HOOPS/MVO Technical Overview
Introduction
HOOPS/MVO is a C++ class library that sits on top of HOOPS 3D graphics system (HOOPS/3DGS). It has a model/view/operator architecture that encapsulates various HOOPS/3DGS data structures and concepts as well as offering a range of common application-level logic. HOOPS/MVO makes the key capabilities of HOOPS/3DGS more accessible, facilitating efficient development and rapid prototyping of full-featured, high-performance CAD/CAM/CAE applications.
The Model/View/Operator Architecture
The Model/View/Operator (MVO) architecture decouples graphical information from its presentation and manipulation. The model represents the data while the view corresponds to the presentation of this information. An operator can be a set of actions involving querying, creation, editing and manipulation of data. HOOPS/MVO implements this architecture via the following three main classes: HBaseModel
(model), HBaseView
(view) and HBaseOperator
(operator).
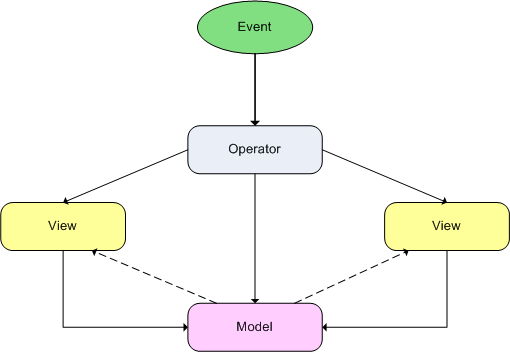
This diagram illustrates the relationship between the individual objects in the Model/View/Operator architecture.
HOOPS/MVO and HOOPS/3DGS
In HOOPS/3DGS, 3D objects are organized into segments containing geometry, attributes and subsegments. Typically, these objects are created and stored under the top-level segment known as the Include Library. In HOOPS/MVO, the HBaseModel
class encapsulates the models found in the Include Library. When application logic needs to build a scene, it would first create a new instance under a Driver segment, which corresponds to an instance of the driver. The HOOPS/MVO class HBaseView
stores a wide variety of information for a given driver instance, negotiating and managing data such as lights, overlay drawing, selection sets, and camera positioning. Once a 3D scene is displayed, the end-user may want to rotate the camera, highlight/select objects, and modify or delete various items. HOOPS/MVO facilitates these actions via the HBaseOperator
class and its derived classes.
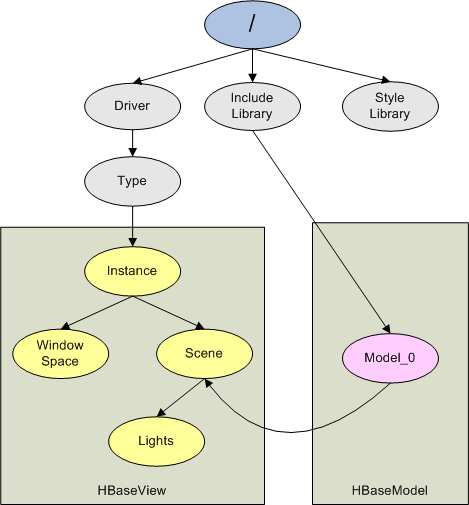
This diagram illustrates the HOOPS segment structure built by HOOPS/MVO Objects.
The HOOPS/MVO Classes
When building an application using the HOOPS/MVO framework, a single instance of the HDB class must be created and initialized during your application’s initialization phase. Once you have an HDB object, you can begin creating models, views and operators. HOOPS/MVO supports multiple models as well as multiple views.
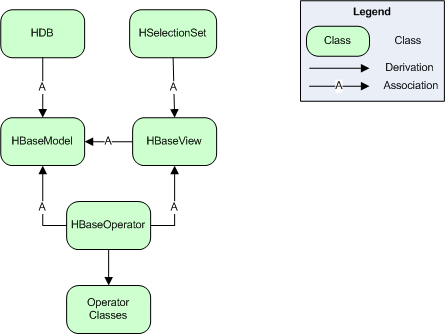
This diagram illustrates the relationship of the various HOOPS/MVO classes.
HBaseModel
In the HDB class, 3D graphics information is stored. To load information into the database, you can use HBaseModel
. This class includes support for loading a variety of file formats, and will store model information in the HOOPS/3DGS segment tree represented by the HOOPS/3DGS include library segment. Once the information is in the database, you can continue to use HBaseModel
to help manage your 3D objects. When you are ready to save your object information, HBaseModel
also includes support for exporting to a variety of formats.
HBaseView
The HBaseView
class manages the presentation of your models. Although an HBaseModel
object can have multiple HBaseView
instances, every HBaseView
is associated with exactly one HBaseModel
instance. In the HOOPS/3DGS database, HBaseView
encapsulates an instance of a driver segment. The driver instance defines a connection to a device such as OpenGL2 or DX11. When HBaseView
is initialized, it creates a default segment structure under the driver instance segment, which is used to manage interaction with HBaseModel
, lights for the scene, camera settings and window attributes such as temporary ‘rubberband’ graphics.
HBaseView
is a versatile class with a multitude of capabilities. It handles how and when model data is displayed. This includes render modes like Gouraud, Phong, and wireframe. HBaseView
also has the capability of managing level of detail based on framerate criteria. The responsiveness and effectiveness of your application can be directly associated with how you use HBaseView
capabilities.
HBaseOperator
In addition to displaying a 3D scene, most applications allow the end-user to interact with, or ‘operate on’ the model or view. In HOOPS/MVO, HBaseOperator
is the abstract base class that defines the interface for handling user input and operating on the model or view. The virtual methods defined in this class provide the basis for mapping user input to interaction logic. In this framework, an object derives from HBaseOperator
and then implements whatever virtual methods it chooses, to handle events such as mouse movements or key presses.
HOOPS/MVO not only presents the framework for handling user events in the HBaseOperator
class, but also provides a set of prebuilt classes derived from HBaseOperator
which handle a wide range of interactions. Prebuilt HOOPS/MVO operators are generally broken down into the following categories:
- Camera manipulation operators for panning, zooming or orbiting the camera. These include
HOpCameraOrbit
,HOpCameraZoom
, andHOpCameraPan
. - Object creation operators for creating and inserting objects in the database. These include
HOpCreateCircle
,HOpCreateCylinder
,HOpCreateSphere
andHOpCreateCuttingPlane
. - Object manipulation operators for translating and rotating objects. These include
HOpObjectRotate
,HOpObjectTranslate
andHOpObjectAnnotate
. - Selection operators for picking objects. These include
HOpSelectPolygon
andHOpSelectPolyline
. - Markup operators for creating notes in the scene. These include
HOpMarkupAnnotate
,HOpMarkupCircle
, andHOpMarkupMeasure
.
File I/O Architecture
The import and export of data is supported in HOOPS/MVO via the file I/O architecture.
At the core of this architecture is the HIOManager
class which handles all file input and
output modules. An instance of HIOManager
is created during the initialization of
the HDB class.

The figure shows how a file is loaded into the database.
When a file is imported into HOOPS/MVO, HBaseModel::Read
initiates the process. This function asks the HIOManager to find the correct input handler for the file. The search is primarily based on the comparison on file extensions. Once a compatible input handler is found, information is written in the database under a specified area of the segment tree. The exporting of data is analagous to its importation.

The figure shows how information from the database is exported to a file.
The HOOPS/MVO file I/O architecture comes with a variety of prebuilt input/output handlers that support many file formats. In addition to the prebuilt handlers, the file I/O architecture also supports custom input and output handlers. To construct a new input handler, create a new class derived from the HInputHandler
and implement the read methods along with the register method which notifies the HIOManager
of the type of files supported by the input handler. Optionally, the input handler can also implement interfaces for progress and error reporting as well as the creation of a log file. The creation of a new output handler is analagous to that of an input handler.
Selection
When a user is presented with a 3D scene, the natural inclination after changing the viewpoint is to select items in the scene. HOOPS/MVO provides the functionality to create, manage and manipulate selected objects. For a given view, HSelectionSet
manages the selected items. This class maintains a list of HOOPS geometry or segment ‘keys’ which have been identified as selected. When an item is added to the selection set, HSelectionSet
highlights the items based on the highlight mode. Currently, supported highlight modes include conditional style highlighting, quick moves reference highlighting and inverse transparency. HSelectionSet
can be easily extended to any number of sophisticated highlighting modes and styles that may better suit your application.
To use HSelectionSet
, create an instance of the object and then associate it with an instance of HBaseView
. When an operator receives a mouse or key event that initiates the selection process, it uses event information to create a set of criteria to pass to the HOOPS/3DGS compute selection function. This method returns a list of items which the operator then uses to update the HSelectionSet object.
In HOOPS/MVO, there are a several prebuilt operators that reflect the most commmon types of selection. The first is HOpSelectArea
whose selection criteria is a rectangular area. During the left mouse button down event, this operator records the mouse position as the first point in the rectangle. As the mouse pointer is dragged over the view, a rubberbanded rectangle is drawn over the scene allowing the user to visualize the selection area. The operator records the second and final point of the selection area during the left mouse button up event. Objects that intersect the rectangle are identified as selected items.
Another commonly used selection operator is HOpSelectAperture
which performs a hit test on a user-selected point. During the left mouse button down event, the operator captures the position of the pointer. If a piece of geometry is within the “selection proximity” of that location, it is considered for selection.
HOOPS/MVO also has two other prebuilt selection operators. HOpSelectPolygon
performs selection based on a user-defined polygon while HOpSelectPolyline
performs selection based on items that intersect a multi-segment line.

This diagram shows how selection works in HOOPS/MVO.
Animation
Displaying high-quality 3D models in a user-navigable scene can be an effective visualization method. However, adding movement through animation can help convey a lot more information. For example, animations that shows pistons turning a crankshaft or the movement of a robotic arm in an assembly line can disburse more information than a static scene. HOOPS/MVO provides the ability to author animations like these through a set of behavior classes.
In HOOPS/MVO, the HBhvBehaviorManager class manages all the behavior classes for a given instance of HBaseModel
. You can create and modify an animation with methods in HBhvBehaviorManager
. For a given animation, any number of different key frames can be added to change the position, orientation or attribute of the target object. By combining multiple behaviors for different objects and playing them simultaneously, complex animations are created. Furthermore, you can construct a highly interactive scene by using actions, like a mouse event, to trigger behaviors for specific objects.
Once an animation is created, HOOPS/MVO provides controls for playback like play, pause and rewind like a movie. Animations can be saved out into an XML file (.bhv) and later loaded in again. Animations can also be exported into a AVI file to be viewed on other players and over the web.
Utilizing the Framework
Depending on the nature of your application, HOOPS/MVO can serve a number of purposes. First, it can be used as the foundation of your application. Because HOOPS/MVO takes care of common, low-level details related to managing data as well as routing user events through the proper channels, designers and programmers can focus on implementing their market-specific feature requirements.
In some cases, an existing application may already have its own framework. Because HOOPS/MVO was built specifically as a framework for HOOPS/3DGS, it can serve as sandbox for rapidly prototyping new and complex features before incorporating them into your application. Because new features can be designed, implemented and tested in a relatively short amount of time in this environment, more time can be spent on fine-tuning performance and quality.
With each new release of HOOPS/3DGS, an increasing number of sophisticated capabilities are added. Application developers will find that HOOPS/MVO acts as a valuable repository for reference implementations, not only for basic functionality like selection and camera manipulation, but also for more complex capabilities such as clash detection and animation.