UnstructGrid: Load model from file and set up model specification
This example shows how to load a data source from a VTF file and set up the model specification.
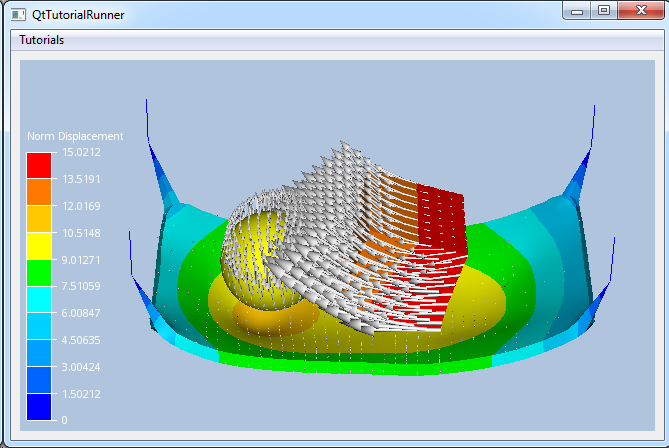
This data source contains multiple states, scalar results, vector results and a displacement result. After opening the file, the available metadata can be queried through the data source directory. In this example you will set up the model specification to show the following:
Set the last state id as current
Set the first scalar result as fringes on the model
Set the first vector result
Set the first displacement result
Remember to toggle on result visibility in each part’s PartSettings.
void TutorialRunnerMainWindow::loadVtfAndSetResults()
{
//--------------------------------------------------------------------------
// Load a UnstructGridModel from file and visualize results
// Get meta data from data source directory
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
// Open the vtfx file
cee::Str vtfxFile = TutorialUtils::testDataDir() + "contact.vtfx";
if (!source->open(vtfxFile))
{
// VTFx file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the last state as current
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
if (stateInfos.size() > 0)
{
int lastStateId = stateInfos[stateInfos.size() - 1].id();
ugModel->modelSpec().setStateId(lastStateId);
}
// Show first scalar result as fringes on the model
std::vector<cee::ug::ResultInfo> scalarResultInfos = source->directory()->scalarResultInfos();
if (scalarResultInfos.size() > 0)
{
int scalarId = scalarResultInfos[0].id();
ugModel->modelSpec().setFringesResultId(scalarId);
}
// Show first vector result on the model
std::vector<cee::ug::ResultInfo> vectorResultInfos = source->directory()->vectorResultInfos();
if (vectorResultInfos.size() > 0)
{
int vectorId = vectorResultInfos[0].id();
ugModel->modelSpec().setVectorResultId(vectorId);
}
// Show first displacement result on the model
std::vector<cee::ug::ResultInfo> dispResultInfos = source->directory()->displacementResultInfos();
if (dispResultInfos.size() > 0)
{
int dispId = dispResultInfos[0].id();
ugModel->modelSpec().setDisplacementResultId(dispId);
}
// Set result visibility for all parts
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setFringesVisible(true);
partSettings->setVectorsVisible(true);
partSettings->setDisplacementVisible(true);
}
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}