Clip Regions
A clip region is a 2D polygonal region defined in a segment in which geometry is either clipped or masked. The clip region applies to all geometry within and beneath the segment where the clip region is defined. Clip regions are polygonal, therefore, they are subject to the same limitations as visual polygons.
Clip regions are enabled using the HPS::DrawingAttributeControl
. You must define the polygon and specify the clip space you are working with. Possible options are world space, window space, or object space.
Then, use either the HPS::Drawing::ClipOperation::Keep
or HPS::Drawing::ClipOperation::Remove
enums to specify whether the operation will clip or mask geometry. Keep
means that the outside of the clip region will be clipped, while Remove
means that the inside of the clip region will be clipped.
In this sample, a triangular clip region is set on the sphere segment:
mySegmentKey.InsertSphere(HPS::Point(0, 0, 0), 0.25f);
mySegmentKey.GetVisibilityControl().SetEdges(true);
HPS::PointArray pointArray;
pointArray.push_back(HPS::Point(10, 10, 0));
pointArray.push_back(HPS::Point(-10, 10, 0));
pointArray.push_back(HPS::Point(-10, -10, 0));
mySegmentKey.GetDrawingAttributeControl().SetClipRegion(
pointArray, HPS::Drawing::ClipSpace::World, HPS::Drawing::ClipOperation::Keep);
mySegmentKey.InsertSphere(new HPS.Point(0, 0, 0), 2.0f);
mySegmentKey.GetVisibilityControl().SetEdges(true);
HPS.Point[] pointArray = new Point[3];
pointArray[0] = new HPS.Point(10, 10, 0);
pointArray[1] = new HPS.Point(-10, 10, 0);
pointArray[2] = new HPS.Point(-10, -10, 0);
mySegmentKey.GetDrawingAttributeControl().SetClipRegion(
pointArray, HPS.Drawing.ClipSpace.World, HPS.Drawing.ClipOperation.Keep);
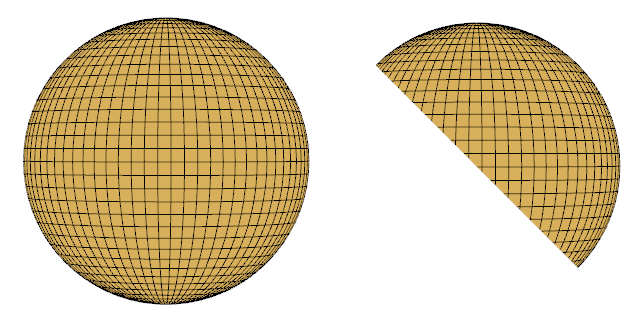
A sphere before clipping (left) and after being clipped by a triangular polygon
Clip regions are implemented as attributes, and therefore inherit down the segment tree like other attributes. However, when you set a clip region on a segment which also inherits a different clip region from a parent, the clip space is limited to the overlap of the clip regions.
Complex Clip Regions
A complex polygonal clip region is a clip region defined by more than one loop. As mentioned in the previous section, loops are closed lines, possibly self-intersecting, defined by a vector of points.
In the example below, there are two loops. The first loop, drawn in red, is defined by 4 points: P0 to P3. The second loop, drawn in green, is defined by 4 points: P4 to P7. When you define a complex polygonal clip region and use HPS::Drawing::ClipOperation::Keep
, the area which is actually clipped is the logical AND of the loops. Using HPS::Drawing::ClipOperation::Remove
, the area which is clipped is the logical XOR or the loops. For example, in the image below, the blue area is what would be clipped during a Remove
, while the other areas would be unaffected.
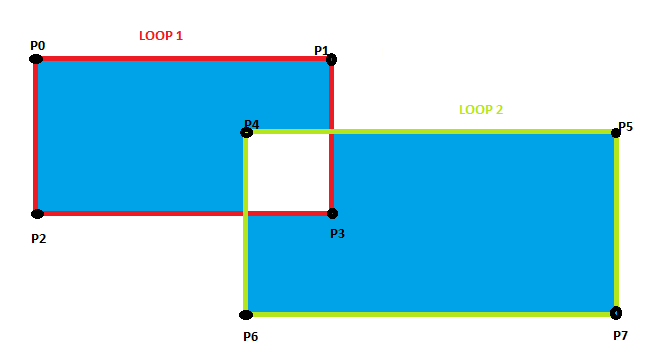
Complex clip region - the blue area is clipped away during a ``Remove`` operation.
Complex clip regions can be set up in the following way:
HPS::PointArrayArray loops;
HPS::PointArray loop1;
loop1.push_back(HPS::Point(0, 0, 0));
loop1.push_back(HPS::Point(1, 0, 0));
loop1.push_back(HPS::Point(1, 1, 0));
loop1.push_back(HPS::Point(0, 1, 0));
HPS::PointArray loop2;
loop2.push_back(HPS::Point(0.5f, 0, 0));
loop2.push_back(HPS::Point(1.5f, 0, 0));
loop2.push_back(HPS::Point(1.5f, 1, 0));
loop2.push_back(HPS::Point(0.5f, 1, 0));
loops.push_back(loop1);
loops.push_back(loop2);
mySegmentKey.GetDrawingAttributeControl().SetClipRegion(
loops, HPS::Drawing::ClipSpace::World, HPS::Drawing::ClipOperation::Keep);
HPS.Point[] loop1 = new HPS.Point[] { new HPS.Point(0, 0, 0),
new HPS.Point(1, 0, 0),
new HPS.Point(1, 1, 0),
new HPS.Point(0, 1, 0) };
HPS.Point[] loop2 = new HPS.Point[] { new HPS.Point(0.25f, 0.25f, 0),
new HPS.Point(-0.75f, 0.25f, 0),
new HPS.Point(-0.75f, -0.75f, 0),
new HPS.Point(0.25f, -0.75f, 0) };
HPS.Point[][] loops = new HPS.Point[][] { loop1, loop2 };
mySegmentKey.GetDrawingAttributeControl().SetClipRegion(loops, HPS.Drawing.ClipSpace.World, HPS.Drawing.ClipOperation.Remove);