Skinned Mesh Shapes
In addition to the default services described in the Mesh Shapes page, the RED::IMeshShape
interface provides functions to set and access skeleton and skinning data. Skeleton describes a hierarchy of bones. Skinning data associates the mesh vertices to one or more skeleton bones. This allows to animate mesh shape vertices by moving the skeleton bones. The main purpose of these data is to animate 3D characters.
Skeleton Definition
The skeleton is simply a hierarchy of bones contained in the mesh shape. It is thus always considered as a child of the mesh shape in the HOOPS Luminate scenegraph. If a transform node moves the mesh shape, the skeleton will follow.
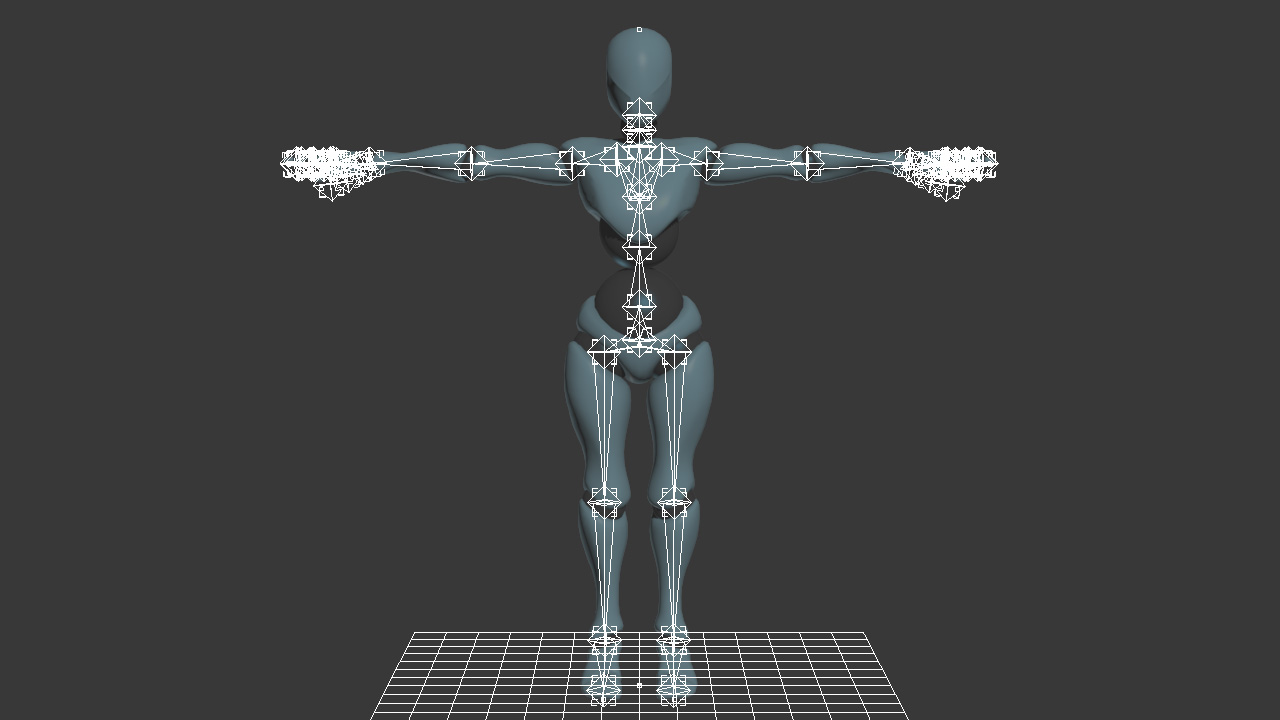
A humanoid skeleton and its mesh in Autodesk 3dsMax
A skeleton bone contains several informations:
the link to its parent and children bones:
RED::IMeshShape::GetBoneParent
,RED::IMeshShape::GetBoneChild
its transform matrix handling position, rotation and scale:
RED::IMeshShape::GetBoneMatrix
the neutral pose is its initial transform matrix before any update has been done:
RED::IMeshShape::GetBoneNeutralPose
an optional name to facilitate bone requests:
RED::IMeshShape::GetBoneName
Each bone transform is related to the bone parent (local transforms). This implies that if a bone is moved, all its children hierarchy will move with him.
The RED::IMeshShape
interface provides one method to create the skeleton: RED::IMeshShape::AddBone
. To create a complete skeleton, simply call this function for each bone. Its parameters are all the data described previously.
Note
The bones must be added from parent to children. A bone can’t be created if its parent have not already been created (with the exception of the root). This implies that the root bone is always added first and will always have the index 0.
Skinning Data
The skinning data are all the informations that associate a vertex to one or several bones of the skeleton.
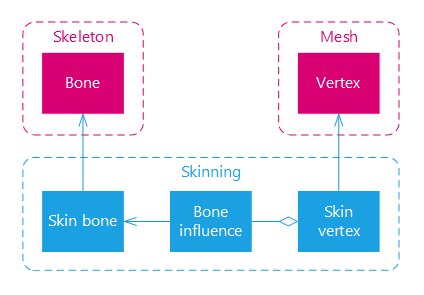
Skinning data relationships
To set skinning data, user must first set the skin bones with RED::IMeshShape::SetSkinBone
. The skin bone is a reference to the real skeleton bone that will be used by the skin vertices. A reference matrix is associated to the skin bone. It is the bone transform in the mesh frame used at binding time (generally called bind pose matrix).
The second skinning data are the skin vertices (RED::IMeshShape::SetSkinVertex
). A skin vertex is a reference to the real mesh vertex.
The skin vertex also contains a reference to one or more skin bones via the bone influence (RED::IMeshShape::SetBoneInfluence
). A bone influence associates a skin vertex to a skin bone with a weight between 0 and 1. A weight of 0 means that the vertex is not influenced at all by the bone. A weight of 1 means that the vertex is entirely moved by this unique bone.
Note
The sum of the weights of the bones influences associated to a skin vertex must always be equal to 1.
Doing the Skinning Operation
To effectively do the skinning operation, i.e. move the mesh vertices according to the skeleton and skinning definition, the user must call the RED::IMeshShape::DoSkinning
function. Before that, an initialization must be done once with RED::IMeshShape::InitializeSkinning
.
Note
The skinning operation updates the vertex and normal data of the mesh (RED::MCL_VERTEX
and RED::MCL_NORMAL channels
). Currently, skinning operations works only with channels of size greater or equal to 3 and of type RED::MFT_FLOAT
.
Note
If the mesh vertex and/or normal array definitions are changed after the skinning initialization, the RED::IMeshShape::InitializeSkinning
function must be called again.
The RED::IMeshShape::ResetSkinning
method resets all the mesh vertices and normals at their initial state (at the moment of the RED::IMeshShape::InitializeSkinning
call) as if RED::IMeshShape::DoSkinning
was never called.
The complete workfow to initialize a mesh for skinning is:
Setup the mesh vertices (
RED::IMeshShape::SetArray
)Setup the mesh skeleton (
RED::IMeshShape::AddBone
)Setup the mesh skinning (
RED::IMeshShape::SetSkinBone
andRED::IMeshShape::SetSkinVertex
)Call the
RED::IMeshShape::InitializeSkinning
function
At update time, the workflow is:
Move the skeleton (
RED::IMeshShape::SetBoneMatrix
or Skeletal Animation)Do the skinning (
RED::IMeshShape::DoSkinning
)