UnstructGrid: Create a cutting plane with a scalar result as fringes
Cutting planes are slices through the structure onto which scalar and vector fields can be visualized. By dynamically changing the position and orientation of the cutting plane, you can quickly analyze the results inside the system.
This example shows how to create a cutting plane and show a scalar result mapped to the surface. To see the cutting plane, the part will be set transparent.
The demo file (30x30x30.vtf) contains a single part geometry with a synthetic scalar result.
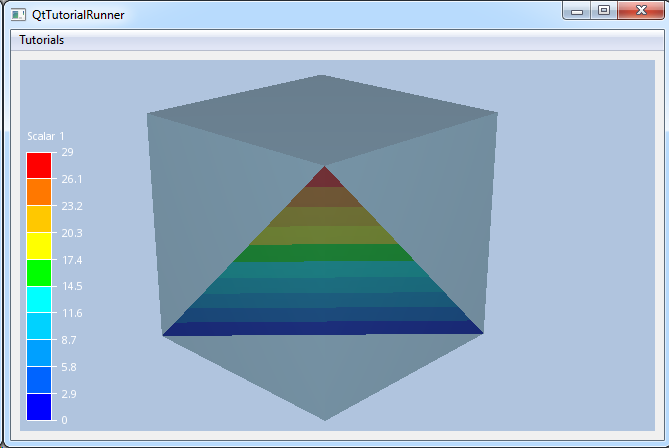
void TutorialRunnerMainWindow::addCuttingPlane()
{
//--------------------------------------------------------------------------
// Load a UnstructGridModel from file
// Create a cutting plane
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTF> source = new cee::ug::DataSourceVTF(42);
// Open the vtf file
cee::Str vtfFile = TutorialUtils::testDataDir() + "30x30x30.vtf";
if (!source->open(vtfFile))
{
// VTF file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the first state as current
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[0].id();
ugModel->modelSpec().setStateId(stateId);
// Get the id of the scalar result
std::vector<cee::ug::ResultInfo> scalarResultInfos = source->directory()->scalarResultInfos();
int scalarId = scalarResultInfos[0].id();
// Create a new cutting plane and set a point and normal
cee::PtrRef<cee::ug::CuttingPlane> cuttingPlane = new cee::ug::CuttingPlane();
cuttingPlane->setPoint(cee::Vec3d(10, 10, 10));
cuttingPlane->setNormal(cee::Vec3d(1, 1, 1));
// Map the scalar result on the cutting plane surface
cuttingPlane->setMapScalarResultId(scalarId);
// Add the cutting plane to the model
ugModel->addCuttingPlane(cuttingPlane.get());
// To be able to see the cutting plane inside the part, set transparency for all parts
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setOpacity(0.5f);
}
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}