Instances
The HOOPS Luminate scene graph supports instancing. An instance can be obtained that way:
Creating an Instanced Shape
An instanced shape is a shape that exists once in the scene graph and that is visualized several times. We’ll assemble the following scene graph in this task example:
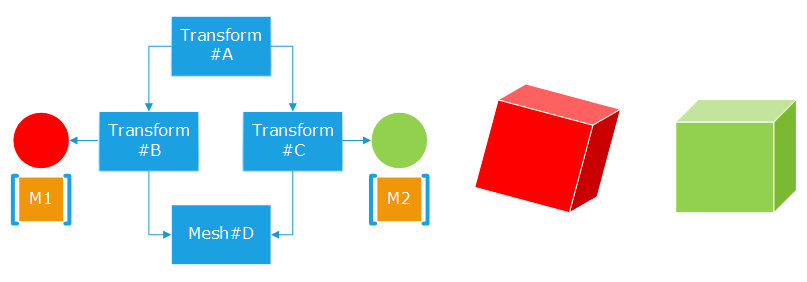
Mesh#D is instanced twice. It has two direct parents: Transform#B and Transform#C. Therefore, if we parse the scene graph from Transform#A, we have two shapes to visualize: The first shape instance has path #ABD and the second one has #ACD. At the Transforms #B and #C level, we see that we have different materials and transformation matrices. This can be used to make each instance unique.
The code needed to construct this assembly is:
// Create our shapes and access their interfaces:
RED::Object* shapeA = RED::Factory::CreateInstance( CID_REDTransformShape );
RED::ITransformShape* ishapeA = shapeA->As< RED::ITransformShape >();
RED::Object* shapeB = RED::Factory::CreateInstance( CID_REDTransformShape );
RED::ITransformShape* ishapeB = shapeB->As< RED::ITransformShape >();
RED::Object* shapeC = RED::Factory::CreateInstance( CID_REDTransformShape );
RED::ITransformShape* ishapeC = shapeC->As< RED::ITransformShape >();
RED::Object* shapeD = RED::Factory::CreateInstance( CID_REDMeshShape );
// Assemble the scene graph:
RC_TEST( ishapeA->AddChild( shapeB, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
RC_TEST( ishapeA->AddChild( shapeC, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
RC_TEST( ishapeB->AddChild( shapeD, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
RC_TEST( ishapeC->AddChild( shapeD, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
Then, from this code, you simply need to setup matrices, materials, layersets or any other shape attribute to distinguish the two instances of Mesh#D. See Shape Attributes in a Scene Graph for details.
Instances are key elements in many scene graphs as they’re generally stored once in memory. However, there are exceptions to this general behavior, mainly for performance reasons, that are detailed here: Analyzing Performances.
Identifying Instances in a Scene Graph
One of the issues that arise with instances is that the address of a given shape object does not let you uniquely identify an instance of a shape. To be able to uniquely identify an instance of a shape, the full path from the root of the scene graph down to the instanced shape is needed. In the Creating an Instanced Shape task quoted above, the first instance is uniquely identified by path #ABD and the second one by path #ACD.
HOOPS Luminate uses the RED::ShapePath
object to deal with instances. For instance, a picking operation done using RED::IWindow::FramePicking
will return RED::ShapePath
instances uniquely identifying all picked objects.
Instancing and Physical Lights
If an instanced mesh is used as a light emitter mesh using RED::IPhysicalLightShape::SetEmitter
, then as many lights as the number of instances of that mesh will be created. A physical light should not be instanced by itself as this will create redundant lights with duplicate positions, all using the same emitter mesh.
Therefore, the solution is to instance the mesh which is chosen once as a light emitter.
Instance Counters
It may be interesting for an application to identify instances at the time of the rendering. There’s a HOOPS Luminate mechanism to pursue this objective: this is the “instance counter callback”, that can be defined using RED::IViewpoint::SetInstanceCounterCallback
. A dedicated tutorial on this topic is available here: Using Instance Counters.