Effects
HOOPS Communicator includes a variety of effects that can alter the appearance of your scene.
Ambient occlusion
Ambient occlusion is a lighting effect that is included in HOOPS Communicator. Ambient occlusion uses the scene’s ambient light to determine the brightness and color of points in the scene. Embedded areas of geometry are shaded by this effect.
In HOOPS Communicator, ambient occlusion is disabled by default. To enable or disable ambient occlusion, call the setAmbientOcclusionEnabled() function with a Boolean:
// Enable Ambient Occlusion
hwv.view.setAmbientOcclusionEnabled(true);
// Disable Ambient Occlusion
hwv.view.setAmbientOcclusionEnabled(false);
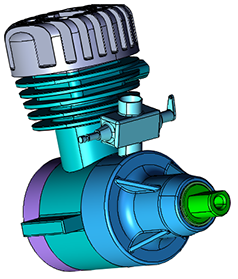
Ambient occlusion off
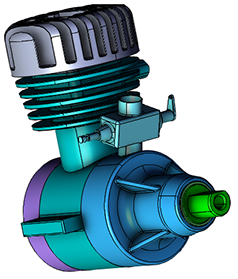
Ambient occlusion on
You can check the current ambient occlusion setting using the getAmbientOcclusionEnabled() function which will return a boolean indicating if ambient occlusion is enabled or disabled.
hwv.view.getAmbientOcclusionEnabled();
Ambient occlusion radius
Ambient occlusion radius is the maximum screen-proportional distance between two points such that one will cast a shadow on the other. Ambient occlusion radius can be updated by passing a number to the setAmbientOcclusionRadius() function.
hwv.view.setAmbientOcclusionRadius(0.15);
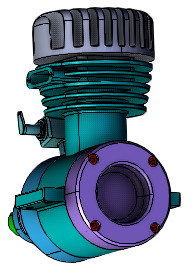
Ambient occlusion radius = 0.065
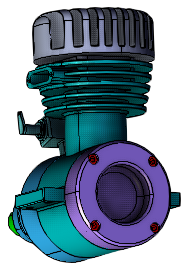
Ambient occlusion radius = 0.15
The current ambient occlusion value can also be retrieved with getAmbientOcclusionRadius():
hwv.view.getAmbientOcclusionRadius();
Ambient light
Ambient light is a nondirectional light which illuminates the scene. To change the ambient light color create a new instance of a color object and pass it to the setAmbientLightColor() function.
The constructor for the color
object takes three parameters: red, green and blue. Each parameter in the constructor is a number from 0 to 255 which describes the intensity (with 0 being no intensity and 255 being max intensity) of red, green or blue in the newly created color.
var ambColor = new Communicator.Color(0, 60, 50);
hwv.view.setAmbientLightColor(ambColor);
The following images have ambient occlusion enabled.
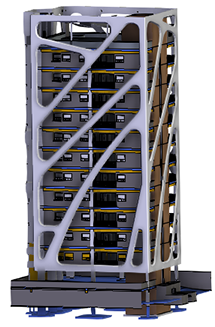
Default ambient light color
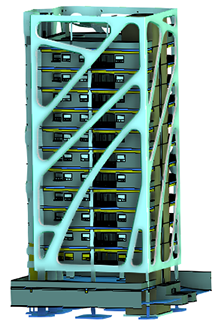
Ambient light color (80, 0, 80)
To retrieve the current ambient light color, call the getAmbientLightColor() function. The default ambient light color in the scene is a white light with values red = 19.125, blue = 19.125 and green = 19.125.
hwv.view.getAmbientLightColor();
Bloom
Bloom is an effect which simulates a bright light entering a camera. Bright areas in the scene, that exceed a specified bloom luminance threshold, will glow casting their color on the surrounding surfaces. The bloom effect is achieved by applying a luminance filter to the source image, then progressively downsampling, blurring, and adding the results together. The result of each downsample/blur operation is fed into the next, which is executed at half the resolution of the previous. The number of stages and the behavior of each stage are controlled by the setBloomLayers() function.
In the images below, a directional light is hitting the models causing areas of their surfaces to exceed the bloom luminance threshold.
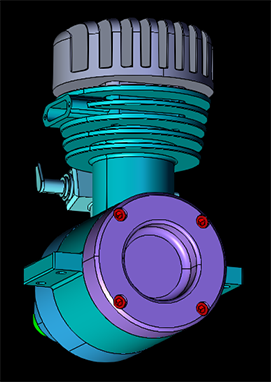
Bloom disabled
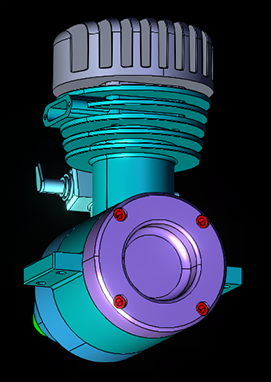
Bloom enabled
To enable and disable bloom pass a boolean to the setBloomEnabled() function:
// Enable Bloom
hwv.view.setBloomEnabled(true);
// Disable Bloom
hwv.view.setBloomEnabled(false);
The viewer’s current bloom state can be obtained using the getBloomEnabled() function.
hwv.view.getBloomEnabled();
Bloom threshold
Bloom threshold is the minimum luminance value a pixel must have for it to contribute to bloom. The viewer’s bloom threshold is set by passing a number in the range [0,1] (with values closer to 0 resulting in more bloom and values closer to 1 resulting in less bloom) to setBloomThreshold(). The viewer’s current view threshold can be acquired using getBloomThreshold(). The default bloom threshold value is 0.65.
// Get the current Bloom Threshold
hwv.view.getBloomThreshold();
// Update Bloom Threshold
hwv.view.setBloomThreshold(0.5);
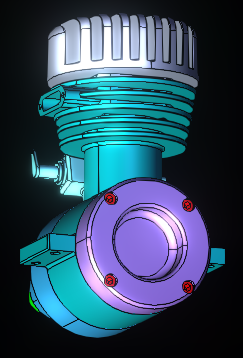
Bloom threshold 0.5
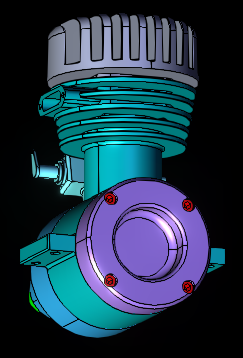
Bloom threshold 0.65 (default)
Bloom threshold ramp width
Determines how much greater the pixel luminance value must be when compared to the threshold set by the setBloomThreshold() method before it contributes fully to the bloom effect. If the pixel’s luminance value does not exceed the threshold by at least the amount set by this function, the pixel’s contribution will be diminished based on how close its luminance value is to the threshold.
Set the viewer’s bloom threshold ramp width using setBloomThresholdRampWidth(). The viewer’s existing bloom threshold ramp width value can be obtained with getBloomThresholdRampWidth(). The default value of the bloom threshold ramp width is 0.1.
// Get the current Bloom Threshold Ramp Width value
hwv.view.getBloomThresholdRampWidth();
// Set the Bloom Threshold Ramp Width value
hwv.view.setBloomThresholdRampWidth(0.2);
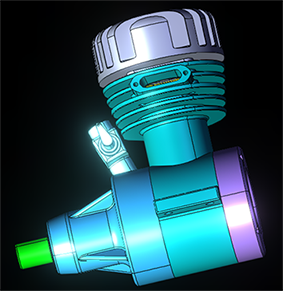
Bloom threshold ramp width 0.1 (default)
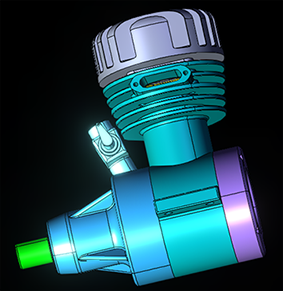
Bloom threshold ramp width 0.2
Bloom intensity scale
The intensity of a bloom layer can be controlled by passing a number to setBloomIntensityScale. The viewer’s current bloom intensity can be retrieved with getBloomIntensityScale(). The default bloom intensity scale is 1.
// Retrieve the current bloom intensity scale
hwv.view.getBloomIntensityScale();
// Update the bloom intensity scale
hwv.view.setBloomIntensityScale(0.25);
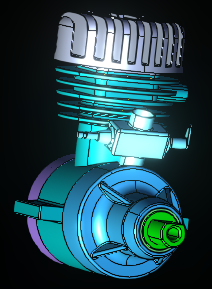
Bloom intensity scale 1
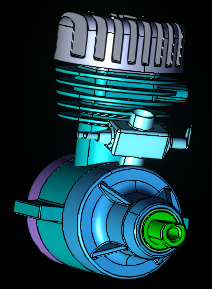
Bloom intensity scale 0.25
Multi-layer bloom
The bloom effect is achieved by applying a luminance filter to the source image, then progressively downsampling, blurring, and adding the results together. The result of each downsample/blur operation is fed into the next, which is executed at half the resolution of the previous. The number of stages and the behavior of each stage are controlled by the setBloomLayers() function.
BloomLayerInfo
Communicator.BloomLayerInfo describes a single layer in the bloom effect. Each bloom layer contains blurInterval
, blurSamples
and intensity
properties.
- blurInterval is the distance between samples taken during the Gaussian blur operation executed when rendering this layer.
blurInterval
is defined as[number, BlurIntervalUnit]
where number is distance between samples the andBlurIntervalUnit
describes the unit used to measure intervals between samples. If unspecified, the value will default to[1, Pixels]
. Information regardingBlurIntervalUnit.Pixels
and othe the otherBlurInteralUnits
can be found at this page. - blurSamples are the number of samples taken in each of the two passes of the Gaussian blur operation executed when rendering this layer. If unspecified, the value will default to 9.
- Intensity is the number used to scale the contribution of the layer to the image. If unspecified, the value will default to 1. Set the intensity using setBloomIntensityScale().
Adding and removing bloom layers
To add a new bloom layer create a javascript object with the properties listed on the Communicator.BloomLayerInfo page.
var bInterval = 1.0;
var bStyle = Communicator.BlurIntervalUnit.Pixels;
var bSample = 9.0;
var layerIntensity = 1.0;
var newLayer = {intensity: layerIntensity, blurSamples: bSample, blurInterval: [bInterval, bStyle]};
With the layer object created you can add it to the existing bloom layer array, which you can get with getBloomLayers(), and then set the bloom layers with the updated array using setBloomLayers().
// Get the current Bloom Layer Array
var bloomLayers = hwv.view.getBloomLayers();
// Add the new bloom layer
bloomLayers.push(newLayer);
// Set the Bloom Layer Array
hwv.view.setBloomLayers(bloomLayers);
If you want to discard all existing elements of the bloom layer array simply create a new array.
// Discard all exisiting bloom layers and use a new array with your layer
var newArray = [newLayer];
hwv.view.setBloomLayers(newArray);
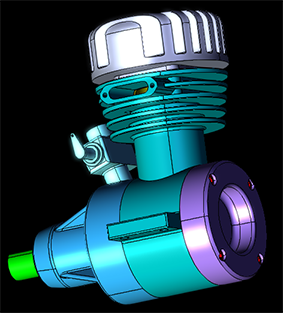
1 bloom layer (using the settings from above)
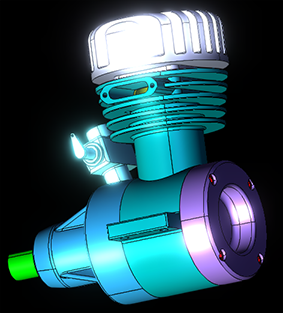
4 bloom layers (Using the settings from above)
Elements of the bloom layer array can be removed like any javascript array. After removing layers from the array you must use setBloomLayers() to update the viewer’s bloom layer array.
// Get the current bloom layer array
var bloomLayers = hwv.view.getBloomLayers();
// Remove the last element from the array
bloomLayers.pop();
// Remove an element at the given index (here we are removing the first element)
bloomLayers.splice(0, 1);
// Update the viewer's bloom layers
hmv.view.setBloomLayers(bloomLayers);
Simple shadows
Simple shadows are full-scene shadow projected onto an invisible ground plane. By default simple shadows are disabled. They can be enabled by passing a true boolean to the setSimpleShadowEnabled() function. To get the viewer’s simple shadow setting use getSimpleShadowEnabled().
// Enable simple shadows
hwv.view.setSimpleShadowEnabled(true);
// Disable simple shadows
hwv.view.setSimpleShadowEnabled(false);
// Get the viewer's simple shadow setting
hwv.view.getSimpleShadowEnabled();
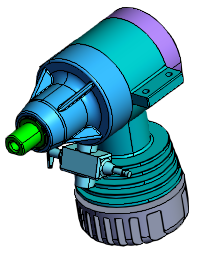
Simple shadow disabled
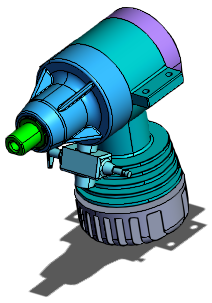
Simple shadow enabled
Simple shadow opacity
The opacity of the simple shadows can be set by passing a number (with higher value numbers being more opaque) to setSimpleShadowOpacity(). To retrieve the viewer’s current simple shadow opacity use getSimpleShadowOpacity(). The default shadow opacity is 0.65.
// Set the simple shadow opacity
hwv.view.setSimpleShadowOpacity(0.2);
// Get the simple shadow opacity
hwv.view.getSimpleShadowOpacity();
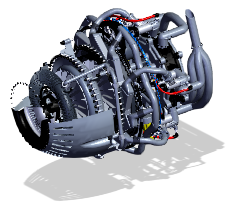
Simple shadow opacity 0.2
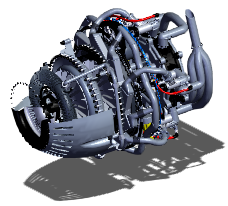
Simple shadow opacity 0.65 (default)
Simple shadow color
The color of the simple shadow can also be changed by calling the setSimpleShadowColor() function with a Communicator.Color object. Get the current simple shadow color by using getSimpleShadowColor(). The default shadow color is black (0, 0, 0).
// Create a red color object
var redShadow = new Communicator.Color(180, 30, 30);
// Set the simple shadow color
hwv.view.setSimpleShadowColor(redShadow);
// Get the current shadow color
hwv.view.getSimpleShadowColor();
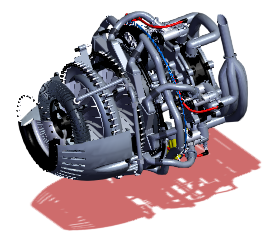
Red simple shadow
Simple shadow resolution
Sets the width and height in pixels of the texture image into which simple shadows are drawn. Set the resolution by passing a number to setSimpleShadowResolution(). Retrieve the viewer’s simple shadow resolution with getSimpleShadowResolution(). The default simple shadow resolution is 512.
// Set Simple Shadow Resolution
hwv.view.setSimpleShadowResolution(1024);
// Get Simple Shadow Resolution
hwv.view.getSimpleShadowResolution();
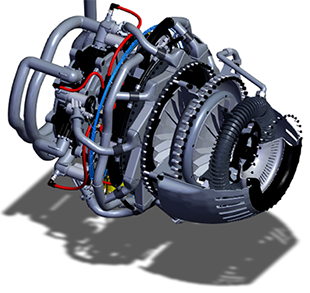
Simple shadow resolution = 256
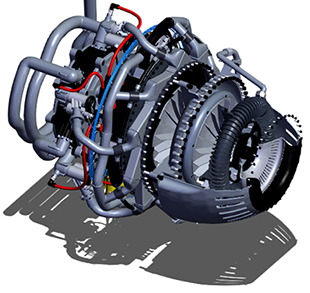
Simple shadow resolution = 2048
Simple shadow blurSamples
Sets the diameter of the blur filter used for simple shadows. Setting the value to 0 will disable blurring. Set the blurSamples
value by passing a number to the setSimpleShadowBlurSamples() function. Get the viewer’s blurSamples value by using getSimpleShadowBlurSamples().
The default blurSamples
value is 5.
// Set simple shadows blurSamples
hwv.view.setSimpleShadowBlurSamples(10);
// Get simple shadows blurSamples
hwv.view.getSimpleShadowBlurSamples();
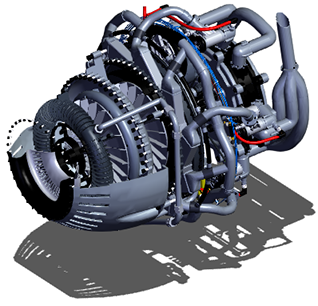
Simple shadow blurSamples = 1
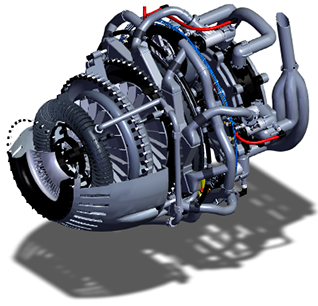
Simple shadow blurSamples = 10
Simple shadow blurInterval
Sets the distance in pixels between samples taken by the blur filter used for simple shadows. Set the blurInterval
value by passing a number to setSimpleShadowBlurInterval(). Get the viewer’s current simple shadow blurInterval value by calling getSimpleShadowBlurInterval().
The default value for simple shadow blurInterval is 1.
// Set the simple shadow blurInterval
hwv.view.setSimpleShadowBlurInterval(5);
// Get the current simple shadow blurInterval
hwv.view.getSimpleShadowBlurInterval();
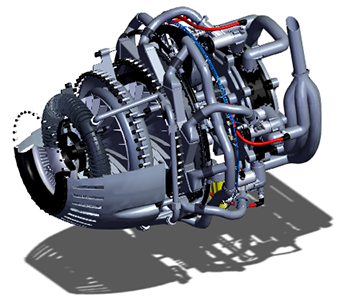
Simple shadow blurInterval = 1
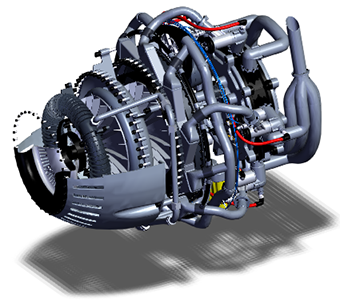
Simple shadow blurInterval = 5
Simple reflections
Simple reflections projected onto an invisible ground plane. Enable or disable by passing a boolean to the setSimpleReflectionEnabled().
Get the viewer’s simple reflection state with the getSimpleReflectionEnabled() function. Simple reflections are disabled by default.
// Disable Simple Reflections
hwv.view.setSimpleReflectionEnabled(false);
// Enable Simple Reflections
hwv.view.setSimpleReflectionEnabled(true);
// Get the viewer's simple reflection value
hwv.view.getSimpleReflectionEnabled();
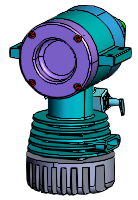
Simple reflection disabled
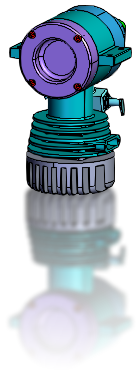
Simple reflection enabled
Simple reflection blurInterval
Sets the distance in pixels between samples taken by the blur filter used for simple reflections. Set the reflection blurInterval
by passing a number and a BlurIntervalUnit to setSimpleReflectionBlurInterval(). Get the current simple reflection blurInterval with getSimpleReflectionBlurInterval().
The default simple reflection blurInterval
value is [1, Pixels].
// Set the viewer's reflection blurInterval
hwv.view.setSimpleReflectionBlurInterval(3, Communicator.BlurIntervalUnit.Pixels);
// Get the reflection blurInterval
hwv.view.getSimpleReflectionBlurInterval();
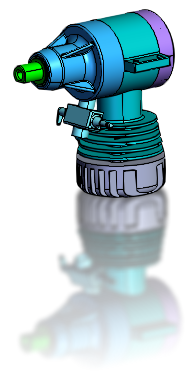
Simple reflection blurInterval (1, Pixels)
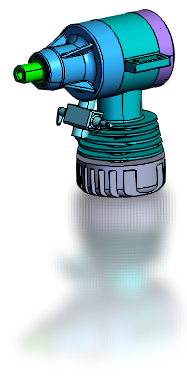
- Simple reflection blurInterval (3, Pixels)*
Simple reflection blurSamples
Sets the diameter of the blur filter used for simple reflections. Setting the value less than or equal to 1 will disable blurring. Set the samples value by passing a number to setSimpleReflectionBlurSamples(). Get the current blurSamples
value using getSimpleReflectionBlurSamples().
The default value is 9.
// Set simple reflection blurSamples
hwv.view.setSimpleReflectionBlurSamples(10);
// Get the simple reflection blurSamples value
hwv.view.getSimpleReflectionBlurSamples();
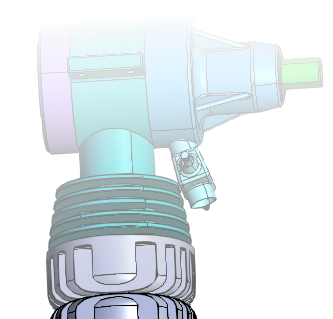
Simple reflection blurSamples = 0
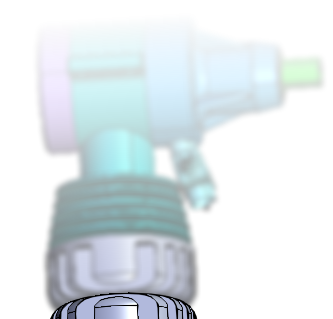
Simple reflection blurSamples = 10
Simple reflection fade angle
Sets the angle, in degrees, between the view vector and the ground plane at which simple reflections begin to fade. Settings the value to 0 will disable the fading effect. Regardless of the value, simple reflections will not be drawn if the camera is below the ground plane. Set the fade angle value by passing a number, representing degrees, to setSimpleReflectionFadeAngle(). Get the current simple reflection fade angle using getSimpleReflectionFadeAngle()
.
The default angle is 10 degrees.
// Set the fade angle
hwv.view.setSimpleReflectionFadeAngle(45);
// Get the fade angle
hwv.view.getSimpleReflectionBlurSamples();
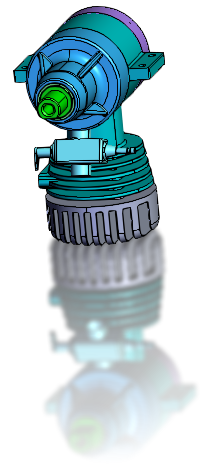
Simple reflection fade angle = 0
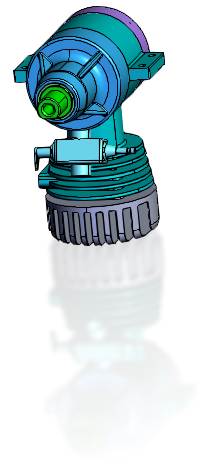
Simple reflection fade angle = 45
Simple reflection opacity
Set the opacity of simple reflections by passing a number (in the range 0 to 1, with values closer to 1 being more opaque) to setSimpleReflectionOpacity(). Get the existing simple reflection opacity with getSimpleReflectionOpacity().
The default simple reflection opacity is 0.65.
// Set the simple reflection opacity
hwv.view.setSimpleReflectionOpacity(0.2);
// Get the simple reflection opacity
hwv.view.getSimpleReflectionOpacity();
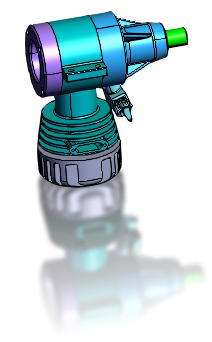
Simple Reflection Opacity = 0.65 (Default)
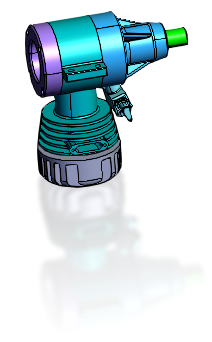
Simple reflection opacity = 0.2
Simple reflection attenuation
The setSimpleReflectionAttenuation() function controls how objects are drawn in simple reflections fade as they move further from the ground plane.
Attenuation begins at nearDistance
, the first argument to the function, and increases linearly such that the model is not visible in the reflection beyond farDistance
. Attenuation is disabled if farDistance
, the second argument to the function, is less than or equal to nearDistance
.
There is a third optional argument to setSimpleReflectionAttenuation() which is :doc:` </api_ref/viewing/enum/Communicator.SimpleReflectionAttenuationUnit>`. SimpleReflectionAttenuationUnit
specifies the unit which attenuation distances are specified for simple reflections. With the ProportionOfBoundingHeight unit distances will be multiplied by the distance from the ground plane to the furthest point on the scene bounding.
The other unit, World, uses world-space units.
Get the view’s current simple reflection attenuation with getSimpleReflectionAttenuation(). The default simple reflection value is {nearDistance: 0, farDistance: 1
, unit:
Communicator.SimpleReflectionAttenuationUnit.ProportionOfBoundingHeight}
// Set the simple reflection attenuation
hwv.view.setSimpleReflectionAttenuation(0, 5, Communicator.SimpleReflectionAttenuationUnit.ProportionOfBoundingHeight);
// Get the simple reflection attenuation
hwv.view.getSimpleReflectionAttenuation();
The captions below list the images simple reflection attenuation settings.
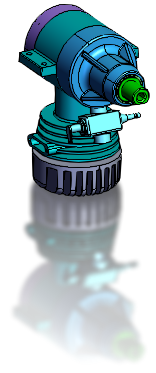
{near: 0, far: 1, unit: ProportionOfBoundingHeight}
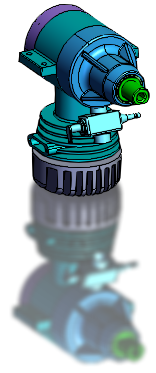
{near: 0, far: 5, unit: ProportionOfBoundingHeight}
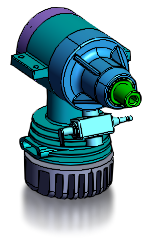
{near: 0, far: 20, unit: World}
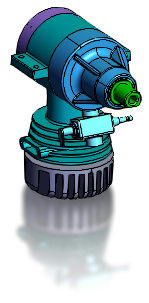
{near: 0, far: 50, unit: World}
Silhouette edges
The silhouette edges effect draws lines around geometrical edges. To enable or disable silhouette edges pass a Boolean to setSilhouetteEnabled(). To get the viewer’s current silhouette edges status call getSilhouetteEnabled(). Silhouette edges are disabled by default. Silhouette edges are always enabled in hidden line mode.
// Disable Silhouette Edges
hwv.view.setSilhouetteEnabled(false);
// Enable Silhouette Edges
hwv.view.setSilhouetteEnabled(true);
// Get the Silhouette Edges status
hwv.view.getSilhouetteEnabled();
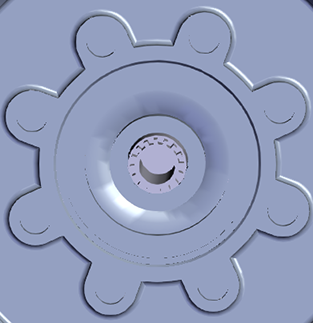
Silhouette edges disabled
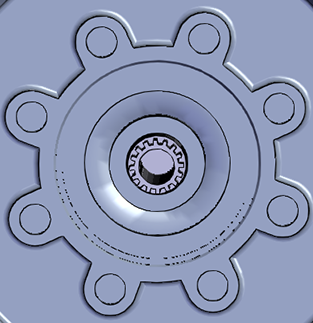
Silhouette edges enabled
Silhouette color
The color of silhouette edges can be set by passing a Communicator.Color to setSilhouetteColor(). Get the current silhouette color by calling getSilhouetteColor(). The default silhouette color is black (0, 0, 0).
// Set the Silhouette Edge Color to red
var redColor = new Communicator.Color(200, 50, 50);
hwv.view.setSilhouetteColor(redColor);
// Get the Silhouette Edge Color
hwv.view.getSilhouetteColor();
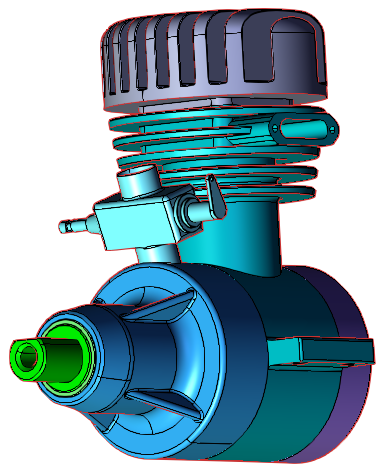
Red silhouette color
Silhouette opacity
Set the opacity of silhouette edges by calling setSilhouetteOpacity() with a number (in the range 0 to 1 with values close to 1 being more opaque). The current silhouette edge opacity can be obtained with getSilhouetteOpacity(). The default silhouette edge opacity is 1.
// Set the silhouette edge opacity
hwv.view.setSilhouetteOpacity(0.5);
// Get the silhouette edge opacity
hwv.view.getSilhouetteOpacity();
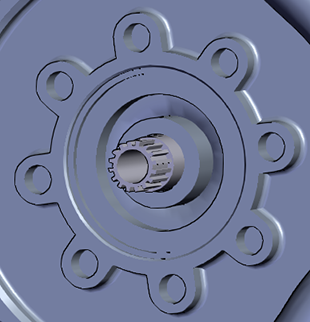
Silhouette Opacity = 1 (Default)
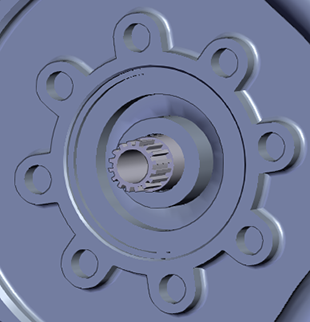
Silhouette Opacity = 0.5
Silhouette threshold
Set the distance threshold for silhouette edges by passing a number to setSilhouetteThreshold(). This value affects the minimum z-distance required between two pixels for an edge to be drawn. A smaller value will result in more edges being drawn on finer details. The value is a proportion of the canvas size and not a world-space distance. Get the current silhouette threshold with getSilhouetteThreshold(). The default threshold is 0.05.
// Set the silhouette threshold
hwv.view.setSilhouetteThreshold(0.2);
// Get the silhouette threshold
hwv.view.getSilhouetteThreshold();
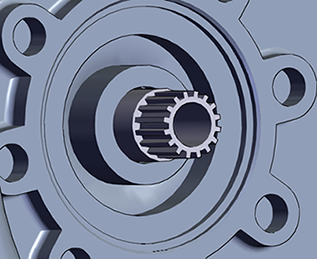
Silhouette Threshold = 0.05 (default)
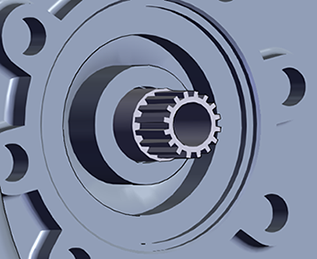
Silhouette Threshold = 0.2
Silhouette threshold ramp width
Control how quickly edges fade as z-distance between pixels decreases by passing a number to setSilhouetteThresholdRampWidth(). This value is added to the one set by setSilhouetteThreshold() to create a secondary threshold. Distances greater than the secondary threshold will result in edges with full opacity, and distances between the two thresholds will result in edges with reduced opacity. Setting this value to 0 will cause all edges to be drawn at full opacity.
Get the current silhouette threshold ramp width by calling getSilhouetteThresholdRampWidth().
The default value is 0.025.
// Set the silhouette threshold ramp width
hwv.view.setSilhouetteThresholdRampWidth(0.2);
// Get the silhouette threshold ramp width
hwv.view.getSilhouetteThresholdRampWidth();
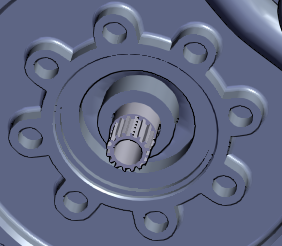
Silhouette Threshold Ramp Width = 0.025 (Default)
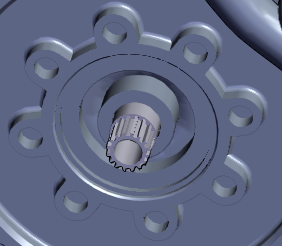
Silhouette Threshold Ramp Width = 0.2