UnstructGrid: Load model from file and set up model specification
This tutorial shows how to create a data source by loading a VTFx file and setup a model specification.
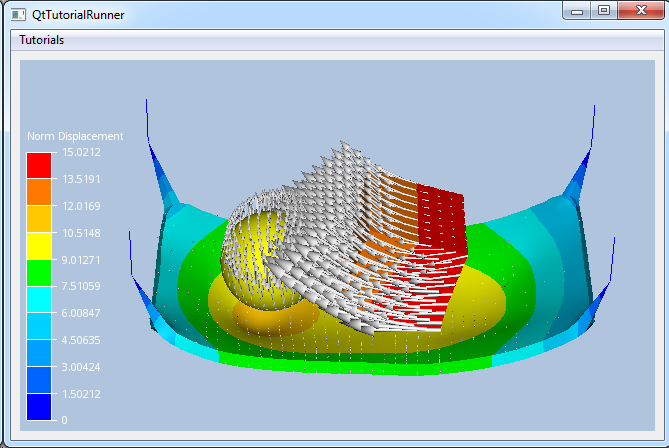
This data source contains multiple states, scalar results, vector results and a displacement result. After opening the file, the available metadata can be queried through the data source directory.
In this example you will set up the model specification to show the following:
Set the last state id as current
Set the first scalar result as fringes on the model
Set the first vector result
Set the first displacement result
Note
This tutorial expect the application to have a correctly configured cee::vis::View in place. See demo applications on how to set up a cee::vis::View in your application.
Create the model and data source
Create a model and a data source.
In this tutorial you will be reading a data source from a VTFx file so you need an instance of the VTFx file interface data source, cee::ug::DataSourceVTFx.
Give the data source a unique id.
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
Open file
Open the test file (contact.vtfx) included in the tutorial data files. The open() function will return true if the file was opened successfully.
Set the data source in the model.
cee::Str vtfxFile = TutorialUtils::testDataDir() + "contact.vtfx";
if (!source->open(vtfxFile))
{
// VTFx file not found
return;
}
ugModel->setDataSource(source.get());
Setup model specification
After opening the file, the metadata will be available through the data source’s directory through cee::ug::DataSource::directory().
The data source directory contains the following metadata for the data source:
State info
Scalar results info
Vector results info
Displacement results info
Parts info
Transformation result info
The cee::ug::ModelSpec object is the model specification. Each cee::ug::UnstructGridModel will own a model specification object.
The model specification describes the following settings for the model:
An array of state ids. Must always be at least one state set in the model specification.
A fringes result
An array of vector results
A displacement result
Use transformation result true/false
Use the information retrieved from the data source directory to set up the model specification.
Set state
Get metadata for all states and set the last state as current in the model specification.
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
if (stateInfos.size() > 0)
{
int lastStateId = stateInfos[stateInfos.size() - 1].id();
ugModel->modelSpec().setStateId(lastStateId);
}
Set result as fringes
Get metadata for all available scalar results and set the first scalar to show as fringes in the model specification.
std::vector<cee::ug::ResultInfo> scalarResultInfos = source->directory()->scalarResultInfos();
if (scalarResultInfos.size() > 0)
{
int scalarId = scalarResultInfos[0].id();
ugModel->modelSpec().setFringesResultId(scalarId);
}
Set result as vector
Get metadata for all available vector results and set the first vector in the model specification.
std::vector<cee::ug::ResultInfo> vectorResultInfos = source->directory()->vectorResultInfos();
if (vectorResultInfos.size() > 0)
{
int vectorId = vectorResultInfos[0].id();
ugModel->modelSpec().setVectorResultId(vectorId);
}
Set displacement result
Get metadata for all available displacement results and set the first displacement in the model specification.
std::vector<cee::ug::ResultInfo> dispResultInfos = source->directory()->displacementResultInfos();
if (dispResultInfos.size() > 0)
{
int dispId = dispResultInfos[0].id();
ugModel->modelSpec().setDisplacementResultId(dispId);
}
Set result visibility
Toggle on result visibility for all parts for the selected result types. For easy access to the settings for all available parts in a model, use the cee::ug::PartSettingsIterator.
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setFringesVisible(true);
partSettings->setVectorsVisible(true);
partSettings->setDisplacementVisible(true);
}
Set up the created model
The model is ready to use and can be added to the view. Exactly where the view exists depends on the platform and solution. These examples uses Qt and the view is set up in a cee::qt::ViewerWidget.
cee::vis::View* gcView = getTutorialView();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
See the complete source code here:
UnstructGrid: Load model from file and set up model specification