Cutting Geometries: Using Multiple Cutting Planes
The GPU based approach to section cutting has a number of limitations:
- The number of cutting planes and the cutting planes equations can’t be freely chosen
- It’s neither possible to extract the cut section mesh nor to extract the contour of the cut
However, the GPU based approach is fast. It’s real-time.
The approach we propose in this tutorial aim at solving the two first points: the method we use will create the geometries resulting of the cut so that all informations will be accessible: the cut mesh, meshes resulting of each plane cut and the corresponding contours. The number of planes is not constrained and any plane equation can be used.
Of course there’s a price to pay for all that: the method is based on a software algorithm, resulting geometries are actually calculated and returned, so the method may take some time to process large meshes.
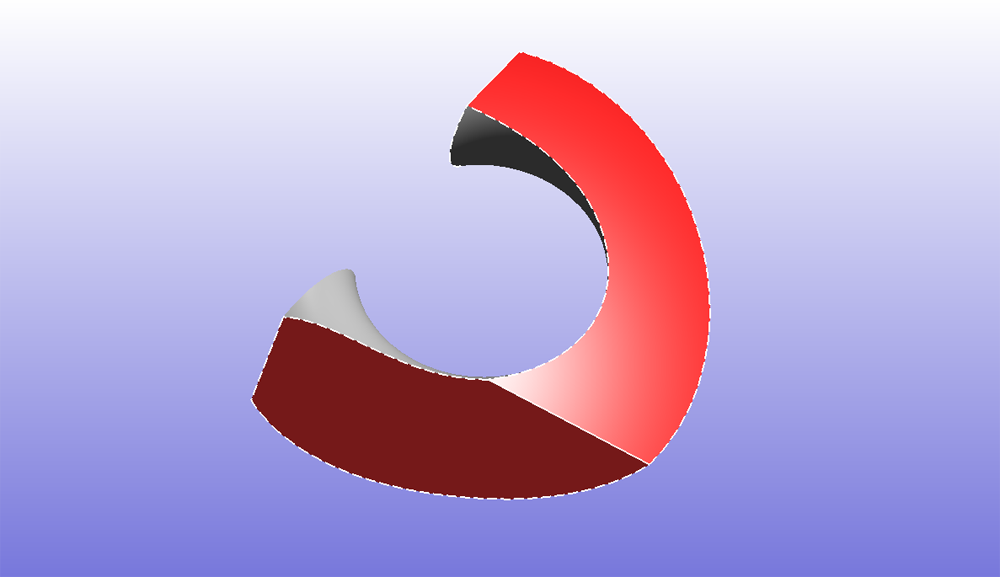
An example of slicing a torus by three planes.
Using RED::IMeshShape::BuildCutMesh
All the slicing is done using one single call:
for( i = 0; i < g_nb_planes; i++ )
{
RED::Object* section_mesh = RED::Factory::CreateInstance( CID_REDMeshShape );
if( !section_mesh )
RC_TEST( RED_ALLOC_FAILURE );
RED::IShape* issection_mesh = section_mesh->As< RED::IShape >();
RC_TEST( issection_mesh->SetMaterial( matr_red, iresmgr->GetState() ) );
RED::Object* contour_line = RED::Factory::CreateInstance( CID_REDLineShape );
if( !contour_line )
RC_TEST( RED_ALLOC_FAILURE );
RED::IShape* iscontour_line = contour_line->As< RED::IShape >();
RC_TEST( iscontour_line->SetMaterial( matr_white, iresmgr->GetState() ) );
RC_TEST( g_plane.push_back( planeeq[ 4 * i ] ) );
RC_TEST( g_plane.push_back( planeeq[ 4 * i + 1 ] ) );
RC_TEST( g_plane.push_back( planeeq[ 4 * i + 2 ] ) );
RC_TEST( g_plane.push_back( planeeq[ 4 * i + 3 ] ) );
RC_TEST( g_section.push_back( section_mesh ) );
RC_TEST( g_contour.push_back( contour_line ) );
}
RC_TEST( immesh->BuildCutMesh( g_cut_mesh, g_section, g_contour, g_plane, g_tol, iresmgr->GetState() ) );
Each cut plane added to the list of cutting planes will produce a RED::ILineShape
containing contours and a RED::IMeshShape
containing the tessellation of these contours. Pleae note that the generation of contours and contours tessellation is optional.
The method also produces the resulting mesh that has survived after the cut. The source mesh is not modified by the method;
Results of the method have to be added to the scene graph for the display and rendered with the appropriate materials.
RC_TEST( icamera->AddShape( g_cut_mesh, iresmgr->GetState() ) );
for( i = 0; i < (int)g_contour.size(); i++ )
RC_TEST( icamera->AddShape( g_contour[i], iresmgr->GetState() ) );
for( i = 0; i < (int)g_section.size(); i++ )
RC_TEST( icamera->AddShape( g_section[i], iresmgr->GetState() ) );
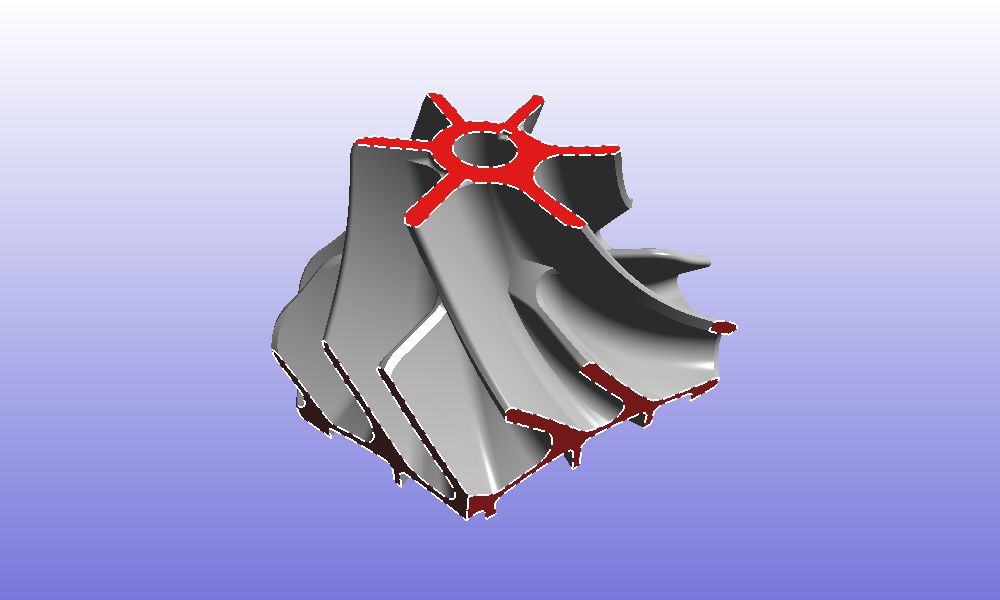
Another example slicing a complex mesh.