Cross Sections (All types)
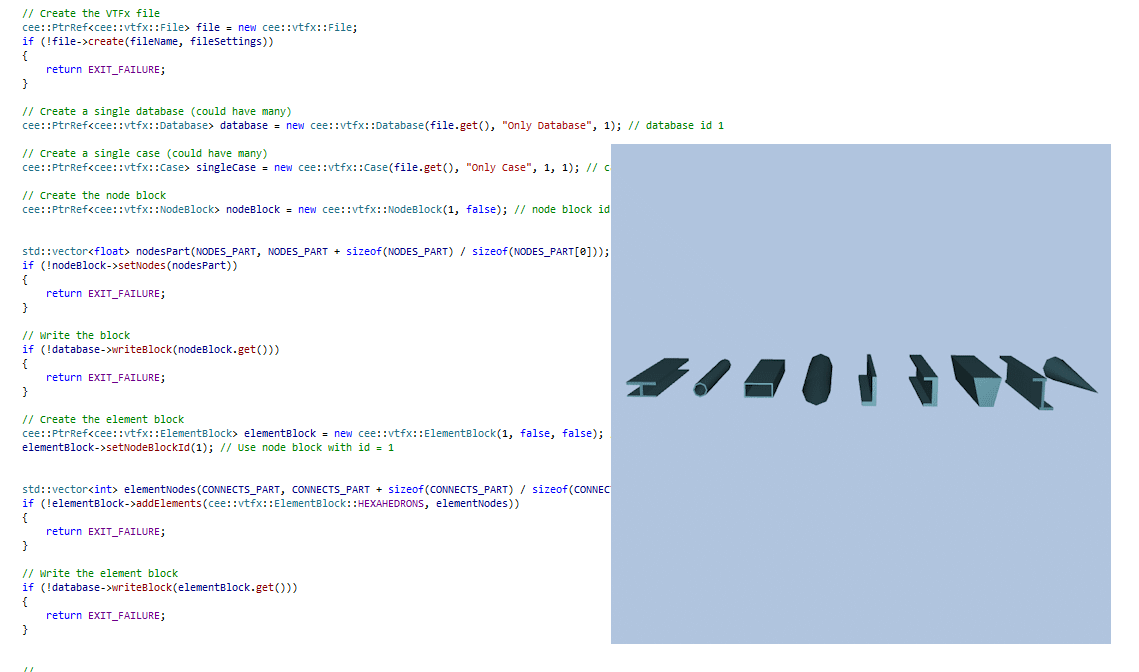
Small example showcasing all cross sections expansions provided in Envision.
This example contains just 9 beams, one for each cross section type:
- BEAM_IORH
- BEAM_PIPE
- BEAM_BOX
- BEAM_CYLINDER
- BEAM_L
- BEAM_CHANNEL
- BEAM_BAR
- BEAM_UNSYMMETRIC_I
- BEAM_CONE
This is a good example to get an overview over all parameter names for all cross section types and play with different parameter values.
//--------------------------------------------------------------------------
// Create the VTFx file
// -------------------------------------------------------------------------
cee::PtrRef<cee::vtfx::File> file = new cee::vtfx::File;
// Create VTFx file settings struct
cee::vtfx::FileSettings fileSettings;
fileSettings.applicationName = "VTFx: VTFxCrossSectionsAll";
fileSettings.vendorName = "My Company AS";
// Let the API create the VTFx file
const cee::Str fileName = "ExampleCrossSectionsAll.vtfx";
if (!file->create(fileName, fileSettings))
{
return EXIT_FAILURE;
}
// Create a single database (could have many)
cee::PtrRef<cee::vtfx::Database> database = new cee::vtfx::Database(file.get(), "Only Database", 1, cee::vtfx::Database::SIMULATION_TYPE_STRUCTURAL, cee::vtfx::Database::SOLUTION_TYPE_TRANSIENT, "Database description"); // database id 1
// Create a single case (could have many)
cee::PtrRef<cee::vtfx::Case> singleCase = new cee::vtfx::Case(file.get(), "Only Case", 1, 1); // case id 1 using database with id 1
//--------------------------------------------------------------------------
// Add nodes
// -------------------------------------------------------------------------
// Create the node block
cee::PtrRef<cee::vtfx::NodeBlock> nodeBlock = new cee::vtfx::NodeBlock(1, false); // node block id 1, not using node ids
// Set the node data (Note: The VTFx component expects interleaved node coordinates (xyzxyzxyz...))
std::vector<float> nodesPart = { 0.0f, 0.0f, 0.0f, 0.0f, 0.0f, 10.0f,
4.0f, 0.0f, 0.0f, 4.0f, 0.0f, 10.0f,
8.0f, 0.0f, 0.0f, 8.0f, 0.0f, 10.0f,
12.0f, 0.0f, 0.0f, 12.0f, 0.0f, 10.0f,
16.0f, 0.0f, 0.0f, 16.0f, 0.0f, 10.0f,
20.0f, 0.0f, 0.0f, 20.0f, 0.0f, 10.0f,
24.0f, 0.0f, 0.0f, 24.0f, 0.0f, 10.0f,
28.0f, 0.0f, 0.0f, 28.0f, 0.0f, 10.0f,
32.0f, 0.0f, 0.0f, 32.0f, 0.0f, 10.0f,
36.0f, 0.0f, 0.0f, 36.0f, 0.0f, 10.0f };
if (!nodeBlock->setNodes(nodesPart))
{
return EXIT_FAILURE;
}
// Write the block
if (!database->writeBlock(nodeBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add elements
// -------------------------------------------------------------------------
// Create the element block
cee::PtrRef<cee::vtfx::ElementBlock> elementBlock = new cee::vtfx::ElementBlock(1, false, false); // element block id 1, not using element ids, referring nodes by index
elementBlock->setNodeBlockId(1); // Use node block with id = 1
elementBlock->setCrossSectionsBlockId(11); // Use cross sections block with id = 1
// Set the element data
// 9 BEAMS
std::vector<int> elementNodes = { 0, 1,
2, 3,
4, 5,
6, 7,
8, 9,
10, 11,
12, 13,
14, 15,
16, 17 };
std::vector<int> crossSectionsMapping = { 0, 1, 2, 3, 4, 5, 6, 7, 8 }; // One mapping per element
std::vector<cee::Vec3f> directions = { cee::Vec3f(0.0f, 1.0f, 0.0f), cee::Vec3f(0.0f, 1.0f, 0.0f),
cee::Vec3f(0.0f, 1.0f, 0.0f), cee::Vec3f(0.0f, 1.0f, 0.0f),
cee::Vec3f(0.0f, 1.0f, 0.0f), cee::Vec3f(0.0f, 1.0f, 0.0f),
cee::Vec3f(0.0f, 1.0f, 0.0f), cee::Vec3f(0.0f, 1.0f, 0.0f),
cee::Vec3f(0.0f, 1.0f, 0.0f), cee::Vec3f(0.0f, 1.0f, 0.0f),
cee::Vec3f(0.0f, 1.0f, 0.0f) }; // One direction per element
if (!elementBlock->addElementsWithCrossSections(cee::vtfx::ElementBlock::BEAMS, elementNodes, crossSectionsMapping, directions))
{
return EXIT_FAILURE;
}
// Write the element block
if (!database->writeBlock(elementBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add cross sections
// -------------------------------------------------------------------------
// Create cross sections block
cee::PtrRef<cee::vtfx::CrossSectionsBlock> csBlock = new cee::vtfx::CrossSectionsBlock(11);
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_IORH,
{ "OffsetY", "OffsetZ", "Height", "WidthTop", "ThicknessTop", "ThicknessWeb", "WidthBottom", "ThicknessBottom" },
{ 0.0f, 0.0f, 1.0f, 2.0f, 0.2f, 0.2f, 2.0f, 0.1f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_PIPE,
{ "OffsetY", "OffsetZ", "OuterDiameter", "Thickness" },
{ 0.0f, 0.0f, 1.0f, 0.1f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_BOX,
{ "OffsetY", "OffsetZ", "Height", "Width", "ThicknessTop", "ThicknessWebs", "ThicknessBottom" },
{ 0.0f, 0.0f, 1.0f, 2.0f, 0.1f, 0.1f, 0.1f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_CYLINDER,
{ "OffsetY", "OffsetZ", "OuterDiameter" },
{ 0.0f, 0.0f, 2.0f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_L,
{ "OffsetY", "OffsetZ", "Height", "Width", "ThicknessWeb", "ThicknessFlange", "Orientation" },
{ 0.3f, 0.0, 2.0f, 1.0f, 0.3f, 0.3f, 1.0f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_CHANNEL,
{ "OffsetY", "OffsetZ", "Height", "Width", "ThicknessWeb", "ThicknessFlange", "Orientation" },
{ 0.0f, 0.0f, 2.0f, 1.0f, 0.4f, 0.2f, 1.0f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_BAR,
{ "OffsetY", "OffsetZ", "Height", "WidthTop", "WidthBottom" },
{ 0.0f, 0.0f, 2.0f, 2.0f, 1.0f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_UNSYMMETRIC_I,
{ "OffsetY", "OffsetZ", "Height", "ThicknessWeb", "WidthTop", "WidthTopPos", "ThicknessTop", "WidthBottom", "WidthBottomPos", "ThicknessBottom" },
{ 0.0f, 0.0f, 2.0f, 0.2f, 1.0f, 0.2f, 0.2f, 1.0f, 0.8f, 0.2f });
csBlock->addCrossSection(cee::vtfx::CrossSectionsBlock::BEAM_CONE,
{ "OffsetY", "OffsetZ", "OuterDiameter1", "OuterDiameter2" },
{ 0.0f, 0.0f, 2.0f, 0.3f });
// Write the geometry info block
if (!database->writeBlock(csBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add geometry
// -------------------------------------------------------------------------
// Create geometry block
cee::PtrRef<cee::vtfx::GeometryBlock> geoBlock = new cee::vtfx::GeometryBlock(1); // Only one geometry per state
size_t geoIndex = 0;
int partId = 1;
// Set to use element block with id 1
if (!geoBlock->addElementBlock(geoIndex, 1, partId)) // Element block with id = 1
{
return EXIT_FAILURE;
}
// Write the geometry block
if (!database->writeBlock(geoBlock.get()))
{
return EXIT_FAILURE;
}
// Create geometry info block
cee::PtrRef<cee::vtfx::GeometryInfoBlock> infoBlock = new cee::vtfx::GeometryInfoBlock(1); // Only one geometry per state
infoBlock->addPartInfo(geoIndex, partId, cee::Str("Only part"));
// Write the geometry info block
if (!database->writeBlock(infoBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add one state
// -------------------------------------------------------------------------
// Create state info block
cee::PtrRef<cee::vtfx::StateInfoBlock> stepInfo = new cee::vtfx::StateInfoBlock;
if (!stepInfo->addStateInfo(1, "Only step", 42.0f, cee::vtfx::StateInfoBlock::TIME))
{
return EXIT_FAILURE;
}
// Write the state info block
if (!database->writeBlock(stepInfo.get()))
{
return EXIT_FAILURE;
}
// Finally close the file
if (!file->close())
{
return EXIT_FAILURE;
}
std::cout << "Exported successfully to file: " << fileName.toStdString() << std::endl;
std::cout << std::endl << "Press enter to exit..." << std::endl;
std::cin.ignore();
return EXIT_SUCCESS;