Updating a Geometry
We saw in a previous tutorial - Building a Geometry - Part 1 - Loading a Source Scene - how to create a geometry (ART::IGeometry
) from a source HOOPS Luminate scene graph.
In this tutorial, we will show how to update the geometry to reflect the changes made in the source scene graph.
This tutorial uses the same desert landscape as in the previous tutorials. See ART::IAssetManager::LoadAtlas
.
As a first step, a geometry is created from a given scene root. The scene contains a ground plane and two shapes: a blue semi-transparent sphere and a red cube.
// Build a basic RED scene.
// g_geometry_root is the returned graph root.
RC_TEST( BuildScene( g_geometry_root ) );
// Create a geometry reflecting the scene.
// g_geometry is the returned geometry.
RC_TEST( iassetmgr->CreateGeometry( g_geometry, g_geometry_root, RED::LayerSet::ALL_LAYERS, excludedNames, g_id2names, false, true, REDartProgressCB, NULL ) );
The geometry have to be added to the planet:
// The geometry is added to the planet.
RC_TEST( iearth->AddGeometry( g_geometry, g_matx ) );
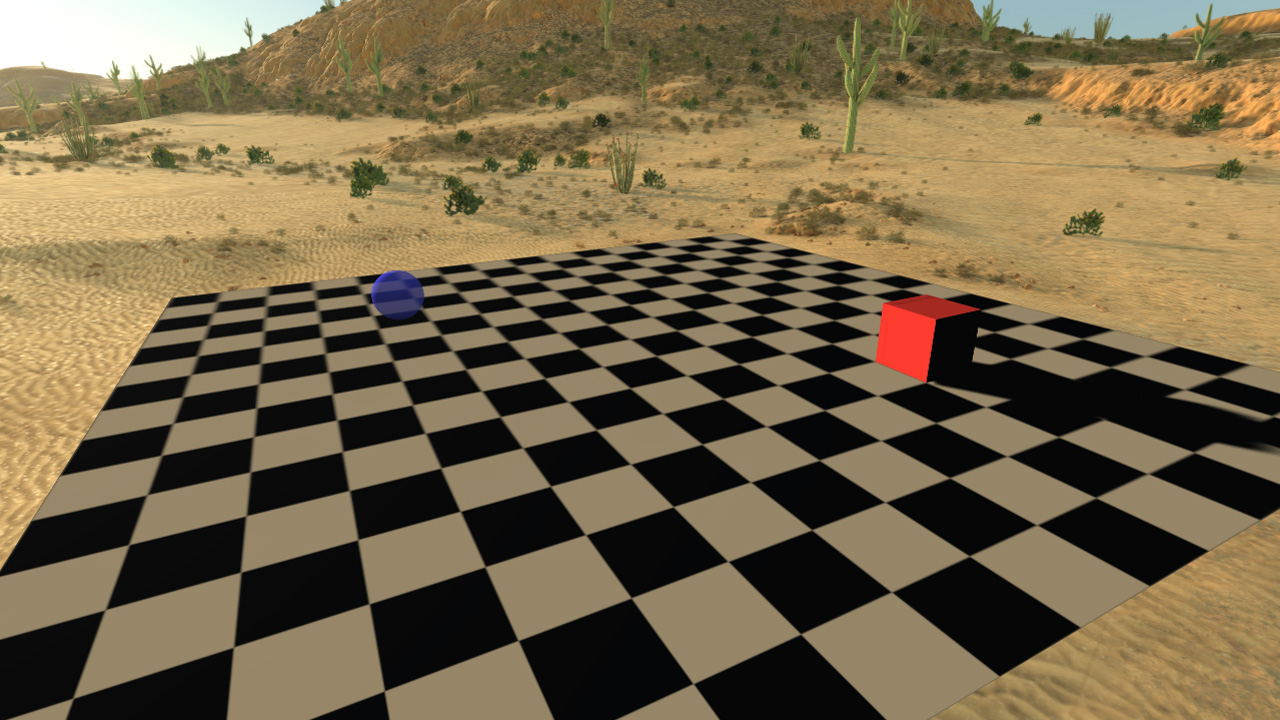
the geometry as defined in the source graph
Now that the geometry is created and visible on the world. We want to move its parts without having to recreate a geometry from scratch. This is possible thanks to the ART::IAssetManager::UpdateGeometryPartTransform
method.
This method takes as parameters:
- the geometry that we want to update
- the source graph where a
RED::ITransformShape
has been modified- the exact node in the graph that we want to update
In the sample, we translate the sphere node and update the geometry accordingly:
// Translate the source graph node matrix and update the geometry.
RED::Matrix* pmtx;
RED::ITransformShape* itnode = node->As< RED::ITransformShape >();
RC_TEST( itnode->GetMatrix( pmtx, iresmgr->GetState() ) );
if( pmtx != NULL )
{
pmtx->Translate( RED::Vector3( 0.0, 1.0, 0.0 ) );
RC_TEST( iassetmgr->UpdateGeometryPartTransform( g_geometry, g_geometry_root, node ) );
}
In the same way, a similar method allows to hide the geometry parts: ART::IAssetManager::SetGeometryPartVisible
.
// Change the node visibility and update the geometry.
RC_TEST( iassetmgr->SetGeometryPartVisible( g_geometry, g_geometry_root, node, visible[ objId ], visible[ objId ] ) );
With this method, you can hide a part of the graph, with option to hide shadows or not.
Note
The geometry part is not removed from the system, therefore hidding shapes will not speed up the rendering.
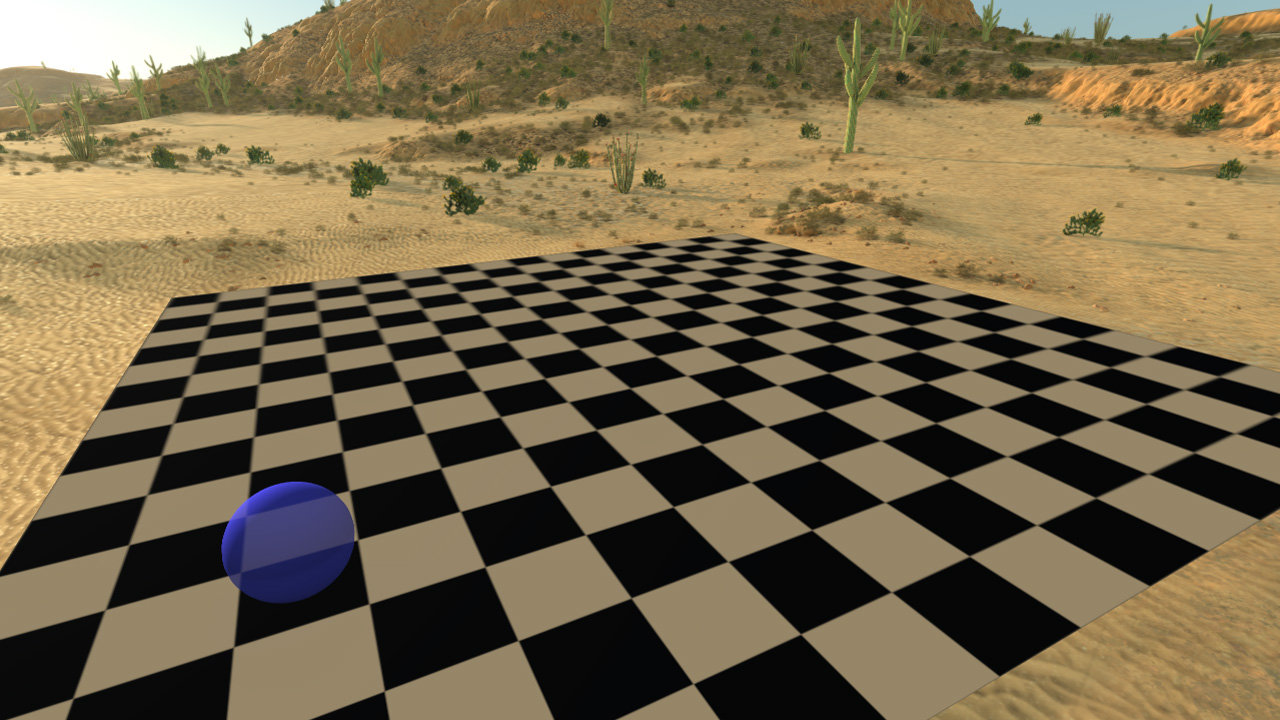
The geometry after the sphere node translation and the cube node has been hidden