Getting B-Rep Data
The following entities contain the geometric data that represents the B-rep data:
- Topology B-rep data: a topological boundary representation comprised of a bounding box and references to multiple Connex entities.
- Connex: a collection of shell entities, such as a hollow sphere that contains another sphere that is represented by two connexes
- Shell: a collection of face entities
- Face: a surface and a collection of loops
It is important to note that B-rep is only available if HOOPS Exchange was able to read it from the original model source file.
Starting from the first (and only) root representation item in our example file
, we can see that it is a B-rep model because it is of type kA3DTypeRiBrepModel (if the model file did not include B-rep, the representation item length field on the parent node would be zero).
Since representation item sets may include other representation item sets, you should parse them recursively. For example, you could do something similar to this:
// Traverse Representation Items recursively
A3DStatus traverseRI(const A3DRiRepresentationItem* pRI)
{
A3DStatus iRet = A3D_SUCCESS;
A3DRiRepresentationItemData sRIData;
// 'riType' is an integer value you can test against to find the type
A3DEEntityType riType;
CHECK_RET(A3DEntityGetType(pRI, &riType));
if (riType == kA3DTypeRiBrepModel)
{
traverseBREPModel(pRI);
}
else if (riType == kA3DTypeRiSet)
{
A3DRiSetData sRiSetData;
A3D_INITIALIZE_DATA(A3DRiSetData, sRiSetData);
CHECK_RET(A3DRiSetGet(pRI, &sRiSetData));
for (int i = 0; i < sRiSetData.m_uiRepItemsSize; ++i)
{
traverseRI(sRiSetData.m_ppRepItems[i]);
}
}
return iRet;
}
B-Rep Hierarchy
The general process used for obtaining all the B-rep information is the same as for tessellation. However, be aware that B-rep has a much deeper assembly hierarchy than tessellation. The B-rep assembly hierarchy is called the topology .
HOOPS Exchange B-rep topology structure:
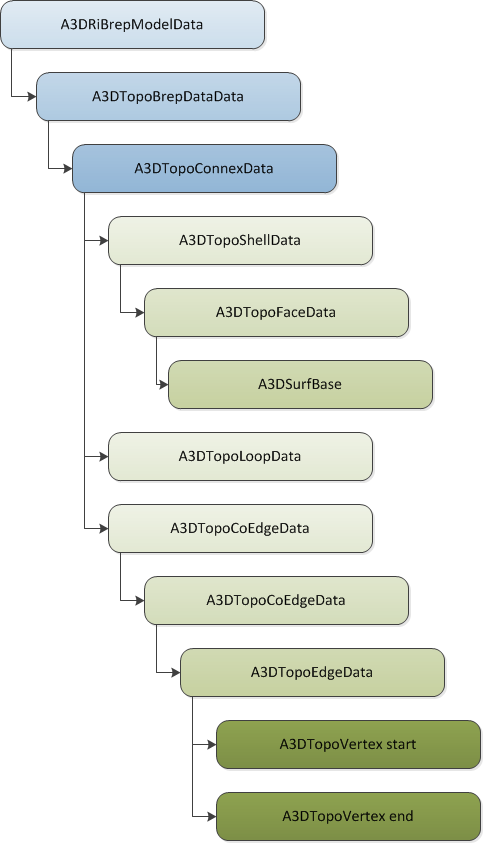