Setup a Mesh Geometry Channels
Here, we define a simple plane as shown below:
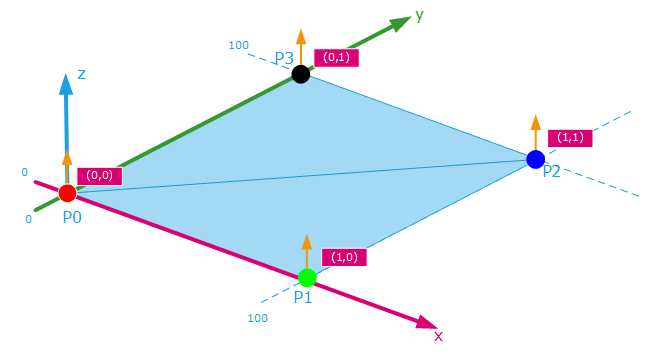
And the code sequence to realize it is:
// Creating our mesh shape:
RED::Object* mesh = RED::Factory::CreateInstance( CID_REDMeshShape );
if( !mesh )
RC_TEST( RED_ALLOC_FAILURE );
RED::IMeshShape* imesh = mesh->As< RED::IMeshShape >();
// We want to create a plane in this example: 4 vertices, with: positions, normals, vertex colors and texture coordinates:
float position[12] = { 0.0f, 0.0f, 0.0f,
100.0f, 0.0f, 0.0f,
100.0f, 100.0f, 0.0f,
0.0f, 100.0f, 0.0f };
float normal[12] = { 0.0f, 0.0f, 1.0f,
0.0f, 0.0f, 1.0f,
0.0f, 0.0f, 1.0f,
0.0f, 0.0f, 1.0f };
unsigned char color[16] = { 255, 0, 0, 255,
0, 255, 0, 255,
0, 0, 255, 255,
0, 0, 0, 255 };
float uv[8] = { 0.0f, 0.0f,
1.0f, 0.0f,
1.0f, 1.0f,
0.0f, 1.0f };
RC_TEST( imesh->SetArray( RED::MCL_VERTEX, position, 4, 3, RED::MFT_FLOAT, iresmgr->GetState() ) );
RC_TEST( imesh->SetArray( RED::MCL_NORMAL, normal, 4, 3, RED::MFT_FLOAT, iresmgr->GetState() ) );
RC_TEST( imesh->SetArray( RED::MCL_COLOR, color, 4, 4, RED::MFT_UBYTE, iresmgr->GetState() ) );
RC_TEST( imesh->SetArray( RED::MCL_TEX0, uv, 4, 2, RED::MFT_FLOAT, iresmgr->GetState() ) );
// Then we need to define our mesh triangles:
int index[6] = { 0, 1, 2,
0, 2, 3 };
RC_TEST( imesh->AddTriangles( index, 2, iresmgr->GetState() ) );