Building a Geometry - Part 1 - Loading a Source Scene
This tutorial will explain how to load an existing RED scene graph and convert it to a correct REDart geometry. The Importing Geometries tutorial shows how to load an already setup geometry. This one uses raw RED data and build the geometry.
The process is done in two steps:
// Loading our geometry. Our scene is an old model, whose unit is ~2cm and our world is in meters:
g_convertMatrix.Scale( 0.02 );
RC_TEST( iassetmgr->LoadGeometrySourceFile( g_geometry_root, g_context, g_id2names, "../Resources/House_Source.red", g_convertMatrix, RED::LayerSet::ALL_LAYERS ) );
RED::Vector< RED::String > excludedNames;
RC_TEST( excludedNames.push_back( "Portal" ) );
RC_TEST( iassetmgr->CreateGeometry( g_geometry, g_geometry_root, RED::LayerSet::ALL_LAYERS, excludedNames, g_id2names, false, true, REDartProgressCB, NULL ) );
The method ART::IAssetManager::LoadGeometrySourceFile
loads a RED file and apply unit and axis conversion.
The first method can be skipped if you already have a valid RED scene graph.
The second method: ART::IAssetManager::CreateGeometry
, creates an optimized geometry object from the RED scene graph. The resulting object implements the ART::IGeometry interface
.
The method does all of this:
- The meshes are compressed into a minimum of unified big meshes
- The material parameters are extracted and used to build a unified realistic material suited for REDart
- The scene graph is flattened in a unique transform shape for performance
- The lights are automatically extracted and setup from the input data (see Building a Geometry - Part 3 - Adding Lights)
Note
The source images properties like wrap modes must be consistent with the local storage of pixels. If there are data in the local pixel storage, then the local properties must be set (RED::IImage::SetLocalWrapModes
).
Once calling this method, we can add the newly created geometry to the scene:
// Setup the geometry positioning matrix.
const RED::Matrix& cmatx1 = cameras[ 1 ]->As< ART::ICamera >()->GetMatrix();
cpos = cmatx1.GetTranslation();
RC_TEST( iearth->GetTangentAxisSystem( g_matx, &( cpos._x ) ) );
RC_TEST( iearth->AddGeometry( g_geometry, g_matx ) );
Note
A geometry can be destroyed using ART::IAssetManager::DeleteGeometry
. The geometry is not deleted immediately because of the multithreading. It will be deleted during the next call to ART::IWorld::Update
. The geometry can be updated by calling ART::IPlanet::RefreshGeometry
.
Currently, the geometry lighting is not very good. It is because the geometry does not have global illumination information yet. It will be built in the Building a Geometry - Part 2 - Setting Up a GI Cache tutorial.
All the lights are turned off. The Building a Geometry - Part 3 - Adding Lights tuturial will explain how to configure them.
There are no reflections neither. While the reflection probes data are extracted from the original graph, the environment maps need to be rendered. This will be done in the Building a Geometry - Part 4 - Adding Reflection Probes tutorial.
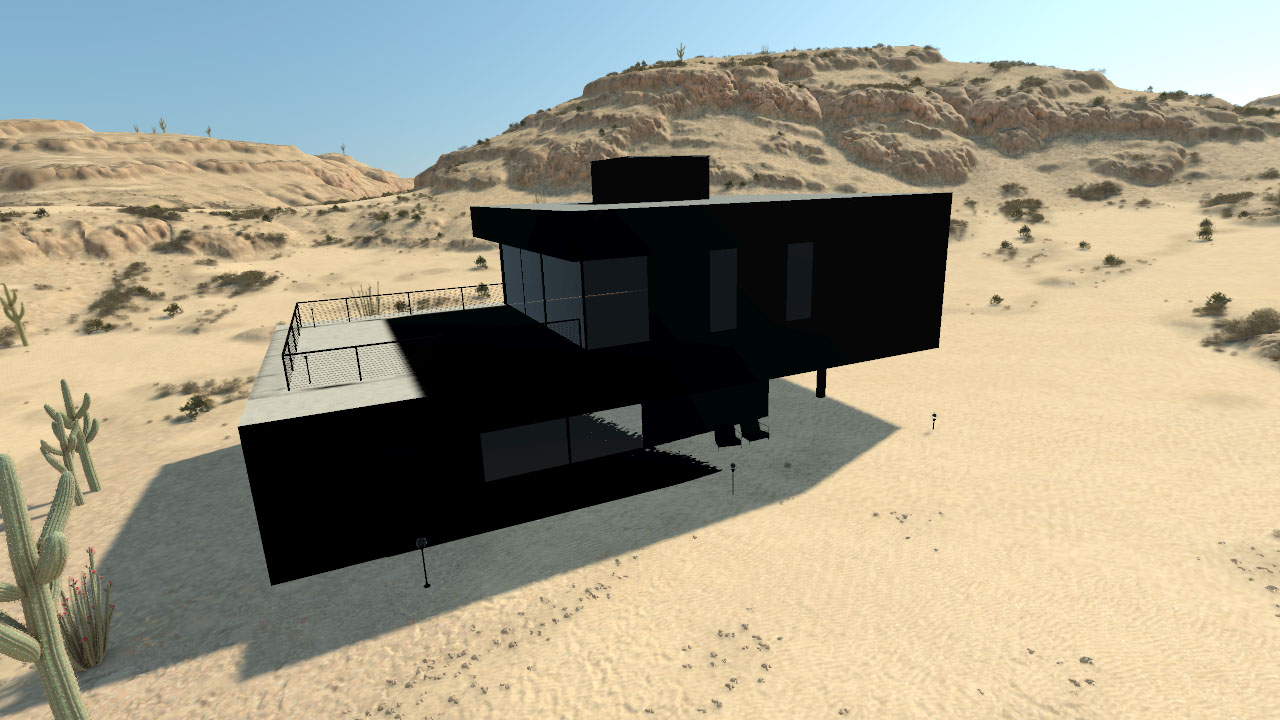
The house lighting is poor and the ground is incorrect