Markup Basics
Markup manager overview
The viewer.markupManager
class facilitates interactions with markup in the viewer. This includes serialization for export and import, as well as adding and deleting markup items. Markup views, notes, measurements, and lines get serialized by default, but it is also possible to include a custom markup type that will have its data included along with the default markup JSON object.
To serialize the markup for export, we will create a JSON object with all the data from the markup in the scene using the exportMarkup() function.
const markupData = JSON.stringify(viewer.markupManager.exportMarkup());
To load the markup data again, we will use the loadMarkupData function.
viewer.markupManager.loadMarkupData(markupData);
Markup types
Redline
Redline markup is 2D markup that is drawn in an SVG layer on top of the canvas. It depends on the camera to display correctly, and it will be hidden if the camera changes. There are four types of redline items:
Circle
Rectangle
Polyline
Text
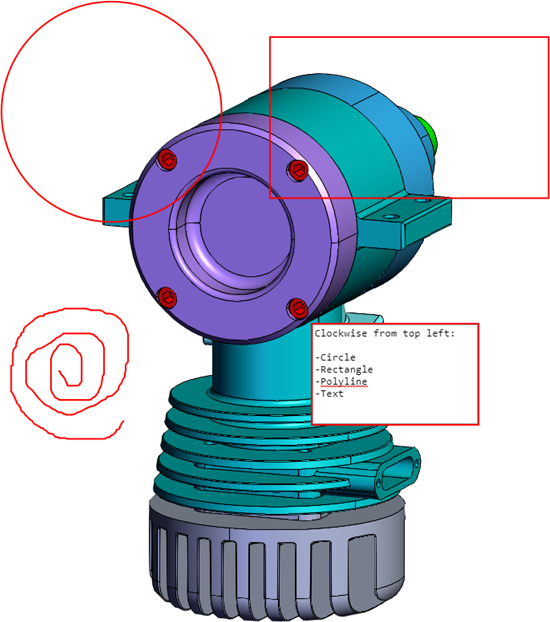
Redline markup type
Note pins
Note pins are attached to a position on the model. 3D geometry is drawn in the scene to represent the ‘pin’ on the model (a line drawn at the normal to the part, and a sphere drawn at the end of the line for the head of the pin). The geometry size is not camera dependent, and it will not scale with the model. A 2D HTML div
is displayed on top of the canvas; this div
moves when the camera changes to stay fixed to the pin’s position. This div
allows changing the color of the pin, deleting the pin, or editing the text associated with the pin.
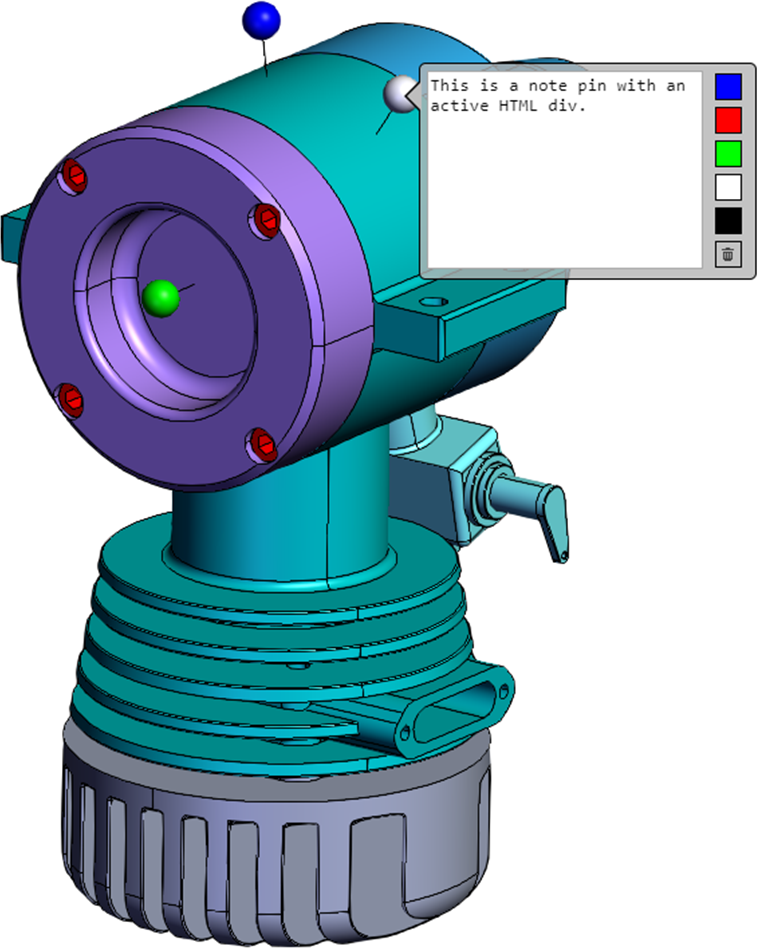
Note pins markup type
Measurement
Measurement markup is 3D markup, drawn in an SVG layer on top of the canvas. When the camera changes, the markup is updated so that it scales and rotates with the camera.
Face-angle markup measures the angle between two faces.
Face-distance markup measures the distance between two faces.
Length markup measures the length of an edge.
Point-distance markup measures the distance between two points.
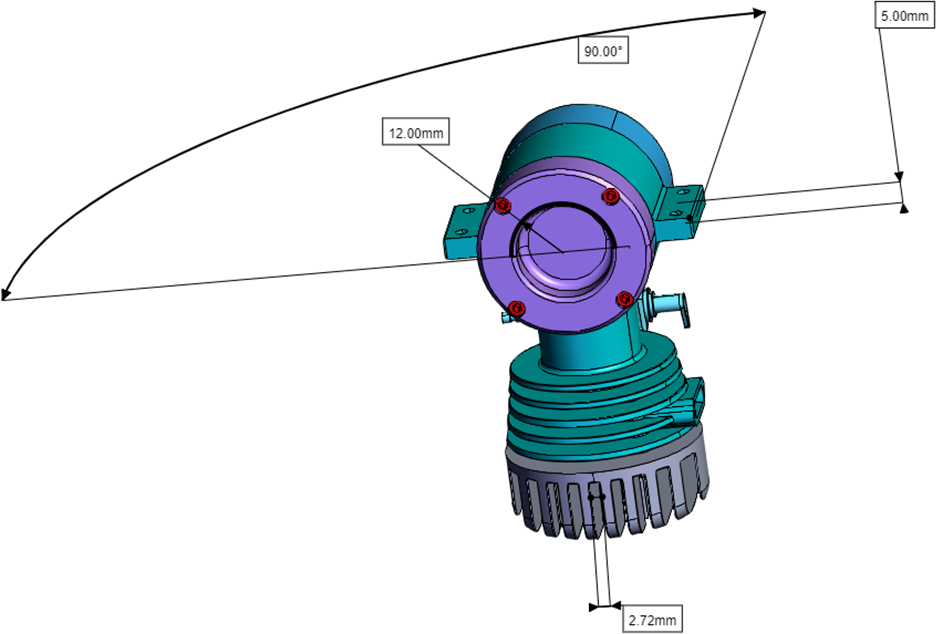
Measurement markup type
Lines
Line markup is 3D geometry that is drawn in the scene. It contains a start point and end point which can each be associated with a node ID.
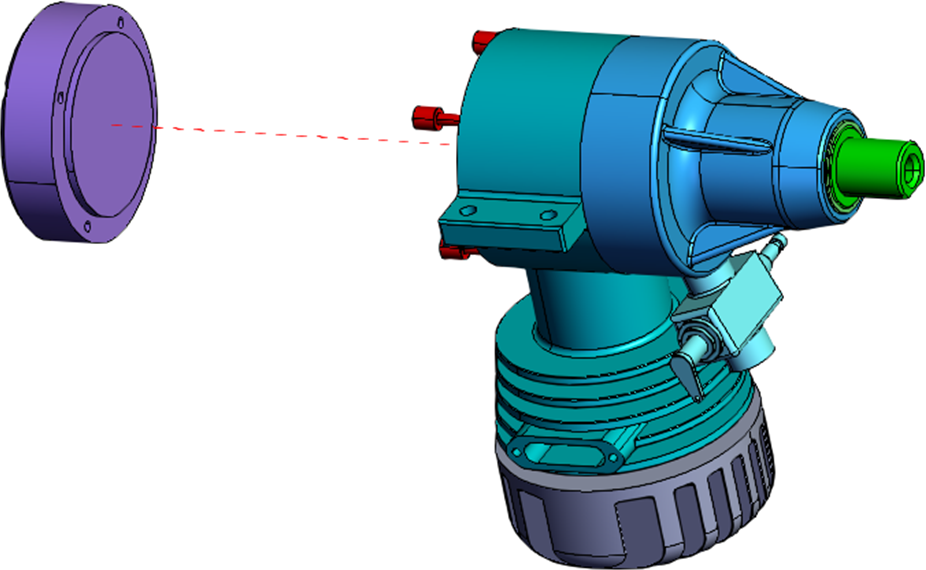
Line markup type
Redline operators
The redline operators create redline markup in the scene. If a redline item is created and there is no active markup view, an active view is created. If a redline item is created when there is already an active view, the redline item is added to the view.
Each redline markup item has a corresponding operator that handles creation of the item and adding it to the view.
Circle operator
Rectangle operator
Polyline operator
Text operator
Markup views
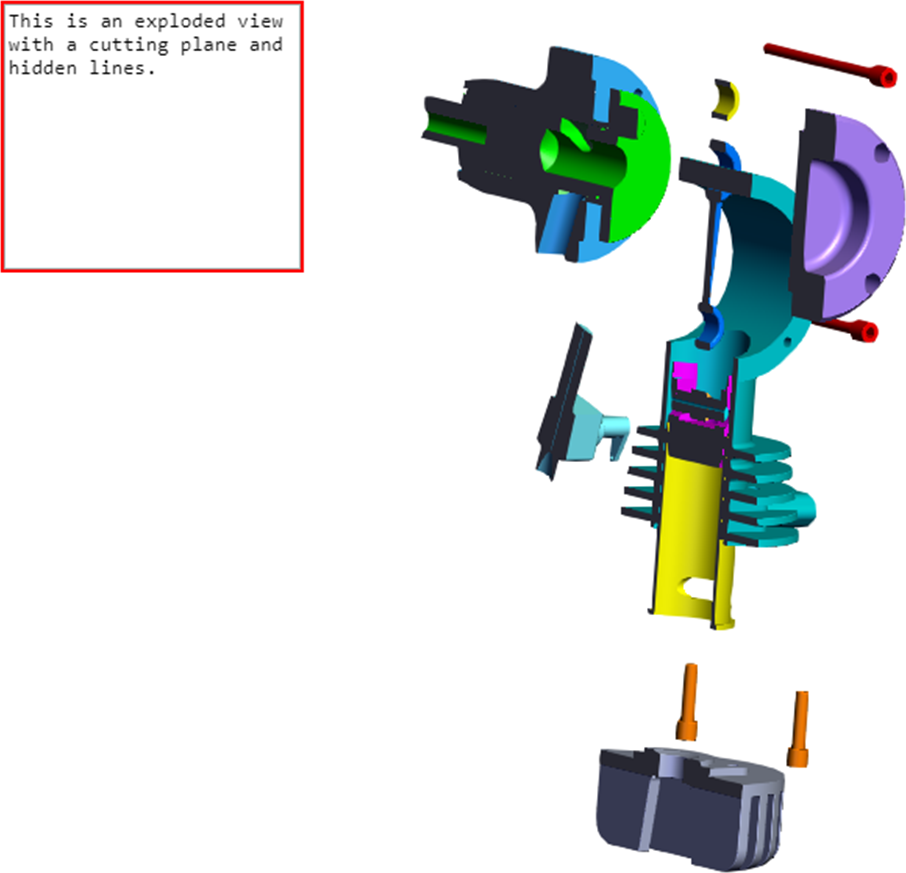
Markup View recreating an exploded state
A markup view contains data that can recreate a state in the viewer. The data may contain the following:
Camera
Sheet ID (If the model is a drawing with sheets)
Exploding magnitude
Line visibility
Face visibility
Markup items
Node ID color map
Snapshot image
The markupManager
can be used to create a new view which stores the model state.
const uniqueId = viewer.markupManager.createMarkupView("Markup View");
At the time the view is created, the model state is saved. Additional data can be added or changed after the markup view is created. The camera and sheet id cannot be changed once a view is created.
When a view is activated, the stored model state is recreated in the scene.
viewer.markupManager.activateMarkupViewWithPromise(uniqueId);