Reading Parasolid Files
To read a Parasolid file, call HP_Read_Xmt_File
. This parses the specified Parasolid file, populates the Parasolid modeling kernel with its contents, and then maps the model to a corresponding HOOPS segment tree hierarchy that includes all geometry and attributes. Example:
// an application specific read function; HSolidModel is derived
// from the HBaseModel MVO class
int HSolidModel::Read(const char * FileName)
{
unsigned int i;
char extension[120];
bool success = true;
extension[0]='\0';
HC_Parse_String(FileName, ".", -1, extension); // make it lower case
for (i = 0; i < strlen(extension); i++)
extension[i] = (char) tolower(extension[i]); // read the file into the model object's model segment
HC_Open_Segment_By_Key(m_ModelKey);
// read each file into its own subsegment of the main model segment so that
// we can easily apply transformations to different parts that might be read
// into the same model object; currently not supported in MVO, but could be
// added in the future. This is also required so that when we do segment
// level deletion later on, we don't delete the model_keysegment
HC_Open_Segment("");
if (streq(extension,"xmt_txt") || streq(extension,"x_t"))
{
PK_PART_receive_o_t options;
PK_ERROR_code_t result;
PK_PART_receive_o_m(options);
options.transmit_format = PK_transmit_format_text_c; // read an XT file
result = HP_Read_Xmt_File(FileName, GetPartition(), 0, 0, &options);
if (result 0)
success = false;
} else
success = false;
HC_Close_Segment();
HC_Close_Segment();
return success;
}
Controlling Tessellation
You can control facet tessellation as an import argument. In HP_Read_Xmt_File
, use the face_tessellation_level
parameter to specify tessellation. Valid options are as follows:
- “Lowest”
- “Low”
- “Medium”
- “High”
- “Highest”
- “Custom”
“Custom” is the default, which means the developer is responsible for setting all the tessellation options himself. You can also set tessellation options in HP_Render_Entity
and HP_Render_Entities
. Examples of the effect of the tessellation are shown below:
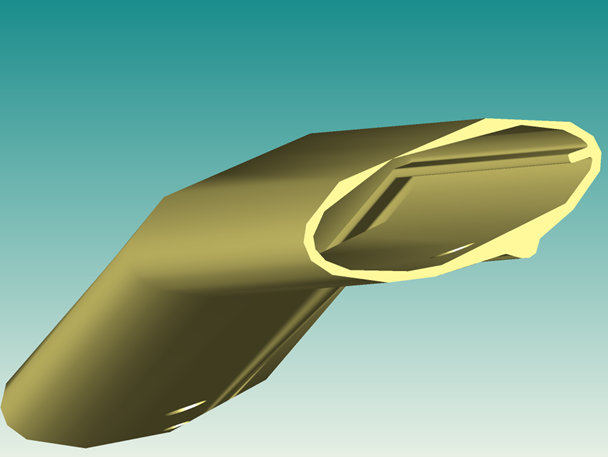
“Custom” tessellation
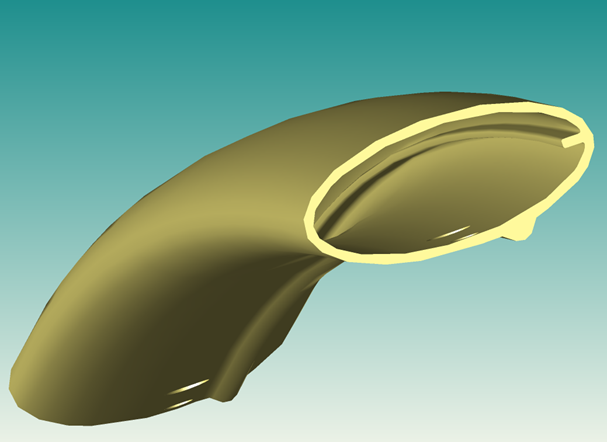
“Medium” tessellation
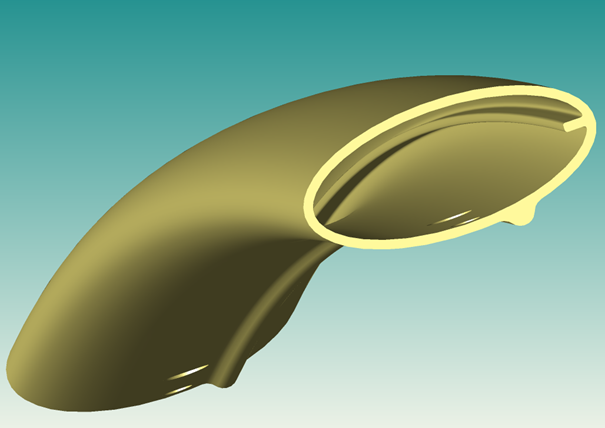
“Highest” tessellation