Polyhedron
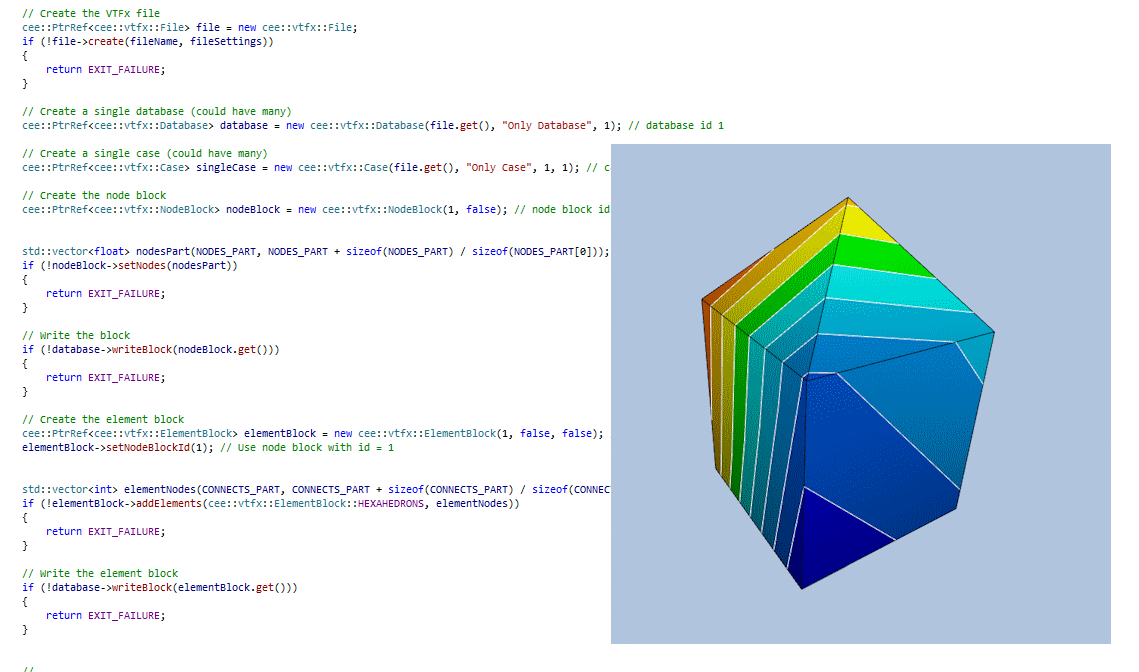
Small example on creating a polyhedron.
Creates two simple polyhedron elements with a scalar result
//--------------------------------------------------------------------------
// Create the VTFx file
// -------------------------------------------------------------------------
cee::PtrRef<cee::vtfx::File> file = new cee::vtfx::File;
// Create VTFx file settings struct
cee::vtfx::FileSettings fileSettings;
fileSettings.applicationName = "VTFx: VTFxPolyhedron";
fileSettings.vendorName = "My Company AS";
// Let the API create the VTFx file
const cee::Str fileName = "ExamplePolyhedron.vtfx";
if (!file->create(fileName, fileSettings))
{
return EXIT_FAILURE;
}
// Create a single database (could have many)
cee::PtrRef<cee::vtfx::Database> database = new cee::vtfx::Database(file.get(), "Only Database", 1, cee::vtfx::Database::SIMULATION_TYPE_STRUCTURAL, cee::vtfx::Database::SOLUTION_TYPE_TRANSIENT, "Database description"); // database id 1
// Create a single case (could have many)
cee::PtrRef<cee::vtfx::Case> singleCase = new cee::vtfx::Case(file.get(), "Only Case", 1, 1); // case id 1 using database with id 1
//--------------------------------------------------------------------------
// Add nodes
// -------------------------------------------------------------------------
// Create the node block
cee::PtrRef<cee::vtfx::NodeBlock> nodeBlock = new cee::vtfx::NodeBlock(1, false); // node block id 1, not using node ids
// Set the node data (Note: The VTFx component expects interleaved node coordinates (xyzxyzxyz...))
std::vector<float> nodesPart =
{
2.0f, 0.0f, 0.0f,
3.0f, 0.0f, 0.0f,
2.0f, 1.0f, 0.0f,
3.0f, 1.0f, 0.0f,
2.5f, 1.5f, 0.5f,
2.0f, 0.0f, 1.0f,
3.0f, 0.0f, 1.0f,
2.0f, 1.0f, 1.0f,
3.0f, 1.0f, 1.0f
};
if (!nodeBlock->setNodes(nodesPart))
{
return EXIT_FAILURE;
}
// Write the block
if (!database->writeBlock(nodeBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add elements
// -------------------------------------------------------------------------
// Create the element block
cee::PtrRef<cee::vtfx::ElementBlock> elementBlock = new cee::vtfx::ElementBlock(1, false, false); // element block id 1, not using element ids, referring nodes by index
elementBlock->setNodeBlockId(1); // Use node block with id = 1
std::vector<int> elementNodes =
{
// Element 0
0, 1, 2, 3, 5, 6, 7, 8,
// Element 1
2, 3, 4, 7, 8
};
std::vector<int> numNodesPerPolyhedron = { 8, 5 };
std::vector<int> numFacesPerPolyhedron = { 6, 5 };
std::vector<int> numNodesPerFace =
{
// Element 0
4, 4, 4, 4, 4, 4,
// Element 1
4, 3, 3, 3, 3
};
std::vector<int> globalFaceNodeIndices =
{
// Element 0
0, 1, 6, 5,
8, 7, 5, 6,
3, 8, 6, 1,
2, 3, 1, 0,
2, 0, 5, 7,
2, 7, 8, 3,
// Element 1
2, 3, 8, 7,
4, 7, 8,
4, 8, 3,
4, 3, 2,
4, 2, 7
};
if (!elementBlock->addPolyhedronElements(elementNodes, numNodesPerPolyhedron, numFacesPerPolyhedron, numNodesPerFace, globalFaceNodeIndices))
{
return EXIT_FAILURE;
}
// Write the element block
if (!database->writeBlock(elementBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add geometry
// -------------------------------------------------------------------------
// Create geometry block
cee::PtrRef<cee::vtfx::GeometryBlock> geoBlock = new cee::vtfx::GeometryBlock(1); // Only one geometry per state
size_t geoIndex = 0;
int partId = 1;
// Set to use element block with id 1
if (!geoBlock->addElementBlock(geoIndex, 1, partId)) // Element block with id = 1
{
return EXIT_FAILURE;
}
// Write the geometry block
if (!database->writeBlock(geoBlock.get()))
{
return EXIT_FAILURE;
}
// Create geometry info block
cee::PtrRef<cee::vtfx::GeometryInfoBlock> infoBlock = new cee::vtfx::GeometryInfoBlock(1); // Only one geometry per state
infoBlock->addPartInfo(geoIndex, partId, cee::Str("Only part"));
// Write the geometry info block
if (!database->writeBlock(infoBlock.get()))
{
return EXIT_FAILURE;
}
// -------------------------------------------------------------------------
// Add scalar result
// -------------------------------------------------------------------------
// Use node coordinates as scalar result values
const std::vector<float>& scalarResults = nodesPart;
// Create the result values block
cee::PtrRef<cee::vtfx::ResultValuesBlock> resultValuesBlock = new cee::vtfx::ResultValuesBlock(1, cee::vtfx::ResultValuesBlock::VECTOR3D, false);
resultValuesBlock->setMapToBlockId(1, cee::vtfx::Block::NODES);
if (!resultValuesBlock->allocateResultValues(static_cast<int>(scalarResults.size()) / 3))
{
return EXIT_FAILURE;
}
for (size_t i = 0; i < scalarResults.size() / 3; i++)
{
resultValuesBlock->addResultValue3d(scalarResults[3 * i], scalarResults[3 * i + 1], scalarResults[3 * i + 2]);
}
// Write the block
// -------------------------------------------------------------------------
if (!database->writeBlock(resultValuesBlock.get()))
{
return EXIT_FAILURE;
}
// Write the scalar result definition
// -------------------------------------------------------------------------
cee::PtrRef<cee::vtfx::ResultBlock> scalarResult = new cee::vtfx::ResultBlock(1, cee::vtfx::ResultBlock::SCALAR, cee::vtfx::ResultBlock::NODE_MAPPING);
if (!scalarResult->addResultValuesBlock(1, 1))// Assign the result values blocks to state IDs.
{
return EXIT_FAILURE;
}
scalarResult->setResultId(1);
scalarResult->setName("My scalar result");
scalarResult->setUnit("m^2");
if (!database->writeBlock(scalarResult.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add one state
// -------------------------------------------------------------------------
// Create state info block
cee::PtrRef<cee::vtfx::StateInfoBlock> stepInfo = new cee::vtfx::StateInfoBlock;
if (!stepInfo->addStateInfo(1, "Only step", 42.0f, cee::vtfx::StateInfoBlock::TIME))
{
return EXIT_FAILURE;
}
// Write the state info block
if (!database->writeBlock(stepInfo.get()))
{
return EXIT_FAILURE;
}
// -------------------------------------------------------------------------
// Write properties
// -------------------------------------------------------------------------
cee::PtrRef<cee::PropertySetCollection> vtfxProps = new cee::PropertySetCollection;
// Show result with id = 1 as fringes and contour lines
cee::PtrRef<cee::PropertySet> scalarSelection = new cee::PropertySet("result_selection");
scalarSelection->setValue("fringes_result_id", 1);
scalarSelection->setValue("contour_lines_result_id", 1);
vtfxProps->addPropertySet(scalarSelection.get());
// Configure camera
cee::PtrRef<cee::PropertySet> camera = new cee::PropertySet("camera");
camera->setValue("eye", cee::Vec3d(4.5, 0.75, 2.5));
camera->setValue("vrp", cee::Vec3d(4, 0.75, 2));
camera->setValue("vup", cee::Vec3d(0, 1, 0));
vtfxProps->addPropertySet(camera.get());
// Background color
cee::PtrRef<cee::PropertySet> backgroundColor = new cee::PropertySet("background");
backgroundColor->setValue("single_background_color", cee::Color3f(0.0, 0.0, 0.0));
vtfxProps->addPropertySet(backgroundColor.get());
if (!singleCase->setProperties(vtfxProps.get()))
{
return EXIT_FAILURE;
}
// -------------------------------------------------------------------------
// Close file
// -------------------------------------------------------------------------
if (!file->close())
{
return EXIT_FAILURE;
}
std::cout << "Exported successfully to file: " << fileName.toStdString() << std::endl;
std::cout << std::endl << "Press enter to exit..." << std::endl;
std::cin.ignore();
return EXIT_SUCCESS;