Publications
From a high-level point of view, a publication, composed of a name, is a reference to an entity. This referenced entity can be geometry, topology, a feature, an attribute, or even an annotation. When several publications are defined, we call a publication set, which is the collection of all such publications.
HOOPS Exchange is able to retrieve the following referenced entities:
Bodies, planes, and sketches;
Geometrical lines and points;
Faces, edges, and vertices.
When the referenced entity is not found, only the publication name is defined, and the reference is left empty. Finally, in an assembly, not all instances from the same model are referenced when one of them is referenced; i.e., it is possible to target a specific instance of a model.
API Overview
Through the HOOPS Exchange API, a publication set is defined on the product occurrence which created it, as shown in the following structure:
1typedef struct
2{
3 // ...
4 A3DAsmPublicationSet* m_pPublicationSet;
5} A3DAsmProductOccurrenceData;
If the publication set is null, then no publications are stored on the given product occurrence. Let’s see how to traverse a publication set.
Publication set
A publication set
is structured as follows:
1typedef struct
2{
3 A3DUns16 m_usStructSize;
4 A3DUns32 m_uiPublicationsSize;
5 A3DAsmPublication** m_ppPublications;
6} A3DAsmPublicationSetData;
The field m_ppPublications
is an array of publications of size m_uiPublicationsSize
.
To get the information from this structure, one needs to call A3DAsmPublicationSetGet()
as shown in the following code:
1A3DStatus traverse_publication_set(const A3DAsmPublicationSet* publication_set)
2{
3 if (!publication_set)
4 {
5 return A3D_SUCCESS;
6 }
7
8 A3DAsmPublicationSetData publication_set_data;
9 A3D_INITIALIZE_DATA(A3DAsmPublicationSetData, publication_set_data);
10
11 if (A3DAsmPublicationSetGet(publication_set, &publication_set_data) == A3D_SUCCESS)
12 {
13 for (unsigned int i = 0; i < publication_set_data.m_uiPublicationsSize; i++)
14 {
15 traverse_publication(publication_set_data.m_ppPublications[i]);
16 }
17
18 A3DAsmPublicationSetGet(0, &publication_set_data);
19
20 return A3D_SUCCESS;
21 }
22
23 return A3D_ERROR;
24}
In the code above, the loop allows us to traverse all publications stored within a publication set. Let’s see how to traverse a publication.
Publication
A publication
is structured as follows:
1 typedef struct
2 {
3 A3DUns16 m_usStructSize;
4 A3DUns32 m_uiLinkedItemsSize;
5 A3DAsmPublicationLinkedItem** m_ppLinkedItems;
6 } A3DAsmPublicationData;
The field m_ppLinkedItems
is an array of references to entities of size m_uiLinkedItemsSize
.
Regarding the publication name, it is not stored directly in this structure but in the root base of the publication.
To get the information from this structure, one needs to call A3DAsmPublicationGet()
as shown in the following code:
1A3DStatus traverse_publication(const A3DAsmPublication* publication)
2{
3 if (!publication)
4 {
5 return A3D_SUCCESS;
6 }
7
8 // The name of the publication is stored in the root base.
9 A3DRootBaseData root_base_data;
10 A3D_INITIALIZE_DATA(A3DRootBaseData, root_base_data);
11 A3DRootBaseGet(publication, &root_base_data);
12
13 A3DAsmPublicationData publication_data;
14 A3D_INITIALIZE_DATA(A3DAsmPublicationData, publication_data);
15
16 if (A3DAsmPublicationGet(publication, &publication_data) == A3D_SUCCESS)
17 {
18 for (unsigned int i = 0; i < publication_data.m_uiLinkedItemsSize; i++)
19 {
20 traverse_publication_linked_item(publication_data.m_ppLinkedItems[i]);
21 }
22
23 A3DAsmPublicationGet(0, &publication_data);
24
25 return A3D_SUCCESS;
26 }
27
28 A3DRootBaseGet(0, &root_base_data);
29
30 return A3D_ERROR;
31}
In the code above, the publication name is retrieved from the root base, and the publication linked item is traversed. Let’s see how to traverse a publication linked item.
Publication linked item
A publication linked item
is structured as follows:
1 typedef struct
2 {
3 A3DUns16 m_usStructSize;
4 A3DAsmProductOccurrence* m_pTargetProductOccurrence;
5 A3DEntity* m_pReference;
6 } A3DAsmPublicationLinkedItemData;
The field m_pTargetProductOccurrence
corresponds to the product occurrence
to which the referenced entity belongs; this is very useful in an assembly context. If the model is a part,
this field is null as the targeted product occurrence would be the same as the one on which the publication is defined.
The field m_pReference
is the referenced entity:
If the referenced entity is not a topological item, then this field directly references an object whose type is defined in “Referenceable Non-Topological Entities.”
Otherwise, if the referenced entity is a topological item, then this field is a
Reference On Topology Item
.
To get the type of the entity referenced by this field, one can call the A3DAsmPublicationLinkedItemGet()
and A3DEntityGetType()
functions as shown in the following code:
1 A3DStatus traverse_publication_linked_item(const A3DAsmPublicationLinkedItem* publication_linked_item)
2 {
3 if (!publication_linked_item)
4 {
5 return A3D_SUCCESS;
6 }
7
8 A3DAsmPublicationLinkedItemData publication_linked_item_data;
9 A3D_INITIALIZE_DATA(A3DAsmPublicationLinkedItemData, publication_linked_item_data);
10
11 if (A3DAsmPublicationLinkedItemGet(publication_linked_item, &publication_linked_item_data))
12 {
13 if (publication_linked_item_data.m_pTargetProductOccurrence)
14 {
15 // Do something with the target product occurrence.
16 }
17
18 A3DEEntityType type = kA3DTypeUnknown;
19 A3DEntityGetType(publication_linked_item_data.m_pReference, &type);
20
21 if (type == kA3DTypeMiscReferenceOnTopology)
22 {
23 // The referenced entity is a topological item.
24
25 return A3D_SUCCESS;
26 }
27
28 // The referenced entity is not a topological item.
29
30 A3DAsmPublicationLinkedItemGet(0, &publication_linked_item_data);
31
32 return A3D_SUCCESS;
33 }
34
35 return A3D_ERROR;
36 }
In the code above, the targeted product occurrence can be traversed if it is not null. Then, the type of the referenced entity is retrieved to see if it is a Reference On Topology Item.
Examples
Let’s see some publications with CATIA V5.
Publication of a body in a part
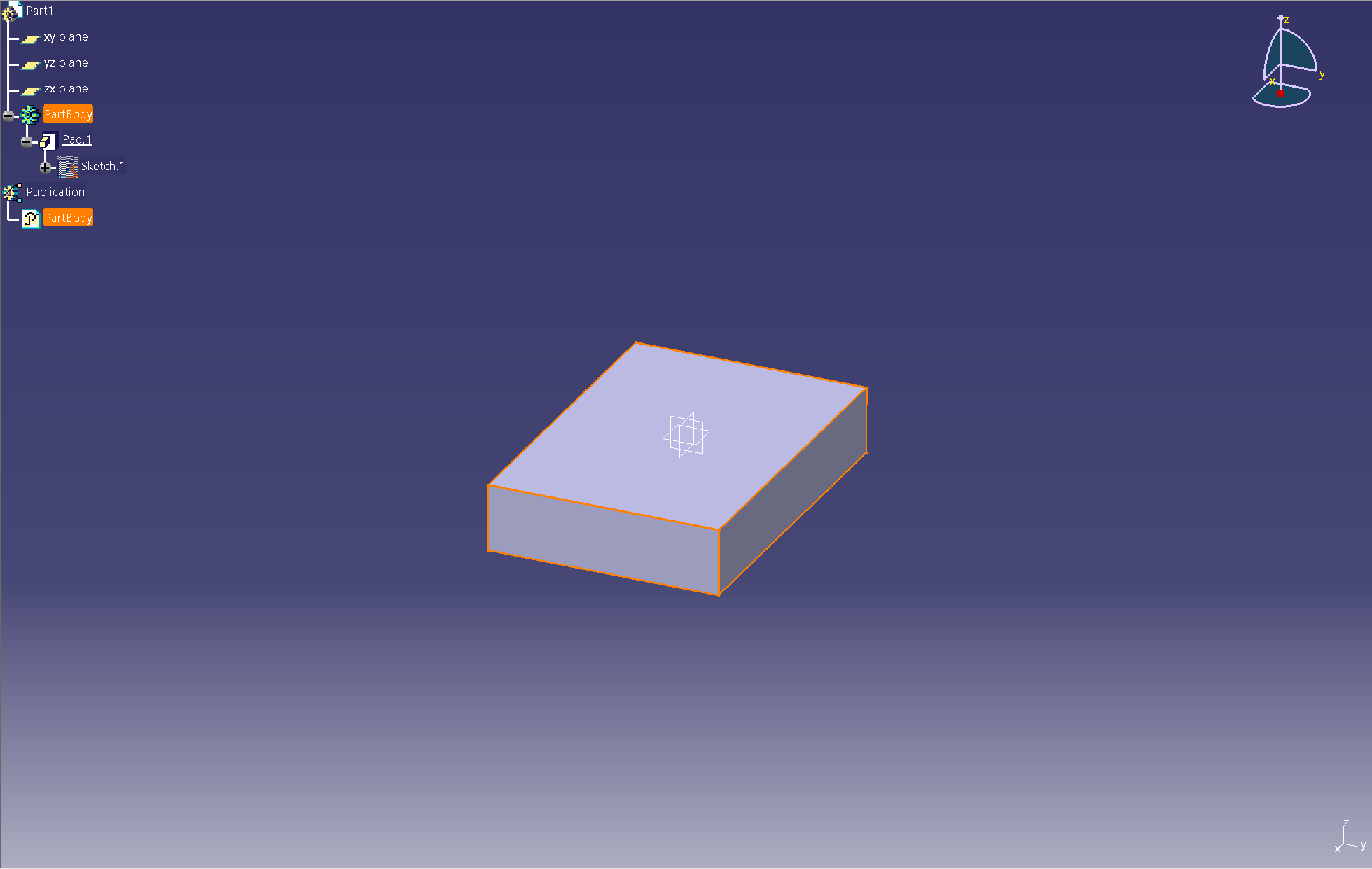
In the screenshot above, the publication “PartBody” references a body in “Part1”.
As the publication is defined in a part, the publication set is defined in the product occurrence of the part.
As the model is not an assembly, the targeted product occurrence is null, i.e., the referenced entity belongs to the product occurrence in which the publication has been created.
As the referenced entity is not a topological item then the reference points directly on the body of the model.
Publication of a face in a part
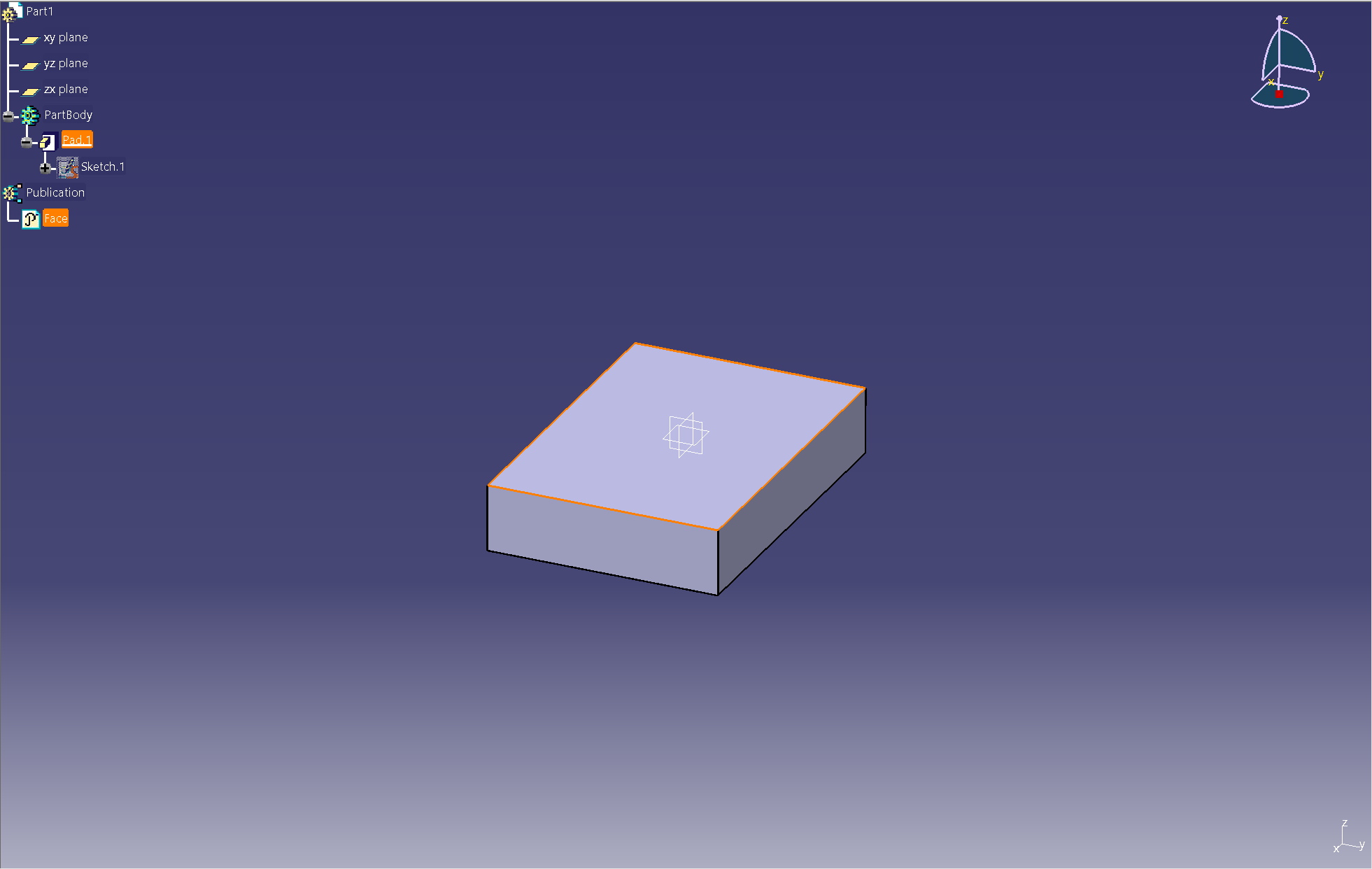
In the screenshot above, the publication “Face” references a face from “PartBody” in “Part1”.
As the publication is defined in a part, the publication set is defined in the product occurrence of the part.
As the model is not an assembly, the targeted product occurrence is null, i.e., the referenced entity belongs to the product occurrence in which the publication has been created.
As the referenced entity is a topological item, the reference is a Reference On Topology Item.
Publication of a body in an assembly
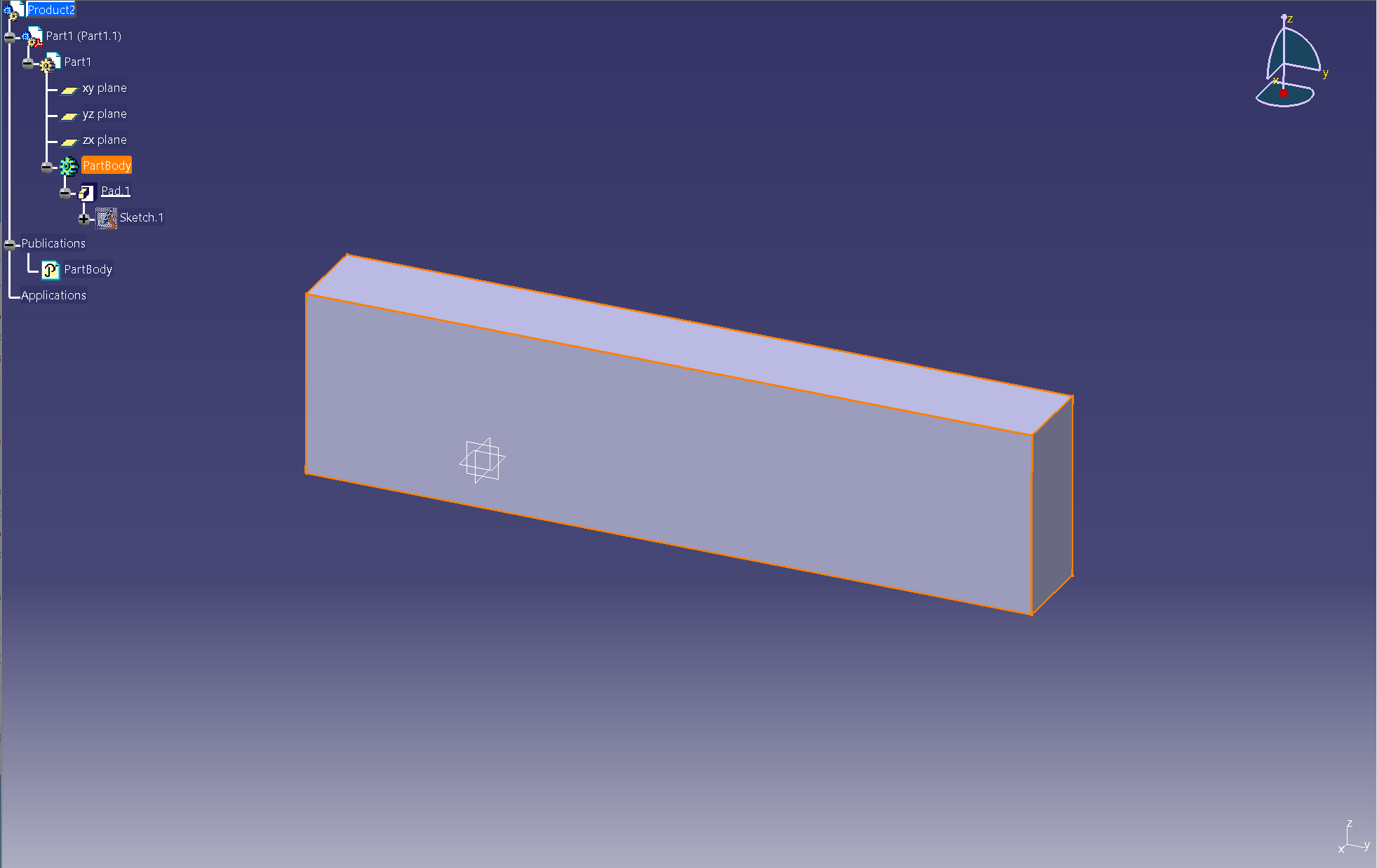
In the screenshot above, the publication “PartBody” references a body from “Part1” in “Product2”.
As the publication is defined in an assembly, the publication set is defined in the product occurrence of the assembly node.
As the model is an assembly, the targeted product occurrence is not null and references the product occurrence of the model to which the body belongs.
As the referenced entity is not a topological item then the reference points directly on the body of the model.