6. Wire Frame and Shaded Surface Rendering - Edge, Face, Cell
VisTools supports the rendering of wireframe and shaded surface geometries with the Edge, Face and Cell modules. These modules are intended to be used primarily for drawing the edges and faces of finite element geometries, however they may also be used to draw the edges and faces of large structured curvilinear or rectilinear grids. There are no functions supported by Edge, Face and Cell directly which map color or transparency to field value. The visualization entities produced by these modules are subject to isosurface clipping. Edges and faces may be drawn with constant color or interpolated color.
The Edge, Face and Cell modules may be used to perform useful computations concerning finite element edges and faces. Edge tangents and face normals may be computed for lighting and other uses. Intersections and closest point locations with points and straight lines segments may be computed as an aid to interactive picking and probing. The natural coordinates of the point of intersection or closest point location is returned as part of the intersection process. Field data, input at the edge, face or cell nodes, may be interpolated to any point specified as natural coordinates.
6.1. Wire Frame Rendering - Edge
Use Edge to render wireframe geometry. Wireframe geometry is input as individual polylines. No specific functions are provided for the special cases of rectilinear or uniform edges. The functions associated with an Edge object are the following.
- Begin and end an instance of an object, return object error flag
vis_EdgeBegin()
- create an instance of a Edge objectvis_EdgeEnd()
- destroy an instance of a Edge objectvis_EdgeError()
- return Edge object error flag
- Operations
vis_EdgeComputeDist()
- compute equivalent nodal loadsvis_EdgeConvertDist()
- compute distributed tractionsvis_EdgeComputeTang()
- compute tangents to edgevis_EdgeCurv()
- draw curvilinear polylinevis_EdgeCurvColor()
- draw curvilinear, color interpolated polylinevis_EdgeInterpolate()
- interpolate field datavis_EdgeIntersectLine()
- compute closet point to input linevis_EdgeIntersectPoint()
- compute closet point to input pointvis_EdgeRST()
- compute natural coordinates of center and nodesvis_EdgeSetObject()
- set pointers to attribute objects.vis_EdgeSetTopology()
- set input edge topologyvis_EdgeTangRST()
- compute material tangents at a point.vis_EdgeTrav()
- traverse and draw a group of element edges.
Instance an Edge object using vis_EdgeBegin()
.
Set attribute objects using vis_EdgeSetObject()
.
The current edge topology is specified using vis_EdgeSetTopology()
.
Draw constant color edges using vis_EdgeCurv()
and color interpolated edges using vis_EdgeCurvColor()
.
The convenience routine, vis_EdgeTrav()
,
will traverse and draw a group of element edges.
The Edge
module supports a number of functions to support useful computations
performed upon edge geometry and data. Use vis_EdgeComputeTang()
to compute tangent vectors at edge nodes. The vis_EdgeIntersectLine()
and vis_EdgeIntersectPoint()
functions are designed to help applications interactively pick and probe
edge geometry and data. They return, among other information, the world
coordinate and natural coordinate points of intersection. Use
vis_EdgeInterpolate()
to compute field data at nodes to a specified edge natural coordinate
location. This function is designed to be used in conjunction with the
edge intersection functions.
Use the functions vis_EdgeComputeDist()
and
vis_EdgeConvertDist()
to compute equivalent nodal loads from distibuted loads and vice versa.
The element edges are assumed to be isoparametric. The function
vis_EdgeConvertDist()
is particularly useful for visualizing the distributed reactions from
the nodal reactions for higher order element edges.
6.2. Attribute Objects
An Edge object uses DrawFun, DataInt,
IsoClip VisContext and
ColorMap
objects to define attributes to generate an edge visualization entity. A
DrawFun object is required for edge drawing using vis_EdgeCurv()
and vis_EdgeCurvColor()
. The DataInt,
IsoClip, VisContext and
ColorMap attribute objects are optional. Use a
DataInt object to activate data interpolation. An
IsoClip
object is required if isosurface clipping is to be performed. If model
traversal is performed using vis_EdgeTrav()
, then a
GridFun
object is required and an
IdTran
object is optional for specifying entity color. An Edge
object uses the following VisContext components.
Color | Edge color |
DistTol | Distance tolerance used in intersection testing |
Draw | Flag to call graphics attribute and graphics primitive drawing functions. Use this flag to disable these drawing functions if only “Data” drawing functions are desired. |
Flags | OR the following flags: VIS_CYLINDERCAP generates disk-like caps at the ends of edges drawn with a LineStyle of VIS_CYLINDER. |
LineWidth | Line width of edges |
LineStyle | Line style of edges |
Refinement | Level of refinement |
Shrink | Shrink factor used during model traversal only |
ShrinkType | Shrink type used during model traversal only |
Size | Unit line width of cylindrical line style |
If the VisContext
attribute object pointer is NULL, then edges are generated without
reference to VisContext and ColorMap
objects. No graphics attribute drawing functions are invoked, all edges
are drawn using line graphics primitives with no refinement assuming the
current line width and line style, vis_EdgeCurv()
assumes the current color, vis_EdgeCurvColor()
uses the input colors at edge nodes.
Figure 6-1 illustrates edges drawn in a finite element model in which internal edges have been clipped to a spherical isosurface. The isosurface in this case has been rendered with fringes and contours of displacement.
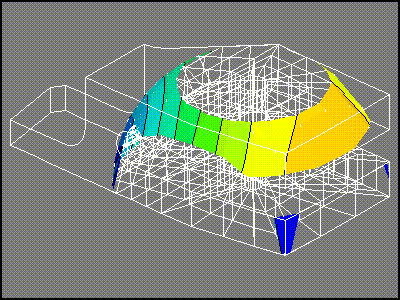
Figure 6-1, Edges Clipped to Spherical Isosurface
6.3. Function Descriptions
The currently available Edge functions are described in detail in this section.
-
vis_Edge *
vis_EdgeBegin
(void) create an instance of an Edge object
Create an instance of an Edge object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL. The edge topology is set to a line element, ie a simple straight line defined by two points.
Destroy an instance of a Edge object using
void vis_EdgeEnd (vis_Edge *edge)
Return the current value of a Edge object error flag using
Vint vis_EdgeError (vis_Edge *edge)
Returns: The function returns a pointer to the newly created Edge object. If the object creation fails, NULL is returned.
-
void
vis_EdgeEnd
(vis_Edge *p) destroy an instance of a Edge object
See
vis_EdgeBegin()
-
Vint
vis_EdgeError
(vis_Edge *p) return the current value of a Edge object error flag
See
vis_EdgeBegin()
-
void
vis_EdgeSetObject
(vis_Edge *p, Vint objecttype, Vobject *object) set pointers to attribute objects
Set a pointer to an attribute object. If an IsoClip attribute object is set then isosurface clipping is activated. To deactivate isosurface clipping, set a NULL IsoClip object pointer. If a DataInt object is set then data interpolation is activated. To deactivate data interpolation, set a NULL DataInt object pointer. The GridFun and IdTran objects are only used by
vis_EdgeTrav()
.- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
Parameters: - p – Pointer to Edge object.
- objecttype – The name of the object type to be set.
x=VIS_COLORMAP ColorMap object =VIS_DATAINT DataInt object =VGL_DRAWFUN DrawFun object =VIS_GRIDFUN GridFun object =VIS_IDTRAN_COLOR IdTran color map index object =VIS_IDTRAN_DATAINDEX IdTran data index object =VIS_ISOCLIP IsoClip object =VIS_VISCONTEXT VisContext object
- object – Pointer to the object to be set.
-
void
vis_EdgeSetTopology
(vis_Edge *p, Vint shape, Vint maxi) set edge topology
Set edge topology. All subsequent draw and computation methods assume edges of this topology. See section Computational Cells for a description of element topology conventions.
- Errors
VIS_ERROR_VALUE
is generated if a maxi less than 0 or equal to 1 is input.VIS_ERROR_ENUM
is generated if an improper shape is input.
Parameters: - p – Pointer to Edge object.
- shape – Edge shape parameter
x=VIS_SHAPELINE Line
- maxi – Topology parameter
-
void
vis_EdgeCurv
(vis_Edge *p, Vfloat x[][3]) draw curvilinear edge
Draw an edge with point locations x. The topology of the edge, in this case the number of points defining the polyline, is defined using
vis_EdgeSetTopology()
. The use ofvis_EdgeCurv()
draws an edge with the constant color specified in the visualization context. The functionvis_EdgeCurvColor()
draws an edge with color interpolation along the edge between the RGB color values, c, specified at the point locations, the color specified in the visualization context is ignored.Note that in the interest of efficiency,
vis_EdgeCurv()
does not issue a Color, LineWidth or LineStyle drawing function each time it is called to ensure that the edge to be drawn is rendered in the color, line width and line style in the current VisContext attribute object. Instead, Color, LineWidth and LineStyle drawing functions are issued only if the VisContext attribute object has been changed in any way since a previous call tovis_EdgeCurv()
. Therefore it is recommended to callvis_VisContextTouch()
with the VisContext attribute object before drawing a series of edges withvis_EdgeCurv()
.- Errors
VIS_ERROR_NULLOBJECT
is generated if no DrawFun attribute object has been set.
Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
-
void
vis_EdgeCurvColor
(vis_Edge *p, Vfloat x[][3], Vfloat c[][3]) draw curvilinear edge
See
vis_EdgeCurv()
- Errors
VIS_ERROR_NULLOBJECT
is generated if no DrawFun attribute object has been set.
Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
- c – Array of RGB color triples at point locations
-
void
vis_EdgeComputeTang
(vis_Edge *p, Vfloat x[][3], Vfloat v[][3]) compute tangents to edge
Compute tangents to a curvilinear edge. The normalized tangent vectors, v, are computed at point locations, x. A zero tangent vector is returned if the edge is degenerate at a point.
Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
- v – [out] Array of tangent vectors at each point.
-
void
vis_EdgeComputeDist
(vis_Edge *p, Vfloat x[][3], Vint nrws, Vfloat v[], Vfloat f[]) compute equivalent nodal loads
Compute equivalent nodal loads from distributed loads along an edge.
Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
- nrws – Number of distributed components per point
- v – Array of distributed loads.
- f – [out] Array of equivalent nodal loads.
-
void
vis_EdgeComputeDistdv
(vis_Edge *p, Vdouble x[][3], Vint nrws, Vdouble v[], Vdouble f[]) compute equivalent nodal loads
Compute equivalent nodal loads from distributed loads along an edge.
Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
- nrws – Number of distributed components per point
- v – Array of distributed loads.
- f – [out] Array of equivalent nodal loads.
-
void
vis_EdgeConvertDist
(vis_Edge *p, Vfloat x[][3], Vint nrws, Vfloat f[], Vfloat v[]) compute distributed tractions
Convert equivalent nodal loads to distributed loads along an edge. This function is the exact inverse of
vis_EdgeComputeDist()
.Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
- nrws – Number of distributed components per point
- f – Array of equivalent nodal loads.
- v – [out] Array of distributed loads.
-
void
vis_EdgeConvertDistdv
(vis_Edge *p, Vdouble x[][3], Vint nrws, Vdouble f[], Vdouble v[]) compute distributed tractions
Convert equivalent nodal loads to distributed loads along an edge. This function is the exact inverse of
vis_EdgeComputeDist()
.Parameters: - p – Pointer to Edge object.
- x – Array of point locations along polyline defining edge.
- nrws – Number of distributed components per point
- f – Array of equivalent nodal loads.
- v – [out] Array of distributed loads.
-
void
vis_EdgeIntersectLine
(vis_Edge *p, Vfloat x[][3], Vfloat xl[2][3], Vfloat *r, Vfloat xr[3], Vfloat xd[3], Vint *status) compute point of intersection
Compute the closest point on the edge defined by node point locations, x, to a line defined by end points, xl, or a point defined by xp. The natural coordinate, r, and the coordinates, xr of the closest point on the edge to the input line or point are returned. The distance vector, xd, from xr to the input point or the closest point on the input line is returned.
The case that the magnitude of xd is zero implies that the intersecting point or line actually intersected the edge.
The status variable is returned as unity if the length of vector xd is less than the DistTol element of the visualization context and zero otherwise. Use
vis_EdgeInterpolate()
to interpolate field data at nodes to the intersection point represented by natural coordinate r.Parameters: - p – Pointer to Edge object.
- x – Array of point locations along edge.
- xl – End points of intersecting line (xp for intersecting point)
- r – [out] Natural coordinate of closest point on edge.
- xr – [out] Coordinates at natural coordinate r.
- xd – [out] Vector from xr to point or closest point on line
- status – [out] Intersection status
-
void
vis_EdgeIntersectPoint
(vis_Edge *p, Vfloat x[][3], Vfloat xp[3], Vfloat *r, Vfloat xr[3], Vfloat xd[3], Vint *status) compute point of intersection
-
void
vis_EdgeInterpolate
(vis_Edge *p, Vfloat r, Vint nrws, Vfloat d[], Vfloat dr[]) interpolate field data
Interpolate nrws of field data, d given at each node point of the edge to the natural coordinate specified by r. A vector of nrws of interpolated values are returned in dr. The natural coordinate r spans the interval [-1.,1]. A natural coordinate outside of this interval will extrapolate the input field data to a point outside the endpoints of the edge.
Parameters: - p – Pointer to Edge object.
- r – Natural coordinate to interpolate field data to
- nrws – Row dimension of the field data array d.
- d – Array of field data at node point locations.
- dr – [out] Vector of interpolated field data at natural coordinate r.
-
void
vis_EdgeRST
(vis_Edge *p, Vfloat *rc, Vint *nix, Vfloat r[]) compute natural coordinates of center and nodes
Compute natural coordinates of center and nodes.
Parameters: - p – Pointer to Edge object.
- rc – [out] Natural coordinate of center
- nix – [out] Number of nodes
- r – [out] Natural coordinates at nodes.
-
void
vis_EdgeTangRST
(vis_Edge *p, Vfloat x[][3], Vfloat r, Vfloat g[3]) compute material tangents at a point.
Compute derivative, g, of the natural coordinate system with respect to the coordinates at a natural coordinate point, r. The vector returned in g is tangent to the natural coordinate direction.
Parameters: - p – Pointer to Edge object.
- x – Array of point locations along edge.
- r – Natural r coordinate of point
- g – [out] Material tangent.
-
void
vis_EdgeTrav
(vis_Edge *p, vis_Group *group) traverse and draw a group of element edges.
Traverse and draw a group of element edges specified in Group. The edges are drawn with the constant color specified in the visualization context unless an IdTran attribute object is set which contains a color map index for each element. All the edges in an element are drawn with this constant color. If a
VIS_IDTRAN_DATAINDEX
IdTran attribute object has been set then a DataIndex drawing function is called with the value contained in the IdTran object prior to drawing the edges for an element.The underlying model information is accessed using the GridFun attribute object. Set the GridFun and IdTran objects using
vis_EdgeSetObject()
. The visualization contexts Shrink and ShrinkType can be used to control shrink.- Errors
Parameters:
6.4. Shaded Surface - Face
Use Face to render shaded surfaces. Surface geometry is input as individual triangles or quadrilaterals or regular meshes of the same. The functions associated with a Face object are the following.
- Begin and end an instance of an object, return object error flag
vis_FaceBegin()
- create an instance of a Face objectvis_FaceEnd()
- destroy an instance of a Face objectvis_FaceError()
- return Face object error flag
- Operations
vis_FaceComputeDist()
- compute equivalent nodal loadsvis_FaceConvertDist()
- compute distributed tractionsvis_FaceComputeNorm()
- compute normals to facevis_FaceCurv()
- draw curvilinear facevis_FaceCurvColor()
- draw curvilinear, color interpolated facevis_FaceInterpolate()
- interpolate field datavis_FaceIntersectLine()
- compute closet point to input linevis_FaceIntersectPoint()
- compute closet point to input pointvis_FaceRST()
- compute natural coordinates of center and nodesvis_FaceSetObject()
- set pointers to attribute objects.vis_FaceSetTopology()
- set input face topologyvis_FaceTangRST()
- compute material tangents at a point.vis_FaceTrav()
- traverse and draw a group of element faces.
Instance an Face object using vis_FaceBegin()
.
Set attribute objects using vis_FaceSetObject()
.
The current face topology is specified using vis_FaceSetTopology()
.
Draw constant color faces using vis_FaceCurv()
and color interpolated faces using vis_FaceCurvColor()
.
The convenience routine, vis_FaceTrav()
,
will traverse and draw a group of element faces.
The Face
module supports a number of functions to support useful computations
performed upon face geometry and data. Use vis_FaceComputeNorm()
to compute normal vectors at face nodes. These normals are useful for
specialized light source shading effects, etc. The
vis_FaceIntersectLine()
and vis_FaceIntersectPoint()
functions are designed to help applications interactively pick and probe
face geometry and data. They return, among other information, the world
coordinate and natural coordinate points of intersection. Use
vis_FaceInterpolate()
to compute field data at nodes to a specified face natural coordinate
location. This function is designed to be used in conjunction with the
face intersection functions.
Use the functions vis_FaceComputeDist()
and
vis_FaceConvertDist()
to compute equivalent nodal loads from distibuted loads and vice versa.
The element faces are assumed to be isoparametric. The function
vis_FaceConvertDist()
is particularly useful for visualizing the distributed reactions from
the nodal reactions for higher order element faces.
6.5. Attribute Objects
A Face object uses DrawFun, DataInt,
IsoClip, VisContext,
ColorMap and TransMap
objects to define attributes to generate a face visualization entity. As
an option, the exterior edges of the face may be drawn. A DrawFun
object is required for face drawing using vis_FaceCurv()
and vis_FaceCurvColor()
.
The DataInt, IsoClip, VisContext
and ColorMap and TransMap
objects are optional. Use a DataInt
object to activate data interpolation. An IsoClip
object is required if isosurface clipping is to be performed. A
GridFun
object is required if model traversal is performed using
vis_FaceTrav()
.
If model traversal is performed using vis_FaceTrav()
,
then a GridFun object is required and
IdTran
objects are optional for specifying entity color and transparency. A
Face object uses the following VisContext
components.
Color | Face fill color |
DistTol | Distance tolerance used in intersection testing |
Draw | Flag to call graphics attribute and graphics primitive drawing functions. Use this flag to disable these drawing functions if only “Data” drawing functions are desired. |
Edge | Edge flag, if on the exterior edges of the face are drawn |
Fill | Fill flag, if off the surface of the face is not filled |
LineWidth | Line width of edges |
LineStyle | Line style of edges |
MinorColor | Edge color |
Refinement | Level of refinement |
Reflect | Flag to reverse sense of output polygons. This flag is useful to draw the bottom face of shell elements. |
Shade | Flag to apply light source shading |
Shrink | Shrink factor used during model traversal only |
ShrinkType | Shrink type used during model traversal only |
Size | Unit line width of cylindrical line style |
Trans | Face transparency |
If the VisContext
attribute object pointer is NULL, then faces are generated without
reference to VisContext, ColorMap
and TransMap
objects. No attribute drawing functions are invoked, all faces are drawn
using flat shaded polygon graphics primitives with no refinement
assuming the current transparency.
vis_FaceCurv()
assumes the current color,
vis_FaceCurvColor()
uses the input colors at face nodes.
Figure 6-2 illustrates both faces and edges drawn in a finite element model. The faces are clipped to one side of an isosurface, the edges to the other. The isosurface is an isosurface of displacement rendered with fringes and contours of stress.
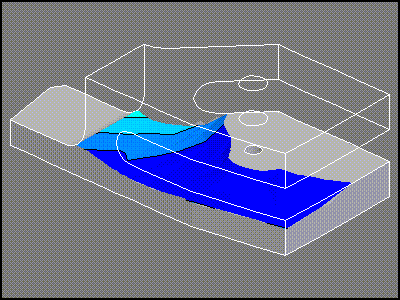
Figure 6-2, Faces and Edges Clipped to an Isosurface
6.6. Function Descriptions
The currently available Face functions are described in detail in this section.
-
vis_Face *
vis_FaceBegin
(void) create an instance of a Face object
Create an instance of a Face object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL and no isosurface clipping is active. The face topology is set to a 4 noded quadrilateral element.
Destroy an instance of a Face object using
void vis_FaceEnd (vis_Face *face)
Return the current value of a Face object error flag using
Vint vis_FaceError (vis_Face *face)
Returns: The function returns a pointer to the newly created Face object. If the object creation fails, NULL is returned.
-
void
vis_FaceEnd
(vis_Face *p) destroy an instance of a Face object
See
vis_FaceBegin()
-
Vint
vis_FaceError
(vis_Face *p) return the current value of a Face object error flag
See
vis_FaceBegin()
-
void
vis_FaceSetObject
(vis_Face *p, Vint objecttype, Vobject *object) set pointers to attribute objects
Set a pointer to an attribute object. If an IsoClip attribute object is set then isosurface clipping is activated. To deactivate isosurface clipping, set a NULL IsoClip object pointer. If a DataInt object is set then data interpolation is activated. To deactivate data interpolation, set a NULL DataInt object pointer. The GridFun and IdTran objects are only used by
vis_FaceTrav()
.- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
Parameters: - p – Pointer to Face object.
- objecttype – The name of the object type to be set.
x=VIS_COLORMAP ColorMap object =VIS_DATAINT DataInt object =VGL_DRAWFUN DrawFun object =VIS_GRIDFUN GridFun object =VIS_IDTRAN_COLOR IdTran color map index object =VIS_IDTRAN_DATAINDEX IdTran data index object =VIS_IDTRAN_TRANS IdTran transparency map index object =VIS_ISOCLIP IsoClip object =VIS_TRANSMAP TransMap object =VIS_VISCONTEXT VisContext object
- object – Pointer to the object to be set.
-
void
vis_FaceSetTopology
(vis_Face *p, Vint shape, Vint maxi, Vint maxj) set face topology
Set face topology. All subsequent draw and computation methods assume faces of this topology. See section Computational Cells for a description of element topology conventions.
- Errors
VIS_ERROR_VALUE
is generated if a maxi or maxj less than 0 or equal to 1 is input.VIS_ERROR_ENUM
is generated if an improper shape is input.
Parameters: - p – Pointer to Face object.
- shape – Face shape parameter
x=VIS_SHAPETRI Triangle =VIS_SHAPEQUAD Quadrilateral =VIS_SHAPEPOLYGON Polygon
- maxi – Topology parameter
- maxj – Topology parameter
-
void
vis_FaceCurv
(vis_Face *p, Vfloat x[][3], Vint vflag, Vfloat v[][3]) draw curvilinear face
Draw a face with point locations x and optional normals v. The topology of the face is defined using
vis_FaceSetTopology()
. The use ofvis_FaceCurv()
draws a face with the constant color specified in the visualization context. The functionvis_FaceCurvColor()
draws a face with color interpolation within the face between the RGB color values, c, specified at the point locations. If normals are not supplied by the user, this function will calculate them if the Shade visualization context is set toVIS_VERTEXSHADE
.Note that in the interest of efficiency,
vis_FaceCurv()
does not issue a Color or Trans drawing function each time it is called to ensure that the face to be drawn is rendered in the color and transparency in the current VisContext attribute object. Instead, Color and Trans drawing functions are issued only if the VisContext attribute object has been changed in any way since a previous call tovis_FaceCurv()
. Therefore it is recommended to callvis_VisContextTouch()
with the VisContext attribute object before drawing a series of faces withvis_FaceCurv()
.- Errors
VIS_ERROR_NULLOBJECT
is generated if no DrawFun attribute object has been set.
Parameters: - p – Pointer to Face object.
- x – Array of point locations defining face.
- vflag – User supplied face normal flag
x=VIS_NODATA No normals provided =VIS_VERTEXDATA Vertex normals are provided
- v – Array of user supplied normals
-
void
vis_FaceCurvColor
(vis_Face *p, Vfloat x[][3], Vfloat c[][3], Vint vflag, Vfloat v[][3]) draw curvilinear face
See
vis_FaceCurv()
Parameters: - p – Pointer to Face object.
- x – Array of point locations defining face.
- c – Array of RGB color triples at point locations.
- vflag – User supplied face normal flag
x=VIS_NODATA No normals provided =VIS_VERTEXDATA Vertex normals are provided
- v – Array of user supplied normals
-
void
vis_FaceIntersectLine
(vis_Face *p, Vfloat x[][3], Vfloat xl[2][3], Vfloat r[2], Vfloat xr[3], Vfloat xd[3], Vint *status) compute point of intersection
Compute the closest point on the face defined by node point locations, x, to a line defined by end points, xl, or a point defined by xp. The natural coordinates, r, and the coordinates, xr of the closest point on the face to the input line or point are returned. The distance vector, xd, from xr to the input point or the closest point on the input line is returned.
The case that the magnitude of xd is zero implies that the intersecting point or line actually intersected the face.
The status variable is returned as unity if the length of vector xd is less than the DistTol element of the visualization context and zero otherwise. Use
vis_FaceInterpolate()
to interpolate field data at nodes to the intersection point represented by natural coordinates r.Parameters: - p – Pointer to Face object.
- x – Array of point locations along face.
- xl – End points of intersecting line (xp for Intersecting point)
- r – [out] Natural coordinates of closest point on face.
- xr – [out] Coordinates at natural coordinates r.
- xd – [out] Vector from xr to point or closest point on line
- status – [out] Intersection status
-
void
vis_FaceIntersectPoint
(vis_Face *p, Vfloat x[][3], Vfloat xp[3], Vfloat r[2], Vfloat xr[3], Vfloat xd[3], Vint *status) compute point of intersection
-
void
vis_FaceInterpolate
(vis_Face *p, Vfloat r[2], Vint nrws, Vfloat d[], Vfloat dr[]) interpolate field data
Interpolate nrws of field data, d given at each node point of the face to the natural coordinates specified by r. A vector of nrws of interpolated values are returned in dr. The natural coordinates r span the interval [-1.,1] for quadrilateral shapes and [0.,1] for triangular shapes. A natural coordinate outside of these intervals will extrapolate the input field data to a point outside the geometric extent of the face.
Parameters: - p – Pointer to Face object.
- r – Natural coordinates to interpolate field data to
- nrws – Row dimension of the field data array d.
- d – Array of field data at node point locations.
- dr – [out] Vector of interpolated field data at natural coordinate r.
-
void
vis_FaceRST
(vis_Face *p, Vfloat rc[2], Vint *nix, Vfloat r[][2]) compute natural coordinates of center and nodes
Compute natural coordinates of center and nodes.
Parameters: - p – Pointer to Face object.
- rc – [out] Natural coordinates of center
- nix – [out] Number of nodes
- r – [out] Natural coordinates at nodes.
-
void
vis_FaceTangRST
(vis_Face *p, Vfloat x[][3], Vfloat r[2], Vfloat g[2][3]) compute material tangents at a point.
Compute derivatives, g, of the natural coordinate system with respect to the coordinates at a natural coordinate point, r. The vectors returned in g are tangent to the respective natural coordinate directions.
Parameters: - p – Pointer to Face object.
- x – Array of point locations along face.
- r – Natural r,s coordinates of point
- g – [out] Material tangents.
-
void
vis_FaceComputeNorm
(vis_Face *p, Vfloat x[][3], Vfloat v[][3]) compute normals to face
Compute normals to a curvilinear face. The normal vectors, v, are computed at point locations, x. A zero normal vector is returned if the face is degenerate at a point.
Parameters: - p – Pointer to Face object.
- x – Array of point locations defining face.
- v – [out] Array of normal vectors at each point.
-
void
vis_FaceComputeDist
(vis_Face *p, Vfloat x[][3], Vint nrws, Vfloat v[], Vfloat f[]) compute equivalent nodal loads
Compute equivalent nodal loads from distributed loads along a face.
Parameters: - p – Pointer to Face object.
- x – Array of point locations along polyline defining face.
- nrws – Number of distributed components per point
- v – Array of distributed loads.
- f – [out] Array of equivalent nodal loads.
-
void
vis_FaceComputeDistdv
(vis_Face *p, Vdouble x[][3], Vint nrws, Vdouble v[], Vdouble f[]) compute equivalent nodal loads
Compute equivalent nodal loads from distributed loads along a face.
Parameters: - p – Pointer to Face object.
- x – Array of point locations along polyline defining face.
- nrws – Number of distributed components per point
- v – Array of distributed loads.
- f – [out] Array of equivalent nodal loads.
-
void
vis_FaceConvertDist
(vis_Face *p, Vfloat x[][3], Vint nrws, Vfloat f[], Vfloat v[]) compute distributed tractions
Convert equivalent nodal loads to distributed loads along a face. This function is the exact inverse of
vis_FaceComputeDist()
.Parameters: - p – Pointer to Face object.
- x – Array of point locations along polyline defining face.
- nrws – Number of distributed components per point
- f – Array of equivalent nodal loads.
- v – [out] Array of distributed loads.
-
void
vis_FaceConvertDistdv
(vis_Face *p, Vdouble x[][3], Vint nrws, Vdouble f[], Vdouble v[]) compute distributed tractions
Convert equivalent nodal loads to distributed loads along a face. This function is the exact inverse of
vis_FaceComputeDist()
.Parameters: - p – Pointer to Face object.
- x – Array of point locations along polyline defining face.
- nrws – Number of distributed components per point
- f – Array of equivalent nodal loads.
- v – [out] Array of distributed loads.
-
void
vis_FaceTrav
(vis_Face *p, vis_Group *group, vis_ElemDat *elemdat) traverse and draw a group of element faces
Traverse and draw a group of element faces specified in Group with optional normals specified by elemdat. If the elemdat object is NULL this function will calculate face normals if the Shade visualization context is set to
VIS_VERTEXSHADE
.The faces are drawn with the constant color and transparency specified in the visualization context unless IdTran attribute objects are set which contain a color map or transparency map index for each element. All the faces in an element are drawn with this constant color or transparency. If a
VIS_IDTRAN_DATAINDEX
IdTran attribute object has been set then a DataIndex drawing function is called with the value contained in the IdTran object prior to drawing the faces for an element.The underlying model information is accessed using the GridFun attribute object. Set the GridFun and IdTran objects using
vis_FaceSetObject()
. The visualization contexts Shrink and ShrinkType can be used to control shrink.- Errors
Parameters:
6.7. Shaded Solid - Cell
Use Cell to render shaded solids. Solid geometry is input as individual tetrahedra, pyramids, wedges or hexahedra or regular meshes of the same. The functions associated with a Cell object are the following.
- Begin and end an instance of an object, return object error flag
vis_CellBegin()
- create an instance of a Cell objectvis_CellEnd()
- destroy an instance of a Cell objectvis_CellError()
- return Cell object error flag
- Operations
vis_CellComputeDist()
- compute equivalent nodal loadsvis_CellConvertDist()
- compute distributed tractionsvis_CellCurv()
- draw curvilinear cellvis_CellCurvColor()
- draw curvilinear, color interpolated cellvis_CellInterpolate()
- interpolate field datavis_CellIntersectPoint()
- compute closet point to input pointvis_CellRST()
- compute natural coordinates of center and nodesvis_CellSetElemNode()
- set input polyhedral cell connectivityvis_CellSetFaceFlag()
- set cell faces to be drawnvis_CellSetObject()
- set pointers to attribute objects.vis_CellSetParami()
- set parameters for cell visualizationvis_CellSetTopology()
- set input cell topologyvis_CellTangRST()
- compute material tangents at a point.vis_CellTrav()
- traverse and draw a group of elements
Instance an Cell object using vis_CellBegin()
.
Set attribute objects using vis_CellSetObject()
.
The current cell topology is specified using vis_CellSetTopology()
.
Draw constant color cells using vis_CellCurv()
and color interpolated cells using vis_CellCurvColor()
.
Use vis_CellSetFaceFlag()
to specify which faces of the cell to draw. The convenience routine,
vis_CellTrav()
, will traverse and draw a group of elements.
The Cell
module supports a number of functions to support useful computations
performed upon cell geometry and data. The vis_CellIntersectPoint()
function is designed to help applications interactively pick and probe
cell geometry and data. It returns, among other information, the world
coordinate and natural coordinate points of intersection. Use
vis_CellInterpolate()
to compute field data at nodes to a specified cell natural coordinate
location. This function is designed to be used in conjunction with the
cell intersection function. If the current cell topology is polyhedral,
ie VIS_SHAPEPOLYHED, the interpolation and intersection computations
require that the polyhedral element connectivity be entered using
vis_CellSetElemNode()
.
The Cell module duplicates many features of the Face module. It is intended primarily for performing point intersection and interpolation on solid elements.
Use the functions vis_CellComputeDist()
and vis_CellConvertDist()
to compute equivalent nodal loads from distibuted loads and vice versa.
The elements are assumed to be isoparametric. The function
vis_CellConvertDist()
is particularly useful for visualizing the distributed reactions from
the nodal reactions for higher order elements.
6.8. Attribute Objects
A Cell object uses DrawFun, DataInt,
IsoClip, VisContext, ColorMap
and TransMap
objects to define attributes to generate a cell face visualization
entity. As an option, the exterior edges of the cell faces may be drawn.
A DrawFun object is required for cell drawing using
vis_CellCurv()
and vis_CellCurvColor()
.
The DataInt, IsoClip, VisContext
and ColorMap and TransMap objects are optional. Use a
DataInt object to activate data interpolation. An
IsoClip
object is required if isosurface clipping is to be performed. A
GridFun
object is required if model traversal is performed using
vis_CellTrav()
.
If model traversal is performed using vis_CellTrav()
, then a
GridFun object is required and IdTran
objects are optional for specifying entity color and transparency. A
Cell object uses the following VisContext
components.
Color | Cell face color |
DistTol | Distance tolerance used in intersection testing |
Draw | Flag to call graphics attribute and graphics primitive drawing functions. Use this flag to disable these drawing functions if only “Data” drawing functions are desired. |
Edge | Edge flag, if on the exterior edges of the cell face are drawn |
Fill | Fill flag, if off the surface of the cell face is not filled |
Flags | OR the following flags: VIS_CREATEPOLYHEDRON generates polyhedral graphics primitives rather than the default polygon primitives. |
LineWidth | Line width of edges |
LineStyle | Line style of edges |
MinorColor | Cell edge color |
Refinement | Level of refinement |
Shade | Flag to apply light source shading |
Shrink | Shrink factor used during model traversal only |
ShrinkType | Shrink type used during model traversal only |
Size | Unit line width of cylindrical line style |
Trans | Cell transparency |
If the VisContext
attribute object pointer is NULL, then cell faces are generated without
reference to VisContext, ColorMap
and TransMap
objects. No attribute drawing functions are invoked, all cell faces are
drawn using flat shaded polygon graphics primitives with no refinement
assuming the current transparency,
vis_CellCurv()
assumes the current color,
vis_CellCurvColor()
uses the input colors at cell nodes.
6.9. Function Descriptions
The currently available Cell functions are described in detail in this section.
-
vis_Cell *
vis_CellBegin
(void) create an instance of a Cell object
Create an instance of a Cell object. Memory is allocated for the object private data and the pointer to the data is returned. By default all attribute object pointers are NULL and no isosurface clipping is active. The cell topology is set to a 8 noded hexahedral element.
Destroy an instance of a Cell object using
void vis_CellEnd (vis_Cell *cell)
Return the current value of a Cell object error flag using
Vint vis_CellError (vis_Cell *cell)
Returns: The function returns a pointer to the newly created Cell object. If the object creation fails, NULL is returned.
-
void
vis_CellEnd
(vis_Cell *p) destroy an instance of a Cell object
See
vis_CellBegin()
-
Vint
vis_CellError
(vis_Cell *p) return the current value of a Cell object error flag
See
vis_CellBegin()
-
void
vis_CellSetParami
(vis_Cell *p, Vint ptype, Vint iparam) set parameters for cell visualization
Specify parameters for controlling cell visualization. The parameter
CELL_USERELEMNODE
is used to enable the use of the user specified polyhedral cell connectivity usingvis_CellSetElemNode()
in those functions in which the specific connectivity is optional. In general the connectivity is optional in those functions which do not pass the polyhedral node coordinates in the argument list. By default,CELL_USERELEMNODE
is off.- Errors
VIS_ERROR_ENUM
is generated if an improper ptype is specified.
Parameters: - p – Pointer to Cell object.
- ptype – Parameter type to set.
x=CELL_USERELEMNODE Enable using polyhedral connectivity
- iparam – Specifies the integer value that pype will be set to.
-
void
vis_CellSetObject
(vis_Cell *p, Vint objecttype, Vobject *object) set pointers to attribute objects
Set a pointer to an attribute object. If an IsoClip attribute object is set then isosurface clipping is activated. To deactivate isosurface clipping, set a NULL IsoClip object pointer. If a DataInt object is set then data interpolation is activated. To deactivate data interpolation, set a NULL DataInt object pointer. The GridFun and IdTran objects are only used by
vis_CellTrav()
.- Errors
VIS_ERROR_OBJECTTYPE
is generated if an improper objecttype is specified.
Parameters: - p – Pointer to Cell object.
- objecttype – The name of the object type to be set.
x=VIS_COLORMAP ColorMap object =VIS_DATAINT DataInt object =VGL_DRAWFUN DrawFun object =VIS_GRIDFUN GridFun object =VIS_IDTRAN_COLOR IdTran color map index object =VIS_IDTRAN_DATAINDEX IdTran data index object =VIS_IDTRAN_TRANS IdTran transparency map index object =VIS_ISOCLIP IsoClip object =VIS_TRANSMAP TransMap object =VIS_VISCONTEXT VisContext object
- object – Pointer to the object to be set.
-
void
vis_CellSetTopology
(vis_Cell *p, Vint shape, Vint maxi, Vint maxj, Vint maxk) set cell topology
All subsequent draw and computation methods assume cells of this topology. See section Computational Cells for a description of element topology conventions.
- Errors
VIS_ERROR_VALUE
is generated if a maxi, maxj or maxk less than 0 or equal to 1 is input.VIS_ERROR_ENUM
is generated if an improper shape is input.
Parameters: - p – Pointer to Cell object.
- shape – Cell shape parameter
x=VIS_SHAPETET Tetrahedron =VIS_SHAPEPYR Pyramid =VIS_SHAPEWED Wedge =VIS_SHAPEHEX Hexahedron =VIS_SHAPEPOLYHED Polyhedron
- maxi – Topology parameter
- maxj – Topology parameter
- maxk – Topology parameter
-
void
vis_CellSetElemNode
(vis_Cell *p, Vint ix[]) set input polyhedral cell connectivity
Set polyhedral element connectivity. This information is currently only required for element shape
VIS_SHAPEPOLYHED
when used with thevis_CellIntersectPoint()
andvis_CellInterpolate()
functions. The use of polyhedral connectivity in functions where it is optional is toggled usingvis_CellSetParami()
.Parameters: - p – Pointer to Cell object.
- ix – Element connectivity
-
void
vis_CellSetFaceFlag
(vis_Cell *p, Vint flag) set cell faces to be drawn
Set face flag. The flag argument contains bit flags indicating which faces are to be drawn. The default face flag is 0x3f.
Parameters: - p – Pointer to Cell object.
- flag – Element face bit flags to indicate faces to be drawn.
-
void
vis_CellCurv
(vis_Cell *p, Vfloat x[][3]) draw curvilinear cell
Draw a cell with point locations x. The topology of the cell is defined using
vis_CellSetTopology()
. The use ofvis_CellCurv()
draws a cell with the constant color specified in the visualization context. The functionvis_CellCurvColor()
draws a cell with color interpolation within the cell faces between the RGB color values, c, specified at the point locations.Note that in the interest of efficiency,
vis_CellCurv()
does not issue a Color or Trans drawing function each time it is called to ensure that the cell to be drawn is rendered in the color and transparency in the current VisContext attribute object. Instead, Color and Trans drawing functions are issued only if the VisContext attribute object has been changed in any way since a previous call tovis_CellCurv()
. Therefore it is recommended to callvis_VisContextTouch()
with the VisContext attribute object before drawing a series of cells withvis_CellCurv()
.- Errors
VIS_ERROR_NULLOBJECT
is generated if no DrawFun attribute object has been set.
Parameters: - p – Pointer to Cell object.
- x – Array of point locations defining cell.
-
void
vis_CellCurvColor
(vis_Cell *p, Vfloat x[][3], Vfloat c[][3]) draw curvilinear cell
See
vis_CellCurv()
.Parameters: - p – Pointer to Cell object.
- x – Array of point locations defining cell.
- c – Array of RGB color triples at point locations.
-
void
vis_CellIntersectPoint
(vis_Cell *p, Vfloat x[][3], Vfloat xp[3], Vfloat r[3], Vfloat xr[3], Vfloat xd[3], Vint *status) compute point of intersection
Compute the closest point within the cell defined by node point locations, x, to a point defined by xp. The natural coordinates, r, and the coordinates, xr of the closest point within the cell to the input point are returned. The distance vector, xd, from xr to the input point is returned. For element shape
VIS_SHAPEPOLYHED
, the element connectivity must have been entered usingvis_CellSetElemNode()
.The case that the magnitude of xd is zero implies that the intersecting point lies within the cell.
The status variable is returned as unity if the length of vector xd is less than the DistTol element of the visualization context and zero otherwise. Use
is_CellInterpolate()
to interpolate field data at nodes to the intersection point represented by natural coordinates r.Parameters: - p – Pointer to Cell object.
- x – Array of point locations on cell.
- xp – Intersecting point
- r – [out] Natural coordinates of closest point within cell.
- xr – [out] Coordinates at natural coordinates r.
- xd – [out] Vector from xr to point or closest point within cell
- status – [out] Intersection status
-
void
vis_CellInterpolate
(vis_Cell *p, Vfloat r[3], Vint nrws, Vfloat d[], Vfloat dr[]) interpolate field data
Interpolate nrws of field data, d given at each node point of the cell to the natural coordinates specified by r. A vector of nrws of interpolated values are returned in dr. The natural coordinates r span the interval [-1.,1] for hexahedral shapes, [0.,1] for tetrahedral shapes and [0.,1] or [-1.,1] for wedge shapes. A natural coordinate outside of these intervals will extrapolate the input field data to a point outside the geometric extent of the cell. For element shape
VIS_SHAPEPOLYHED
, the element connectivity must have been entered usingvis_CellSetElemNode()
.Parameters: - p – Pointer to Cell object.
- r – Natural coordinates to interpolate field data to
- nrws – Row dimension of the field data array d.
- d – Array of field data at node point locations.
- dr – [out] Vector of interpolated field data at natural coordinate r.
-
void
vis_CellRST
(vis_Cell *p, Vfloat rc[3], Vint *nix, Vfloat r[][3]) compute natural coordinates of center and nodes
Compute natural coordinates of center and nodes.
Parameters: - p – Pointer to Cell object.
- rc – [out] Natural coordinates of center
- nix – [out] Number of nodes
- r – [out] Natural coordinates at nodes.
-
void
vis_CellTangRST
(vis_Cell *p, Vfloat x[][3], Vfloat r[3], Vfloat g[3][3]) compute material tangents at a point.
Compute derivatives, g, of the natural coordinate system with respect to the coordinates at a natural coordinate point, r. The vectors returned in g are tangent to the respective natural coordinate directions.
Parameters: - p – Pointer to Cell object.
- x – Array of point locations on cell.
- r – Natural r,s,t coordinates of point
- g – [out] Material tangents.
-
void
vis_CellTangRSTdv
(vis_Cell *p, Vdouble x[][3], Vdouble r[3], Vdouble g[3][3]) compute material tangents at a point.
-
void
vis_CellComputeDist
(vis_Cell *p, Vfloat x[][3], Vint nrws, Vfloat v[], Vfloat f[]) compute equivalent nodal loads
Compute equivalent nodal loads from distributed loads in a cell.
Parameters: - p – Pointer to Cell object.
- x – Array of point locations along polyline defining cell.
- nrws – Number of distributed components per point
- v – Array of distributed loads.
- f – [out] Array of equivalent nodal loads.
-
void
vis_CellComputeDistdv
(vis_Cell *p, Vdouble x[][3], Vint nrws, Vdouble v[], Vdouble f[]) compute equivalent nodal loads
Compute equivalent nodal loads from distributed loads in a cell.
Parameters: - p – Pointer to Cell object.
- x – Array of point locations along polyline defining cell.
- nrws – Number of distributed components per point
- v – Array of distributed loads.
- f – [out] Array of equivalent nodal loads.
-
void
vis_CellConvertDist
(vis_Cell *p, Vfloat x[][3], Vint nrws, Vfloat f[], Vfloat v[]) compute distributed tractions
Convert equivalent nodal loads to distributed loads in a cell. This function is the exact inverse of
vis_CellComputeDist()
.Parameters: - p – Pointer to Cell object.
- x – Array of point locations along polyline defining cell.
- nrws – Number of distributed components per point
- f – Array of equivalent nodal loads.
- v – [out] Array of distributed loads.
-
void
vis_CellConvertDistdv
(vis_Cell *p, Vdouble x[][3], Vint nrws, Vdouble f[], Vdouble v[]) compute distributed tractions
Convert equivalent nodal loads to distributed loads in a cell. This function is the exact inverse of
vis_CellComputeDist()
.Parameters: - p – Pointer to Cell object.
- x – Array of point locations along polyline defining cell.
- nrws – Number of distributed components per point
- f – Array of equivalent nodal loads.
- v – [out] Array of distributed loads.
-
void
vis_CellTrav
(vis_Cell *p, vis_Group *group) traverse and draw a group of elements
Traverse and draw a group of elements specified in Group. Only elements which have a shape accepted by
vis_CellSetTopology()
are processed. If an element face group is input then only the faces of the elements specified in the element face group are drawn. Otherwise the element faces specified usingvis_CellSetFaceFlag()
are drawn.The element faces are drawn with the constant color and transparency specified in the visualization context unless IdTran attribute objects are set which contain a color map or transparency map index for each element. All the faces in an element are drawn with this constant color or transparency. If a
VIS_IDTRAN_DATAINDEX
IdTran attribute object has been set then a DataIndex drawing function is called with the value contained in the IdTran object prior to drawing the faces for an element.The underlying model information is accessed using the GridFun attribute object. Set the GridFun and IdTran objects using
vis_CellSetObject()
. The visualization contexts Shrink and ShrinkType can be used to control shrink.Parameters: