoked after the VGL_DRAG_TERM stage for all actions. The VGL_DRAG_MOVE stage should be called for all "rate" actions even if there is no change in the device coordinate location.Note that for the IACTOR_FRAME, IACTOR_SQUIGGLE and IACTOR_WINDOW actions, if the px and py values passed at the VGL_DRAG_INIT stage are the same as the values passed at the VGL_DRAG_TERM stage, then no translation or scaling is performed.
IActor
NAME
vgl_IActorDrawBackground - draw drop backgroundC SPECIFICATION
void vgl_IActorDrawBackground (vgl_IActor *iactor, Vfloat cbot[3], Vfloat ctop[3])
INPUT ARGUMENTS
iactor Pointer to IActor object. cbot Color of background at bottom of window ctop Color of background at top of window
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw a Gouraud shaded background polygon with RGB color cbot at the bottom of the window and ctop at the top of the window. The background polygon is drawn at the back of the viewing volume. Call this function for drawing the background before rendering the scene. Try cbot = (.5,.5,.5) and ctop = (0.,0.,.16) for a pleasing background.
IActor
NAME
vgl_IActorDrawClipCenter - draw clip plane center iconC SPECIFICATION
void vgl_IActorDrawClipCenter (vgl_IActor *iactor, Vint action, Vint px, Vint py, Vint *xaction, Vfloat xrotvec[3])
INPUT ARGUMENTS
iactor Pointer to IActor object. action Action type =IACTOR_CLIPPLANEDETECT Draw icon and detect clipping plane specific action. =IACTOR_CLIPPLANETRANSLATE Draw translate normal icon =IACTOR_CLIPPLANETRANSLATE_DEGEN Draw translate normal icon =IACTOR_CLIPPLANETRANSLATE_INPLANE Draw translate inplane icon =IACTOR_CLIPPLANEROTATE Draw rotate icon =IACTOR_CLIPPLANEROTATE_DEGEN Draw rotate icon =IACTOR_CLIPPLANEDATA Draw basic icon px Horizontal device coordinate. py Vertical device coordinate.
OUTPUT ARGUMENTS
xaction Detected specific action type =IACTOR_NONE No specific action detected =IACTOR_CLIPPLANETRANSLATE =IACTOR_CLIPPLANETRANSLATE_DEGEN =IACTOR_CLIPPLANETRANSLATE_INPLANE =IACTOR_CLIPPLANEROTATE =IACTOR_CLIPPLANEROTATE_DEGEN xrotvec Detected rotation vector
DESCRIPTION
Draw the clipping plane icon. The clipping plane icon consists of a center sphere and concentric circle which lies in the plane of the clipping plane. Use vgl_IActorSetParamfv is set the current clipping plane center and normal vector. The overall radius of the concentric circle is set in units of device coordinates (pixels) using vgl_IActorSetParami. There are 2 basic categories of input actions.The first category is clipping plane action detection using IACTOR_CLIPPLANEDETECT. In this case the clipping plane icon is drawn and the input device coordinates are used to detect a specific clipping plane action. If the input coordinates lie on the center sphere, a translation is detected. If the coordinates lie in the vicinity of the concentric circle, a rotation is detected. The detected action is returned in xaction and a rotation vector is return in xrotvec if a specific rotation action is detected. If the input coordinates lie outside of the icon, then IACTOR_NONE is returned. The icon is drawn with translation or rotation vectors drawn depending on the specific action detected.
The second category is a specific clipping plane action or no action using IACTOR_CLIPPLANETRANSLATE, IACTOR_CLIPPLANETRANSLATE_DEGEN, IACTOR_CLIPPLANETRANSLATE_INPLANE, IACTOR_CLIPPLANEROTATE, IACTOR_CLIPPLANEROTATE_DEGEN or IACTOR_CLIPPLANEDATA. In this case, the icon is drawn specific to the input action. The action IACTOR_CLIPPLANEDATA simply draws the basic icon with no rotation or translation vectors. The return values are undefined.
IActor
NAME
vgl_IActorDrawRadialBackground - draw a radial backgroundC SPECIFICATION
void vgl_IActorDrawRadialBackground (vgl_IActor *iactor, Vfloat ccenter[3], Vfloat cedge[3])
INPUT ARGUMENTS
iactor Pointer to IActor object. ccenter Color in center of window cedge Color at the edges (corners) of the window
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw a Gouraud shaded radial background with RGB color ccenter in the center of the window and cedge at the outer edges. This function will draw a circle using a number of triangles where the outer edge of the circle intersects the corners of the window. The background is drawn at the back of the viewing volume. Call this function for drawing the background before rendering the scene.
IActor
NAME
vgl_IActorDrawRectangle - draw last drag rectangleC SPECIFICATION
void vgl_IActorDrawRectangle (vgl_IActor *iactor)
INPUT ARGUMENTS
iactor Pointer to IActor object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw last rectangle rendered as a result of a IACTOR_WINDOW or IACTOR_FRAME drag action. It may be necessary to set XOR mode, line width, line style and color to match the rectangle generated during window and frame drag actions. Note that the window and drag operations both set the XOR mode on, the color to white and the line width to 2. Window drag actions set the line style to solid, VGL_LINESTYLE_SOLID, and frame drag actions set the line style to dotted, VGL_LINESTYLE_DOT.
IActor
NAME
vgl_IActorDrawRotCenter - draw triad at the center of rotationC SPECIFICATION
void vgl_IActorDrawRotCenter (vgl_IActor *iactor, Vint censiz)
INPUT ARGUMENTS
iactor Pointer to IActor object. censiz Size of a triad axis in pixels
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw a simple triad at the center of rotation. The axes point along the model x, y and z axes and are colored red, green and blue respectively by default. Try a pixel size of censiz = 50. The axis colors may be changed with vgl_IActorSetParamfv. The rotation center is calculated automatically when vgl_IActorFit is called. It may be set explicitly with vgl_IActorSetParamfv. It may be independently calculated with vgl_IActorFitTrackBall.
IActor
NAME
vgl_IActorDrawTrackBall - draw trackballC SPECIFICATION
void vgl_IActorDrawTrackBall (vgl_IActor *iactor)
INPUT ARGUMENTS
iactor Pointer to IActor object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw three concentric circles in the xy, yz and zx planes. The three circles are colored red, green and blue by default. The radius of each circle is the trackball radius. The circle colors may be changed with vgl_IActorSetParamfv. The IACTOR_COLOR_XAXIS color is associated with the circle in the xy plane, the IACTOR_COLOR_YAXIS color with the yz plane and the IACTOR_COLOR_ZAXIS color with the zx plane. The trackball radius is calculated automatically when vgl_IActorFit is called. It may be set explicitly with vgl_IActorSetParamf. It may be independently fit with vgl_IActorFitTrackBall.
IActor
NAME
vgl_IActorResize - resize functionC SPECIFICATION
void vgl_IActorResize (vgl_IActor *iactor, Vobject *dummy)
INPUT ARGUMENTS
iactor Pointer to IActor object. dummy Pointer to object which is ignored by this function
OUTPUT ARGUMENTS
None
DESCRIPTION
By default, this function is set as the callback function for an expose event. The function tests for a resizing of the window and adjusts the projection to account for a changing of the window aspect ratio. Then the display, IACTOR_FUN_DISPLAY, callback function is invoked.If a child window is to be resized in response to a resizing of its parent window then the desired size of the child window must be set using the ConfigureWindow drawing function prior to calling vgl_IActorResize.
IActor
NAME
vgl_IActorFit - fit functionC SPECIFICATION
void vgl_IActorFit (vgl_IActor *iactor, Vobject *dummy)
INPUT ARGUMENTS
iactor Pointer to IActor object. dummy Pointer to object which is ignored by this function
OUTPUT ARGUMENTS
None
DESCRIPTION
Change the modelview matrix and the projection far clipping plane to fit the current object to the size of the window. The center of rotation is set to the center of the object bounding box and the trackball radius is set to the distance from the center of rotation to a bounding box corner.If the fit type is IACTOR_FITTYPE_EXTENT or IACTOR_FITTYPE_RADIUS, the graphics subsystem is placed in VGL_EXTENT_DEVICE or VGL_EXTENT_FIT render mode and the display callback is invoked to compute the extent of the scene. The render mode is then set to VGL_RENDER.
If the fit type is IACTOR_FITTYPE_BOUNDBOX, then the user supplied bounding box is used which is set by calling vgl_IActorSetParamfv with IACTOR_BOUNDBOX. The render mode of the graphics subsystem is not changed.
A modelview matrix is computed and loaded which centers the scene in the viewing volume. Finally a polygon offset is computed and set so that line primitives appear clearly superimposed upon underlying polygon primitives.
Use the IACTOR_FITTYPE parameter to set the overall type of fit to be performed. The far clipping plane is adjusted depending upon the type of depth fitting selected. Use the IACTOR_FITZTYPE parameter to set the type of depth fit to be performed. Use vgl_IActorSetParami to set these parameters.
Use the IACTOR_FITZFACT parameter to adjust the padding used to place the far clipping plane and the IACTOR_FITZLENGTH parameter to specify a length to place the far clipping plane. Use vgl_IActorSetParamf to set these parameters.
Use the IACTOR_FITSHRINK parameter to shrink the fit model from the window margins. Use vgl_IActorSetParamf to set the shrink factor.
IActor
NAME
vgl_IActorFitNearFar - fit near and far clipping planes functionC SPECIFICATION
void vgl_IActorFitNearFar (vgl_IActor *iactor, Vobject *dummy)
INPUT ARGUMENTS
iactor Pointer to IActor object. dummy Pointer to object which is ignored by this function
OUTPUT ARGUMENTS
None
DESCRIPTION
Change the near and far projection clipping planes to fit the current object. If the projection is perspective, the left, right, bottom and top projection limits may be changed to preserve the angle of the field of view. The modelview matrix is unchanged.
IActor
NAME
vgl_IActorFitTrackBall - fit trackball functionC SPECIFICATION
void vgl_IActorFitTrackBall (vgl_IActor *iactor, Vobject *dummy)
INPUT ARGUMENTS
iactor Pointer to IActor object. dummy Pointer to object which is ignored by this function
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the radius of the trackball so that it is sized to the window. Use the IACTOR_FITSHRINK parameter to shrink the radius of the trackball from the window margins. Use vgl_IActorSetParamf to set the shrink factor.
IActor
NAME
vgl_IActorGetFloat - query float informationC SPECIFICATION
void vgl_IActorGetFloat (vgl_IActor *iactor, Vint type, Vfloat params[])
INPUT ARGUMENTS
iactor Pointer to IActor object. type Type of parameter to return =IACTOR_ROTCENTER Coordinates of rotation center. =IACTOR_ROTCENTERDEVICE Device coordinates of rotation center
OUTPUT ARGUMENTS
params Pointer to floating point return values
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
Query the current location of the center of rotation in either global coordinates or device coordinates.
IActor
NAME
vgl_IActorGetInteger - query integer informationC SPECIFICATION
void vgl_IActorGetInteger (vgl_IActor *iactor, Vint type, Vint params[])
INPUT ARGUMENTS
iactor Pointer to IActor object. type Type of parameter to return =IACTOR_DRAG Current Drag state
OUTPUT ARGUMENTS
params Pointer to floating point return values
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
Query the current state of the vgl_IActorDrag drag state. By default the drag state is VGL_DRAG_TERM.
IActor
NAME
vgl_IActorProject - project a point to device coordinatesC SPECIFICATION
void vgl_IActorProject (vgl_IActor *iactor, Vfloat w[3], Vfloat d[3])
INPUT ARGUMENTS
iactor Pointer to IActor object. w World coordinates
OUTPUT ARGUMENTS
d Device coordinates
DESCRIPTION
Transform the specified world coordinate to device coordinates using the current modelling, projection and viewport transformations. Use vgl_IActorUnproject to perform the inverse operation
IActor
NAME
vgl_IActorUnproject - unproject a point from device coordinatesC SPECIFICATION
void vgl_IActorUnProject (vgl_IActor *iactor, Vfloat d[3], Vfloat w[3])
INPUT ARGUMENTS
iactor Pointer to IActor object. d Device coordinates
OUTPUT ARGUMENTS
w World coordinates
DESCRIPTION
Transform the specified device coordinate to world coordinates using the current modelling, projection and viewport transformations. Use vgl_IActorProject to perform the inverse operation
IActor
NAME
vgl_IActorSetFunction - set a callback functionC SPECIFICATION
void vgl_IActorSetFunction (vgl_IActor *iactor, Vint funtype, void (*function)(vgl_IActor*, Vobject*), Vobject *object)
INPUT ARGUMENTS
iactor Pointer to IActor object. funtype Type of callback function to set =IACTOR_FUN_DISPLAY Display callback function Pointer to callback function object Pointer to the object to be returned as function argument
OUTPUT ARGUMENTS
None
DESCRIPTION
Set callback functions. By default the display callback is NULL. A callback is not invoked if it is NULL.
IActor
NAME
vgl_IActorSetObject - set attribute objectsC SPECIFICATION
void vgl_IActorSetObject (vgl_IActor *iactor, Vint objecttype, Vobject *object)
INPUT ARGUMENTS
iactor Pointer to IActor object. objecttype The name of the object type to be set. =VGL_DRAWFUN DrawFun object object Pointer to the object to be set.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OBJECTTYPE is generated if an improper objecttype is specified.DESCRIPTION
Set a pointer to an attribute object. The DrawFun attribute object is required.Get object as an output argument using
void vgl_IActorGetObject (vgl_IActor *iactor, Vint objecttype, Vobject **object)
IActor
NAME
vgl_IActorSetParamf - set floating display parametersC SPECIFICATION
void vgl_IActorSetParamf (vgl_IActor *iactor, Vint type, Vfloat param)
INPUT ARGUMENTS
iactor Pointer to IActor object. type Type of display parameter to set =IACTOR_FITSHRINK Fit shrink factor =IACTOR_FITZFACT Fit depth padding factor =IACTOR_FITZLENGTH Fit depth length =IACTOR_TRACKBALLRADIUS Trackball radius =IACTOR_DOLLYGAIN Gain of dolly actions =IACTOR_PLANEGAIN Gain of near and far plane actions =IACTOR_SCALEGAIN Gain of scale actions =IACTOR_SCALEFACTOR Scale factor param Specifies the float value that type will be set to.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified. VGL_ERROR_VALUE is generated if a shrink factor greater than or equal to 1. is specified.DESCRIPTION
Set trackball radius. By default IACTOR_TRACKBALLRADIUS is 1.Set fit shrink factor. The shrink factor is a value in the interval [0.,1.) which is used by vgl_IActorFit to determine the amount to shrink the object away from the viewport boundaries. A shrink factor of 0. results in no shrink. By default IACTOR_FITSHRINK is .1 .
Set depth padding factor using IACTOR_FITZFACT. The padding factor is used to set the far clipping plane so that the model comfortably fits in the center between the near and far clipping planes. The distance between the near and far clipping planes is the padding factor times the model extent in eye coordinates. Set IACTOR_FITZFACT to unity if the model extent is meant to be bounded exactly by the near and far clipping planes. The type of depth fitting is selected using function vgl_IActorSetParami with parameter IACTOR_FITZTYPE. Use a parameter value of IACTOR_FITZTYPE_FACT to enable depth padding factor fitting. By default IACTOR_FITZFACT is 100.
Set depth length using IACTOR_FITZLENGTH. The length is used to set the far clipping to be the depth length from the near clipping plane. The model is centered between the near and far planes. The type of depth fitting is selected using function vgl_IActorSetParami with parameter IACTOR_FITZTYPE. Use a parameter value of IACTOR_FITZTYPE_LENGTH to enable depth length fitting. By default IACTOR_FITZLENGTH is 1.
Set "gain" of scaling, near/far plane and dolly actions using IACTOR_SCALEGAIN, IACTOR_PLANEGAIN and IACTOR_DOLLYGAIN. The scale, plane and dolly gains are the incremental scale, plane and dolly factors applied per unit pixel moved. By default IACTOR_SCALEGAIN is .005 and IACTOR_PLANEGAIN and IACTOR_DOLLYGAIN are .001 .
Set scale factor used with the IACTOR_SCALEBYFACTOR clicker operation. By default IACTOR_SCALEFACTOR is 1.5 .
IActor
NAME
vgl_IActorSetParamfv - set floating display parametersC SPECIFICATION
void vgl_IActorSetParamfv (vgl_IActor *iactor, Vint type, Vfloat param[])
INPUT ARGUMENTS
iactor Pointer to IActor object. type Type of display parameter to set =IACTOR_BOUNDBOX Coordinates of bounding box =IACTOR_CLIPPLANECENTER Coordinates of clip plane center =IACTOR_CLIPPLANENORMAL Clip plane normal vector =IACTOR_CLIPPLANEROTVEC Clip plane rotation vector =IACTOR_COLOR_DRAG Color of squiggle. =IACTOR_COLOR_DRAGSTRIPE Color of squiggle stripe color =IACTOR_COLOR_XAXIS Color of x-axis =IACTOR_COLOR_YAXIS Color of y-axis =IACTOR_COLOR_ZAXIS Color of z-axis =IACTOR_ROTCENTER Coordinates of rotation center. =IACTOR_ROTCENTERDEVICE Device coordinates of rotation center =IACTOR_SPINMATRIX Modelview matrix modification used for each Spin operation. param Specifies the float values that type will be set to.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
Set the world coordinates of a bounding box. The bounding box is input as six world coordinate values, the minimum x,y,z coordinates followed by the maximum x,y,z coordinates The bounding box is used in conjunction with the fit type IACTOR_FITTYPE_BOUNDBOX set using vgl_IActorSetParami. By default IACTOR_BOUNDBOX is 0.,0.,0.,1.,1.,1.Set clipping plane center and normal using IACTOR_CLIPPLANECENTER and IACTOR_CLIPPLANENORMAL respectively. By default the center and normal are (0.,0.,0.). These values are changed by the vgl_IActorDrag function when a clipping plane action is performed. The current values may be queried using vgl_IActorGetFloat.
Set center of rotation. By default IACTOR_ROTCENTER is (0.,0.,0.). The center of rotation may be set in device coordinates using IACTOR_ROTCENTERDEVICE. The current rotation center may be queried in either global or device coordinates using vgl_IActorGetFloat.
Set colors of graphics associated with the squiggle drag operation. By default IACTOR_COLOR_DRAG is (1.,1.,1.) By default IACTOR_COLOR_DRAGSTRIPE is (1.,.2,.2)
Set colors of graphics associated with the rotation center and trackball icons. The x, y and z-axis colors affect the trackball circles in the xy, yz and zx planes respectively. By default IACTOR_COLOR_XAXIS is (1.,0.,0.), IACTOR_COLOR_YAXIS is (0.,1.,0.) and IACTOR_COLOR_ZAXIS is (0.,0.,1.).
Set the matrix which is used to increment the current modelview matrix for each time vgl_IActorSpin is called using IACTOR_SPINMATRIX.
IActor
NAME
vgl_IActorSetParami - set integer display parametersC SPECIFICATION
void vgl_IActorSetParami (vgl_IActor *iactor, Vint type, Vint iparam)
INPUT ARGUMENTS
iactor Pointer to IActor object. type Type of display parameter to set =IACTOR_CURSORS Display rotation, translation and scale cursors during display manipulation. =IACTOR_CLIPPLANEICONNORMAL Toggle drawing of clip plane icon normal vector =IACTOR_CLIPPLANEICONRADIUS Radius of clip plane icon in pixels =IACTOR_FITTYPE Fit type. =IACTOR_FITZTYPE Fit depth type. =IACTOR_FARADJUST Automatic far plane adjustment =IACTOR_LINEWIDTH_DRAG Linewidth of drag lines =IACTOR_NEARADJUST Automatic near plane adjustment =IACTOR_SMOOTHMOVE Smooth movement =IACTOR_SMOOTHSTEPS Number of smooth steps =IACTOR_INDPROJ Modify projection limits independently =IACTOR_USEPROJ Modify projection limits =IACTOR_USEFRONTBUFFER Toggle front buffer use =IACTOR_VERTDOLLY Dolly to vertical movements =IACTOR_VERTSCALE Scale to vertical movements =IACTOR_VERTZOOM Zoom to vertical movements iparam Specifies the integer value that type will be set to. =VGL_ON Enable =VGL_OFF Disable =IACTOR_FITTYPE_BOUNDBOX Model user supplied bounding box =IACTOR_FITTYPE_EXTENT Model projected extent =IACTOR_FITTYPE_RADIUS Model object radius =IACTOR_FITZTYPE_FACT Depth padding factor =IACTOR_FITZTYPE_LENGTH Depth length
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
Enable the automatic setting of the cursor to indicate a display rotation, translation or scaling. By default IACTOR_CURSORS is set to VGL_ON.Set fit type. A fit type of IACTOR_FITTYPE_EXTENT uses the projected extent of the model to fit to the window. The scaling of the model is then dependent upon its current orientation. A fit type of IACTOR_FITTYPE_RADIUS uses the radial distance of the object extent of the model to fit to the window. The scaling of the model is then independent of its current orientation. A fit type of IACTOR_FITTYPE_BOUNDBOX uses the projected bounding box supplied by the user using vgl_IActorSetParamfv with IACTOR_BOUNDBOX to fit to the window. The scaling of the model is then dependent upon its current orientation. By default IACTOR_FITTYPE is IACTOR_FITTYPE_EXTENT.
Set depth fit type. A depth fit type of IACTOR_FITZTYPE_FACT uses a depth padding factor supplied by the user using vgl_IActorSetParamf with IACTOR_FITZFACT to fit the near and far planes. A depth fit type of IACTOR_FITZTYPE_LENGTH uses a depth length supplied by the user using vgl_IActorSetParamf with IACTOR_FITZLENGTH to fit the near and far planes. By default IACTOR_FITZTYPE is IACTOR_FITZTYPE_FACT.
Use IACTOR_LINEWIDTH_DRAG to set the line width of lines drawn during the IACTOR_WINDOW, IACTOR_FRAME and IACTOR_SQUIGGLE drag operations. By default IACTOR_LINEWIDTH_DRAG is set to 2.
Use IACTOR_NEARADJUST and IACTOR_FARADJUST to enable the automatic adjustment of the near and far clipping planes when performing "dolly" drag operations. This automatic adjustment is designed to move the near and far clipping planes so that the model is not clipped. By default IACTOR_NEARADJUST and IACTOR_FARADJUST are set to VGL_OFF.
Use IACTOR_USEPROJ to enable the use of projection limit modifications rather than model view matrix modifications for IACTOR_CENTER click operations and IACTOR_WINDOW, IACTOR_FRAME and IACTOR_SQUIGGLE drag operations. By default IACTOR_USEPROJ is set to VGL_OFF.
Use IACTOR_INDPROJ to enable independent projection limit modifications IACTOR_WINDOW, IACTOR_FRAME and IACTOR_SQUIGGLE drag operations. If enabled the projection limits are modified independently without preserving aspect ratio. If disabled the projection limits are modified to preserve aspect ratio. By default IACTOR_INDPROJ is set to VGL_OFF.
Use IACTOR_USEFRONTBUFFER to toggle the use of the front buffer in squiggle and window graphics. By default IACTOR_USERFRONTBUFFER is set to VGL_ON.
Use IACTOR_SMOOTHMOVE to enable all projection modifications to be performed smoothly using a specified number of intermediate display callbacks. This option is available only for vgl_IActorFit, IACTOR_CENTER click operations and IACTOR_WINDOW, IACTOR_FRAME and IACTOR_SQUIGGLE drag operations with IACTOR_USEPROJ enabled. The number of intermediate display callbacks is specified using IACTOR_SMOOTHSTEPS. By default IACTOR_SMOOTHMOVE is set to VGL_OFF. By default IACTOR_SMOOTHSTEPS is set to 8.
Use IACTOR_VERTDOLLY, IACTOR_VERTSCALE and IACTOR_VERTZOOM to enable dolly, scaling and zooming to be sensitive to vertical mouse movements only. This option is available for all dolly, scale and zoom drag operations. By default IACTOR_VERTDOLLY, IACTOR_VERTSCALE and IACTOR_VERTZOOM are set to VGL_OFF.
Use IACTOR_CLIPPLANEICONNORMAL to toggle the display of the clip plane icon normal vector. By default IACTOR_CLIPPLANEICONNORMAL is set to VGL_OFF.
Use IACTOR_CLIPPLANEICONRADIUS to set the radius of the clip plane rotation icon in pixels. By default IACTOR_CLIPPLANEICONRADIUS is set to 50.
IActor
NAME
vgl_IActorSpin - spin functionC SPECIFICATION
void vgl_IActorSpin (vgl_IActor *iactor, Vobject *dummy)
INPUT ARGUMENTS
iactor Pointer to IActor object. dummy Pointer to object which is ignored by this function
OUTPUT ARGUMENTS
None
DESCRIPTION
This function reapplies the last rotation transformation produced by the IACTOR_TRACKBALL, IACTOR_ROTATE or IACTOR_ROTATERATE actions. The display callback function is invoked.
IActor
NAME
vgl_IActorTransformInit - initialize modelview matrixC SPECIFICATION
void vgl_IActorTransformInit (vgl_IActor *iactor, Vint action)
INPUT ARGUMENTS
iactor Pointer to IActor object. action Action type =IACTOR_ROTATE Initialize rotations =IACTOR_TRANSLATE Initialize translations =IACTOR_SCALE Initialize scale =IACTOR_TRANSFORMSNAP Snap rotations
OUTPUT ARGUMENTS
None
DESCRIPTION
Initialize various components of the current modelview matrix. The rotation components may be either set to the identity using action set to IACTOR_ROTATE or the rotation components can be set so that the rotation matrix is "snapped" to the closest screen oriented axes using action set to IACTOR_TRANSFORMSNAP. The translation components of the current modelview matrix may be initialized to zero using IACTOR_TRANSLATE. The scale components of the current modelview matrix may be initialized to unity using IACTOR_SCALE.The display callback function is invoked.
IActor
NAME
vgl_IActorTransformView - specify modelview matrixC SPECIFICATION
void vgl_IActorTransformView (vgl_IActor *iactor, Vint action, Vfloat tm[4][4])
INPUT ARGUMENTS
iactor Pointer to IActor object. action Action type =IACTOR_ROTATE Specify rotations =IACTOR_TRANSLATE Specify translations =IACTOR_SCALE Specify scale tm Modelview matrix
OUTPUT ARGUMENTS
None
DESCRIPTION
Specify various components of the current modelview matrix. The rotation, translation or scale components of the specified modelview matrix, tm, are enforced in the current modelview matrix.The display callback function is invoked.
10.4 Popup Menus - Popup
The Popup module has been provided as an efficient and portable method of supporting popup menus which are drawn under the cursor location in the graphics window as a result of a button press of some kind. Since popup menus are normally drawn in the graphics window under the cursor, they are generally not associated with any other user interface element. Popup menus are convenient in that a user need not move the mouse to another part of the graphics screen to make a selection. Instead, by pressing a mouse button for example, a popup menu is drawn under the cursor location. The popup menus support toggles, radio button arrays, cascading popups in addition to normal menu items such as one-shot buttons, titles and separators. The functions associated with a Popup module are the following.
-
Begin and end an instance of an object, return object error flag
*vgl_PopupBegin - create an instance of a Popup object vgl_PopupEnd - destroy an instance of a Popup object vgl_PopupError - return Popup object error flag
-
Drawing functions, attributes, query
vgl_PopupAddItem - add a button item vgl_PopupAddLine - add a line separator vgl_PopupAddPopup - add a cascading popup menu vgl_PopupAddRadio - add a radio button item vgl_PopupAddToggle - add a toggle button item vgl_PopupDrag - control dragger actions vgl_PopupGetIndex - get user index of selected item vgl_PopupSetActive - set activity of an item GetActive - get activity of an item vgl_PopupSetFunction - set a call back function vgl_PopupSetObject - set attribute object GetObject - get attribute object vgl_PopupSetParamfv - set display parameters vgl_PopupSetRadio - set radio button value GetRadio - get radio button value vgl_PopupSetToggle - set toggle button value GetToggle - get toggle button value vgl_PopupTitle - define popup title
A popup menu visually consists of a title; button, radio box, toggle and cascading menu items; and line separators. The menu items are defined using vgl_PopupAddItem, vgl_PopupAddRadio, vgl_PopupAddToggle and vgl_PopupAddPopup. Line separators are defined using vgl_PopupAddLine. The menu title is defined using vgl_PopupTitle. The title always appears at the top of the popup menu with a line separator drawn immediately below it. All other menu items and line separators are added to the popup menu below the title in the order in which they are defined.
Each menu item, (except for cascading popup menus) is assigned a user defined index, a label and a callback function which is invoked when the item is selected. The index of the selected item is not passed directly to the callback but must be queried for using vgl_PopupGetIndex. The menu item index is used by many functions as a unique "name" for the associated menu item. Toggle items are drawn with a small square box to the left of the label indicating the value of the toggle. Radio box items are drawn with a small diamond box to the left of the label indicating which item in the array is enabled. Cascading menu items are drawn with a pointer icon to the right of the item label.
The activity (selectability) of menu items may be changed using vgl_PopupSetActive. An item with its activity disabled is drawn with a lightened appearance and may not be selected. An example of a popup menu illustrating some item types and an inactive radio box array appears in Figure 10-1.
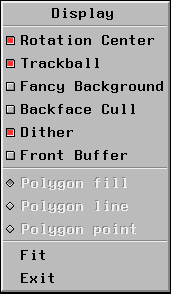
Figure 10-2, Example Popup Menu
The interaction with a popup menu is considered to be a dragger operation and is controlled by the function vgl_PopupDrag. Upon dragger initialization the popup menu is drawn with its upper left hand corner under the specified device coordinate. The position of the upper left hand corner may be altered in order to be able to draw the popup menu completely within the graphics window. This may occur if the specified device coordinate is too far to the left or bottom of the graphics window. Dragger movements will highlight the menu item under the device coordinate. At dragger termination the item under the device coordinate (if any) is selected. The current index of the selected item is set and its value altered if it is a radio box or toggle item. The associated item callback function is invoked. The Popup module provides for a display callback functions to be invoked upon the completion of a menu selection, ie dragger termination. The display callback function is set using vgl_PopupSetFunction10.5 Function Descriptions
The currently available Popup functions are described in detail in this section.Popup
NAME
*vgl_PopupBegin - create an instance of a Popup objectC SPECIFICATION
vgl_Popup *vgl_PopupBegin ()
ARGUMENTS
None
FUNCTION RETURN VALUE
The function returns a pointer to the newly created Popup object. If the object creation fails, NULL is returned.DESCRIPTION
Create an instance of a Popup object. Memory is allocated for the object private data and the pointer to the data is returned. The display callback function is set to NULL. The background RGB color is set to (.7,.7,.7).Destroy an instance of a Popup object using
void vgl_PopupEnd (vgl_Popup *popup)
Return the current value of a Popup object error flag using
Vint vgl_PopupError (vgl_Popup *popup)
Popup
NAME
vgl_PopupAddItem - add a button itemC SPECIFICATION
void vgl_PopupAddItem (vgl_Popup *popup, Vint index, Vtchar *label, void (*function)(vgl_Popup*, Vobject*), Vobject *object)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item label Item label function Pointer to callback function object Pointer to the object to be returned as function argument
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a simple button item. The button item is drawn as a label only. If selected, the index is set as the current index and the specified callback function is invoked. Use vgl_PopupGetIndex to return the current index.
Popup
NAME
vgl_PopupAddLine - add a line separatorC SPECIFICATION
void vgl_PopupAddLine (vgl_Popup *popup)
INPUT ARGUMENTS
popup Pointer to Popup object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a line separator. Line separators are used to visually group related menu items. Line separators should be used to delimit radio button item arrays. A line separator is automatically placed below the menu title.
Popup
NAME
vgl_PopupAddPopup - add a cascading popup menuC SPECIFICATION
void vgl_PopupAddPopup (vgl_Popup *popup, Vint index, Vtchar *label, vgl_Popup *caspopup)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item label Item label caspopup Pointer to cascading Popup object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a cascading popup menu item. The cascading menu item is drawn as a label followed by a right directed pointer icon. The caspopup popup menu is drawn to the right of the pointer icon when the device coordinate input to vgl_PopupDrag lies over this item.
Popup
NAME
vgl_PopupAddRadio - add a radio button itemC SPECIFICATION
void vgl_PopupAddRadio (vgl_Popup *popup, Vint index, Vint subindex, Vtchar *label, void (*function)(vgl_Popup*, Vobject*), Vobject *object)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item subindex Subindex identifier for menu item label Item label function Pointer to callback function object Pointer to the object to be returned as function argument
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a radio button item. The radio button item is drawn as a small diamond button icon followed by the item label. The diamond button icon indicates whether the radio button item is the currently selected item in the array. Each radio button item in an array must be assigned the same index and a unique subindex. By default the radio box item given subindex equal to 1 is set as the selected item.If selected, the index is set as the current index, the item becomes the currently selected item in the radio button array and the specified callback function is invoked. Use vgl_PopupGetIndex to return the current index. Use vgl_PopupGetRadio to return the currently selected item in the radio box array given the index. The currently selected item in a radio box array may be set using vgl_PopupSetRadio.
Popup
NAME
vgl_PopupAddToggle - add a toggle button itemC SPECIFICATION
void vgl_PopupAddToggle (vgl_Popup *popup, Vint index, Vtchar *label, void (*function)(vgl_Popup*, Vobject*), Vobject *object)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item label Item label function Pointer to callback function object Pointer to the object to be returned as function argument
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a toggle button item. The toggle button item is drawn as a small square button icon followed by the item label. The square button icon indicates the value of the toggle button item. By default the toggle item value is set to zero (off).If selected, the index is set as the current index, the value of the item is toggled and the specified callback function is invoked. Use vgl_PopupGetIndex to return the current index. Use vgl_PopupGetToggle to return the current value of the toggle item given the index. The value of a toggle may be set using vgl_PopupSetToggle.
Popup
NAME
vgl_PopupDrag - control dragger actionsC SPECIFICATION
void vgl_PopupDrag (vgl_Popup *popup, Vint drag, Vint px, Vint py)
INPUT ARGUMENTS
popup Pointer to Popup object. drag Drag stage =VGL_DRAG_INIT Dragger initialization =VGL_DRAG_MOVE Dragger movement =VGL_DRAG_TERM Dragger termination px Horizontal device coordinate. py Vertical device coordinate.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper drag is specified. VGL_ERROR_OPERATION is generated if the drag stages are not performed in the proper order.DESCRIPTION
Perform a stage, specified by drag, of a dragger action as a function of device coordinate location px and py. No check is made on the input values of px and py. When this function is called with drag set to VGL_DRAG_INIT the popup menu is displayed with its upper left hand corner under the specified device coordinate with the constraint that the entire popup must be able to be drawn in the graphics window. During dragger movements, VGL_DRAG_MOVE, the menu item under the device coordinate (if any) is highlighted. At dragger termination the highlighted item is selected and the associated item callback is invoked. The display callback is invoked after the VGL_DRAG_TERM stage. The display callback is used to erase the popup menu and refresh the graphics window.
Popup
NAME
vgl_PopupGetIndex - get user index of selected itemC SPECIFICATION
void vgl_PopupGetIndex (vgl_Popup *popup, Vint *index)
INPUT ARGUMENTS
popup Pointer to Popup object. index Index of current selected item
OUTPUT ARGUMENTS
None
DESCRIPTION
Get the user defined index of the last selected item. If no item is selected during a menu drag sequence, then index is returned as -1. For this reason user defined menu item indices should be restricted to non-negative numbers.
Popup
NAME
vgl_PopupSetActive - set activity of an itemC SPECIFICATION
void vgl_PopupSetActive (vgl_Popup *popup, Vint index, Vint flag)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item flag Flag to enable or disable menu item activity. =VGL_ON Enable =VGL_OFF Disable
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the activity of a menu item. If an item is inactive, the item label is drawn with a light, beveled appearance. In this state it can not be selected by a drag operation. When the item is active the label is drawn in black. By default all items are active when they are initially created.Get flag as an output argument using
void vgl_PopupGetActive (vgl_Popup *popup, Vint index, Vint *flag)
Popup
NAME
vgl_PopupSetFunction - set a callback functionC SPECIFICATION
void vgl_PopupSetFunction (vgl_Popup *popup, Vint funtype, void (*function)(vgl_Popup*, Vobject*), Vobject *object)
INPUT ARGUMENTS
popup Pointer to Popup object. funtype Type of callback function to set =POPUP_FUN_DISPLAY Display callback function Pointer to callback function object Pointer to the object to be returned as function argument
OUTPUT ARGUMENTS
None
DESCRIPTION
Set callback functions. By default the display callback is NULL. A callback is not invoked if it is NULL.
Popup
NAME
vgl_PopupSetObject - set attribute objectsC SPECIFICATION
void vgl_PopupSetObject (vgl_Popup *popup, Vint objecttype, Vobject *object)
INPUT ARGUMENTS
popup Pointer to Popup object. objecttype The name of the object type to be set. =VGL_DRAWFUN DrawFun object object Pointer to the object to be set.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OBJECTTYPE is generated if an improper objecttype is specified.DESCRIPTION
Set a pointer to an attribute object. The DrawFun attribute object is required.Get object as an output argument using
void vgl_PopupGetObject (vgl_Popup *popup, Vint objecttype, Vobject **object)
Popup
NAME
vgl_PopupSetParamfv - set display parametersC SPECIFICATION
void vgl_PopupSetParamfv (vgl_Popup *popup, Vint type, Vfloat param[])
INPUT ARGUMENTS
popup Pointer to Popup object. type Type of display parameter to set =POPUP_COLOR_BACKGROUND Set popup background RGB color. param Specifies the float values that type will be set to.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
Set the popup menu background color as an RGB color triple. By default the POPUP_COLOR_BACKGROUND color is light gray, (.7,.7,.7). The colors used for beveling which decorates the popup menu are derived from the base background color. It is best to use a background color which contains a component of gray (ie. no RGB component is zero) and is not close to white.
Popup
NAME
vgl_PopupSetRadio - set radio button valueC SPECIFICATION
void vgl_PopupSetRadio (vgl_Popup *popup, Vint index, Vint subindex)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item subindex Subindex identifier for menu item
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the currently selected item in a radio box array. Normally this operation need only be performed once for any one radio box array to set the initial state of the radio box array. By default subindex equal to 1 is set as the currently selected item in a radio box array when the item is created.Get subindex as an output argument using
void vgl_PopupGetRadio (vgl_Popup *popup, Vint index, Vint *subindex)
Popup
NAME
vgl_PopupSetToggle - set toggle button valueC SPECIFICATION
void vgl_PopupSetToggle (vgl_Popup *popup, Vint index, Vint flag)
INPUT ARGUMENTS
popup Pointer to Popup object. index Identifier for menu item flag Flag to enable or disable toggle menu item. =VGL_ON Enable =VGL_OFF Disable
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current value of a toggle menu item. Normally this operation need only be performed once for any one toggle item. to set the initial state of the toggle. By default a toggle value is zero (disabled) when the item is created.Get flag as an output argument using
void vgl_PopupGetToggle (vgl_Popup *popup, Vint index, Vint *flag)
Popup
NAME
vgl_PopupTitle - define popup titleC SPECIFICATION
void vgl_PopupTitle (vgl_Popup *popup, Vtchar *title)
INPUT ARGUMENTS
popup Pointer to Popup object. title Popup menu title
OUTPUT ARGUMENTS
None
DESCRIPTION
Define the title of the popup menu. The title is drawn centered at the top of the menu. A line separator is always drawn directly below the title. By default no title is defined.