UnstructGrid: Create an isosurface
An isosurface is a surface defined of an area with a constant value within a volume of space. Several visual settings can be applied to the isosurface, such as mapped fringes, visibility and highlighting.
This example shows how to create an isosurface based on a scalar result id and a scalar value. In addition, the scalar result will be shown as fringes on the isosurface.
The demo file (contact.vtfx) contains a simple model with various results. In this tutorial you will ‘ create an isosurface for the “All displacements” result with iso scalar value == 6.5 for time step 4.
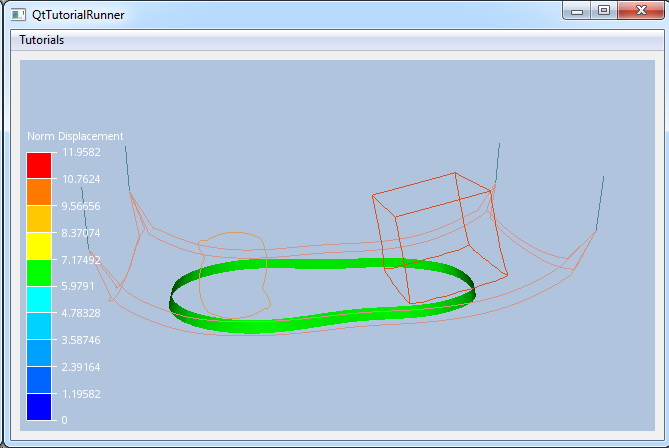
void TutorialRunnerMainWindow::addIsosurface()
{
//--------------------------------------------------------------------------
// Load an UnstructGridModel from file
// Create an isosurface
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
// Open the VTFx file
cee::Str vtfxFile = TutorialUtils::testDataDir() + "contact.vtfx";
if (!source->open(vtfxFile, 0))
{
// VTFx file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the fourth state as current.
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[3].id();
ugModel->modelSpec().setStateId(stateId);
// Create a new isosurface object and set the scalar result id and iso scalar value used to calculate the isosurface topography.
cee::PtrRef<cee::ug::Isosurface> isoSurface = new cee::ug::Isosurface();
// User "displacements" scalar result
std::vector<cee::ug::ResultInfo> resultInfos = source->directory()->scalarResultInfos();
int scalarId = resultInfos[0].id();
isoSurface->setIsoScalarResultId(scalarId);
isoSurface->setIsoValue(6.5);
// Set the same result mapped on the surface also
isoSurface->setMapScalarResultId(scalarId);
// Add the isosurface to the model
ugModel->addIsosurface(isoSurface.get());
// To be able to see the isosurface inside the part, set draw styles to LINES for all parts
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setDrawStyle(cee::ug::PartSettings::OUTLINE);
}
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}