Loading a Simple Landscape
In this first ART tutorial, we’ll see how we can load a pre-packaged landscape, display it and navigate within it. Here’s an image of the loaded terrain below:
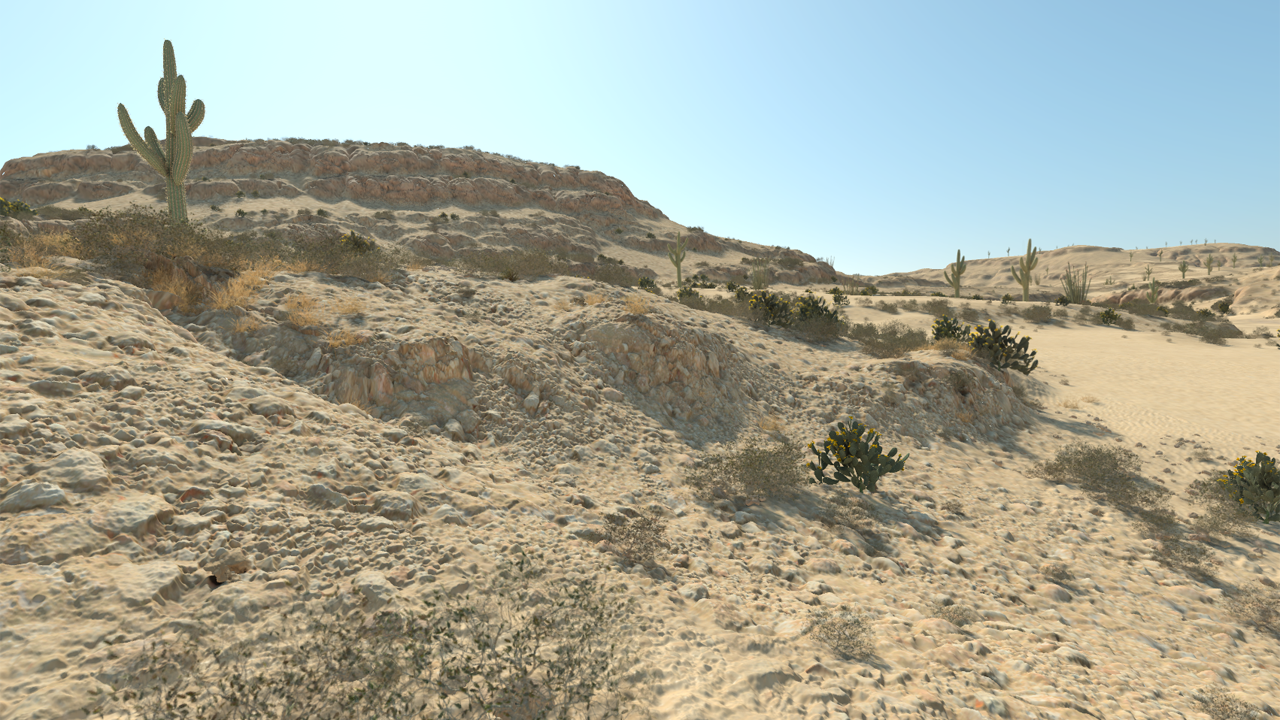
The loaded terrain
This terrain and all its data are accessible using the ART Asset Editor.
The terrain itself is a 1 km x 1 km square. It has a base accuracy of 1 meter for its first fractal component, and it’s a layered terrain composed of 4 octaves plus the base sculpting landscape. There are three different rocks and a sand layer to fill in the gaps. Here’s below a close-up view on some features of this terrain:
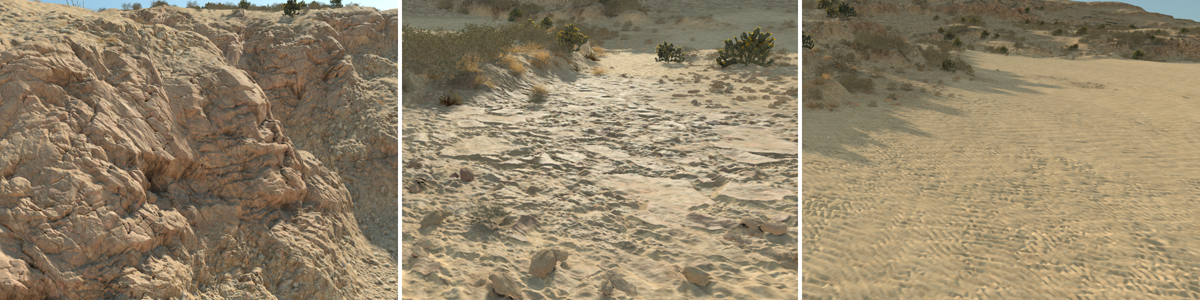
Close-up view on some features of this simple terrain.
The landscape is covered with a few desertic plants in 4 categories: grass, bushes, stones and trees. There are only two variations for each category, so only a small total of 8 vegetal species or stones.
The landscape scaling is correct:
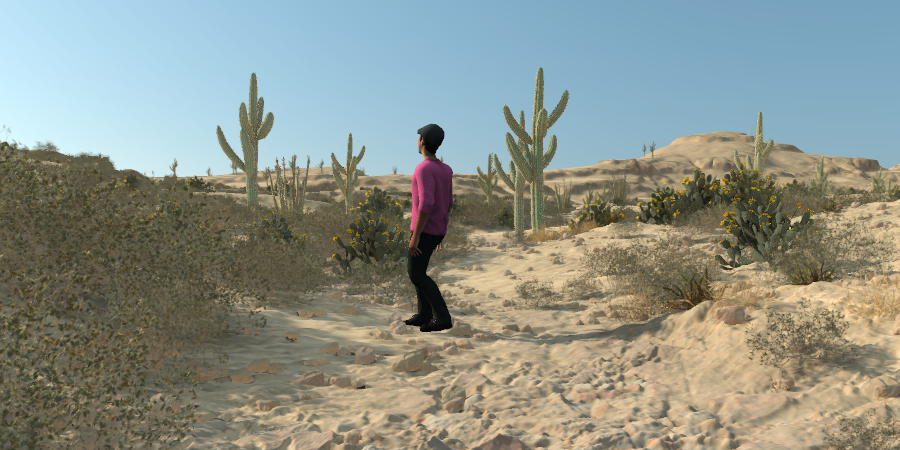
Landscape scaling is correct.
Do not forget to recalibrate the tonemapping from time to time (left toolbar button or press SPACE) if you wish to adjust the intensity of the image:
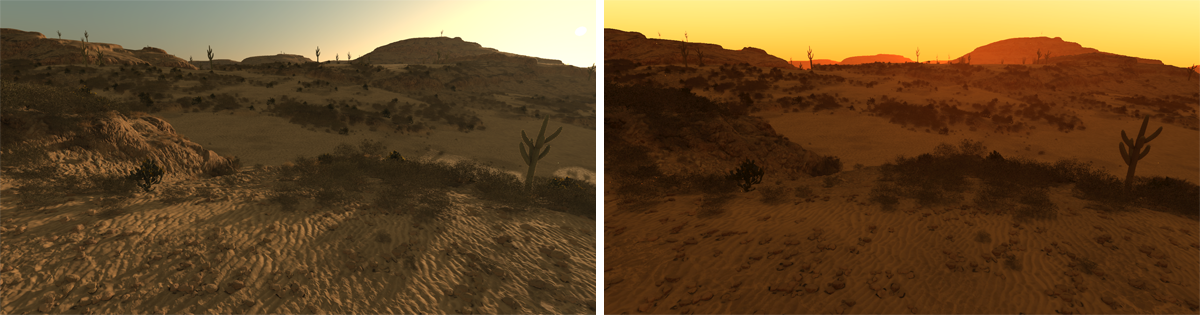
Recalibrate the tonemapping to re-expose the image according to its luminance.
Accessing ART Classes
ART is accessed using the ART namespace API in HOOPS Luminate. The ART API is quite simple to use. A number of singletons can be accessed to operate ART:
A
ART::IWorld
object. This is the main class of ART that provide an access to everything contained in the simulationA
ART::IAssetManager
object. As its name states, this class maintains all resources used by the simulationA
ART::IObserver
object. This is the camera. The observer. There’s only one observer in ART
The code to access these singletons is very similar to using the RED::Factory
, except that it uses the ART::Factory
instead:
// Access the ART world:
RED::Object* world = ART::Factory::CreateInstance( CID_ARTWorld );
if( !world )
RC_TEST( RED_ALLOC_FAILURE );
ART::IWorld* iworld = world->As< ART::IWorld >();
// Access the ART asset manager:
RED::Object* assetmgr = ART::Factory::CreateInstance( CID_ARTAssetManager );
if( !assetmgr )
RC_TEST( RED_ALLOC_FAILURE );
ART::IAssetManager* iassetmgr = assetmgr->As< ART::IAssetManager >();
// Access the observer:
RED::Object* observer = ART::Factory::CreateInstance( CID_ARTObserver );
if( !observer )
RC_TEST( RED_ALLOC_FAILURE );
ART::IObserver* iobserver = observer->As< ART::IObserver >();
Setting up a World in ART
Well, the code below is pretty self-explanatory:
iworld->SetErrorTrackingCallback( REDartErrorCallback, NULL );
RC_TEST( iworld->SetupEarthSystem() );
RED::Object* earth = iworld->GetPlanet( ART::PID_EARTH );
if( !earth )
RC_TEST( RED_FAIL );
ART::IPlanet* iearth = earth->As< ART::IPlanet >();
We start by the setup of an ‘earth’ system. This is done using ART::IWorld::SetupEarthSystem
. This method setups the sun and the earth orbital trajectory, so that we can have day & night cycles and correct orbiting periods. Then, we start the simulation using ART::IWorld::Start
.
Loading a First Asset
Let’s load the terrain. This one has been exported from the Asset Editor. It’s a .red file:
// Load our landscape:
RED::Object* atlas;
unsigned int context;
RED::Vector< RED::Object* > cameras;
RC_TEST( iassetmgr->LoadAtlas( atlas, cameras, context, "../Resources/atlas_Desert.red", REDartProgressCB, (void*)0x1 ) );
RC_TEST( iearth->SetAtlas( atlas ) );
// Start with the earth as main axis system and apply a camera position (camera for the house, observer, ground):
if( cameras.size() == 3 )
{
RC_TEST( iobserver->SetParentCelestialBody( earth ) );
RC_TEST( iobserver->SetFromCamera( cameras[ 1 ] ) );
}
// Start the world:
RC_TEST( iworld->Start( window, REDartProgressCB, NULL ) );
Note
The loading of the atlas containing the desert landscape also return a list of cameras. These cameras are defined in the asset editor and can be used as reference positions for various purposes.
Resizing and Updating ART
Call ART::IWorld::ResizeWindow
to adjust the simulation window size to the size of the hosting window.
Call ART::IWorld::Update
to update the simulation every frame.
Shutting Down ART
Call ART::IWorld::Shutdown
to terminate the simulation. All loaded resources are released. HOOPS Luminate remains alive.