Using the Generic Material
Material creation and shader programming can be quite complex and takes time. Fortunately, HOOPS Luminate provides a convenient tool for users who don’t want to dive into this vast world: the generic material. The generic material is a ready-to-use, fast and reliable material composed of built-in shaders for any types of rendering from GPU real-time to software photorealism.
A generic material can be easily created using the function RED::IMaterial::SetupGenericMaterial
which embeds all the complexity and builds an optimal material according to the needs and parameters.
The user can provide up to two layer sets (RED::LayerSet
) to generate the real-time version of the material, the photo-realistic one or both. Moreover, a material controller (RED::IMaterialController
) is automatically created and setup to give the user a high-level view of the generated material.
Even with both version, only a single consolidated set of parameters is exposed through the material controller. Hence, when modifying the diffuse color of a generic material, both real-time and photo-realistic versions of the material will be updated accordingly.
The generic material features:
- a Lambertian diffuse model (optionally Phong if specular is used)
- bump mapping
- glossy reflections (following the Fresnel equations or not)
- colored ior-based glossy transparencies
Creating a Generic Material
// Get a pointer to the RED resource manager.
RED::Object* resmgr = RED::Factory::CreateInstance( CID_REDResourceManager );
RED::IResourceManager* iresmgr = resmgr->As< RED::IResourceManager >();
// Create a material.
RED::Object* mat;
RC_TEST( iresmgr->CreateMaterial( mat, iresmgr->GetState() ) );
RED::IMaterial* imat = mat->As< RED::IMaterial >();
// Create the two layer sets for real-time and photorealism.
RED::LayerSet realtime, photo;
realtime.AddLayer( 01234567 );
photo.AddLayer( 76543210 );
RC_TEST( imat->SetupGenericMaterial( true, false,
RED::Color::BLACK, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0,
RED::Color::GREY, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0,
RED::Color::GREY, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0,
RED::Color::BLACK, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, 0.0f,
RED::Color::GREY, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, 0.0f,
true, false, NULL, RED::Matrix::IDENTITY, 1.0, true,
RED::Color::WHITE, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, 0.0f,
NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, RED::MCL_USER0,
&realtime, &photo, resmgr, iresmgr->GetState() ) );
// Get the material controller to later update its properties.
RED::Object* matctrl = iresmgr->GetMaterialController( mat );
RED::IMaterialController* imatctrl = matctrl->As< RED::IMaterialController >();
In this sample code, a simple generic material is created. Its parameters are:
- double sided
- don’t use Fresnel
- emissive properties (color and texture, no emission)
- ambient properties (color and texture, ambient similar to diffuse )
- diffuse properties (color and texture)
- specular properties (color and texture, no specular)
- reflection and refraction properties (the auto environment is computed for the real-time version)
- opacity properties
- no bump mapping
- the two layer sets for real-time and photorealism
At the end, the material controller can be retrieved from the material to update its properties.
Although the function and parameters are the same for real-time and photo-realistic rendering, each mode has its own capabilities and uses its own shaders.
For Real-Time
Real-time configuration is intended for fast interactive rendering. Therefore environmental mapping is used instead of ray-traced reflections. The mapping must be either automatic or done with a valid provided cube texture.
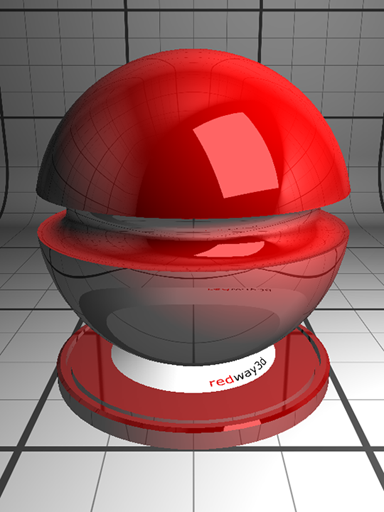
Generic material in real-time
For Photo-Realistic
The photo-realistic configuration is the first choice for high quality rendering. This rendering mode uses global illumination (if enabled) instead of ambient lighting and real ray-traced reflections.
Unlike the realistic material (see Using the Realistic Material), in order to produce photo-realistic images with the generic material, it’s up to the user to ensure that the parameters are consistent. The sum of the diffuse, reflection and refraction terms must be lower or equal to 1 to preserve the energy conservation.
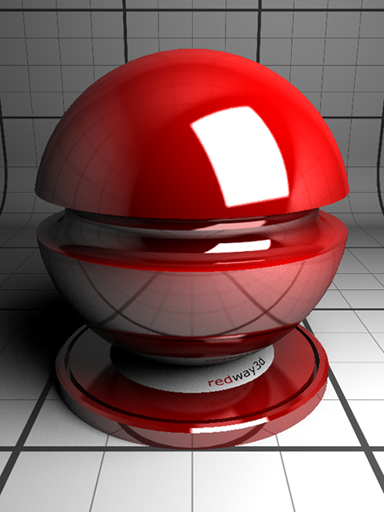
Generic material in software photo-realistic mode
Other Generic Material Creation Functions
The RED::IMaterial::SetupGenericMaterial
function is the main function to build generic materials. It contains all the parameters to handle all the available options.
The sdk provides other helper functions to create the generic material handling less parameters. Their purpose is to simplify the call when just a basic material is wanted. These functions are:
RED::IMaterial::SetupGenericDiffuseMaterial
: handles only the diffuse optionsRED::IMaterial::SetupGenericBumpyDiffuseMaterial
: handles only the diffuse and bump optionsRED::IMaterial::SetupGenericLambertMaterial
: handles emissive, ambient, diffuse, transparency and bump optionsRED::IMaterial::SetupGenericPhongMaterial
: handles Lambert options plus specular shading