Setup a Beam Light
A beam light is like a directional one, it emits light along a light direction but has a finite radial extension defined by an inner radius and an outer radius. Contrary to directional light, beam light can cast shadow maps.
Like all the lights, the first thing to do is to create it:
// Create the light shape:
RED::Object* light = RED::Factory::CreateInstance( CID_REDLightShape );
if( light == NULL )
RC_TEST( RED_ALLOC_FAILURE );
RED::ILightShape* ilight = light->As< RED::ILightShape >();
Then we have to define it as a beam light:
// The beam light parameters:
RED::Vector3 position = RED::Vector3( 20.0, 20.0, 60.0 );
RED::Vector3 direction = RED::Vector3::ZERO - position;
RED::Vector3 up = RED::Vector3::ZAXIS;
RED::Color diffuse = RED::Color::WHITE;
RED::Color specular = RED::Color::WHITE;
float in_radius = 10.0f;
float out_radius = 2.0f;
float att_values[7] = { 1.f, 0.f, 0.f, 0.f, 0.f, 0.f, 0.f };
// Define the light as a beam light:
RC_TEST( ilight->SetBeamLight( RED::ATN_NONE, att_values, position, direction, up, diffuse, specular, in_radius, out_radius, iresmgr->GetState() ) );
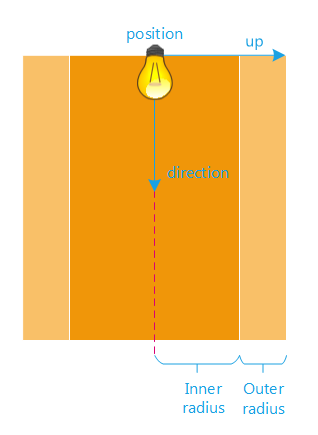
Beam light parameters
See the Decay Equations chapter to learn about light attenuation modes and settings.
After the light as been created, you can set some RED::RENDER_MODE
and shadow parameters. Shadow parameters include:
- shadow color
- shadow map resolution
- shadow map bias
- shadow map blur
- etc.
// Set the render modes:
RC_TEST( ilight->SetRenderMode( RED::RM_SHADOW_CASTER, 1, iresmgr->GetState() ) );
// Set the shadow parameters:
RC_TEST( ilight->SetShadowMapResolution( 1024, iresmgr->GetState() ) );
And finally, we have to add it to the viewpoint:
// Add the scene data to the viewpoint:
RC_TEST( icamera->AddShape( light, iresmgr->GetState() ) );