Windows
The window is the second object to be created in a HOOPS Luminate application, after the resource manager:
Creating a HOOPS Luminate Window using the RED::Factory
// 'application_window' must be a valid application HWND pointer.
RED_RC rc;
HWND hwnd = application_window;
RED::Object* resmgr = RED::Factory::CreateInstance( CID_REDResourceManager );
int width = 1920;
int height = 1080;
// Create a rendering window:
RED::Object* window = RED::Factory::CreateREDWindow( *resmgr, hwnd, width, height, NULL, rc );
if( !window )
{
// Handle critical errors here.
}
if( rc != RED_OK )
{
// Handle error codes here.
}
In this example, we only show the windows OS code. The window creation works similarly on Linux and MacOS, provided the operating specific parameters. See the RED::Factory::CreateREDWindow
method for details. Please note that it’s mandatory to check the window creation return code to be sure that the operation has succeeded.
The window can be created using an operating system specific window handle, or it can be created directly using an existing window OpenGL setup:
Integrating a HOOPS Luminate Window into an Existing OpenGL Window
A HOOPS Luminate window can be created from a window handle provided by the application (a HWND on Windows, a NSView on OSX, a Display / Visual / Screen info on Linux). In this case, the HOOPS Luminate window internally creates and manages the OpenGL context it’ll use for the rendering. In HOOPS Luminate, it’s possible to create a window that uses an OpenGL context that exist already and that is used by the hosting application already:
// Assuming we have an application that has a running OpenGL window:
// - 'app_hwnd' must be be a valid application handle (HWND).
// - 'app_hdc' is a valid application device context (HDC).
// - 'app_hglrc' is a valid application OpenGL context (HGLRC).
RED_RC rc;
RED::Object* resmgr = RED::Factory::CreateInstance( CID_REDResourceManager );
int width = 1920;
int height = 1080;
// Create a RED::WindowRenderInfo instance to setup external OpenGL parameters:
RED::WindowRenderInfo winfo;
winfo.SetHostingContext( app_hdc, app_hglrc );
// Create a rendering window using the external context specification:
RED::Object* window = RED::Factory::CreateREDWindow( *resmgr, app_hwnd, width, height, &winfo, rc );
if( !window )
{
// Handle critical errors here.
}
if( rc != RED_OK )
{
// Handle error codes here.
}
Once created, HOOPS Luminate can be used to draw in the application window directly, co-existing with the application’s OpenGL code. See Integration into an External OpenGL Window for details on how to proceed.
The window is used to:
See something on screen
Manage a list of offscreen rendering buffers
It implements the
RED::IWindow
andRED::IOptions
interfaces
Image and all hardware operations can’t be performed before the creation of a first window by the application, because the creation of the window initializes the OpenGL driver layer. This behavior also applies even if HOOPS Luminate is started in pure software mode.
A window contains rendering buffers (Viewpoint Render Lists) that contain cameras and scene graph to display.
As many windows as wished can be created by the application. The list of windows in the application can be retrieved from a call to RED::IResourceManager::GetWindowList
.
This chapter also reviews different topics about window setup and usage in a HOOPS Luminate based application:
Configuring a Window: Review of the different parameters that can be setup at the window creation time.
Integration into an External OpenGL Window: This is a very important topic. HOOPS Luminate can be added to an existing OpenGL application without breaking the existing application graphics. Therefore, HOOPS Luminate graphics can be added to the application without having to rewrite everything.
Managing Multiple Windows and External OpenGL Contexts: This paragraph will go further in the configuration and detail how HOOPS Luminate can be used in the scope of an existing multiple window application and smoothly insert its own graphics.
Window and Viewpoint Render Lists
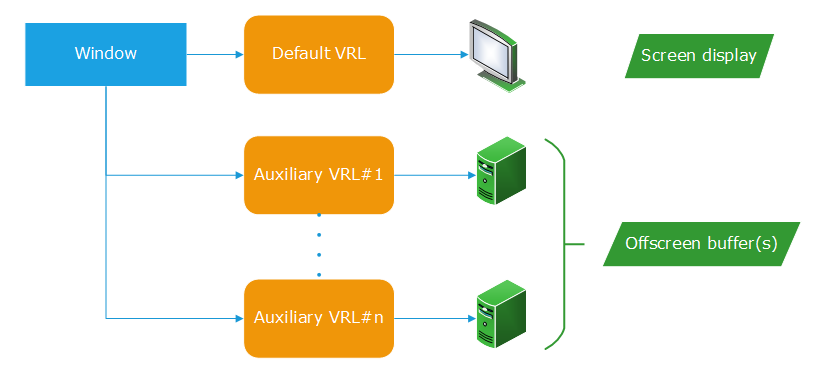
The relationship between a window and the list of VRL it stores
A ‘viewpoint render list’ or VRL for short is either an onscreen or offscreen rendering buffer that owns a list of viewpoints to render. The window owns a default VRL at its creation. This VRL can be accessed using RED::IWindow::GetDefaultVRL
. This default VRL is associated with the onscreen rendering buffer of the application window specified at the HOOPS Luminate window creation. This means that the data rendered by this VRL will be displayed in the application window display area - if the application has a screen display, of course.
An auxiliary VRL must be created using RED::IWindow::CreateVRL
and accessed using RED::IWindow::GetVRL
. The contents of an auxiliary VRL is rendered into an OpenGL offscreen rendering buffer. Depending on the hardware driver, GPU and operating system, this may be an OpenGL p-buffer or an OpenGL Framebuffer object.
If HOOPS Luminate runs in software mode, all the VRLs are rendered in software into the CPU memory. If HOOPS Luminate runs in hybrid modes, all the VRLs are rendered in hardware into the GPU memory.
Rendering Methods
The RED::IWindow
interface provides all the HOOPS Luminate rendering methods. A rendering method is one of the ‘RED::IWindow::FrameXXX’ methods that can be found in the method’s listing of the interface. Any rendering method will render all the VRLs of the window in sequence, from the last VRL to the first VRL (the default VRL).
We have three different categories of rendering methods:
RED::IWindow::FrameDrawing
: This is the hardware rendering call used to render a window contents using the GPU
RED::IWindow::FrameTracing
,RED::IWindow::FrameTracingImages
,RED::IWindow::FrameTracingImageLayers
,RED::IWindow::FrameTracingGICache
: All these rendering methods will render all ray-traced viewpoints found in all VRLs using HOOPS Luminate’s software ray-tracer, and will render all non ray-traced viewpoints found using hardware rendering
RED::IWindow::FramePicking
andRED::IWindow::FramePickingRectangle
: these are two pure CPU rendering calls (they don’t do any GPU operation) that perform picking inside the window contents to find which data is hit by a picking ray or rectangular area