The MarkupManager Class
Overview
The HMarkupManager
class allows developers to manage 2D and 3D markup data. In addition to allowing the user to set the coloring and weighting of markup data, the markup manager also allows the users to store and restore markup layers. The MarkupManager
class is used by the various HOpMarkup
operators.
Each HBaseView
object has a markup manager associated with it. The manager works by creating a separate segment for each markup layer, these layer segments reside in the top-level markup segment which is contained in the “Scene” segment of HBaseView
.
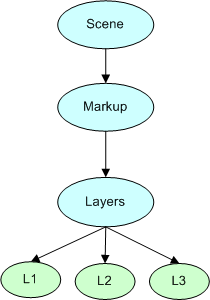
The diagram above shows the segment hierarchy that a markup manager would have created after three layers were created. We have a segment underneath the HBaseView
’s “scene” segment called “markup” which has a single child segment called “layers”. When you instruct the markup manager to create a new layer, it will reside as subsegment of the ‘layers’ segment. The markup manager names each segment l1, l2, l3 etc. according to the number of layers you create and within that segment, it stores a user option detailing the camera setting when the layer was created. This information is used to reset the camera when a layer is restored.
To create a new layer, you use HMarkupManager::OpenLayer
which will return the segment key of the layer. When you want to insert geometry into the MarkupLayer
, you simply open up the segment which represents the layer and insert the geometry into that segment tree. The HMarkupManager
class also provides methods which allow you to delete, copy, rename and find layers, as well as query camera, weight, and color information associated with each layer.
Let’s look at the example where we want to insert a 2D note into a markup layer:
// First let's get the Markup Manger associated with the HBaseView object
HMarkupManager *markupManager = m_pView->GetMarkupManager();
HC_KEY layerKey;
// From the markupManager we can get the key to the current active layer
// if there is not active layer then it will return a -1
if ((layerkey = markupManager->GetCurrentLayerKey()) == -1)
layerkey = markupManager->OpenLayer("");
// Now we open the layer and insert the text in its own segment
HC_Open_Segment_By_Key(layerKey);
HC_KEY note = HC_KOpen_Segment("note");
HC_Insert_Text(x,y,z, (char *)myText);
HC_Close_Segment();
HC_Close_Segment();
m_pView->Update();
The coloring of the geometry inserted in the markup layer can be set via the appropriate markup manager interfaces. The default coloring and weighting is red and 5.0 respectively:
HMarkupManager::SetMarkupColor(HPoint markup_color);
HMarkupManager::SetMarkupWeight(float weight)
It is recommended for each distinct markup type (rectangles, notes, freehand etc.), a new segment be created under the active markup layer. For setting attributes other than the coloring and weighting, you should set the appropriate attributes within that child segment. For example, most applications want the markup data to be drawn on top of the rest of the geometry in the scene so they set a HImUtility_draw_*_infront
callback in the child segment containing the markup data.
A markup layer can be activated by calling one of the HMarkupManager::OpenLayer
variants:
HMarkupManager::OpenLayer(const char *layername, bool setcamera);
HMarkupManager::OpenLayer(HC_KEY *layerkey, bool setcamera);
The setcamera
parameter allows you to specify whether or not to restore the camera that was used when the markup data was created. Since most markup data is 2D, this is on by default.
Anytime the camera is moved in the scene, the currently active markup layer is disabled.