Curves
Curves are a type of primitive geometry in Visualize. They include circles, circular arcs, ellipses, elliptical arcs, and NURBS curves. Circles, circular wedges, and ellipses are facetted objects whereas the others are not. This differentiation is important because facetted objects have edges while non-facetted objects are made of lines. This section does not cover NURBS - see section 2.11 for that discussion.
The HPS::CurveAttributeControl
is a segment-level control that groups attributes related to rendering curved lines and edges. Examples of attributes that can be controlled are tessellation, maximum angles between adjacent line segments, and whether the tessellation of curves will be view independent. See the reference manual page on this control for more information about these settings. Note that all of the example screenshots on this page are shown with face visibility turned off for clarity. If you would like to show geometry in this way, you should create the geometry in a segment that has disabled face visibility.
Circles
A circle is a type of geometric primitive in Visualize. Note that although all curves, including circles, are 2D objects, all exist in 3D space. Visualize has no exclusive concept of 2D. To draw a circle, simple provide the center point, normal vector, and radius:
HPS::CircleKit circleKit;
// setting the attributes on the kit
circleKit.SetCenter(Point(0, 0, 0));
circleKit.SetNormal(Vector(0, 0, 1));
circleKit.SetRadius(0.75f);
// inserting circle into the database
HPS::CircleKey circleKey = mySegmentKey.InsertCircle(circleKit);
HPS.CircleKit circleKit = new HPS.CircleKit();
// setting the attributes on the kit
circleKit.SetCenter(new Point(0, 0, 0));
circleKit.SetNormal(new Vector(0, 0, 1));
circleKit.SetRadius(0.75f);
// inserting circle into the database
HPS.CircleKey circleKey = mySegmentKey.InsertCircle(circleKit);
The normal vector sets the circle’s orientation in space. In order for it to appear circular, the normal must point directly at the camera.
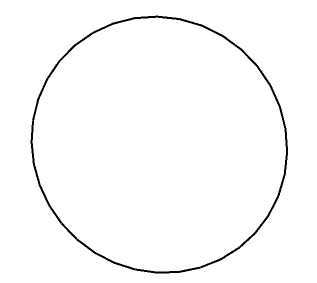
A simple circle
Circular Arcs
Circular arcs are made using the circular curve over three distinct points along the circumference of a circle. If a circle cannot be formed using the three supplied points, the geometry is not inserted. As circular arcs are not facetted, its line attributes are controlled by the LineAttributeControl
.
mySegmentKey.InsertCircularArc(Point(-0.5f, -0.5f, 0), Point(0, 0.75f, 0), Point(0.25f, 0.5f, 0));
mySegmentKey.InsertCircularArc(new Point(-0.5f, -0.5f, 0), new Point(0, 0.75f, 0), new Point(0.25f, 0.5f, 0));
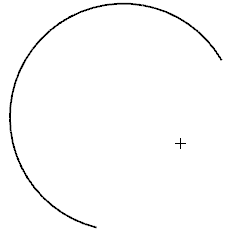
A circular arc. The marker indicates the origin.
Circular Wedges
Circular wedges are similar to circular arcs, except the endpoints are drawn to the center of the “circle”. They are defined analogously as three points along a circumference. Like other facetted geometry, the circular wedge can be filled with a color.
mySegmentKey.InsertCircularWedge(Point(-0.5f, -0.5f, 0), Point(0, 0.75f, 0), Point(0.25f, 0.5f, 0));
mySegmentKey.InsertCircularWedge(new Point(-0.5f, -0.5f, 0), new Point(0, 0.75f, 0), new Point(0.25f, 0.5f, 0));
A circular wedge
Ellipses
In the real world, a circle is a type of ellipse, but in Visualize they are unrelated from a class inheritance perspective. To insert an ellipse, all that is needed is the center point, the major axis, and the minor axis. The ellipse is considered to be faceted geometry.
HPS::EllipseKit ellipseKit;
ellipseKit.SetCenter(Point(0, 0, 0));
ellipseKit.SetMajor(Point(0.75f, 0, 0)); // major axis
ellipseKit.SetMinor(Point(0, 0.5f, 0)); // minor axis
mySegmentKey.InsertEllipse(ellipseKit);
HPS.EllipseKit ellipseKit = new EllipseKit();
ellipseKit.SetCenter(new Point(0, 0, 0));
ellipseKit.SetMajor(new Point(0.75f, 0, 0)); // major axis
ellipseKit.SetMinor(new Point(0, 0.5f, 0)); // minor axis
mySegmentKey.InsertEllipse(ellipseKit);
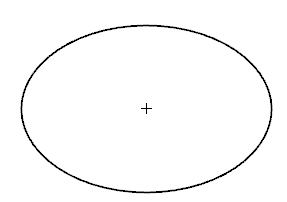
An ellipse
Elliptical Arc
An elliptical arc will make an arc out of a portion of an ellipse. The portion that is to be drawn is specified using start and end parameters. These parameters indicate the normalized parametric angle along the ellipse’s perimeter, in the direction from the major to minor axis, where the arc begins and ends. For example, use the code below to create an arc out of about 75% of an ellipse:
HPS::EllipticalArcKit ellipticalArcKit;
ellipticalArcKit.SetCenter(Point(0, 0, 0));
ellipticalArcKit.SetMajor(Point(0.75f, 0, 0)); // major axis
ellipticalArcKit.SetMinor(Point(0, 0.5f, 0)); // minor axis
ellipticalArcKit.SetStart(0.0f);
ellipticalArcKit.SetEnd(0.75f);
mySegmentKey.InsertEllipticalArc(ellipticalArcKit);
HPS.EllipticalArcKit ellipticalArcKit = new EllipticalArcKit();
ellipticalArcKit.SetCenter(new Point(0, 0, 0));
ellipticalArcKit.SetMajor(new Point(0.75f, 0, 0)); // major axis
ellipticalArcKit.SetMinor(new Point(0, 0.5f, 0)); // minor axis
ellipticalArcKit.SetStart(0.0f);
ellipticalArcKit.SetEnd(0.75f);
mySegmentKey.InsertEllipticalArc(ellipticalArcKit);
The start of the ellipse is the point that locates the major axis, and the retained portion is swept over the ellipse in a counter-clockwise fashion.
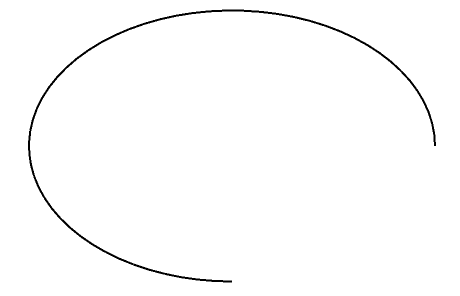
An elliptical arc