HOOPS Communicator Access
HOOPS Communicator is Tech Soft 3D’s web application framework. It gives you the ability to load and stream model files over a network directly into a 3D application window. Using the HOOPS Communicator sprocket, you can read all the file formats supported by HOOPS Visualize or HOOPS Exchange from a remote server.
The Communicator sprocket is provided as a way for HOOPS Visualize to render and interact with model files served from a HOOPS Communicator server. The HOOPS Communicator Stream Cache system enables very large files to be loaded via streaming which would otherwise be infeasible to view.
In order to use the Communicator sprocket, you must be using the same version as the HOOPS Communicator server you’re streaming files from. Please see the compatibility chart in the release notes to find the proper version.
This page assumes basic familiarity with HOOPS Communicator. To read more, see the Communicator documentation.
Required DLLs
The sprocket depends on a set of DLLs that are not normally required for %HPS to work. When you are ready to distribute your HOOPS Communicator-enabled application, be sure to include these additional DLLs found in the %HPS package:
- cc_exchange_lib.dll
- hc_access.dll
- libcrypto-3-x64.dll
- libssl-3-x64.dll
- v8.dll
- v8_libbase.dll
- v8_libplatform.dll
- websockets.dll
Loading a Model Over a Network
There are two ways in which HOOPS Communicator data can be loaded: over a network (Stream Cache model, .sc), or locally (.scs files) - see below. Loading a stream cache model is fundamentally different from loading a file from the file system because the file is transferred over the network using a HOOPS Communicator server. As such, you will need an accessible HOOPS Communicator server in addition to the client application. The general steps needed to get the system running are as follows:
- Set up the environment. To use the Stream Cache server, it will need to be running and accessible. Know the IP address and port it is using.
- Include the HCA libraries in your project. The Visualize-Communicator integration library must be included as a dependency. C++ users will need to link to the hps_sprk_hca.lib. C# users will need to add a reference to the hps_sprk_hca.dll. These files are located in the bin directory of your HOOPS Visualize package.
- Include the header file sprk_hca.h in your source (C++ only).
- Include the Javascript file hca_access.js in your path.
All classes that implement the HOOPS Communicator sprocket logic are members of the HPS::HCA
namespace. The process of loading a file using this sprocket is similar to the process for other HPS sprockets:
- Populate a
HPS::HCA::NetworkImportOptionsKit
with data related to your session. - Import the file using
HPS::HCA::File::Import
. - Wait on the notifier so you know when the model is finished loading.
Example of loading a stream cache model:
HPS.HCA.NetworkImportOptionsKit importOptionsKit;
// be sure to include the port number where applicable
importOptionsKit.SetNetworkPath("http://127.0.0.1:11182");
importOptionsKit.SetLimitMiB(1024);
importOptionsKit.SetTarget(myModel);
importOptionsKit.SetJavascriptPath(jsPath); // path to hc_access.js - this file is distributed with Communicator
importOptionsKit.SetView(defaultView); // sets the HPS.View to use by default
HPS.HCA.ImportNotifier notifier = new HPS.HCA.ImportNotifier();
HPS.HCA.File.Import(filename, importOptionsKit, notifier);
notifier.Wait();
HPS.IOResult result = notifier.Status();
if (result == HPS.IOResult.Success)
success = true;
The code above will load a model from a HOOPS Communicator server (in this case, a server running on localhost). Notice the use of notifier.Wait()
. When loading a model over the network, calling Wait()
will wait only until the import starts streaming data, and will return control to the user while geometry is still being added to the scene. This is because an import over the network is used for very large files, which might never completely load (for example because a memory limit has been set).
The HOOPS Communicator Stream Cache server serves a portion of a model’s geometry based on camera position and geometry size. This is ideal for very large models, which may be too large to load all at once into memory. Using the streaming server enables a Visualize application to stream data in and out of the Visualize scene graph.
Loading a Local Model
Stream cache models can be made available as SCS files, which are a locally-available alternative to the stream cache system. Loading a .scs file does not require a server, however, these files cannot be streamed. The entire model is loaded at once, therefore, you will have to more carefully consider the resources available in the execution environment. The process is similar to what is described above, however, instead of using a NetworkImportOptionsKit
, you need to use a HPS::HCA::ImportOptionsKit
.
HPS.HCA.ImportOptionsKit importOptionsKit = new HPS.HCA.ImportOptionsKit();
// be sure to include the port number where applicable
importOptionsKit.SetTarget(myModel);
importOptionsKit.SetJavascriptPath(jsPath); // path to hc_access.js - this file is distributed with Communicator
importOptionsKit.SetView(defaultView); // sets the HPS.View to use by default
HPS.HCA.ImportNotifier notifier = new HPS.HCA.ImportNotifier();
HPS.HCA.File.Import(filename, importOptionsKit, notifier);
notifier.Wait();
HPS.IOResult result = notifier.Status();
if (result == HPS.IOResult.Success)
success = true;
The code above will load a HOOPS Communicator model from a local source. Notice the use of notifier.Wait()
. When loading a model in this way, calling Wait()
will wait for the whole file to be loaded, just like it does for other local file types.
Getting a Reference to the Model
After the model is loaded, you most likely will want a reference to it so that you can interact with it. To do this, simply call HCA::ImportNotifier::GetTarget()
, which provides the HPS::Model
. From there, you can construct the HPS::HCA::Model
.
Model hpsModel = notifier.GetTarget();
HCA.Model hcaModel = new HCA.Model(hpsModel);
HOOPS Communicator models are comprised of a collection of nodes. All model information is addressable via node ID. To query your newly loaded model, start with the HCA::Model
and drill down using RequestNodeProperties
and RetrieveNodeProperties
. These functions are designed to work asynchronously, so you may have to account for network latency.
Int64 myNodeId;
UInt32 myRequestId;
bool myValid;
hcaModel.ShowRootNode(out myNodeId);
// get the properties for the root node ID
hcaModel.RequestNodeProperties(myNodeId, out myRequestId);
string[] out_keys;
string[] out_values;
// since the previous function is asynchronous, we may have to wait
hcaModel.RetrieveNodeProperties(myRequestId, 1000, out myNodeId, out myValid, out out_keys, out out_values);
So far we’ve only been working with the root node. To get other nodes, use ShowNodeChildren
to recursively walk the node tree:
Int64[] out_ids;
hcaModel.ShowNodeChildren(myNodeId, out out_ids);
For a complete example application, see this subsection and also the mfc_hca_sandbox application, which is distributed with the HOOPS Visualize solution.
Supported File Types
Before Communicator can serve models, it is necessary to convert them to its own file formats. These native formats include SC, SCS, and SCZ. They are optimized for streaming, and are tesselated, unlike CAD file formats which are usually B-rep. This conversion can take place ahead of time, or on demand. The three types of files are described below:
SCS files do not require a Communicator server to access. They can be loaded directly via the HOOPS Communicator Sprocket. In this case, the entire SCS file will be loaded into Visualize. This is possible only for models which are small enough to fit in system memory of the client application.
SC files are streamed over the network with a server, and are typically broken into multiple files behind the scenes.
An SCZ file is a single file version of the SC format. Details on all the file types can be found here.
Other types of models undergo a conversion process before the data is streamed to the client. In the general case, the HOOPS Communicator server will use HOOPS Exchange to convert a model from its native format into the Stream Cache format.
The details are all described in the HOOPS Communicator documentation.
Highlighting
Behind the scenes, loading HOOPS Communicator models is a fundamentally different process than loading traditional models. It follows that certain operations need to be done in a different way. Highlighting, unhighlighting, isolate, hide, and show are all examples of this. HCA::Model
has its own collection of these function variants. When you need to use them, simply make sure you use those variants instead of the normal HPS::HighlightControl
or HPS::ComponentPath
versions.
MFC HCA Sandbox
The MFC HCA Sandbox is an application provided with the HPS package which intends to demonstrate how the HOOPS Communicator process works. The project source is provided and can be used as a starting point for your own application. Please see the mfc_hca_sandbox project in your package.
When you first start the sandbox, you’ll notice two buttons on the toolbar called “Connect” and “Options”. In order to load a stream cache model, you need to be running (or have access to) a HOOPS Communicator server. It’s also important that you fill out the options dialog so its values match your environment.
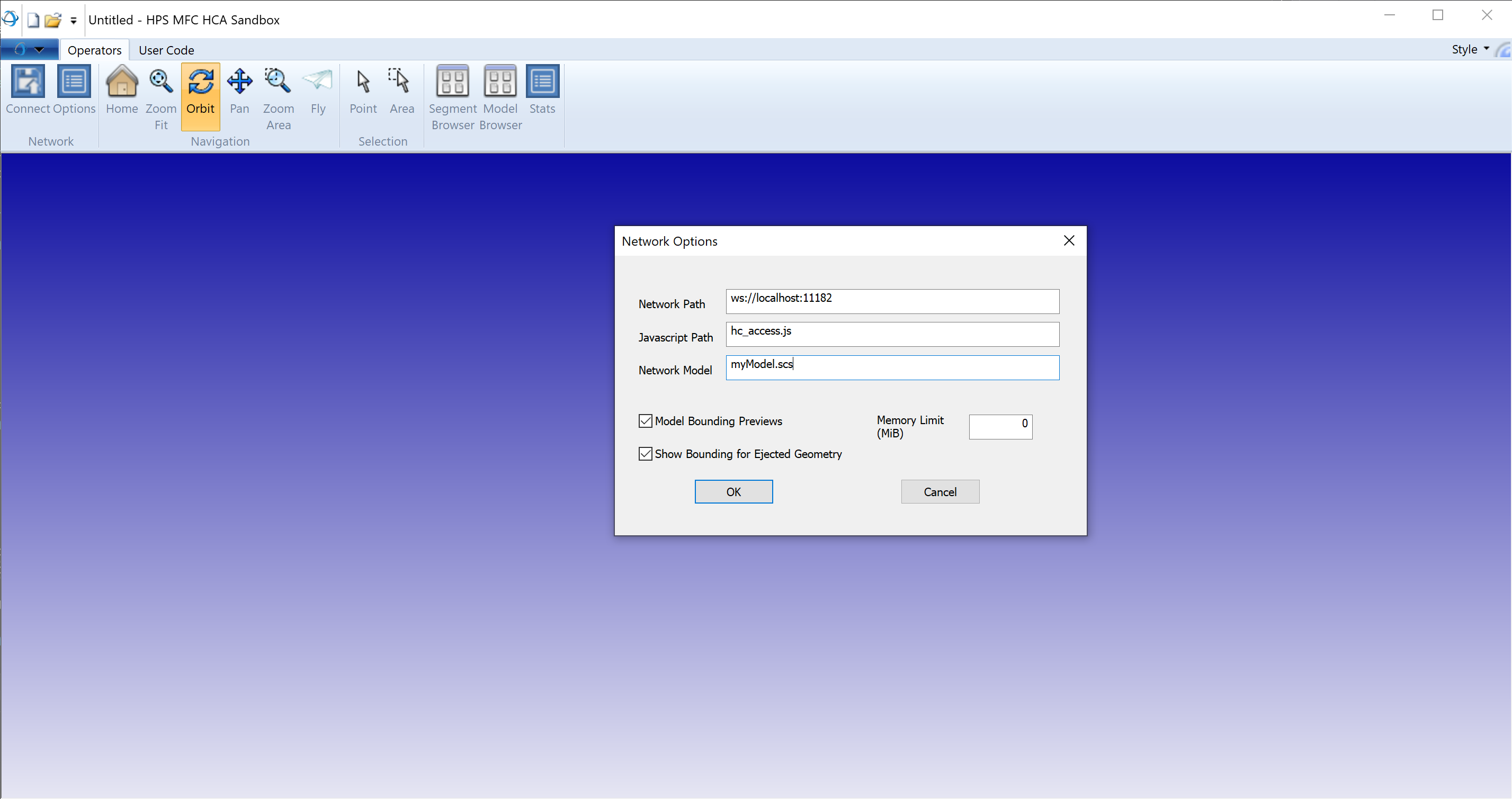
The network path will be the URL and port for your HOOPS Communicator server. The Javascript path is where the hc_access.js file is located (it is provided as part of HOOPS Communicator). The network model is the name of the file you want to load. This file needs to be in (or relative to) one of the directories listed in HOOPS Communicator’s Config.js
file (you can find the setting under the “modelDirs” value). The memory limit corresponds to the value set in HPS::HCA::NetworkImportOptionsKit::SetLimitMiB()
.
Loading a .scs file doesn’t require setting any network options, because .scs files are loaded from a local source.
Whichever way you load the model, you can interact with it like any other model:
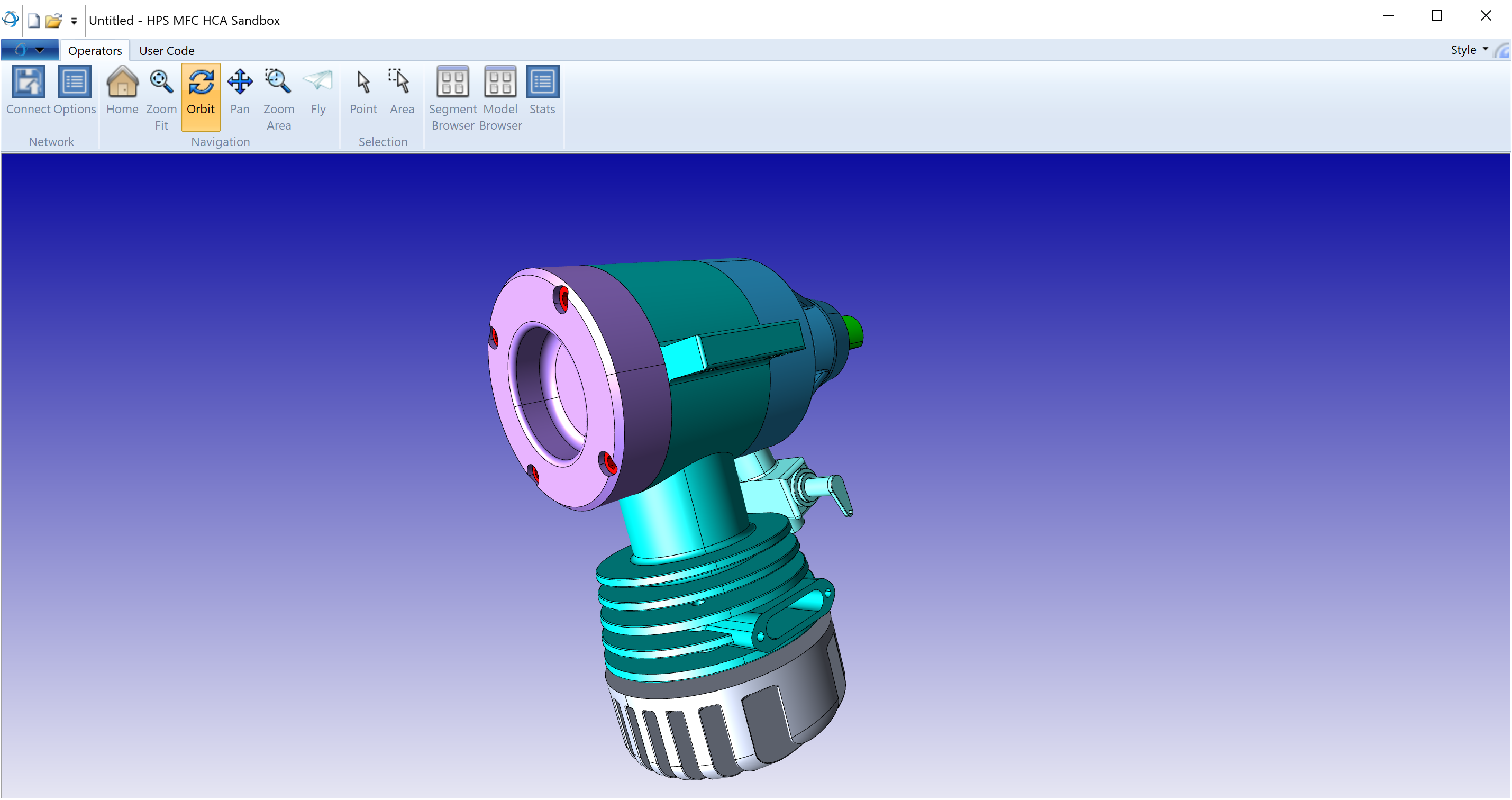
You will also notice the User Code tab, which is available to execute your own code in the context of the MFC HCA Sandbox.
Once your model is loaded, the model and segment browsers will be populated. Activate them by clicking on their respective icons in the ribbon bar. These browsers have a tree control with nodes that correspond to each part of the model. The segment browser represents the %HPS scene graph - the segments and attributes which make up the data you see on screen. The data in the model browser corresponds to the structure of the model as loaded from its source format. Clicking on any node in either browser will highlight the corresponding part of the model in orange. Similarly, clicking on the model will highlight that part in each browser (to do this, make sure the “Point” selection operator is active).
Finally, there is a statistics window which shows various statistics about the model and how it is rendered, such as number of triangles, memory usage, and the GPU.
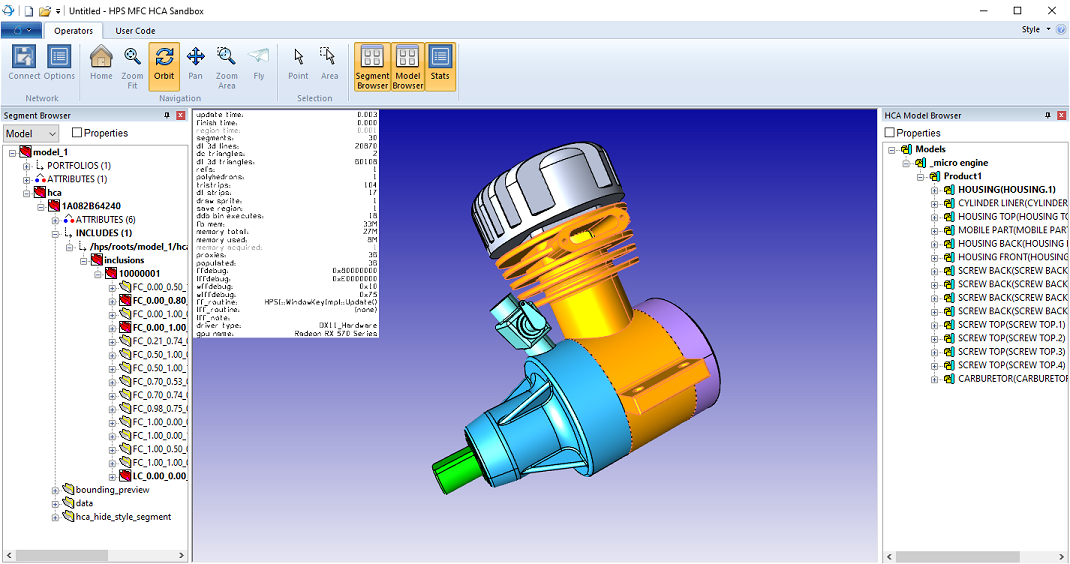
Limitations
Loading multiple files into a scene is not possible at this time. If you need to load a second model, you need to unload the first model by deleting your model segment using HPS::Model::Delete
.
Models generally can’t be modified and saved directly back to the server. If you need to modify a HOOPS Communicator model, we recommend you make your changes, export the model, and get it back to the server independently.
Exchange views are not currently supported.