Report: Create a simple Word report with a 3D model
Using the Report Component, one can generate highly customizable reports in Word, PowerPoint and HTML with integrated and interactive 3D models.
In this example we will load a VTFx model file and use this in a Word report together with some other information and metadata gathered from the model.
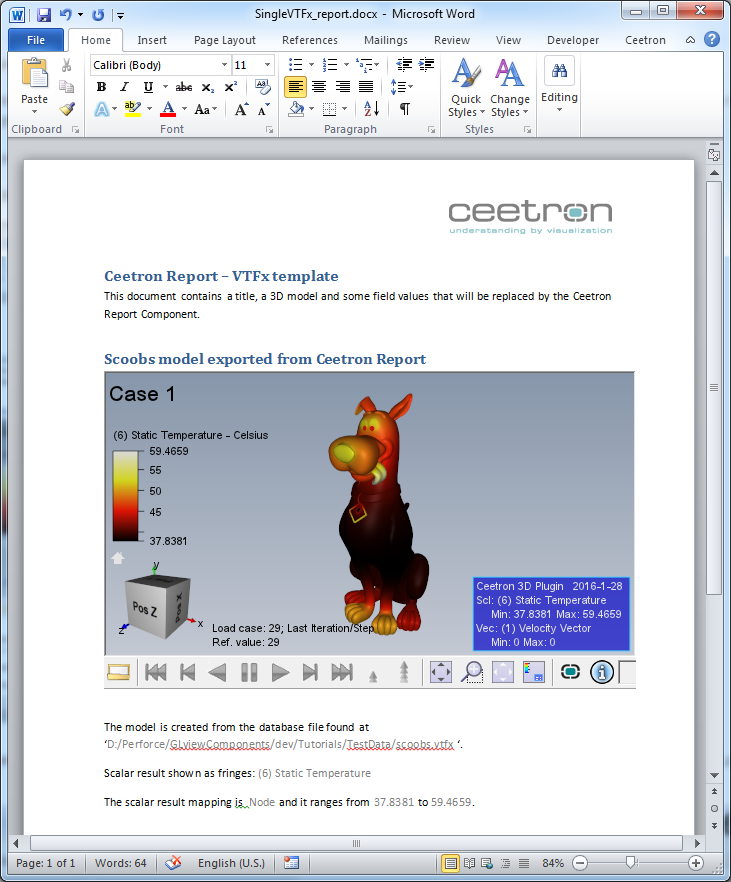
void TutorialRunnerMainWindow::createReport()
{
//--------------------------------------------------------------------------
// Load a UnstructGridModel with properties from file and
// visualize in view
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
// Open the VTFx file
cee::Str vtfxFile = TutorialUtils::testDataDir() + "scoobs.vtfx";
if (!source->open(vtfxFile, 0))
{
// VTFx file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
// Set the first state as current.
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[0].id();
ugModel->modelSpec().setStateId(stateId);
// Read model properties and view properties from the VTFx data source
cee::PtrRef<cee::PropertySetCollection> propertyCollection = new cee::PropertySetCollection;
cee::PtrRef<cee::ImageResources> imgResources = new cee::ImageResources;
source->caseProperties(propertyCollection.get(), imgResources.get());
cee::ug::PropertyApplierVTFx propApplier(propertyCollection.get(), imgResources.get());
propApplier.applyToModel(ugModel.get());
// Set up the initial visualization of this model
ugModel->updateVisualization();
// Default camera setup
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
// Apply properties to view
// Apply properties after default view setup so we get a default view if camera is not specified
propApplier.applyToView(gcView);
gcView->requestRedraw();
//--------------------------------------------------------------------------
// Create a repository of snapshots.
// This repository will only contain one VTFx snapshot.
//--------------------------------------------------------------------------
// Create a VTFx file exporter
cee::exp::ExportVTFx exporter(cee::exp::ExportVTFx::DISPLAY_MODEL_ONLY, ugModel.get());
// Add properties and resources to the export
cee::PtrRef<cee::PropertySetCollection> propColl = new cee::PropertySetCollection;
{
cee::PtrRef<cee::ImageResources> resources = new cee::ImageResources;
cee::exp::PropertyBuilderVTFx propBuilder(propColl.get(), resources.get());
propBuilder.addFromModel(*ugModel.get());
propBuilder.addFromView(*gcView);
exporter.addProperties(*propColl);
exporter.addResources(*resources);
}
// Export the VTFx model to a memory file
cee::PtrRef<cee::ug::VTFxMemoryFile> memoryFile = new cee::ug::VTFxMemoryFile();
if (!exporter.saveCase(memoryFile.get()))
{
// Export failed
return;
}
// Create a VTFx snapshot and add it to the repository
cee::PtrRef<cee::rep::Repository> repository = new cee::rep::Repository();
{
cee::PtrRef<cee::rep::Snapshot> snapshot = new cee::rep::Snapshot(cee::rep::Snapshot::OBJECT_VTFX);
snapshot->setTitle("Scoobs model exported from Ceetron Report");
snapshot->setFieldValues(cee::rep::FieldValuesGenerator::getFieldValuesFromModel(ugModel.get()));
snapshot->setModelVTFx(memoryFile.get());
repository->addSnapshot(snapshot.get());
}
// Generate a Word report based on the repository and a Word document
cee::Str templateFile = TutorialUtils::testDataDir() + "SingleVTFx.docx";
cee::rep::ReportCreatorWord generator(repository.get(), templateFile);
if (generator.generate("SingleVTFx_report.docx"))
{
// Report generation failed
return;
}
}