Line Patterns
Line patterns give you the ability to customize the lines on nodes.
Setting/unsetting line patterns
To set a node’s line pattern call the setNodesLinePattern() function. The first argument to the function is a NodeId array, elements of this array are the nodes who will have their line pattern set. The next argument is the LinePattern you want to use. A line pattern is an array of 1’s or 0’s where 1’s represent visible pixels and 0’s represent invisible pixels. Following the LinePattern argument pass a number that specifies the patternLength which is the length of a single repetition of the pattern. The final argument to the function is the patternLengthUnit which specifies the unit used to measure the length of the pattern. The code snippet below sets a basic dashed line pattern on nodes selected in the Web Viewer.
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
// Set the selected nodes' lines to follow a basic pattern with a pattern length of 1 measured in object space units
hwv.model.setNodesLinePattern(partIds, [1, 0], 1, Communicator.LinePatternLengthUnit.Object);
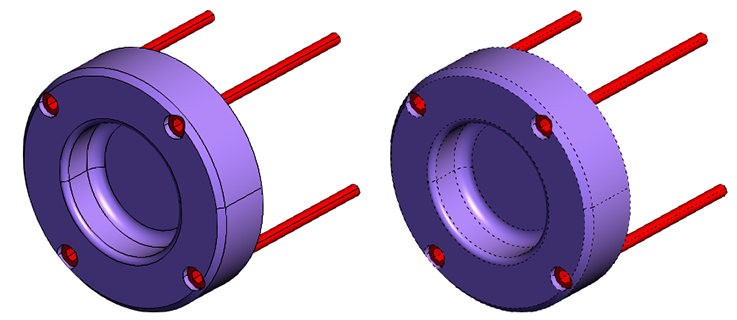
On the right, a basic dashed line pattern has been set.
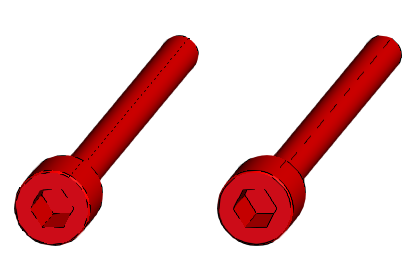
Pattern length is 1 on the left and 4 on the right. Both use object space as a unit of measurement.
To remove a line pattern from a node use the unsetNodesLinePattern() function.
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
hwv.model.unsetNodesLinePattern(partIds);
Define a style
To customize the appearance of your line pattern you can pass a unique array of 1’s and 0’s as the second argument to setNodesLinePattern(). Additionally, users can set a line pattern in conjunction with setting nodes’ line colors to further customize their nodes’ lines. In the snippet below the selected nodes’ lines are set to a red color and are set to follow a pattern described as [1, 1, 1, 1, 0, 0, 1, 0, 0]. This will produce a pattern with a dash followed by a gap, then a dot, then another gap before the pattern repeats itself. The size of the pattern below has been set to 5 using World space as a unit of length measurement.
var partIds = [];
hwv.selectionManager.each(function (selectionItem) {
partIds.push(selectionItem.getNodeId());
});
// Set the color of the nodes lines
hwv.model.setNodesLineColor(partIds, Communicator.Color.red());
// Set the nodes line pattern
hwv.model.setNodesLinePattern(
partIds,
[1, 1, 1, 1, 0, 0, 1, 0, 0],
5,
Communicator.LinePatternLengthUnit.World,
);
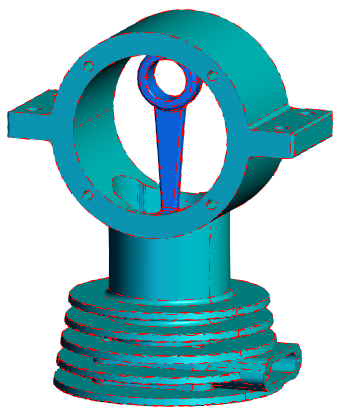
A model using the line color and pattern settings from the snippet above.
Limitations
Line patterns limitations concern the LinePatternLengthUnit.ProportionOfScreenHeight and LinePatternLengthUnit.ProportionOfScreenWidth properties.
When using these units to scale your line pattern it will cause the pattern to appear the same regardless of zoom or distance from the camera. Measuring the length for line patterns these ways requires a calculation for each vertex in the line reducing prerformance compared to the other LinePatternLengthUnits.