Interacting With the Scene
During interactive input, it is important to quickly provide feedback to the user. Consider drawing a rubberband box to define an area to zoom into or a set of objects to select. To define the rubberband box, we need to be able draw a polyline, and during each subsequent mouse move, erase the polyline and redraw it. Another example of interaction would be to edit or move a piece of geometry. This involves making a change to the geometry and redrawing it.
HOOPS/3dGS contains a drawing heuristic called “quick moves” that allows these kinds of interactions to be performed quickly. You can set this heuristic using Set_Heuristics
on a segment that contains geometry that you expect will be changing rapidly. Quick moves tell HOOPS/3dGS to draw this geometry such that it can be erased without the rest of the scene being disturbed. Specifically, if changes have only occurred within “quick moves” segments between updates, then only the geometry in those segments will be redrawn. (The “quick moves” geometry is erased and then redrawn.) If a segment has the “quick moves” heuristic set, then all of its parent segments will be visited first in the tree walk. This ensures that they receive preferential processing which is necessary in a variety of situations.
Regular Quick Moves
Regular quick moves is enabled by setting the “quick moves” heuristic. HOOPS/3dGS will automatically choose from a variety of methods in order to draw the geometry quickly; the default method may vary depending on the platform and graphics card:
Overlay: Geometry is drawn using hardware overlay planes. The quick-moved geometry will be drawn in the requested color. This is the default method for 3D drivers such as OpenGL and Direct3D.
XOR: Geometry is drawn using exclusive-or (XOR) operations on the contents of the frame buffer. Additionally, the color of the quick-moves geometry may be drawn in a color other then the one requested in those areas where the quick moved geometry overlaps other geometry in the scene. This is because quick moved geometry is XOR’ed with the window background color, thus geometry that doesn’t overlap with the window background will not have the requested color. This will be the default for 2D drivers such as GDI and X11. Note that this option is not available for the OpenGL2 and DX11 drivers.
You can request that one of the aforementioned drawing approaches be used by calling:
HC_Set_Driver_Options("quick moves preference = [xor/overlay/default]");
This simply denotes a preference that the system will try to use; there is no guarantee that the request will be honored. Refer to the reference manual docs on the option for details.
Quick Moves Properties/Limitations
- All geometry residing in segments with the “quick moves” setting will be redrawn on every update. Therefore, the amount of geometry drawn in this mode should be kept to a minimum. This setting should only be used for temporary rubberband geometry (such as select box or similar ‘handle’ or ‘grips’ geometry that is changing as you construct or edit something), or when there is a relatively small amount of geometry that is being edited (an item that is being moved from one location to another).
- As discussed previously, ‘regular’ quick-moved geometry will be drawn ‘on top’ of the scene, regardless of the z-values of the quick moved geometry.
- Polygons or shell/mesh faces/edges cannot be quick-moved if they have any advanced rendering options such as “smooth-shading” or “texturing”. They can only be quick-moved if they are either unlit (non-shaded) or have flat-shading rendering attributes.
- If a segment has ‘quickmoves’ Heuristic set, then all of its parent segments will be visited first in the tree walk. This ensures they receive preferential processing which is necessary in a variety of situations.
Example
A common example of when regular quick moves should be used is when drawing a ‘rubberband box’ to denote a selection area. The HOOPS/MVO class called HOpConstructRectangle
(defined in HOpConstructRectangle.h and implemented in HOpConstructRectangle.cpp) is based on the HBaseOperator
base class which provides virtual methods for various mouse and keyboard events. HOpConstructRectangle
draws a ‘rubberband box’ (represented by a polyline) by implementing the following methods:
#. HOpConstructRectangle::OnLButtonDown
Records the first mouse position and initiates the rectangle-drawing mechanism.
#. HOpConstructRectangle::OnLButtonDownAndMove
Draws a rubberbanded line from the first point in the rectangle to the current mouse position. Note that the basic drawing work is done by HUtility::InsertRectangle
, while OnLButtonDownAndMove
keeps track of the current points and draws the rubberband centroid. The rectangle is insert into the ‘windowspace’ segment, which had the “quick moves” heuristic set in it when it was first created.
#. HOpConstructRectangle::OnLButtonUp
Finalizes the size of the rectangle and cleans up.
Spriting-Based Quick Moves
Another quick moves drawing method called spriting can be used instead of regular quick moves by setting the HOOPS/3dGS heuristic “quick moves = spriting”. Just like with regular quick moves, if changes have only occurred within “quick moves=spriting” segments between updates, then only the geometry in those segments will be redrawn. However, spriting does not have the limitations of regular quick moves. Spriting ensures that:
- the object will be drawn in true 3d (rather than ‘on top’ of the scene)
- the object can be sprited regardless of the rendering attributes
- the color of the object will always look correct
Spriting thus enables transformation and editing of geometry in a large scene in true 3D, without redrawing the entire scene. This feature allows the developer to greatly enhance the way that the end-user interacts with individual pieces of geometry, particularly if there is a lot of geometry in the scene.
One might ask, why not always use the spriting quick moves features, instead of using the regular quick moves described above? There are several reasons. First, the performance of spriting quick moves depends on whether underlying hardware acceleration is available (though usually it is), and how many objects are being sprited. If the underlying hardware doesn’t accelerate spriting quick moves, the picture will still look correct, but the objects will not redraw as fast as just drawing some wireframe geometry using XOR or overlay planes.
Additionally, spriting requires a full update at the start to generate the “background” color and depth buffers.
Finally, it is sometimes NOT desirable to have the geometry be drawn in true 3D; you may require the geometry to be drawn on top of the scene (like a selection rectangle). Finally, if you simply want to draw something like a rubberband box, it will still be much faster to use regular quickmoves instead of spriting.
Spriting should generally be used when you wish to interact with or edit 3D objects (in true 3D) within a complex scene, while regular quickmoves should be used for drawing overlayed temporary/rubberband geometry that is not a part of the model but rather provides visual feedback during the interaction.
Quick Moves In-Place
The “inplace” overlay option becomes useful when you are using a highlight style to overlay geometry and that style contains transparency. In this situation, the overlay geometry is not drawn - the highlight itself is drawn in its place. Using this method incurs a small performance penalty relative to the other overlay options. If you are not using a transparent highlight style, this option will have no visible effect.
If you want a transparent highlight style while still having the quick move geometry visible, you need to enable spriting in the highlight style itself.
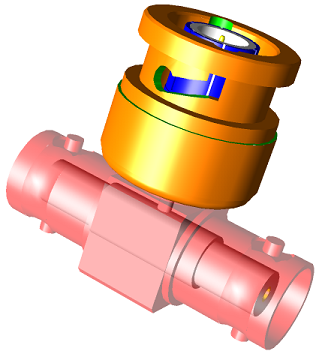
The model’s bottom tube has a transparent highlight style, using the “quick moves=inplace” overlay setting.