Reading Model Geometry
When loading a file using direct integration, it is necessary to traverse over the model’s assembly tree in order to read all model geometry and attributes. Recall that once loaded into memory, the model is stored as a PRC assembly tree. When reading data, you will always traverse the tree from root to leaf. Conversely, if you ever have a need to create a PRC structure, it is recommended that you proceed from leaf to root.
Geometric data, whether it is in the form of B-rep or tessellation, is always stored in a representation item . See PRC for information on how representation items are related to the other entity types.
As you are traversing an assembly tree, how can the various geometries be identified?
All PRC entities have an associated base data structure which aggregates information common to all entities.
The structure is of type ref A3DRootBaseData and contains fields such as attributes, entity name, and ID.
If the entity also has graphical information associated with it, then the entity will also have a A3DRootBaseWithGraphicsData
.
This structure encapsulates information that affects the appearance of the entity, such as color, line pattern, and display style.
For this example, we will use a model file that contains a simple cube.
The sample PRC file can be found here
.
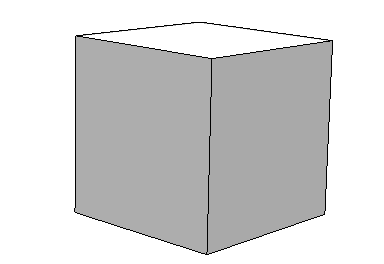
Since the geometric data is always stored in a representation item node, we must traverse the assembly tree until we find that node.
Traversing the Model
Step 1: Accessing the Assembly Tree
If you’re doing a direct integration, you will need to parse the PRC structure after loading the model.
The first thing to do is to initialize HOOPS Exchange and get a reference to the model file data structure.
Using that structure (of type A3DAsmModelFileData
), you can get access to the assembly tree.
The model file data structure - ref A3DAsmModelFileData - always has a reference to at least one product occurrence. In complex models, the model file may contain references to a list of product occurrences - this is called an assembly . However, for our example cube, the model file contains just one root product occurrence.
Our goal is to reach the representation item node.
Representation items are always associated with an individual part in the model, so we’ll need to get that reference first.
In HOOPS Exchange, these part definitions are represented by the A3DAsmPartDefinition
class.
int parseProductOccurrence(A3DAsmProductOccurrence** poList, int poListLength)
{
for (int i = 0; i < poListLength; i++)
{
// get reference to product occurrence node
A3DAsmProductOccurrence* po = poList[i];
// now get the node's data
A3DAsmProductOccurrenceData poData;
A3D_INITIALIZE_DATA(A3DAsmProductOccurrenceData, poData);
A3DAsmProductOccurrenceGet(po, &poData);
if (poData.m_pPart != NULL)
{
// use the product occurrence data to get the part definition data
A3DAsmPartDefinitionData pdData;
A3D_INITIALIZE_DATA(A3DAsmPartDefinitionData, pdData);
A3DAsmPartDefinitionGet(poData.m_pPart, &pdData);
}
// ....
Step 2: Finding the Part You’re Looking for
At this point, you have a part definition structure pdData. In this simple example, we know there is only one part definition. However, in practice there are usually many definitions. In order to find the part definition you’re interested in, you need to find the part’s name or ID. This is done by examining the root base entity data .
A3DRootBaseData rootBaseData;
A3D_INITIALIZE_DATA(A3DRootBaseData, rootBaseData);
if (A3DRootBaseGet(poData.m_pPart, &rootBaseData) != A3D_SUCCESS)
// handle error
// get the name of the part
rootBaseData.m_pcName;
// get part ID
if (rootBaseData.m_uiPersistentId == myPartID)
// this is the part we're interested in
Step 3: Inspect the Representation Item
When you find the specific part you want from step 2, you are ready to examine the representation item attached to it. Note that if the part is particularly complex, there could be multiple representation items present to describe the part. As mentioned previously, the representation item encapsulates tessellation and B-rep data. HOOPS Exchange is able to generate tessellation data from B-rep. Therefore, once a model file is loaded into memory as PRC, tessellation is always available, even if the original file did not include it. For information on how to get tessellation, as well as how tessellation delivery is controlled, please see Reading Tessellation.