Basics
Set/unset face colors
To set the face color of one or more nodes you can use the setNodesFaceColor function. Provide setNodesFaceColor()
with a NodeId
array and a color to set all the array elements faces to that color.
The following code gets nodeIds from the selection in webviewer and sets the color of the nodes’ faces to black. Multiple nodes can be selected by holding Ctrl while left clicking.
var partIds = [];
// Add selected parts to partIds
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
// Color nodes black
hwv.model.setNodesFaceColor(partIds, Communicator.Color.black());
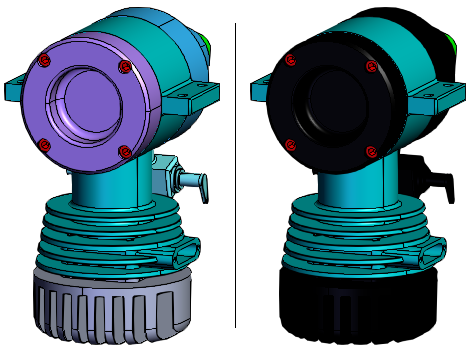
Several nodes’ faces in the model on the right have been colored black
To unset the face color of nodes you can call unsetNodesFaceColor and provide it the array of NodeIds whose color you want to unset.
// Unset the color of the selected nodes
hwv.model.unsetNodesFaceColor(partIds);
You can get nodes’ face color with getNodesFaceColor or getNodesEffectiveFaceColor(). Both functions take an array of NodeIds
and return a promise containing a color array. getNodesFaceColor will return colors set using setNodesFaceColor, but if a node’s color hasn’t been set this function will return null
at that index in the array. getNodesEffectiveFaceColor() will return the set color, but if not color has been set it will return the color specified when the model was authored.
// Print the face color for the selected nodes only if they have been set
hwv.model.getNodesFaceColor(partIds).then(function (result) {
console.log(result);
});
// Print the face color for the selected nodes
hwv.model.getNodesEffectiveFaceColor(partIds).then(function (result) {
console.log(result);
});
You can also retrieve the color of a specific face on a node with getNodeEffectiveFaceColor(). This function takes a partId and a faceIndex
then returns a Promise
containing the color of the specified face.
// Use the node id of the selected item and its face at the 0th index
var promiseColor = hwv.model.getNodeEffectiveFaceColor(selectionItem.getNodeId(), 0);
// Print the color of the face to the console
promiseColor.then(function (result) {
console.log(result);
});
Set/unset line colors
To set the line color of a one or more nodes the setNodesLineColor function can be used. Provide setNodesLineColor() with a nodeId
array and a color to set all the listed nodes’ lines to that color.
The following code gets nodeIds from the selection in webviewer and sets their line color to red. Multiple nodes can be selected by holding CTRL while left clicking.
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
hwv.model.setNodesLineColor(partIds, Communicator.Color.red());
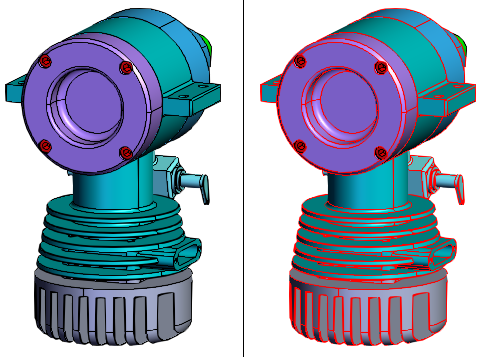
Several nodes’ lines in the model on the right have been colored red
To unset the nodes line color call the unsetNodesLineColor() function and pass it an array of node ids whose color you wish to unset.
hwv.model.unsetNodesLineColor(partIds);
You can retrieve the current color of several nodes’ lines with getNodesLineColor() or getNodesEffectiveLineColor(). For getNodesLineColor()
pass in a NodeId array and the function will return a promise containing a color array for the nodes’ lines, note that it will return null for nodes that have not had their line color set. The getNodesEffectiveLineColor()
function takes a NodeId array and returns a promise containing a color array with an element for each node in the argument array. For this function, if no line color has been set it will return the color of the line that was specified when the model was authored.
// Make a NodeId array from the selected nodes
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
// Print the color of the input nodes' lines only if they have been set
hwv.model.getNodesLineColor(partIds).then(function (result) {
console.log(result);
});
// Print the color of input nodes' lines
hwv.model.getNodesEffectiveLineColor(partIds).then(function (result) {
console.log(result);
});
To get the color from a specific line element of a node call getNodesEffectiveLineColor()
and pass it a PartId
and a lineIndex. The function will return a promise containing the color of the line element at the input index.
// For each selected item print off the color of the 0th line element
hwv.selectionManager.each(function (selectionItem) {
hwv.model.getNodeEffectiveLineColor(selectionItem.getNodeId(), 0).then(function (result) {
console.log(result);
});
});
Reset node face and line color
To reset all nodes’ faces and lines back to their default color call resetNodesColor(). This will also reset colors set using a color map as described in the next section.
hwv.model.resetNodesColor();
Color map
Communicator gives users the ability to color many nodes at once with the setNodesColors(). By providing the function with a map (from NodeId to Color) it will color nodes in the model with a specific color. setNodesColors()
also takes an optional third argument, in the form of a Boolean, that will also apply the color from the map to the models lines.
The code below creates a map from node ID to color and uses it with setNodesColors()
:
var red = Communicator.Color.red();
var green = Communicator.Color.green();
var blue = Communicator.Color.blue();
// Create a mapping from node id to color
var colorMap = { 6: red, 8: green, 9: blue, 10: blue, 11: green };
// Use the color map to set the nodes' colors
hwv.model.setNodesColors(colorMap);
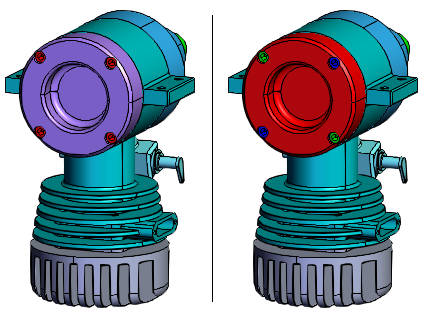
Several nodes on the right have been colored using a color map
Color maps can be obtained from models with the getNodeColorMap() function. The first argument to the function is the startNodeId
. This is the starting node to walk from to build the color map. The second argument is an ElementType (faces, lines or points). The function will return a color map for the specified ElementType
. Note that the array will only contain entries for nodes colored using setNodesColors()
.
// Walk from the first node and construct a map of all nodes face colors
var promiseMap = hwv.model.getNodeColorMap(0, Communicator.ElementType.Faces);
// Print the color map to the console
promiseMap.then(function (result) {
console.log(result);
});
Point color
When creating a mesh instance composed of points several properties can be set. The function below generates data that can be used to create a cube mesh instance made of many points.
function createPointCube(size) {
const points = [];
const pointCube = new Communicator.MeshData();
for (let x = -size; x <= size; x++) {
for (let y = -size; y <= size; y++) {
for (let z = -size; z <= size; z++) {
points.push(x, y, z);
}
}
}
pointCube.addPoints(points);
return pointCube;
}
Using the function from above we create a mesh and obtain a MeshId.
const size = 20;
const cubeMeshData = createPointCube(size);
const cubeMeshId = await hwv.model.createMesh(cubeMeshData);
With the MeshId
, we can create a meshInstanceData
object, before doing this we can specify the color and other properties of the points.
let meshInstanceData = new Communicator.MeshInstanceData(cubeMeshId);
// Set the color of the points to blue
meshInstanceData.setPointColor(Communicator.Color.blue());
hwv.view.setPointShape(Communicator.PointShape.Sphere);
hwv.view.setPointSize(10, Communicator.PointSizeUnit.ScreenPixels);
meshInstanceData.setPointOpacity(1.0);
const nodeId = await hwv.model.createMeshInstance(meshInstanceData);
In the code above the color of the points is set to red. The opacity, shape and size of the points are also specified. The images below demonstrate the effect these settings can have on meshes composed of points.
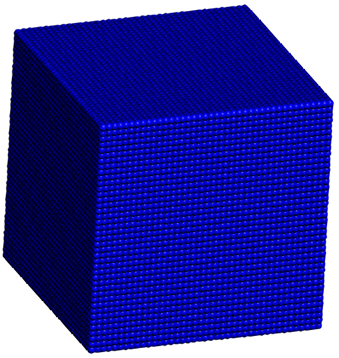
Cube made of blue sphere points
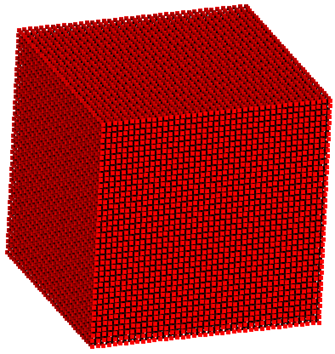
Cube made of red square points
Opacity
The transparency of several nodes can be set at once using setNodesOpacity(). The first argument to setNodesOpacity()
is a NodeId
array and the second argument is the opacity to set the nodes to. The value of the second argument should be in the range 0.0 to 1.0, with values near 0.0 being more transparent and values nearing 1.0 being more opaque.
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
hwv.model.setNodesOpacity(partIds, 0.3);
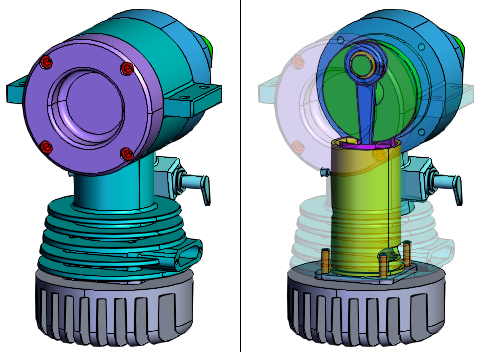
Several nodes on the right have had their opacity set to 0.3
To get the opacity of several nodes use either getNodesOpacity() or getNodesEffectiveOpacity(). getNodesOpacity()
requires a NodeId
array as input and will return a Promise containing an array of opacities for each node. The value will only be returned for nodes whose opacities have been set. getNodesEffectiveOpacity()
takes a NodeId
array and an ElementType
as input and returns the opacity for the specified elements of the input nodes. If the nodes opacity has not been set this function will return the opacity for the node as it specified when it was authored.
// Make a NodeId array from the selected nodes
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
// Print the opacity of the input nodes only if it has been set
hwv.model.getNodesOpacity(partIds).then(function (result) {
console.log(result);
});
// Print the opacity of input nodes
hwv.model
.getNodesEffectiveOpacity(partIds, Communicator.ElementType.Faces)
.then(function (result) {
console.log(result);
});
To reset the opacity of specific nodes use resetNodesOpacity. The opacity of all nodes in a model can be reset with resetModelOpacity().
// Get the node ids of the selected nodes
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
// Reset the input nodes' opacities
hwv.model.resetNodesOpacity(partIds);
// Reset the opacity of all nodes in the model
hwv.model.resetModelOpacity();
Visibility
The visibility of nodes can be disabled or enabled by using setNodesVisibility(). The first argument to the function is a NodeId
array. The second argument is a boolean that determines if nodes will be visible or hidden. The third argument is a boolean and is optional it will control if initially hidden geometries will stay hidden.
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
hwv.model.setNodesVisibility(partIds, false);
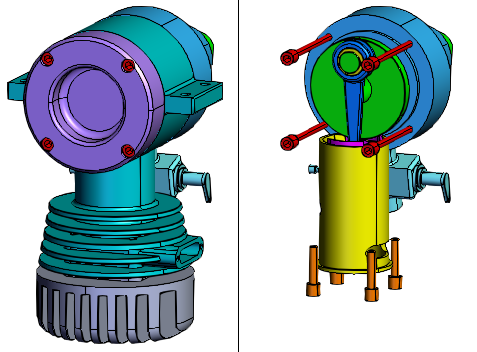
Several nodes on the right have been hidden
You can get the visibility setting of a node using getNodeVisibility().
// Print the visibility of the selected nodes to the console
hwv.selectionManager.each(function (selectionItem) {
console.log(hwv.model.getNodeVisibility(selectionItem.getNodeId()));
});
The visibility of all nodes can be reset with resetNodesVisibility().
hwv.model.resetNodesVisibility();
Visibility map
Node visibilities can also be set using a map (from node ID to Boolean) using setNodesVisibilities().
var visibilityMap = { 6: false, 8: false, 9: false, 10: true, 11: true };
hwv.model.setNodesVisibilities(visibilityMap);
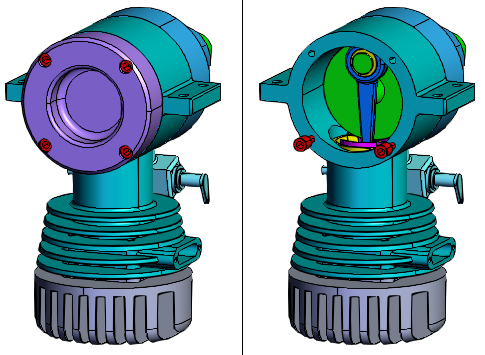
Several nodes on the right have been hidden using a visibility map