HOOPS/Java SWT Programming Guide
Introduction
The HOOPS/Java integration consists of a connection between HOOPS/3dGS and the Java/SWT ‘Canvas’ GUI object. This document describes how to use the HOOPS/Java integration to build a Java/SWT application that incorporates the HOOPS/3DF components. Some familiarity with Java/SWT, HOOPS/3DGS and HOOPS/MVO is assumed.
Developers should start by compiling and running the basic java_simple_swt application as the starting point for their application. This is an example for Java developers wishing to incorporate the HOOPS/3DF components into either existing or new Java applications. This integration uses the Java/SWT libraries. The readable source code is located in your <hoops>/demo/java/java_simple_swt directory.
Platform/Compiler Support
The HOOPS/Java integration is supported under the J2SE Development Kit 6.0 (JDK 6.0), on Windows 32-bit and 64-bit platforms.
As of the 23.20 release, the java_simple_swt application is no longer supported on Linux platforms.
Compilation and Runtime Information
The following steps are required to compile and run a HOOPS/Java based application:
Compiling: You must place the HOOPS/3DF and HOOPS/Java .jar files in your classpath. This can either be done via the command line for both compiling and running, or it can be set in the manifest file for your project. The files are:
- HJ.jar
- HJMVO.jar
- HJSTREAM.jar
- HoopsSwtCanvas.jar
- swt.jar
Executing: Ensure that the following files are in your application’s directory.
.jar files: (discussed above)
Java wrapper libraries:
- hj.dll
- hjmvo.dll
- hjstream.dll
Native libraries:
- hoops_vc.dll
- hoops_mvo_vc.dll
- hoops_stream_vc.dll
The above files should all be located in your <VISUALIZE_INSTALL_DIR>/bin/<PLATFORM> directory.
Component-Object Relationships
This section discusses the relationship between Java and HOOPS/3DF components. Building an application with both these toolkits minimally involves using the following objects from each component.
Java
A Java/SWT application would typically have a master document object.
HOOPS/Java
Your application should have at least one HoopsSwtCanvas; typically, you would create a custom canvas derived from HoopsSwtCanvas
. The readable source for the HoopsSwtCanvas
class can be found the <hoops>/Dev_Tools/hoops_java/source directory. This canvas interacts with the master document object.
HOOPS/MVO
HBaseModel
, HBaseView
, and an operator class derived from HBaseOperator
. Applications that want to implement selection of geometry will also need a HSelectionSet
object. These objects are all connected by private data members which store pointers to other objects in the following manner:
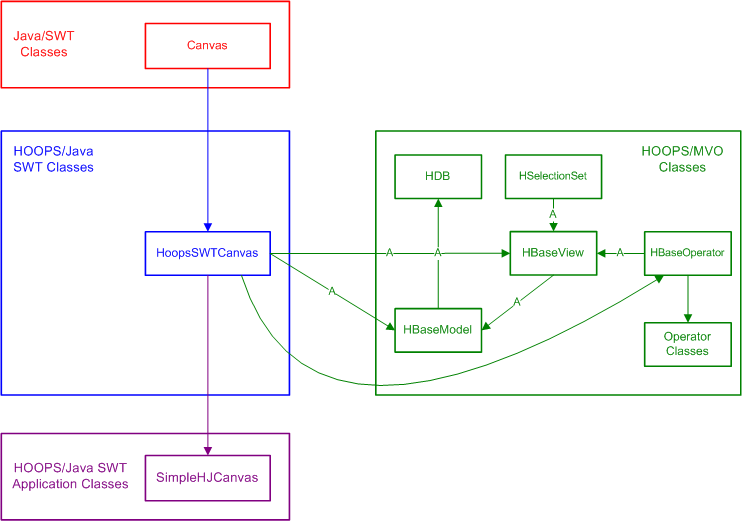
Steps to Building an Aplication with Java/SWT and HOOPS
Programming with an object oriented GUI framework like Java/SWT involves creating a set of objects and defining the ways in which they are connected, the manner in which they send and receive messages, and then launching the framework’s event loop. Building an application using Java/SWT and HOOPS/3DF specifically requires creation and initialization of:
Creating and Initializing the Application
A Java/SWT application typically creates a master application object which implements the function main()
. The java_simple_swt application provides an example which creates a custom class called SWTApplicaton
and calls its CreateNewDocument
method:
public class java_simple_swt
{
static public SWTApplicaton theApp;
public static void main(String[] argv)
{
theApp = new SWTApplicaton();
theApp.CreateNewDocument();
}
}
The sample SWTApplicaton
implementation includes a method called CreateNewDocument
which will create a our document object called SWTDocument
:
class SWTApplicaton
{
...
public void CreateNewDocument()
{
SWTDocument doc1 = new SWTDocument();
doc1.start();
}
}
class SWTDocument extends Thread
{
...
}
Creating and Initializing HOOPS/Java Objects
The GUI aspect of the HOOPS/Java integration simply consists of a customized Java/SWT canvas called SimpleHJCanvas
as diagramed up above. As many HoopsSwtCanvas
objects can be created as needed to implement the GUI’s design. The application should create a custom HoopsSwtCanvas
class, as shown in java_simple_swt:
class SimpleHJCanvas extends HoopsSwtCanvas
{
public SimpleHJCanvas(final Composite parent, final int style)
{
super(parent, style );
}
}
Your custom canvas will most likely be created and attached in your custom document. In this case, the SWTDocument
class extends the Thread
class. When start is called from SWTApplicaton::CreateNewDocument
, the Java Virtual Manual calls run. This is where the SimpleHJCanvas
is created, included as part of the shell and set to visible:
class SWTApplicaton
{
...
public void CreateNewDocument()
{
SWTDocument doc1 = new SWTDocument();
doc1.start();
}
}
class SWTDocument extends Thread
{
private SimpleHJCanvas m_SimpleHJCanvas;
public void run()
{
...
m_SimpleHJCanvas = new SimpleHJCanvas(shell, SWT.BORDER);
m_SimpleHJCanvas.setVisible(true);
}
}
Creating and Initializing HOOPS/MVO Objects
HDB
One global pointer to a HOOPS/MVO HDB object should be declared and initialized in the application’s main class. The java_simple_swt app does this in the SWTApplicaton
constructor:
class SWTApplicaton
{
static public HDB m_HDB;
public SWTApplicaton()
{
m_HDB = new HDB();
m_HDB.Init();
}
...
}
HBaseModel
For each SimpleHJCanvas
object there is one HBaseModel
. In the java_simple_swt app, HoopsSwtCanvas
, which is the parent class of SimpleHJCanvas, creates one in its constructor. SWTDocument
uses its instance of SimpleHJCanvas
to access the model for loading files:
class HoopsSwtCanvas extends Canvas
{
...
public HoopsSwtCanvas(final Composite parent, final int style) {
super(parent, style);
...
m_HModel = createDefaultModel();
m_HView = createView(m_HModel);
...
}
protected HBaseModel createDefaultModel() {
final HBaseModel model = new HBaseModel();
model.Init();
return model;
}
...
}
class SWTDocument extends Thread
{
...
public void loadFile(String dir)
{
m_SimpleHJCanvas.m_HModel.Flush();
m_SimpleHJCanvas.m_HModel.Read(dir);
m_SimpleHJCanvas.m_HView.FitWorld();
m_SimpleHJCanvas.m_HView.Update();
}
...
}
HBaseView
Multiple HBaseView
objects can be created, with one object created for each SimpleHJCanvas
as define in its parent HoopsSWTCanvas
constructor. In the java_simple_swt example, the SWTDocument
accesses the view via its instance of SimpleHJCanvas
to perform such operations as writing out to a file:
class HoopsSwtCanvas extends Canvas
{
...
public HoopsSwtCanvas(final Composite parent, final int style) {
super(parent, style);
...
m_HModel = createDefaultModel();
m_HView = createView(m_HModel);
...
}
protected HBaseView createView(final HBaseModel model) {
final SWIGTYPE_p_void swig = new SWIGTYPE_p_void(handle, true);
final HBaseView m_HView = new HBaseView(model, "", "opengl", "", swig);
m_HView.Init();
...
}
...
}
class SWTDocument extends Thread
{
...
class fileSaveAsItemListener implements SelectionListener
{
public void fileSaveAs()
{
String file;
String fullFileName;
FileDialog fd2 = new FileDialog(shell, SWT.SAVE);
while(true)
{
fullFileName = fd2.open();
file = fd2.getFileName();
if(fullFileName == null)
return;
if( file.matches(".*.hmf.*")||file.matches(".*.hsf.*"))
break;
else
{
MessageBox mb = new MessageBox(shell, SWT.CENTER);
mb.setMessage("Only .hmf or .hsf File formats are supported.");
mb.open();
}
}
m_SimpleHJCanvas.m_HModel.Write(fullFileName,m_SimpleHJCanvas.m_HView);
}
...
}
...
}
HBaseOperator
A default operator should be created during view initialization as it is done HoopsSwtCanvas::CreateView
. In the The java_simple_swt app, SWTDocument
sets the current operator by using its instance of HSimpleHJCanvas
:
class HoopsSwtCanvas extends Canvas
{
...
protected HBaseView createView(final HBaseModel model)
{
final SWIGTYPE_p_void swig = new SWIGTYPE_p_void(handle, true);
final HBaseView m_HView = new HBaseView(model, "", "opengl", "", swig);
m_HView.Init();
//Set the default operator
m_HOperator = new HOpCameraManipulate(m_HView);
m_HView.SetCurrentOperator((HBaseOperator)m_HOperator);
...
}
}
class SWTDocument extends Thread
{
...
class orbitCameraItemListener implements SelectionListener
{
public void orbitCamera()
{
m_SimpleHJCanvas.SetCurrentOperator(new HOpCameraManipulate(m_SimpleHJCanvas.m_HView));
}
...
}
...
}