Setup a Spot Light
Spot lights are the basic lights in HOOPS Luminate. Like every light, the spot light is created using the RED::Factory
and the RED::ILightShape
interface.
// Create the light shape:
RED::Object* light = RED::Factory::CreateInstance( CID_REDLightShape );
if( light == NULL )
RC_TEST( RED_ALLOC_FAILURE );
RED::ILightShape* ilight = light->As< RED::ILightShape >();
The second step is to define the light as a point light, then as a spot light. All the specific light parameters are set during this step.
// The spot light parameters:
RED::Vector3 position = RED::Vector3( 20.0, 20.0, 60.0 );
RED::Vector3 direction = RED::Vector3::ZERO - position;
RED::Vector3 up = RED::Vector3::ZAXIS;
RED::Color diffuse = RED::Color::WHITE;
RED::Color specular = RED::Color::WHITE;
float spot_angle = RED_PI / 6.0f;
float spot_falloff = 0.2f;
float spot_dropoff = 0.0f;
float att_values[7] = { 1.f, 0.f, 0.f, 0.f, 0.f, 0.f, 0.f };
// Define the light as a point light and spot light:
RC_TEST( ilight->SetPointLight( RED::ATN_NONE, att_values, position, diffuse, specular, iresmgr->GetState() ) );
RC_TEST( ilight->SetSpotLight( position, direction, up, spot_angle, spot_falloff, spot_dropoff, iresmgr->GetState() ) );
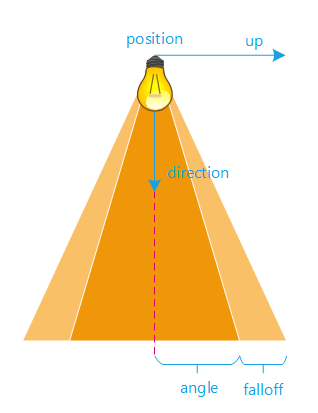
Spot light parameters
See the Decay Equations chapter to learn about light attenuation modes and settings.
After the light as been created, you can set some RED::RENDER_MODE
and shadow parameters. Shadow parameters include:
- shadow color
- shadow map resolution
- shadow map bias
- shadow map blur
- etc.
// Set the render modes:
RC_TEST( ilight->SetRenderMode( RED::RM_SHADOW_CASTER, 1, iresmgr->GetState() ) );
// Set the shadow parameters:
RC_TEST( ilight->SetShadowMapResolution( 1024, iresmgr->GetState() ) );
Finally the light can be added to the camera scene graph.
// Add the scene data to the viewpoint:
RC_TEST( icamera->AddShape( light, iresmgr->GetState() ) );