Input-Output API
In addition to the RED file format (see The .red File Format) HOOPS Luminate allows to load external data in other file formats:
- .FBX (v7.3)
- .OBJ and .MTL
- .DXF (v13)
- .DAE (v1.5)
The import-export operations can be done using the RED::IOTools
functions:
RED::IOTools::Load
andRED::IOTools::Save
This API needs an auxiliary module to work: REDFbx.dll provided with HOOPS Luminate. Be sure to have it in your application folder before using RED::IOTools
. The module is based on the Autodesk FBX SDK 2016.0.
Note
The REDFbx library requires Microsoft Visual Studio 2010 redistributables.
Loading and Saving External Files
During a loading operation, all the content of the file is stored in the RED::IDataManager
using contexts like with the .RED files (see Loading .red Files). A context corresponds to a whole file. So, when the loading is successful, the context array is returned with at least one new entry. Contexts are logical views of the content of a file. They let you access to parts of file even after it may have been merged with other into the RED::IDataManager
.
Loading a File using the Input-Output API
The external file loading and saving functions are exposed through the RED::IOTools
class.
// The array containing context IDs:
RED::Vector< unsigned int > contexts;
// Material options:
// - Load material as RED generic ones.
// - Load texture transparency informations in their ALPHA channel.
// - Convert file units to cm.
// - Use a layerset for realtime and no layerset for photorealistic.
RED::IO_LOAD_MATERIAL_TYPE materialType = RED::IOLMT_GENERIC;
RED::IO_LOAD_TEXTURE_TRANSPARENCY transparencyData = RED::IOLTT_ALPHA;
RED::IO_SYSTEM_UNIT units = RED::IOSU_CM;
RED::LayerSet rtLayer;
rtLayer.AddLayer( 12345 );
// Naming map used to associate the RED object ID to their loaded names.
RED::Map< unsigned int, RED::String > namingTable;
RC_TEST( RED::IOTools::Load( contexts, "../resources/scene.fbx", RED::IOOT_ALL,
materialType, &rtLayer, NULL, transparencyData, units, false, iresmgr->GetState(),
0, &namingTable, (RED::ProgressCallback)loadingProgressCallback ) );
Like .RED file loading, RED::IOTools
loads file inside a context an add it to the list in parameters.
The optional naming map parameter is filled during the loading and associates the RED object ID to their name in the file.
Finally, an optional progress callback can be given. The function will be called regularly during the loading process. The function looks like this:
void loadingProgressCallback( const RED::String& iMessage,
float iProgress )
{
// Display the message and a progress bar...
}
Accessing to Loaded Data
Here is an example of access to all the viewpoints loaded from a file:
// iresmgr is a pointer to the RED::IResourceManager interface.
// context is the vector of loaded contexts.
RED::IDataManager* idatamgr = iresmgr->GetDataManager()->As< RED::IDataManager >();
for( unsigned int c = 0; c < context.size(); ++c )
{
// Get the number of viewpoints in the current context.
unsigned int vcount;
RC_TEST( idatamgr->GetViewpointsCount( vcount, context[c] ) );
for( unsigned int v = 0; v < vcount; ++v )
{
RED::Object* viewpoint;
RC_TEST( idatamgr->GetViewpoint( viewpoint, context[c], v, iresmgr->GetState() ) );
}
}
Data stored in the RED data manager are not managed by the RED resource manager. Therefore, to destroy loaded data, you need to call a dedicated method in RED::IDataManager
. The example below illustrates the destruction of an image, a full context or the whole stored data:
// image is a pointer to a loaded image.
// image_context is the context of the loaded image.
// Delete the image.
RC_TEST( idatamgr->ReleaseData( image_context, image, iresmgr->GetState() ) );
// Delete all the other image context data.
RC_TEST( idatamgr->ReleaseContext( image_context, false, iresmgr->GetState() ) );
// Delete all the contexts.
RC_TEST( idatamgr->Release( iresmgr->GetState() ) );
With the RED::IOTools::Save
function, HOOPS Luminate can export a list of complete scenegraphes and viewpoints to an external file format.
Saving a File using the Input-Output API
The external file loading and saving functions are exposed through the RED::IOTools
class.
// The arrays containing the data to save:
// - RED scene graphes;
// - RED viewpoints.
// - RED animation clip controllers.
RED::Vector< RED::Object* > dag;
RED::Vector< RED::Object* > viewpoints;
RED::Vector< RED::Object* > animations;
// Naming map used to associate the RED object ID to their loaded names.
RED::Map< unsigned int, RED::String > namingTable;
RC_TEST( RED::IOTools::Save( &dag, &viewpoints, &animations, "../resources/scene.fbx", RED::IOOT_ALL,
true, false, RED::IOSU_CM, false, RED::IOFV_DEFAULT, resmgr,
&namingTable, (RED::ProgressCallback)loadingProgressCallback ) );
The RED::IOTools::Save
function allows to save a list of RED::IViewpoint
and a list of scenegraphes given the root (RED::IShape
).
The optional naming map parameter is used to save the RED object names associated with their ID. While the loading operation fills the table, the save operation reads it.
Finally, an optional progress callback can be given. The function will be called regularly during the saving process. The function looks like this:
void savingProgressCallback( const RED::String& iMessage,
float iProgress )
{
// Display the message and a progress bar...
}
Importing FBX File
From an external FBX file, the RED::IOTools
function imports the following features:
- Complete scenegraph node hierarchy and the transform matrices
- Mesh and line shapes
- Materials with textures
- Cameras
- Lights
The RED::IOTools::Load
function lets the user defines which objects he want to import using the RED::IO_OBJECT_TYPE
flags.
HOOPS Luminate converts the FBX scenes to right-handed Z-up coordinates. Units are converted to cm.
Scene Graph
The FBX node hierarchy and their transform matrices are imported as RED::ITransformShape
.
Mesh
FBX meshes data are imported into HOOPS Luminate as RED::IMeshShape
following these rules:
- Vertex position in the
RED::MCL_VERTEX
channel- Vertex color in the
RED::MCL_COLOR
channel- Normal in the
RED::MCL_NORMAL
channel- Texture coordinates in the
RED::MCL_TEX0
toRED::MCL_TEX7
channels- Tangent and binormal are stored in the
RED::MCL_USER0
andRED::MCL_USER1
channels
FBX skeleton and skinning data are also imported and stored in the RED::IMeshShape
.
If the FBX file contains a skinned mesh and a skeleton, the skinned mesh parent nodes will be ignored and the mesh object will be moved as child of the (animated) skeleton parent node.
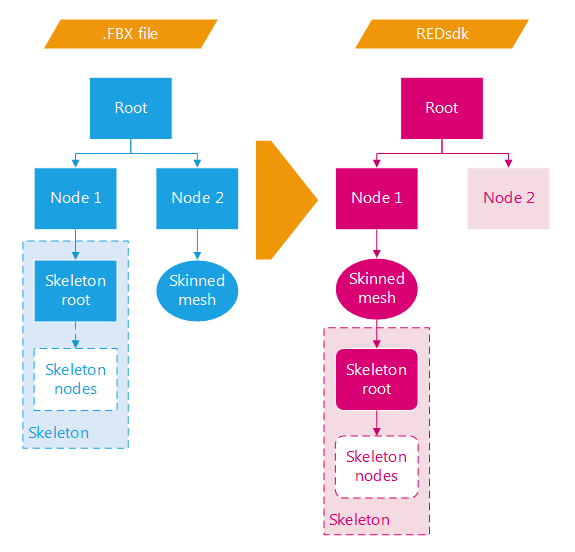
Skinned mesh moved under its skeleton
During the loading process, the meshes are triangulated and split to have one material per mesh. The vertices are duplicated to have one normal per vertex if needed.
Note
To speed-up the loading function, one should ensure that his models are already in the correct RED format (triangles, one color / normal / texcoord per vertex).
FBX line data are imported as RED::ILineShape
following the same rules as meshes. FBX line shapes don’t handle materials. The HOOPS Luminate default material will be applied and used for display. RED::ILineShape
objects will contain segment primitives.
Note
The fbx file format doesn’t support point primitives (RED::IPointShape
).
Material
According to a parameter, user can specify which type of RED material he wants to create during the FBX material import (RED::IO_LOAD_MATERIAL_TYPE
):
- Generic material (uses
RED::IMaterial::SetupGenericMaterial
)- Realistic material (uses
RED::IMaterial::SetupRealisticMaterial
)
All the FBX Lambert or Phong material properties are translated to the RED generic material. This includes:
- Emissive color and texture
- Ambient color and texture
- Diffuse color and texture
- Specular color and texture as well as shininess
- Transparency color and texture
- Bump texture
The Realistic material only handles these parameters:
- Diffuse color and texture
- Transparency color and texture
- Bump texture
Material textures are loaded from the FBX specified filename. If not found, HOOPS Luminate searches images next to the FBX file. For transparency textures, HOOPS Luminate lets the user choose the transparency channel (RED::IO_LOAD_TEXTURE_TRANSPARENCY
). Sometimes transparency data can be in the alpha channel of the diffuse texture, sometimes it can be in the RGB channels. Material textures are automatically bound to the correct mesh texture coordinate channels.
Light
HOOPS Luminate imports the following FBX light types and their parameters as well as their attenuation data:
- Ambient Light
- Directional Light
- Spot Light
- Point Light
- Rectangular Area Light
The rectangular area lights are built with the RED::ILightShape::SetRectangularAreaLight
function. The number of sample is set to 4 by default. If you want to change it, just get the light and call RED::ILightShape::SetSamplesCount
. The size of the area is set to 1 unit since FBX sdk only supports unit areas. The size is controlled by the parent node scale transform.
Camera
FBX target and free cameras are loaded as RED::IViewpoint
. They can be perspective or orthographic.
Note
HOOPS Luminate viewpoints are always at the root of the scenegraph. All the node hierarchy upper to the FBX camera will be ignored during the loading of the file.
Animation
FBX node transform animations are imported as RED::IAnimationClipController
. They are not linked to any transform shape as they can be used to animate anything. User must use it manually to move its target object (see the tutorial: Playing an Animation Clip).
FBX skeletal animations are imported as RED::ISkeletalAnimationController
. This type of animation is linked to a skinned RED::IMeshShape
object if the associated skinned mesh is included in the FBX. If not, it must be associated by the user later (RED::ISkeletalAnimationController::AddSkinnedMesh
).
Exporting FBX File
The RED::IOTools
function exports the following HOOPS Luminate features into FBX:
- Complete scenegraph node hierarchy and the transform matrices
- Mesh and line shapes
- Generic and realistic materials with textures
- Viewpoints
- Lights
The RED::IOTools::Save
function lets the user defines which objects he want to export using the RED::IO_OBJECT_TYPE
flags.
Scene Graph
The RED Direct Acyclic scene Graph is completely saved in FBX as node containing transform matrices.
Mesh
RED::IMeshShape
are exported as FBX meshes. The exported meshes contain only triangles. Their data are:
- Vertex position (
RED::MCL_VERTEX
)- Vertex color (
RED::MCL_COLOR
)- Normal (
RED::MCL_NORMAL
)- Texture coordinates (
RED::MCL_TEX0
toRED::MCL_TEX7
)RED::MCL_USER0
andRED::MCL_USER1
are saved as tangent and binormal data
Skeletons are exported as a hierarchy of FBX nodes. Skinning data are exported as FBX mesh data.
RED::ILineShape
are exported as FBX lines. Arbitrary line strips are built during export for RED::ILineShape
objects containing segment primitives.
Note
The fbx file format doesn’t support point primitives (RED::IPointShape
).
Material
The HOOPS Luminate material export is based on the associated RED::IMaterialController
and its RED::IMaterialControllerProperty
list. Only materials with valid property names are exported to FBX.
Properties currently exported are:
- Emissive (
RED_MATCTRL_EMISSIVE_COLOR
,RED_MATCTRL_EMISSIVE_TEXTURE
,RED_MATCTRL_EMISSIVE_CHANNEL
,RED_MATCTRL_EMISSIVE_MATRIX
) - Ambient (
RED_MATCTRL_AMBIENT_COLOR
,RED_MATCTRL_AMBIENT_TEXTURE
,RED_MATCTRL_AMBIENT_CHANNEL
,RED_MATCTRL_AMBIENT_MATRIX
) - Diffuse (
RED_MATCTRL_DIFFUSE_COLOR
,RED_MATCTRL_DIFFUSE_TEXTURE
,RED_MATCTRL_DIFFUSE_CHANNEL
,RED_MATCTRL_DIFFUSE_MATRIX
) - Specular (
RED_MATCTRL_SPECULAR_COLOR
,RED_MATCTRL_SPECULAR_TEXTURE
,RED_MATCTRL_SPECULAR_CHANNEL
,RED_MATCTRL_SPECULAR_MATRIX
,RED_MATCTRL_SPECULAR_EXPONENT
) - Opacity (
RED_MATCTRL_OPACITY_COLOR
,RED_MATCTRL_OPACITY_TEXTURE
,RED_MATCTRL_OPACITY_CHANNEL
,RED_MATCTRL_OPACITY_MATRIX
) - Bump (
RED_MATCTRL_BUMP_TEXTURE
,RED_MATCTRL_BUMP_CHANNEL
,RED_MATCTRL_BUMP_MATRIX
)
Generic and realistic materials can be exported to FBX as they have a controller handling these valid property names.
The RED::IOTools::Save
function saves all the material textures on disk next to the output file. They are then embedded into the FBX file and thus can be deleted from the output folder. Textures are saved as PNG files. Material textures are automatically bound to the correct mesh texture coordinate channels.
Light
RED::ILightShape
objects are exported to FBX files. Light type can be:
- Ambient
- Directional
- Spot
- Point
- Rectangular Area
The size of the rectangular area light is set to 1 unit at export since FBX sdk only supports unit areas. The size set in HOOPS Luminate is converted to scaling and applied to the parent node.
Camera
RED::IViewpoint
objects are exported as FBX cameras. The first viewpoint of the provided list is defined as the default FBX camera. Orthographic and perspective cameras are exported.
Animation
RED::IAnimationClipController
objects are exported as FBX animated nodes.
In HOOPS Luminate, the RED::IAnimationClipController
objects are not linked with any scenegraph object. The basic animations are saved as animated nodes located under a node named “Animations” directly under the FBX root. When opening the .FBX file with another 3D graphics software, the user have to reassign the animations to their proper objects.
Skeletal animations (RED::ISkeletalAnimationController
) are exported as animation curves for the skeleton nodes already exported.
Importing and Exporting OBJ, DAE and DXF Files
HOOPS Luminate uses the FBX SDK to deal with OBJ, DAE and DXF files. Therefore, the majority of the FBX options described previously also applied for these formats.
Several limitations may occurs when using these formats. They may come from the format itself or from the FBX sdk.
OBJ Format
OBJ files contains only geometry data:
- Vertex
- Color
- Normal
- Texture Coordinates
Unlike .FBX, .OBJ does not contain:
- Node Matrix Hierarchy
- Lights
- Camera
- Animation
A separate .MTL file can be associated to the .OBJ to specify material informations:
- Ambient Color and Texture
- Diffuse Color and Texture
- Specular Color and Texture
- Transparency Value and Texture
Due to the FBX sdk, only diffuse textures are correctly exported to the .MTL file (no ambient texture, specular texture or transparency texture). The OBJ file format does not handle bump data. The texture coordinates translations, scales and rotations are not saved.
The OBJ format handles only one texture coordinates channel. The FBX sdk does not export the materials of objects with more than one texture coordinates channel.
DAE Format
The DAE format allows to store:
- Vertex Data (Vertex, Normal, Color, Texture Coordinates)
- Node Matrix Hierarchy
- Lights
- Camera
- Materials
The DAE format does not handle bump data. UV transforms are not exported in the DAE file.
The FBX sdk prevents the transparency textures to be saved. However they can be loaded correctly.
DXF Format
The DXF file format is the most restrictive. It can only store the vertex positions, but not the normals nor the texture coordinates.
Also, only material diffuse color is correctly exported and imported.
No lights, camera or scene hierarchy is handled by the DXF format.