Animation System
This book describes how to use the HOOPS Luminate animation system to play animations at runtime.
Basic Animation
The Animation Clip
The base of the animation system is the animation clip. Its purpose is to store an animation timeline containing key frames. Key frames are animation data associated to a timestamp in the timeline.
In HOOPS Luminate, the animation data is contained in the RED::AnimationData
object. It stores the following transformations:
- Position as a 3 coordinates vector
- Rotation as a 4 coordinates quaternion
- Scale as a 3 coordinates vector
The RED::AnimationClip
object provides all the functions to access the raw key frames or to interpolate between them:
RED::AnimationClip::GetKeyTime
RED::AnimationClip::GetKeyData
RED::AnimationClip::Interpolate
In a single animation clip, HOOPS Luminate allows to define one or more timelines, they are called channels. All the clip methods are related to one channel.
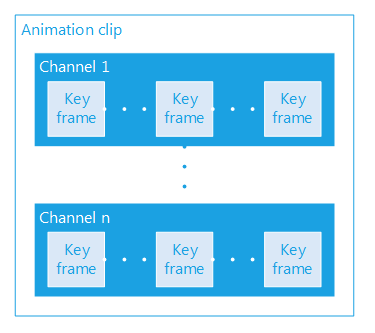
The animation clip contains multiple channels
Note
All the channels should have the same duration, the first keys starting at time 0 and the last ones at the same time (duration). However, multiple channels do not necessarily need to have the same number of keys.
The Animation Clip Controller
In the API, the clip is handled by an animation clip controller. The RED::IAnimationClipController
interface provides several functions to control an animation:
RED::IAnimationClipController::Play
RED::IAnimationClipController::Pause
RED::IAnimationClipController::Stop
RED::IAnimationClipController::Rewind
RED::IAnimationClipController::JumpToTime
The controller also handle the loop mode and the play speed with the RED::IAnimationClipController::SetLoop
and RED::IAnimationClipController::SetSpeed
functions.
Note
A normal speed is 1, a speed of 0 will pause the animation, a negative speed will reverse the animation.
Finally, the RED::IAnimationClipController::Update
function is meant to be called at each frame of the application runtime. This is the function that will correctly move the animation according to the parameters you set in the controller.
Once the controller is correctly initialized, at each frame, the user have to update it and get the resulting animation data using the RED::IAnimationClipController::GetChannelOutput method. This method returns a RED::AnimationData resulting of the animation play. The transformations can then be applied to any HOOPS Luminate object (for instance a RED::ITransformShape matrix or a RED::IViewpoint position).
Like other HOOPS Luminate objects, the clip controller is created by the factory with the RED::Factory::CreateAnimationClipController
function and deleted with RED::Factory::DeleteInstance
.
Creating an Animation Clip Controller
An animation clip controller allows to play an animation clip. It is created thanks to the RED::Factory
.
// Create an animation clip controller to control a RED::AnimationClip.
RED_RC rc;
RED::Object* animController = RED::Factory::CreateAnimationClipController( *resmgr, clip, rc );
RC_TEST( rc );
RED::IAnimationClipController* ianimController = animController->As< RED::IAnimationClipController >();
The controller will allow to play the animation clip by calling its update function at each frame.
The destruction of the animation clip controller can be done like this:
// Delete the animation clip controller.
RC_TEST( RED::Factory::DeleteInstance( animController, iresmgr->GetState() ) );
Skeletal Animation
Now that we have put in place the keyframe layer of the animation system, the next chapter will describes the skeletal animation API built upon it: Skeletal Animation. After this chapter, you will be able to animate a skinned mesh by playing one or more skeletal animations and mixing them in real-time.