Setup a Sun Shadowing Workflow
The second step of our processing is to setup a sun shadowing calculation workflow. In HOOPS Luminate, all light sources are dynamic, so all shadow maps are recomputed each frame to account for any change that could have occurred in the source model. This may have a non neglectable cost if we render a huge CAD assembly model on limited graphics hardware.
Sun Shadowing Rendering Pipeline
The REDCADGraphicsBuilder class setups the following rendering pipeline:
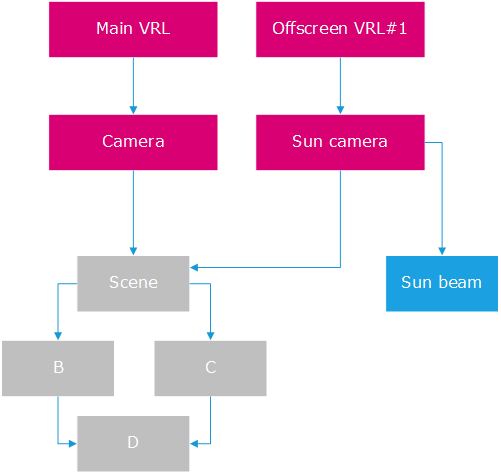
The sun shadow rendering pipeline.
The global assembly is linked to another camera (whose position don’t matter) to which a sun beam is being added.
Sun Beam Setup
In order to remain real-time, we setup a sun beam light that uses shadow mapping as main technique to produce shadows. The sun beam is setup according to the scene bounding sphere informations computed earlier, as detailed below:
RED::ILightShape* ibeam = _sun_beam->As< RED::ILightShape >();
float att_values[7] = { 1.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 };
RED::Vector3 lpos, ldir, ltop;
lpos = _bcenter + _sun_dir * 2.0 * _bradius;
ldir = -_sun_dir;
ltop = RED::Vector3::XAXIS;
if( fabs( ltop.Dot( ldir ) ) < RED_TOL2 )
ltop = RED::Vector3::YAXIS;
ltop = ltop.Cross2( ldir );
RC_TEST( ibeam->SetBeamLight( RED::ATN_NONE, att_values,
lpos, ldir, ltop,
RED::Color::WHITE, RED::Color::WHITE,
(float)_bradius, (float)_bradius + 0.1f,
iresmgr->GetState() ) );
RC_TEST( ibeam->SetShadowMapping( true, iresmgr->GetState() ) );
RC_TEST( ibeam->SetShadowMapResolution( SHADOW_MAP_SIZE, iresmgr->GetState() ) );
RC_TEST( ibeam->SetShadowMapCustomRange( (float)_bradius, 3.0f * (float)_bradius, iresmgr->GetState() ) );
RC_TEST( ibeam->SetShadowMapDirectAccess( true, iresmgr->GetState() ) );
RC_TEST( ibeam->SetShadowMapPolygonOffset( 0.75f * (float)SHADOW_MAP_BLUR, 5.0f, iresmgr->GetState() ) );
The scene bounding informations are required to concentrate the effect of the shadow map onto meaningful regions of our assembly.
Using a VRL Toggle
We enable the sun VRL to render the sun shadows and we disable it once our shadowing calculations have been made. This way we update the sun shadow map on demand, and we don’t have any rendering time to pay to use our last update.
Accessing the Sun Shadow Map
The access to the sun shadow map uses the shadow map direct access detailed in RED::ILightShape::SetShadowMapDirectAccess
.